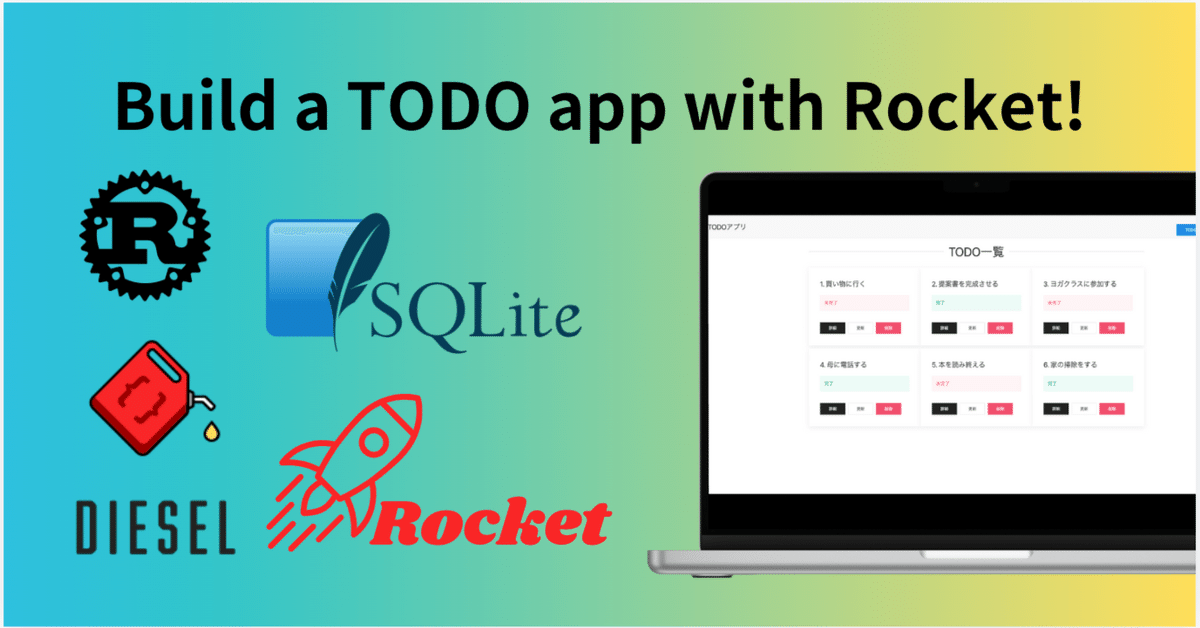
Let's build a TODO app using the Rust framework Rocket!
Have you heard of Rocket, a framework that makes it easy to build web apps in Rust? This framework can be developed like Python's “Flask” or “Django”.
Rocket is recommended for building web apps in Rust because of its fast processing speed.So in this article, we will actually create an app in Rocket using Rust, HTML, CSS, and Sqlite.
This article will help you master the three techniques below!
Can build simple apps with Rust and Rocket
Be able to build MVT model apps
Can learn how to implement the Repository pattern
The first half of the article will introduce the basic usage of Rocket and its powerful features. It will explain in detail in what situations it is suitable for use. The second half of the article will be an actual experience of developing and deploying a TODO application in order to learn Rust.
If you are interested in web development using Rust, please take this opportunity to try your hand at Rocket!
What is Rocket?
Rocket features an API designed to be easy to use and intuitive; it is also highly secure, taking advantage of Rust's type system. Below is a detailed description of Rocket's key features and use cases.
Rocket Features
Intuitive Routing
Routing is very easy to define because you can directly define functions for HTTP requests using Rust macros. Request methods and URL paths can be easily specified with macros, allowing development to proceed with a high degree of readability.
Safety by utilizing the type system
When compiling, request data is checked using Rust's powerful type system for automatic parsing and validation of request data. For example, form data and JSON requests are automatically parsed according to type, allowing you to write safe code.
