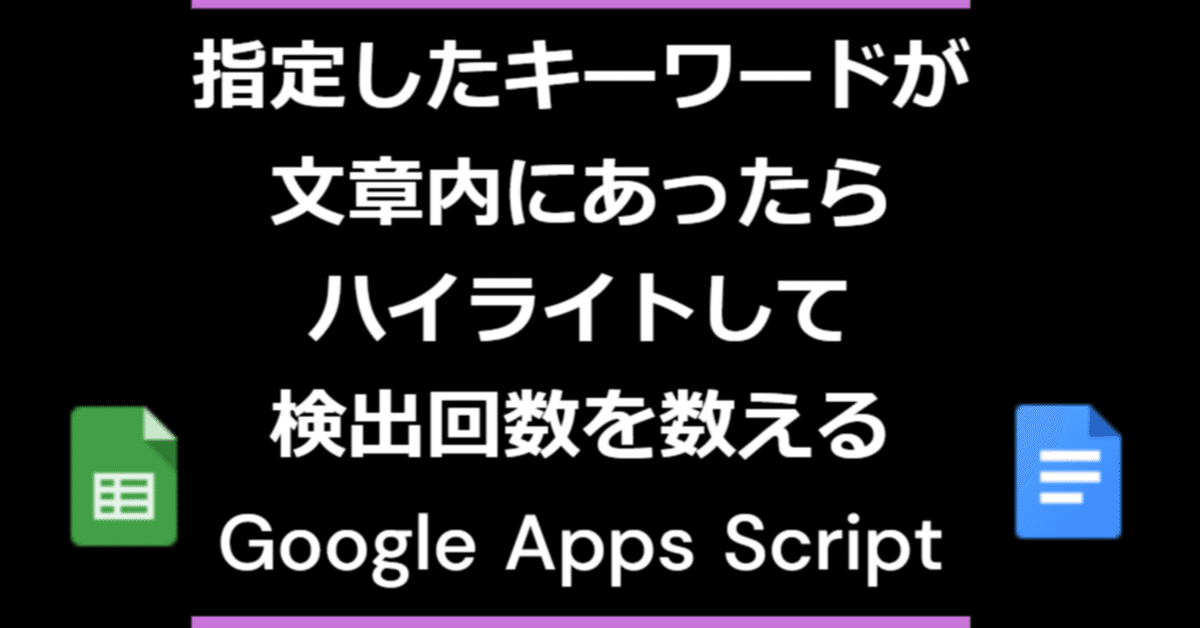
指定したキーワードが文章内にあったらハイライトして検出回数を数えるGoogle Apps Scriptの例
この説明は、ChatGPTで作成しています。
このスクリプトは、Googleスプレッドシート に登録されたキーワードを使って、Googleドキュメント の文章内でそのキーワードを自動的にハイライト表示するものです。また、ハイライトされたキーワードが何回出現したかをスプレッドシートに記録します。
このスクリプトの動作の流れ
スプレッドシートからキーワードとハイライト色を取得
「KWマスタ」 という名前のシートから、A列(キーワード)とB列(ハイライト色)を読み取ります。
シート名はスクリプト内を編集することで変更できます。
Googleドキュメントを開く
指定された Googleドキュメント を開き、以前のハイライトをすべて解除します。
キーワードを検索し、指定された色でハイライト
ドキュメント内でキーワードを探し、B列の指定色でハイライトします。
色はスクリプト内を編集することで変更できます。
キーワードの出現回数をスプレッドシートに記録
C列に各キーワードの出現回数を記入します。
事前準備
このスクリプトを実行するには、スプレッドシートID と GoogleドキュメントID を設定する必要があります。
スプレッドシートIDの取得方法
Googleスプレッドシートを開く。
URLを確認し、次のような形式の文字列をコピーする。
edit より前の部分が スプレッドシートID です。
スクリプト内の SPREADSHEET_ID に設定してください。
GoogleドキュメントIDの取得方法
Googleドキュメントを開く。
URLを確認し、次のような形式の文字列をコピーする。
edit より前の部分が ドキュメントID です。
スクリプト内の DOCUMENT_ID に設定してください。
スクリプトの貼り付け方法
スプレッドシートを開く
メニューから「拡張機能」 → 「Apps Script」を開く
エディターにスクリプトを貼り付ける
スプレッドシートIDとGoogleドキュメントIDを設定する
保存して実行する
このスクリプトのポイント
✅ スプレッドシートとGoogleドキュメントを連携できる
✅ キーワードごとにハイライト色を自由に設定可能
✅ キーワードの出現回数をスプレッドシートに記録
✅ シート名やハイライトの色をカスタマイズできる
🔗 参考リンク
function KeywordshighlightInDoc() {
// 設定情報
const SPREADSHEET_ID = "●●●ここにスプレッドシートIDをいれてください●●●";
const SHEET_NAME = "●●●ここにシートタブ名をいれてください●●●※動画では「KWマスタ」";
const DOCUMENT_ID = "●●●ここにドキュメントIDをいれてください●●●";
// スプレッドシートを開く
const ss = SpreadsheetApp.openById(SPREADSHEET_ID);
const sheet = ss.getSheetByName(SHEET_NAME);
const data = sheet.getDataRange().getValues(); // 全データ取得
if (data.length < 2) {
Logger.log("キーワードリストが空です。処理を終了します。");
return;
}
// キーワードリスト取得(A列:キーワード, B列:ハイライト色)
let keywords = [];
let highlightColors = {};
data.slice(1).forEach(row => {
if (row[0] && row[1]) {
keywords.push(row[0]);
highlightColors[row[0]] = getColorCode(row[1]); // ハイライト色を取得
}
});
// Googleドキュメントを開く
const doc = DocumentApp.openById(DOCUMENT_ID);
const body = doc.getBody();
// **既存のハイライトをすべて解除**
removeAllHighlights(body);
// 検出回数カウンター
let keywordCounts = {};
keywords.forEach(keyword => { keywordCounts[keyword] = 0; });
// すべてのキーワードを検索し、指定色でハイライト
keywords.forEach(keyword => {
let searchResult = body.findText(keyword); // 最初の出現箇所を検索
let highlightColor = highlightColors[keyword] || "#FFFF00"; // デフォルト: 黄色
while (searchResult !== null) {
let rangeElement = searchResult.getElement();
let startOffset = searchResult.getStartOffset();
let endOffset = searchResult.getEndOffsetInclusive();
// 部分的にハイライト
if (rangeElement.editAsText) {
let textElement = rangeElement.editAsText();
textElement.setBackgroundColor(startOffset, endOffset, highlightColor);
}
keywordCounts[keyword] += 1;
searchResult = body.findText(keyword, searchResult); // 次の検索
}
});
// スプレッドシートに検出回数を書き込む(C列)
data.slice(1).forEach((row, index) => {
let keyword = row[0];
let count = keywordCounts[keyword] || 0;
sheet.getRange(index + 2, 3).setValue(count);
});
Logger.log("処理が完了しました。");
}
/**
* **既存のハイライトをすべて解除する**
