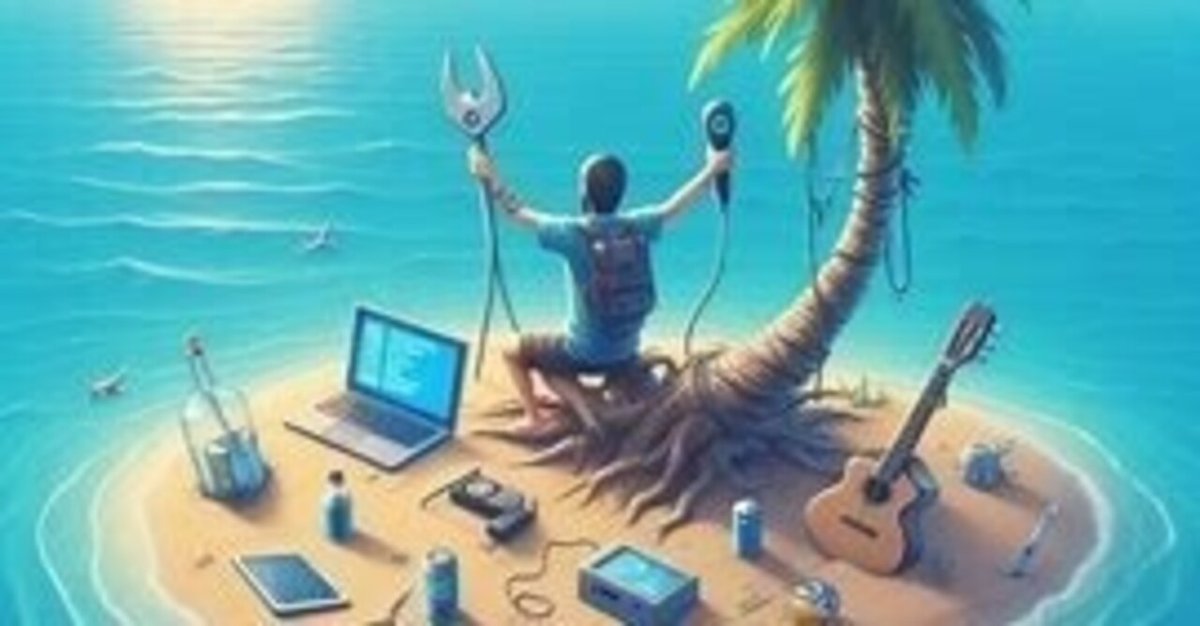
TypeScriptで生き抜く!無人島サバイバル計画をコードで立てる方法 🏝️💻
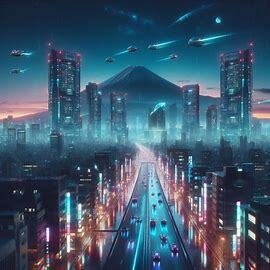
🌟 もしあなたが無人島に漂着したら、何を持ち込む?
今週、TypeScriptの勉強会行くんですけどなにも知らないんで、勉強してみます・・・・。
山田さんと太郎というキャラクターを使用してストーリー展開していきます。
深い森の中、GPSが圏外。方向感覚を失い、一瞬パニックに。その時、ふと思いました。「もし、これが無人島だったら?」そして、「プログラマーである私たちの強みは何だろう?」
そう、TypeScriptです!今回は、TypeScriptの型システムと関数を駆使して、無人島サバイバルをシミュレートしてみましょう。プログラミング初心者の太郎くんと一緒に、コードで命をつなぐ方法を探っていきます!
🧭 TypeScriptで立てる、サバイバル計画
1. リソース管理:生存の鍵
太郎くん:「山田さん、無人島で最初にすべきことって何ですか?」
山田:「そうだね、まずは持っているリソースを把握することだ。TypeScriptを使って、リソース管理システムを作ってみよう。」
// リソースの種類を定義
type ResourceType = 'water' | 'food' | 'wood' | 'tools';
// リソースインターフェースを定義
interface Resource {
type: ResourceType;
quantity: number;
}
// リソース管理クラスを定義
class ResourceManager {
private resources: Map<ResourceType, number> = new Map();
// リソースを追加するメソッド
addResource(type: ResourceType, quantity: number): void {
const currentQuantity = this.resources.get(type) || 0;
this.resources.set(type, currentQuantity + quantity);
console.log(`Added ${quantity} ${type}. New total: ${this.resources.get(type)}`);
}
// リソースを使用するメソッド
useResource(type: ResourceType, quantity: number): boolean {
const currentQuantity = this.resources.get(type) || 0;
if (currentQuantity < quantity) {
console.log(`Not enough ${type}. Current: ${currentQuantity}, Needed: ${quantity}`);
return false;
}
this.resources.set(type, currentQuantity - quantity);
console.log(`Used ${quantity} ${type}. Remaining: ${this.resources.get(type)}`);
return true;
}
// 全リソースの状態を表示するメソッド
displayResources(): void {
console.log('Current Resources:');
this.resources.forEach((quantity, type) => {
console.log(`${type}: ${quantity}`);
});
}
}
// リソース管理システムを使用する
const survivorResources = new ResourceManager();
survivorResources.addResource('water', 5);
survivorResources.addResource('food', 3);
survivorResources.addResource('wood', 10);
survivorResources.displayResources();
survivorResources.useResource('water', 2);
survivorResources.displayResources();
太郎くん:「なるほど!TypeScriptの型システムを使うことで、リソースの種類を明確に定義できるんですね。これなら間違いも少なくなりそうです。」
山田:「そうだね。型安全性はプログラミングだけでなく、サバイバル状況でも重要なんだ。次は、このシステムを使って日々の行動計画を立ててみよう。」
2. 行動計画:効率的なサバイバル
// 行動の種類を定義
type ActionType = 'search for water' | 'gather food' | 'build shelter' | 'create tools';
// 行動インターフェースを定義
interface Action {
type: ActionType;
duration: number;
resourceCost: Partial<Record<ResourceType, number>>;
resourceGain: Partial<Record<ResourceType, number>>;
}
// サバイバー行動管理クラスを定義
class SurvivorActions {
private resourceManager: ResourceManager;
constructor(resourceManager: ResourceManager) {
this.resourceManager = resourceManager;
}
// 行動を実行するメソッド
performAction(action: Action): void {
console.log(`Performing action: ${action.type}`);
// リソースコストの確認と消費
for (const [resourceType, cost] of Object.entries(action.resourceCost)) {
if (!this.resourceManager.useResource(resourceType as ResourceType, cost)) {