State Management
Rocket makes it easy to manage global state (data shared throughout your app). This makes it easier to handle database connections, configuration files, authentication information, etc. throughout your app.
Rocket Use Cases
Small to medium scale web apps
The intuitive routing and request processing mechanisms allow web apps to be built with very concise code. Developers can quickly understand how to use the framework and quickly implement it, especially when creating small projects.
Fast Development Cycle: “Develop secure web apps quickly.
Rocket's design philosophy of “developing secure web apps quickly” makes it easy to write simple and readable code. This makes it an easy framework to adopt for team development and beginners.
Simple Web API
It is also ideal for building simple APIs. Route definition and data validation are easy, and prototypes can be developed quickly.
Comparison of Rocket with other frameworks
For your information, here is a table comparing the frameworks mainly used in Rust.
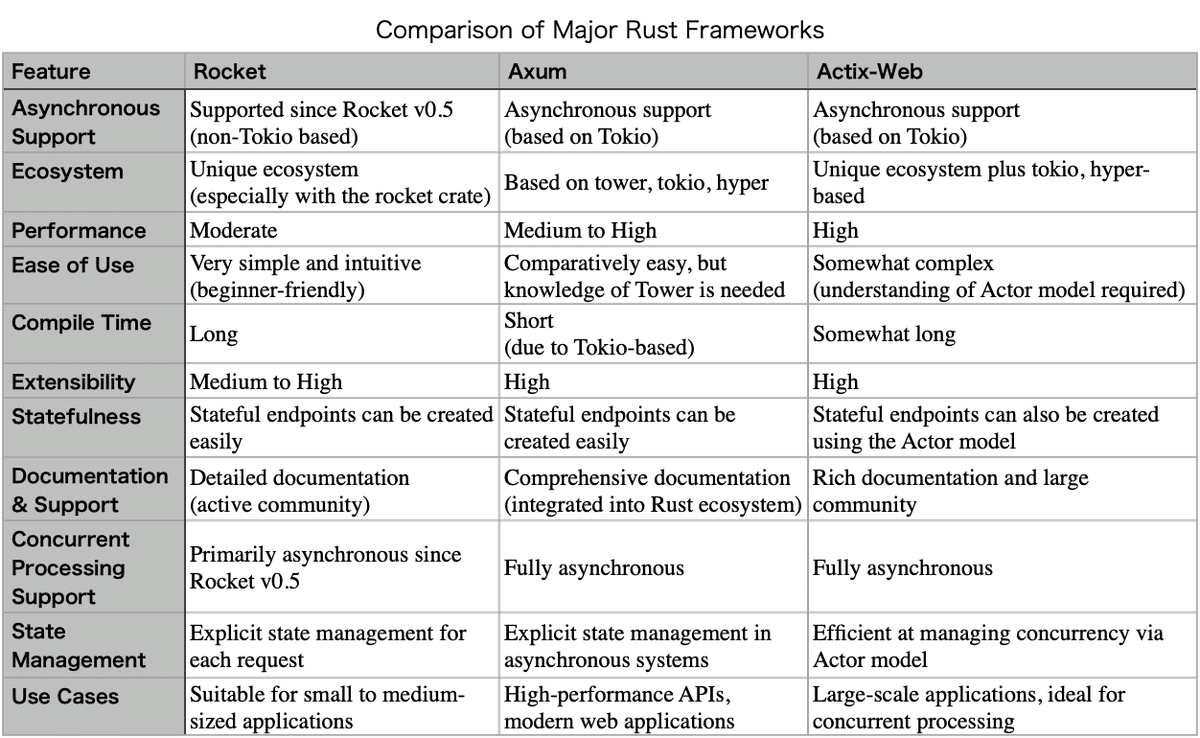
It is the latest framework of AxumRust and specializes in Tokio-based asynchronous processing. Its simple and flexible design makes it easy to implement asynchronous functions and middleware as handler functions. It is also scalable and extensible.
