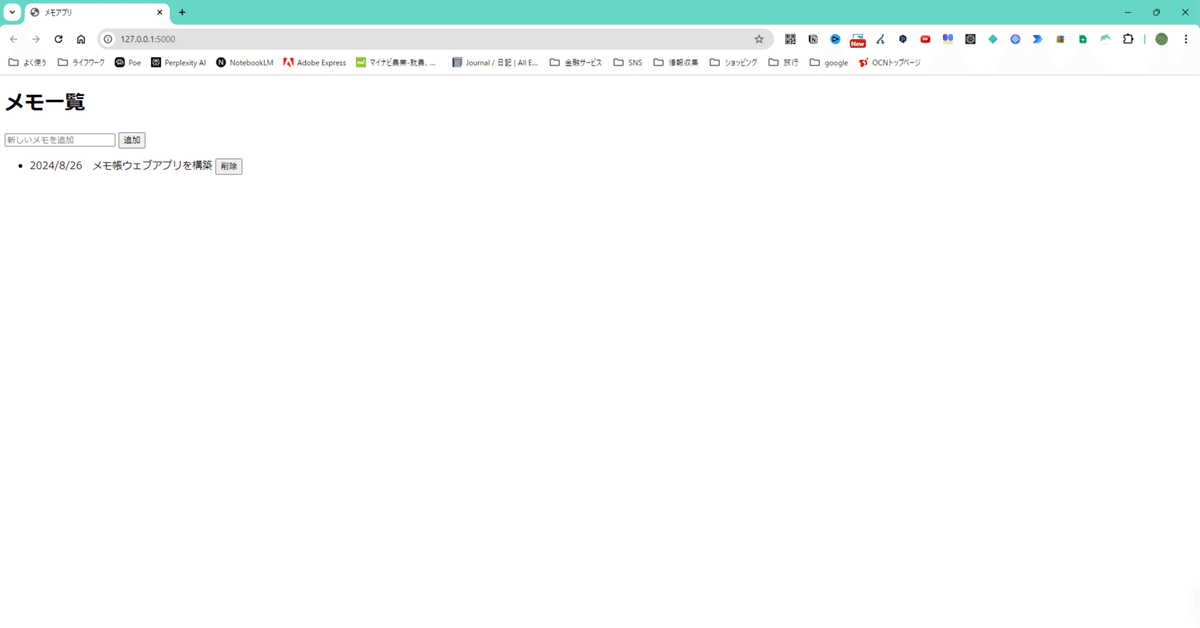
メモ帳アプリを作成しました
初めてのウェブアプリを作成しました。
ちょっと感動しています。
ここからさらに発展できるように精進します。
コードを書いておきますので、参考にしてみてください。
Pythonのコード
from flask import Flask, render_template, request, redirect, url_for
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///notes.db'
db = SQLAlchemy(app)
class Note(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(200), nullable=False)
@app.route('/')
def index():
notes = Note.query.all()
return render_template('index.html', notes=notes)
@app.route('/add', methods=['POST'])
def add_note():
note_title = request.form.get('title')
new_note = Note(title=note_title)
db.session.add(new_note)
db.session.commit()
return redirect(url_for('index'))
@app.route('/delete/<int:note_id>', methods=['POST'])
def delete_note(note_id):
note = Note.query.get(note_id)
if note:
db.session.delete(note)
db.session.commit()
return redirect(url_for('index'))
if __name__ == '__main__':
with app.app_context():
db.create_all() # データベースの初期化
app.run(debug=True)
create.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>新しいメモを作成</title>
</head>
<body>
<h1>新しいメモを作成</h1>
<form method="POST">
<textarea name="content" required></textarea>
<button type="submit">保存</button>
</form>
<a href="/">戻る</a>
</body>
</html>
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>メモアプリ</title>
</head>
<body>
<h1>メモ一覧</h1>
<form action="/add" method="post">
<input type="text" name="title" placeholder="新しいメモを追加" required>
<button type="submit">追加</button>
</form>
<ul>
{% for note in notes %}
<li>
{{ note.title }}
<form action="{{ url_for('delete_note', note_id=note.id) }}" method="post" style="display:inline;">
<button type="submit">削除</button>
</form>
</li>
{% endfor %}
</ul>
</body>
</html>
ディレクトリの構成例
/your_project_directory
│
├── app.py (または test.py)
├── templates/
│ ├── index.html
│ └── create.html
└── ...
以上です。
これでウェブアプリが完成するはずです。
これ、プロンプトプログラミングでやりました。
凄い時代になったものです。
ありがとうPoe!
この記事が気に入ったらサポートをしてみませんか?