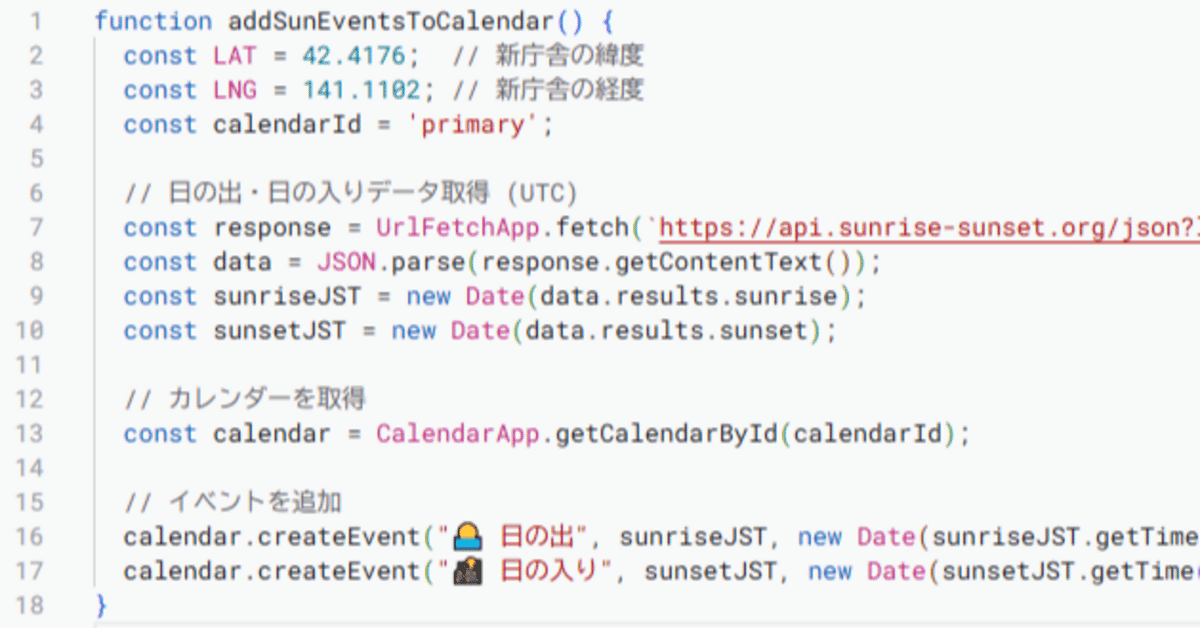
日の出・日の入りをGoogle Calendarに自動登録するスクリプト
スクリプトの使い道
狩猟で銃を撃てる時間は、「日の出🌅から日の入🌇まで」
そのため、ハンターは日の出と日の入り時間を知る方法として
・朝刊でチェック
・スマホでググる
のどちらかだ。特に、エゾシカは日の出と日の入あたりで山や森から出てきて餌を探すため、この時間を把握しておくことが重要。
そこで僕は、毎日Google Calendarに自動で登録されるようにしている。
スクリプトの解説
スクリプトの流れ
「日の出・日の入り時間を取得したい場所」の緯度・経度を定義
無料のAPI( https://api.sunrise-sunset.org/ )を使って日の出・日の入り時刻をリクエスト
取得した日の出・日の入り時間をGoogle カレンダーのイベントを作成
スクリプト(全部)
以下にGoogle Calendarに「日の出」と「日の入」の2つのイベントを作成するGAS(Google Apps Script)を記載する。
function addSunEventsToCalendar() {
const LAT = 42.4176; // 新庁舎の緯度
const LNG = 141.1102; // 新庁舎の経度
const calendarId = 'primary';
// 日の出・日の入りデータ取得 (UTC)
const response = UrlFetchApp.fetch(`https://api.sunrise-sunset.org/json?lat=${LAT}&lng=${LNG}&formatted=0`);
const data = JSON.parse(response.getContentText());
const sunriseJST = new Date(data.results.sunrise);
const sunsetJST = new Date(data.results.sunset);
// カレンダーを取得
const calendar = CalendarApp.getCalendarById(calendarId);
// イベントを追加
calendar.createEvent("🌅 日の出", sunriseJST, new Date(sunriseJST.getTime() + 30 * 60 * 1000)); // 30分間のイベント
calendar.createEvent("🌇 日の入り", sunsetJST, new Date(sunsetJST.getTime() + 30 * 60 * 1000)); // 30分間のイベント
}
経度と緯度の設定
const LAT = 42.4176; // 新庁舎の緯度
const LNG = 141.1102; // 新庁舎の経度
ここで、緯度(LAT)と経度(LNG)を設定
LAT と LNG は、日の出・日の入りの時刻を取得するために使用する位置情報。
僕は「登別市の新庁舎」を使用。
日の出・日の入りデータの取得