Actix-WebHigh performance asynchronous web framework. It can be used for parallel processing and
TODO App Development
From this point on, we will actually develop TODO apps by hand.
Application Overview
A simple TODO list management tool. You can view a list of tasks, check their details, and update and edit them.
On the list screen, you can check all TODOs at a glance, and on the detail screen, you can check the description of each task and the date it was registered. You can also modify the contents of tasks on the update screen.
Technologies Used
Rocket (Rust)
Rocket, a lightweight and flexible framework for Rust, is used to build server-side logic.SQLiteSQLite
A simple and lightweight relational database, is used for data storage. Data can be easily managed in a local environment.DieselDiesel
A powerful library for Rust, is used for database connection and ORM (object-relational mapping). Data manipulation is streamlined through type-safe queries.UIkitFront-end
UI uses UIkit, a CSS framework that provides simple, sophisticated design and easy-to-use components.
Implementing with the MVT Model
The MVT model (Model-View-Template) is one of the most commonly used software design patterns when implementing in a web framework. It is especially used in web frameworks such as Django.
Why use the MVT model?
Simple Structure MVT organizes the structure of your app and clarifies the division of code responsibilities. Models, views, and templates have their own roles, making development easier.
MaintainabilityBecause each part is independent, models linked to the DB are less susceptible to changes in views and templates.
Optimizing the systemThe MVT model allows efficient application construction because of the division of roles.
Each of them is divided into roles to build the system.
Overall diagram
The file we are going to implement can be applied to the MVT model as follows.
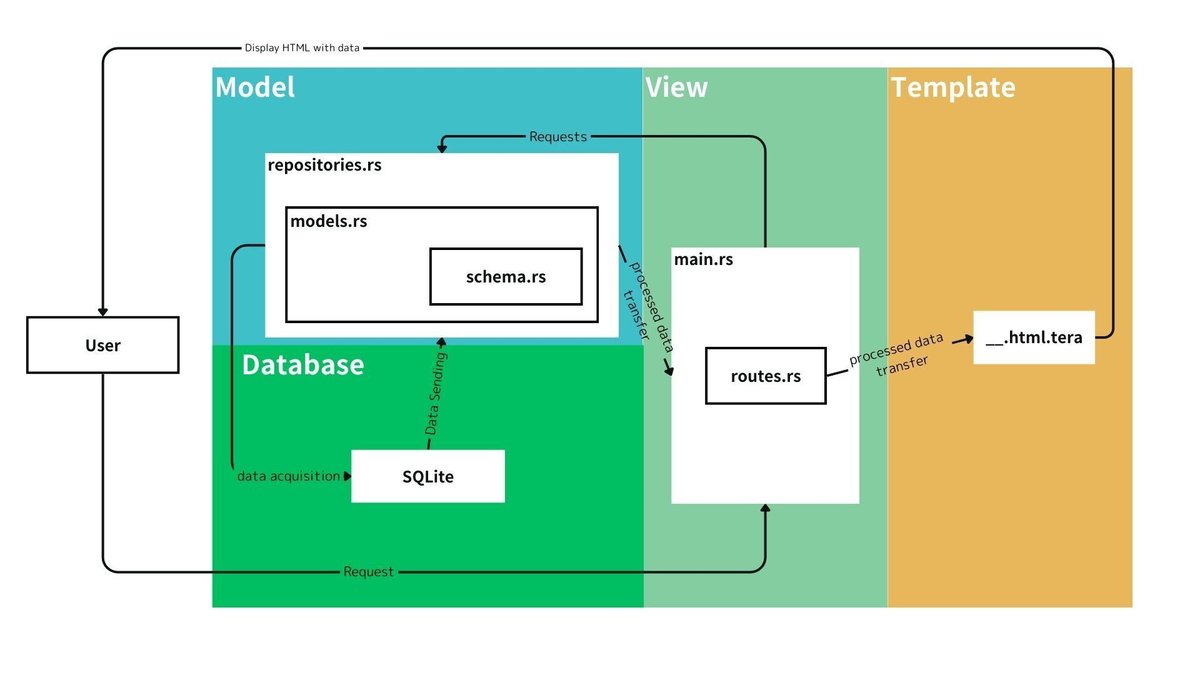
User RequestA user accesses the TODO application and sends a request from the browser to the server.