console.log(`Cannot perform ${action.type} due to lack of resources.`);
return;
}
}
// 行動の結果、リソースを獲得
for (const [resourceType, gain] of Object.entries(action.resourceGain)) {
this.resourceManager.addResource(resourceType as ResourceType, gain);
}
console.log(`Completed ${action.type}. Duration: ${action.duration} hours.`);
}
}
// 行動計画の例
const searchWater: Action = {
type: 'search for water',
duration: 2,
resourceCost: { food: 1 },
resourceGain: { water: 3 }
};
const gatherFood: Action = {
type: 'gather food',
duration: 3,
resourceCost: { water: 1 },
resourceGain: { food: 2 }
};
// 行動計画の実行
const survivorActions = new SurvivorActions(survivorResources);
survivorActions.performAction(searchWater);
survivorActions.performAction(gatherFood);
太郎くん:「わぁ、これはすごいです!各行動にかかる時間やコスト、得られるリソースまで計算できるんですね。」
山田:「そうだね。これで効率的な行動計画が立てられるよ。でも、サバイバルには予期せぬ事態がつきものだ。次は、環境の変化をシミュレートしてみよう。」
3. 環境シミュレーション:変化に適応する
// 天候の種類を定義
type Weather = 'Sunny' | 'Rainy' | 'Stormy';
// 環境クラスを定義
class Environment {
private weather: Weather = 'Sunny';
// 天候を変更するメソッド
changeWeather(): void {
const weathers: Weather[] = ['Sunny', 'Rainy', 'Stormy'];
this.weather = weathers[Math.floor(Math.random() * weathers.length)];
console.log(`Weather changed to: ${this.weather}`);
}
// 天候に基づいて行動の効果を調整するメソッド
adjustActionEffect(action: Action): Action {
const adjustedAction = { ...action };
switch (this.weather) {
case 'Rainy':
if (action.type === 'search for water') {
adjustedAction.resourceGain.water! *= 1.5; // 水の獲得量50%増加
}
break;
case 'Stormy':
adjustedAction.duration *= 1.5; // すべての行動に50%多く時間がかかる
break;
// Sunny weather doesn't affect actions
}
return adjustedAction;
}
}
// 環境を考慮したサバイバルシミュレーション
const environment = new Environment();
for (let day = 1; day <= 5; day++) {
console.log(`\n--- Day ${day} ---`);
environment.changeWeather();
const adjustedSearchWater = environment.adjustActionEffect(searchWater);
const adjustedGatherFood = environment.adjustActionEffect(gatherFood);
survivorActions.performAction(adjustedSearchWater);
survivorActions.performAction(adjustedGatherFood);
survivorResources.displayResources();
}
太郎くん:「環境の変化まで考慮できるなんて、TypeScriptってすごいですね!」
山田:「そうだね。プログラミングの考え方を使えば、複雑な状況でも論理的に対処できるんだ。」
🌟 まとめ:コードが教えてくれたサバイバルの知恵
この仮想的な無人島サバイバル体験を通じて、私たちは新しい発見をしました。TypeScriptというプログラミング言語が、単なるウェブ開発のツールではなく、思考力を鍛え、問題解決能力を高める強力な道具だということです。
実際の無人島でコーディングはできませんが、日々のコーディングで培った以下のスキルは、どんな困難な状況でも必ず役立つはずです:
論理的思考と問題分解能力
リソース管理と最適化の習慣
予測と対策の重要性
データに基づく意思決定
さらに、今回のシミュレーションを通じて学んだサバイバルの基本も押さえておきましょう:
水の確保が最優先(1日約3リットル必要)
食料の調達と保存方法(野生植物の見分け方、魚の保存方法など)
シェルター作りの基本(地形を利用し、雨風をしのぐ)
道具の作り方(石器、木製の道具など)
これらの知識とプログラミングスキルを組み合わせれば、どんな困難な状況でも道は開けるはずです!
🔍 もっと詳しく知りたい方へ:
💡 次回予告:「都市サバイバル:TypeScriptで災害対策アプリを作ろう」(気が向いたら書きます。。。。多分)
太郎くん:「山田さん、今回の記事で学んだことを、普段の生活にも活かせそうですね。次回も楽しみです!」
山田:「ありがとう、太郎くん。プログラミングの可能性は無限大だ。次回も一緒に学んでいこう!」
みなさんも、日々のコーディングを「サバイバル訓練」だと思って取り組んでみてはいかがでしょうか? きっと新しい視点が得られるはずです。
この記事が気に入ったらサポートをしてみませんか?