const response = UrlFetchApp.fetch(`https://api.sunrise-sunset.org/json?lat=${LAT}&lng=${LNG}&formatted=0`);
const data = JSON.parse(response.getContentText());
UrlFetchApp.fetch() は外部APIからデータを取得するためのメソッド。
ここでは、https://api.sunrise-sunset.org/json というAPIにリクエストを送信。
lat=${LAT}&lng=${LNG} で、先に設定した緯度(LAT)と経度(LNG)をAPIに渡して、指定した場所の日の出・日の入り時刻を取得。
formatted=0 は、APIのレスポンスフォーマットをISO 8601(時間帯を含む)形式にするオプション。
取得したデータはJSON形式で返されるため、JSON.parse() を使ってJavaScriptオブジェクトに変換。
Google カレンダーを取得
const calendar = CalendarApp.getCalendarById(calendarId);
CalendarApp.getCalendarById(calendarId) は、Google カレンダーの指定したカレンダーを取得するメソッド。
ここでは、'primary' というカレンダーIDを使って、ユーザーのプライマリカレンダーを取得。
イベントの追加
calendar.createEvent("🌅 日の出", sunriseJST, new Date(sunriseJST.getTime() + 30 * 60 * 1000)); // 30分間のイベント
calendar.createEvent("🌇 日の入り", sunsetJST, new Date(sunsetJST.getTime() + 30 * 60 * 1000)); // 30分間のイベント
createEvent() メソッドを使用して、カレンダーにイベントを追加。
イベント名には絵文字で「🌅 日の出」や「🌇 日の入り」に。
イベントの開始時間として、先に取得した日の出・日の入り時刻(sunriseJST と sunsetJST)を設定。
イベントの終了時間は、開始時刻から30分後に設定。これは、日の出と日の入りの時刻を30分間のイベントとして追加するため。
設定方法(初心者向け)
Google Apps Script を開く
1.Google ドライブを開きます。(Google ドライブ)
2.左上の「新規」ボタンをクリックし、次に「Google Apps Script」を選択します。これで新しいスクリプトエディタが開きます。
スクリプトを設定する
1.スクリプトエディタに、以下のコードをコピー&ペーストします。
function addSunEventsToCalendar() {
const LAT = 42.4176; // 新庁舎の緯度
const LNG = 141.1102; // 新庁舎の経度
const calendarId = 'primary';
// 日の出・日の入りデータ取得 (UTC)
