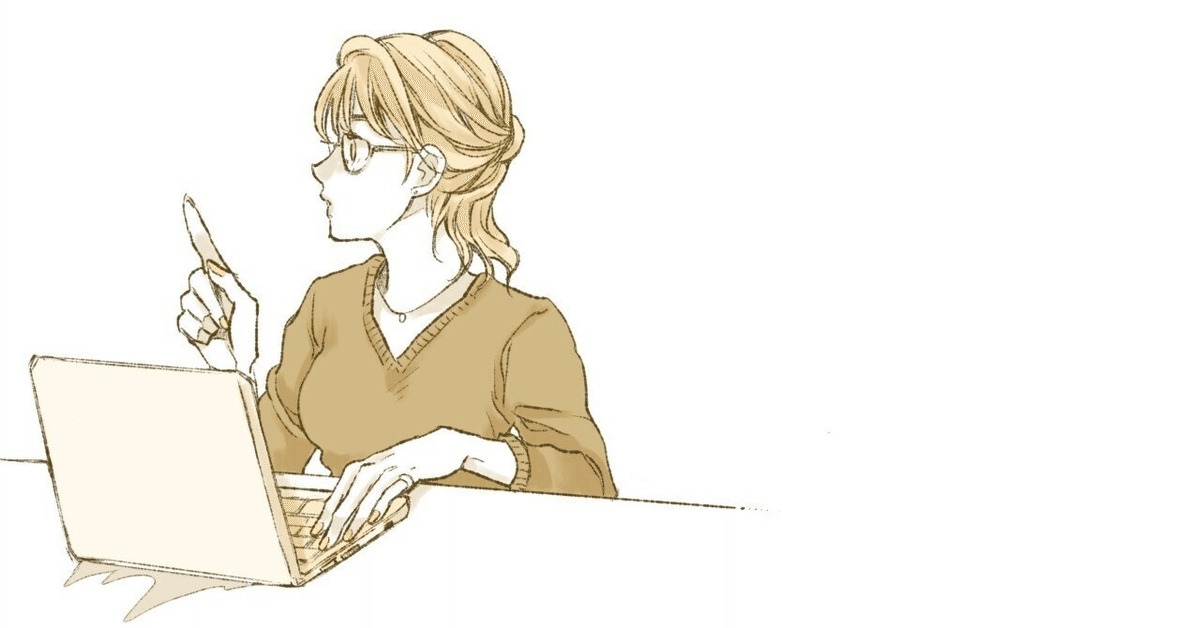
Photo by
aoming
【Python】サクッとメモアプリを作る
必要なライブラリのインストール
以下のコマンドを実行します。
$ pip install PySimpleGUI
ソースコード
以下のコードをコピペします。
import PySimpleGUI as sg
# メモ保存関数
def save_note(title, content):
if not title or not content:
sg.popup("エラー", "タイトルと内容を入力してください")
return
with open(f"{title}.txt", "w", encoding="utf-8") as file:
file.write(content)
sg.popup("保存完了", f"メモ '{title}' を保存しました!")
# GUIのレイアウト
layout = [
[sg.Text("タイトル")],
[sg.Input(key="-TITLE-", size=(40, 1))],
[sg.Text("内容")],
[sg.Multiline(key="-CONTENT-", size=(40, 10))],
[sg.Button("保存"), sg.Button("終了")]
]
# ウィンドウの作成
window = sg.Window("メモアプリ", layout)
# イベントループ
while True:
event, values = window.read()
if event == sg.WIN_CLOSED or event == "終了":
break
if event == "保存":
title = values["-TITLE-"]
content = values["-CONTENT-"].strip()
save_note(title, content)
window.close()
実行
メモの入力: タイトルと内容を入力します。
メモの保存: 「保存」ボタンを押すと、タイトル.txt の形式で保存されます。
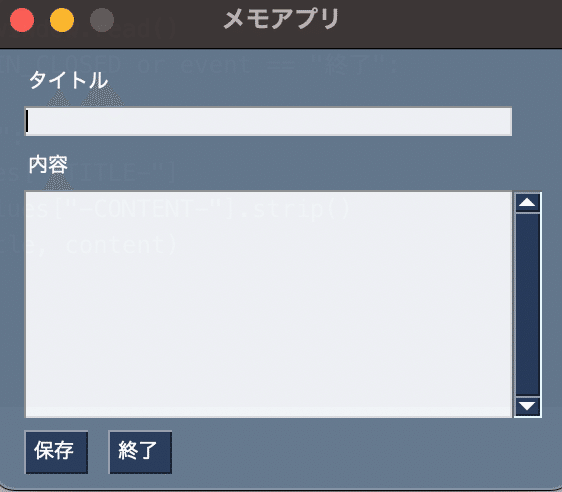