ViewThe server accesses the model to retrieve the necessary data based on the request.
Upon request from the Model view, the Model retrieves the required data from the database, SQLite, and returns it to the View.
The View view processes the data received from the Model and passes it to the Template.
The template embeds the data passed from the view into HTML and generates a web page for the user to view.
The response to the user is the HTML generated by the template, which is returned to the user and displayed in the browser.
Initial Setup
The first step is to create a project.
Create a folder
On the desktop, create a project using “cargo”.
shell
~/Desktop $ cargo new rust_todo
~/Desktop $ cd rust_todo
Library Settings
Configure rocket in Cargo.toml. The libraries set here will automatically download the dependency crates when the Rust project is built and include them in the project.
Cargo.toml
...
[dependencies]
rocket = "0.5.0-rc.3"
Write the code to build the web server in main.rs.
src/main.rs
#[macro_use]
extern crate rocket;
#[get("/")]
fn index() -> &'static str {
"Hello world"
}
#[rocket::main]
async fn main() -> Result<(), rocket::Error> {
let _rocket = rocket::build().mount("/", routes![index]).launch().await?;
Ok(())
}
#[macro_use] extern crate rocket;
Declaration for using Rocket framework features. #[macro_use] to use Rocket macros such as “routes!”
#[get(“/”)]
Defines a route handler to handle HTTP GET requests. The “/” indicates a request for the root URL (top page).
fn index() -> &'static str { “Hello world” }
If a GET request comes to “/”, the function index is called and returns a &'static str type. In this case, “Hello world” is returned. As a result, “Hello world” is displayed when the top page is accessed.
#[rocket::main] async fn main() -> Result<(), rocket::Error>
#[rocket::main] is a macro that defines an asynchronous entry point. This main function is the entry point for the application, and async is used to perform asynchronous processing. The return value is Result<(), rocket::Error>, which returns Ok() for a successful completion or rocket::Error if an error occurs.
rocket::build().mount(“/”, routes![index])
rocket::build() creates an app instance of Rocket. mount(“/”, routes![index]) mounts the index function as a route for the URL path “/”. In other words, the index function is set to be executed when the top page is accessed.
.launch().await?
This part launches the app. launch() is an asynchronous function that launches the Rocket's server and waits for its result using await. If an error occurs, “?” is used to propagate the error to the caller. to propagate the error to the caller.
Ok(())
If the application starts successfully, this line returns Ok(()) and terminates the main function.
After implementation is complete, run the program in a terminal.
~/Desktop/rust_todo $ cargo run
...
🛡️ Shield:
>> Permissions-Policy: interest-cohort=()
>> X-Content-Type-Options: nosniff
>> X-Frame-Options: SAMEORIGIN
🚀 Rocket has launched from http://127.0.0.1:8000
The text “Hello world” will appear on the web at http://127.0.0.1:8000.
db setup
diesel setup
Add diesel to cargo.toml so that it can connect to sqlite. diesel is Rust's ORM framework and allows for easy database manipulation using type-safe queries.
diesel is an ORM framework for Rust that allows for easy database manipulation using type-safe queries. sqlite is an embedded, lightweight database used especially in small apps and development environments. to easily read, write, and manage databases.
ここから先は
¥ 500
サポートよろしくお願いいたします! いただいたサポートの一部ははクリエイターとしての活動費に使わせていただきます! ※ サポートの一部は子供たちの教育などの団体に寄付する予定です。