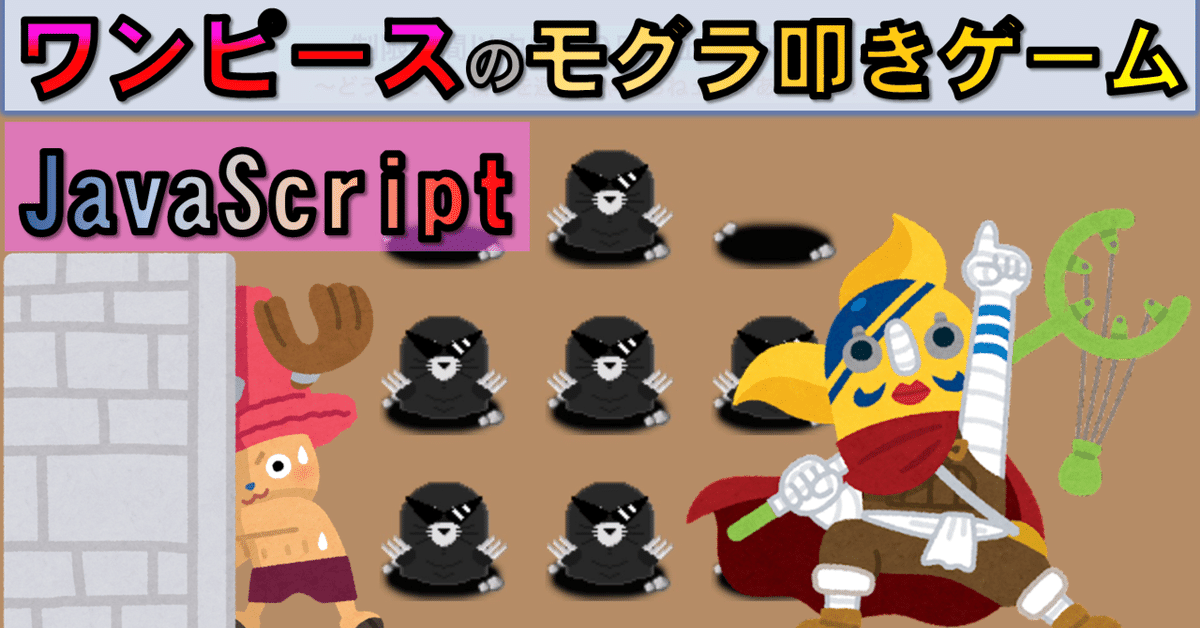
【ワンピース】モグラ叩きゲームをプログラミングで作成(JavaScript)
JavaScriptでモグラ叩きゲームを作成しました。
ワンピースのあのシーンをイメージしてみました!
ウソップの名言が光る場面ですね。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8">
<title>モグラたたきゲーム</title>
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="stylesheet" media="screen" href="style.css">
</head>
<body id="game">
<div class="field">
<h1>制限時間以内に10匹以上たおせ!!</h1>
<h2>〜どうしても…戦いを避けちゃならねェ時がある…!!〜</h2>
<div class="container">
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
</div>
<div class="container">
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
</div>
<div class="container">
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
<div class="relative">
<img class="ana" src="img/hole.png">
<img class="mogura over" src="">
</div>
</div>
</div>
<div id="time_area">
残り<p id="timer">20</p>
</div>
<div id="hit_count_area">
ヒット<p id="hit_count">0</p>
</div>
<p id="result"></p>
<div id="usopp">
<img src="img/usopp.png">
</div>
<div id="chopper">
<img src="img/chopper.png">
</div>
<script src="index.js"></script>
</body>
</html>
#game {
cursor: url("img/hammer.png") 40 40, auto;
background-color: rgb(193, 152, 111);
}
#game:active {
cursor: url("img/hit_hammer.png") 40 40, auto;
}
.field {
margin: 40px;
}
h1 {
text-align: center;
margin: auto;
width: 700px;
background: rgba(140, 248, 155, 0.7);
}
h2 {
text-align: center;
margin: auto;
width: 700px;
background: rgba(248, 237, 140, 0.7);
color: rgb(255, 0, 0);
}
.container {
width: 520px;
height: 160px;
margin: 20px auto;
text-align: center;
display: flex;
}
.container img {
margin: 20px;
width: 140px;
}
.over {
position: absolute;
top: 0;
left: 0;
}
.relative {
position: relative;
}
#time_area {
position: absolute;
text-align: center;
width: 150px;
height: 150px;
top: 100px;
left: 80px;
font-size: 50px;
background-color: rgb(255, 255, 255);
}
#timer {
margin: 0px;
}
#hit_count_area {
position: absolute;
text-align: center;
width: 150px;
height: 150px;
top: 100px;
right: 80px;
font-size: 50px;
background-color: rgb(255, 255, 255);
}
#hit_count {
margin: 0px;
color: rgb(244, 8, 8);
}
#result {
display: none;
position: absolute;
top: 10px;
left: 500px;
font-size: 200px;
font-weight: bold;
background-color: white;
}
#usopp {
position: absolute;
top: 300px;
left: 80px;
}
#usopp img,
#chopper img {
width: 350px;
height: 350px;
}
#chopper {
position: absolute;
top: 300px;
right: 80px;
}
const moguras = document.querySelectorAll(".mogura");
const hitMogura = "img/hit_mogura.png";
const normalMogura = "img/mogura.png";
let timer = 20;
let timeArea = document.getElementById("timer");
let hitCount = 0;
let hitCountArea = document.getElementById("hit_count");
let result = document.getElementById("result");
function init() {
for (let i = 0; i < moguras.length; i++) {//全てのモグラにクリックイベントを登録
moguras[i].addEventListener("click", function (e) {
let mogura = e.target;
mogura.src = hitMogura;//叩かれた画像に変える
mogura.moguraStatus = 2;//ステータス[0:非表示、1:ノーマル、2:叩かれた]
hitCount += 1
hitCountArea.textContent = hitCount;
setTimeout(kakureruMogura, 700, mogura);//0.7秒後に非表示
});;
}
}
function kakureruMogura(mogura) {
mogura.moguraStatus = 0;//ステータスは非表示
mogura.src = ""; //画像は削除
}
function gameStart() {
let countDown = setInterval(() => {
timer += -1
timeArea.textContent = timer;
if (timer <= 0) {
clearInterval(countDown) //タイムが0になったら停止
}
finish()
}, 1000);
let deruMogura = setInterval(() => {
//モグラを1.2秒ごとに表示させる
let random = Math.floor(Math.random() * 9);
let mogura = moguras[random];//0~8までのランダムな数字
mogura.src = normalMogura;
mogura.moguraStatus = 1; //ステータス:ノーマル
setTimeout((mogura) => {
if (mogura.moguraStatus != 2) { //ステータス:叩かれた でないならば
kakureruMogura(mogura); //0.8秒後に非表示
}
}, 800, mogura);
if (timer <= 2) {
clearInterval(deruMogura)
}
}, 1200)
}
function finish(){
if (timer == 0 && hitCount >= 10){
result.textContent = "勝利";
result.style = "display: block;"
result.style.color = "red";
} else if (timer == 0 && hitCount < 10){
result.textContent = "敗北";
result.style = "display: block;"
result.style.color = "blue";
}
}
init()
gameStart();