const response = UrlFetchApp.fetch(`https://api.sunrise-sunset.org/json?lat=${LAT}&lng=${LNG}&formatted=0`);
const data = JSON.parse(response.getContentText());
const sunriseJST = new Date(data.results.sunrise);
const sunsetJST = new Date(data.results.sunset);
// カレンダーを取得
const calendar = CalendarApp.getCalendarById(calendarId);
// イベントを追加
calendar.createEvent("🌅 日の出", sunriseJST, new Date(sunriseJST.getTime() + 30 * 60 * 1000)); // 30分間のイベント
calendar.createEvent("🌇 日の入り", sunsetJST, new Date(sunsetJST.getTime() + 30 * 60 * 1000)); // 30分間のイベント
}
2.日の出・日の入時間を取得したい場所の「緯度・経度」を調べます。
GoogleMapで取得したい場所を探して、そこで右クリックすると表示されます。(画像の赤枠)
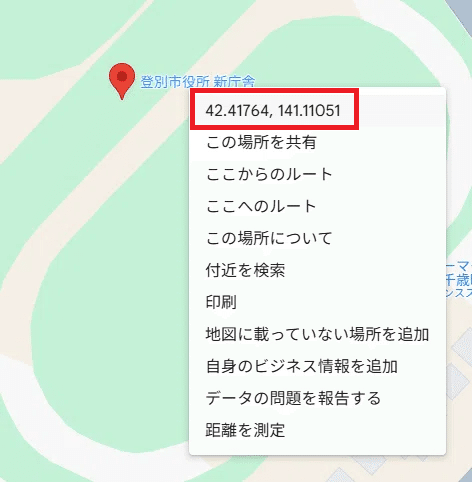
3.スクリプトの「緯度・経度」を変更します。
const LAT = 42.4176; // 新庁舎の緯度
const LNG = 141.1102; // 新庁舎の経度
4.エディタの左上にある「無題のプロジェクト」をクリックし、プロジェクト名を適当に入力します。(例: Sun Events Script)
必要な権限を設定する
Google カレンダーを操作するために、スクリプトがアクセス権を持っている必要があります。最初にスクリプトを実行すると、認証を求められます。
スクリプトエディタの画面上部にある「実行」ボタン(再生アイコン)をクリックします。
認証の要求が表示されたら、「レビュー権限」をクリックします。
自分のGoogleアカウントを選択し、「許可」をクリックします。
スクリプトを定期的に実行する設定をする
スクリプトエディタの画面上部にある時計アイコン(トリガー)をクリックします。
「新しいトリガーを追加」ボタンをクリックします。
以下の設定に変更します。
「実行する関数」を addSunEventsToCalendar
「イベントのソース」を「時間主導型」
「トリガーのタイプ」を「日付ベース」
時刻を「午前0時~1時」
「保存」をクリックします。
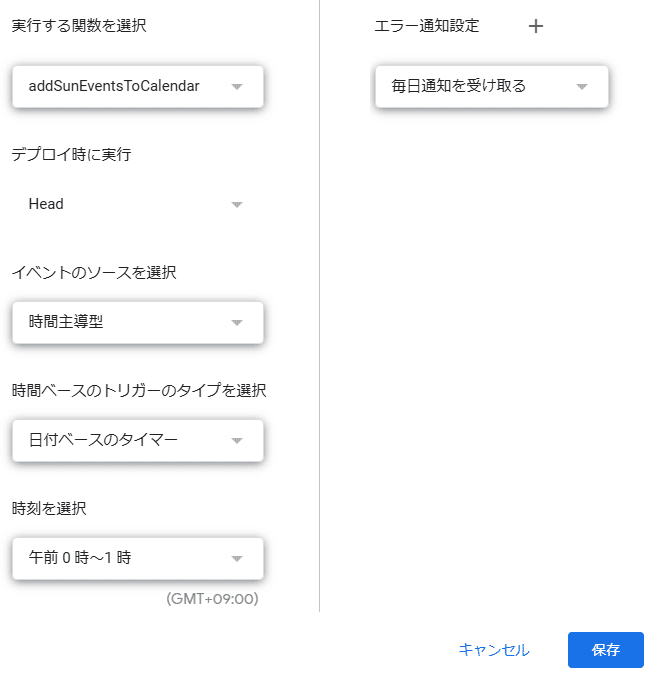
完了
これで、スクリプトが指定した頻度で自動的に実行され、日の出と日の入りのイベントがカレンダーに追加されます。
終わりに
山に入ったら電波が届かなくて日の出・日の入り時間を調べられないことがあるので、Google Calendarに予定を自動登録しておくと便利!