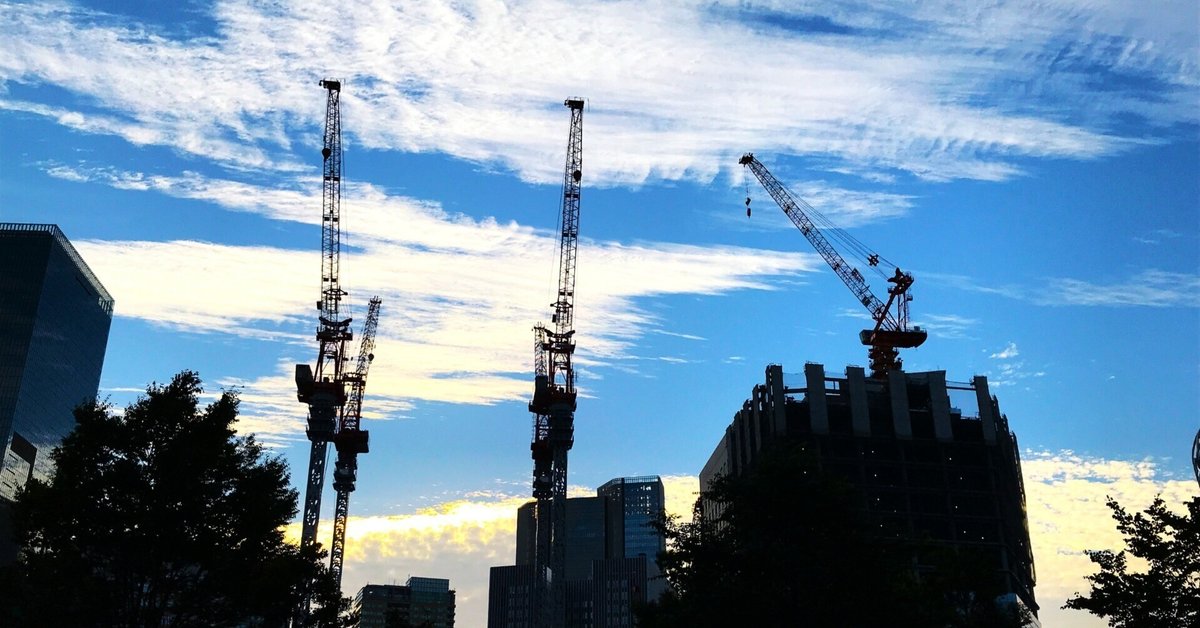
プログラミング学習の記録 #004(C)
今年の11月は、11月祭の準備があって非常に忙しく、思ったように学習を進めることができなかった。最近、やっと事後の事務処理も終えて、ようやく学習を進めることができるようになった。当初の予定よりもだいぶ遅れているので割と焦ってきたが、悪い焦りは抑えて、しっかりと着実に進めていきたいところである。
前回の復習
教科書に練習問題がいくつか記載されていたので、それに沿って、コードを書いてみた。
練習問題 3.4
「標準体重」にはいろいろな計算方法が提案されている。身長をキーボードから入力して
$$
(\mathrm{身長}[\mathrm{cm}] - 100) \times 0.9 \\
(\mathrm{身長}[\mathrm{m}])^{2} \times 22
$$
により定義される二種類の標準体重を計算して表示するプログラムを作成せよ。
#include <stdio.h>
#include <math.h>
int main()
{
double he, nwe1, nwe2;
printf("Input your height[cm]: ");
scanf("%lf",&he);
nwe1 = ( he - 100 ) * 0.9 ;
nwe2 = pow( he / 100 , 2) * 22;
printf("Your standard weight is %5.2f or %5.2f .\n",nwe1,nwe2);
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
途中、数値の2乗を計算するために、このサイトを参照して、「pow」を用いた。結果を表示する「printf」において、「%5.2f」とあるが、これは、数値の桁数を指定するものである。これについては、このサイトを参照した。
練習問題 3.5
野球選手の打撃成績の一つ「長打率」は、
$$
長打率
= \frac{単打数 + 二塁打数 \times 2 + 三塁打数 \times 3 + 本塁打数 \times 4 }{打数}
$$
により定義される。安打数(= 単打数 + 二塁打数 + 三塁打数 + 本塁打数)、二塁打数、三塁打数、本塁打数と打数を読み込んで、長打率を計算するプログラムを作成せよ。
#include <stdio.h>
#include <math.h>
int main()
{
int h_all, h1, h2, h3, h4;
double bats, per_l;
printf("Input the number of At-Bat: ");
scanf("%lf",&bats);
printf("Input the number of Hit: ");
scanf("%d",&h_all);
printf("Input the number of Double: ");
scanf("%d",&h2);
printf("Input the number of Triple: ");
scanf("%d",&h3);
printf("Input the number of Home Run: ");
scanf("%d",&h4);
h1 = h_all - h2 - h3 - h4 ;
per_l = (h1 + (h2 * 2) + (h3 * 3) + (h4 * 4) ) / bats ;
printf("Your slugging percentage is %4.3f .\n", per_l);
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
練習問題 3.6
二つの空間ベクトルを入力し、内積と外積ベクトルを計算するプログラムを作成せよ。
#include <stdio.h>
#include <math.h>
int main()
{
double a1, a2, a3;
double b1, b2, b3;
double dot;
double cross1, cross2, cross3;
printf("About vector A.\n");
printf("x: ");