* - Googleドキュメントの本文から、すべてのテキストの背景色をクリア
*/
function removeAllHighlights(body) {
let totalElements = body.getNumChildren();
for (let i = 0; i < totalElements; i++) {
let element = body.getChild(i);
if (element.getType() == DocumentApp.ElementType.PARAGRAPH ||
element.getType() == DocumentApp.ElementType.TEXT) {
let textElement = element.asText();
textElement.setBackgroundColor(null); // 背景色をクリア
}
}
Logger.log("既存のハイライトを解除しました。");
}
/**
* **ハイライト色を取得**
* - 「緑色」「青色」「黄色」の3種類をサポート
* - 未指定や不明な色はデフォルト「黄色」に設定
*/
function getColorCode(colorName) {
const colorMap = {
"緑色": "#00FF00",
"青色": "#00FFFF",
"黄色": "#FFFF00"
};
return colorMap[colorName] || "#FFFF00"; // デフォルトは黄色
}
関連キーワード
#GoogleAppsScript #GoogleDrive #スプレッドシート #Googleドキュメント #ハイライト #キーワード検索 #自動化 #スクリプト #プログラミング #スプレッドシート連携 #文章解析 #GAS #Googleスクリプト #AppsScript活用 #業務効率化 #初心者向け #スクリプト開発 #テキスト処理 #自動ハイライト
English Version
This explanation is generated by ChatGPT.
Script Name: Automatic Keyword Highlighting in Google Docs
This script automatically highlights specific keywords in a Google Document using a keyword list stored in a Google Spreadsheet. Additionally, it records how many times each keyword appears in the document.
How This Script Works
Retrieve Keywords and Highlight Colors from the Spreadsheet
Reads keywords from column A and highlight colors from column B in the "KW Master" sheet.
You can change the sheet name by editing the script.
Open the Google Document
Opens the specified Google Document and removes any previous highlights.
Search for Keywords and Apply the Specified Highlight Color
Finds each keyword in the document and highlights it using the color specified in column B.
You can change the highlight colors by editing the script.
Record the Keyword Appearance Count in the Spreadsheet
Writes the number of times each keyword appears in column C.
Pre-Setup Instructions
To use this script, you must set the Spreadsheet ID and Google Document ID.
How to Get the Spreadsheet ID
Open your Google Spreadsheet.
Check the URL, and copy the highlighted part as shown below:
The portion before /edit is the Spreadsheet ID.
Set this ID in the SPREADSHEET_ID variable inside the script.
How to Get the Google Document ID
Open your Google Document.
Check the URL, and copy the highlighted part as shown below:
The portion before /edit is the Google Document ID.
Set this ID in the DOCUMENT_ID variable inside the script.
How to Insert and Run the Script
Open the Google Spreadsheet
Go to "Extensions" → "Apps Script"
Paste the script into the editor
Set the Spreadsheet ID and Google Document ID
Save and execute the script
Key Features of This Script
✅ Integrates Google Spreadsheets with Google Docs
✅ Allows you to customize highlight colors for each keyword
✅ Automatically records keyword appearance counts in the spreadsheet
✅ Allows customization of sheet names and highlight colors
🔗 Reference Links