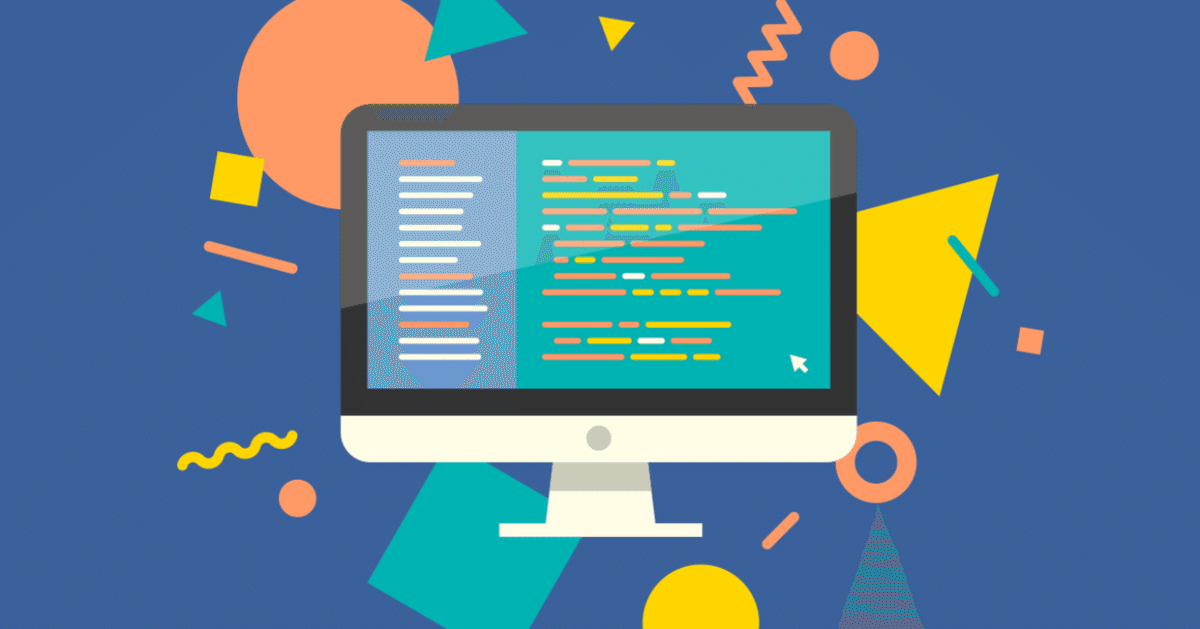
Photo by
peishum
Nuxt.jsでCloud FirestoreやCloud Storageを使う
Webアプリを開発したい場合、フロントエンドでNuxt.js、バックエンドでFirebaseを利用する組み合わせが非常に手軽で強力である。本記事では、NoSQLなデータベースであるCloud Firestore、ファイルストレージであるCloud Storageの基本的な使い方についてまとめる。
Firebaseの導入
こちらのページに沿って必要なパッケージをインストールして、nuxt.config.jsで設定を行うことで簡単にFirebaseを導入できる。
// firebaseをインストール
npm install firebase
// Nuxt用のモジュールをインストール
npm install @nuxtjs/firebase
// nuxt.config.js
modules: [
[
'@nuxtjs/firebase',
{
// Firebaseのプロジェクト設定(マイアプリ > SDKの設定と構成)を参照
config: {
apiKey: '<apiKey>',
authDomain: '<authDomain>',
projectId: '<projectId>',
storageBucket: '<storageBucket>',
messagingSenderId: '<messagingSenderId>',
appId: '<appId>',
measurementId: '<measurementId>'
},
// 必要なサービスを設定する
services: {
auth: true,
firestore: true,
functions: false,
storage: true,
database: false,
messaging: false,
performance: false,
analytics: false,
remoteConfig: false
}
}
]
],
Cloud Firestore
基本的なドキュメント登録・更新・削除・取得方法は以下の通り。
※詳細な使い方はFirebaseのドキュメントを参照
// ドキュメント登録(ドキュメントIDが自動で付与される)
this.$fire.firestore.collection(<コレクション名>).add({
// ドキュメントオブジェクト
}).then(response => {
// 登録成功時の処理
}).catch(error => {
// 登録失敗時の処理
})
// ドキュメント更新(指定IDのドキュメントが存在しない場合は新規作成される)
this.$fire.firestore.collection(<コレクション名>).doc(<ドキュメントID>).set({
// ドキュメントオブジェクト
}).then(response => {
// 更新成功時の処理
}).catch(error => {
// 更新失敗時の処理
})
// ドキュメント削除
this.$fire.firestore.collection(<コレクション名>).doc(<ドキュメントID>).delete().then(response => {
// 削除成功時の処理
}).catch(error => {
// 削除失敗時の処理
})
// ドキュメント取得
this.$fire.firestore.collection(<コレクション名>).doc(<ドキュメントID>)
.where("<論理型プロパティ>", "==", "true") // 指定の論理型プロパティがtrueの場合
.where("<配列型プロパティ>", "array-contains", "<アイテム>") // 指定の配列型プロパティにアイテムが含まれる場合
.where("<数値型プロパティ>", ">", "<数値>") // 指定の数値型プロパティが指定値より大きい場合
.orderby("<プロパティ>", "desc") // 指定プロパティで昇順(asc)または降順(desc)で並び替え
.limit(<上限値>) // 取得件数の上限数
.get() // 取得
.then(response => {
// 取得成功時の処理
}).catch(error => {
// 取得失敗時の処理
})
Cloud Storage
基本的なファイルのアップロードやダウンロード、削除の方法は以下の通り。
※詳細な使い方はFirebaseのドキュメントを参照
// ファイルのアップロード
this.$fire.storage.ref()
.child(<ファイルパス>) // ファイルへのフルパス
.put(<ファイル>) // BlobまたはFile API
.then(response => {
// アップロード成功時の処理
}).catch(error => {
// アップロード失敗時の処理
})
// ファイルの削除
this.$fire.storage.ref().child(<ファイルパス>).delete().then({
// 削除成功時の処理
}).catch(error => {
// 削除失敗時の処理
})
// ファイルのダウンロード
this.$fire.storage.ref().child(<ファイルパス>).getDownloadURL().then(url => {
// 取得したダウンロードURLでファイルにアクセス
}).catch(error => {
// URL取得失敗時の処理
})