scanf("%lf",&a1);
printf("y: ");
scanf("%lf",&a2);
printf("z: ");
scanf("%lf",&a3);
printf("About vector B.\n");
printf("x: ");
scanf("%lf",&b1);
printf("y: ");
scanf("%lf",&b2);
printf("z: ");
scanf("%lf",&b3);
dot = a1 * b1 + a2 * b2 + a3 * b3 ;
cross1 = a2 * b3 - a3 * b2 ;
cross2 = a3 * b1 - a1 * b3 ;
cross3 = a1 * b2 - a2 * b1 ;
printf("Dot product is %6.2f.\n", dot);
printf("Cross product is ( %6.2f, %6.2f, %6.2f ).\n", cross1, cross2, cross3);
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
スニペット(余談)
このサイトを参照した。「first」という名称で以下のようなコードを呼び出せるように設定した。
#include <stdio.h>
#include <math.h>
int main()
{
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
見ての通り、このコード自体には、特に意味はない。
if
教科書を参考にしつつ、2個の数を入力し、分母の数が0でないときにのみ、割り算を実行して表示するようなプログラムコードを書いた。
#include <stdio.h>
int main()
{
double n1, n2;
printf("Input the number1: ");
scanf("%lf",&n1);
printf("Input the number2: ");
scanf("%lf",&n2);
if(n2 != 0)
{
printf("number1 / number2 = %6.3f\n", n1/n2);
}
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
「if(n2 != 0)」によって、n2が0でないときにのみ、「{ }」内のprintfが実行されるということである。実際、 n2 = 0 を入力したところ、「HAPPY SMILE (^_^)v」が表示されてプログラムが終了したが、 n2 = 0 でない数を入力したところ、「number1 / number2 = 1.857」のように、割り算の計算結果が表示された。
if … else
こちらも教科書を参考にしつつ、同様のコードを書いた。
#include <stdio.h>
#include <math.h>
int main()
{
double n1, n2, n3;
printf("Input the number1: ");
scanf("%lf",&n1);
printf("Input the number2: ");
scanf("%lf",&n2);
if(n2 == 0)
{
printf("You cannot divide by 0.\n");
}else
{
n3 = n1 / n2 ;
printf("number1 / number2 = %6.3f\n", n3);
}
printf("HAPPY SMILE (^_^)v\n");
return 0;
}
先ほどのコードと異なる点は、number2に0を入力したときに、計算を行わずに「You cannot divide by 0.」と表示する点である。Terminal上で急にプログラムが終了しないので、先ほどのコードよりも親切なコードである。
関係演算子
忘れたときのためにまとめておく。
a == b 等しい
a != b 等しくない
a < b
a > b
a <= b
a >= b
-----
動け!タイムライン
いいなと思ったら応援しよう!
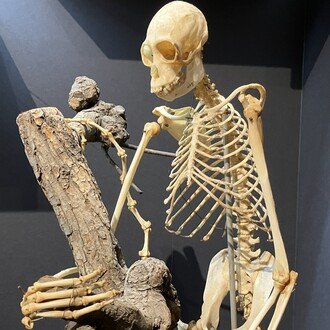