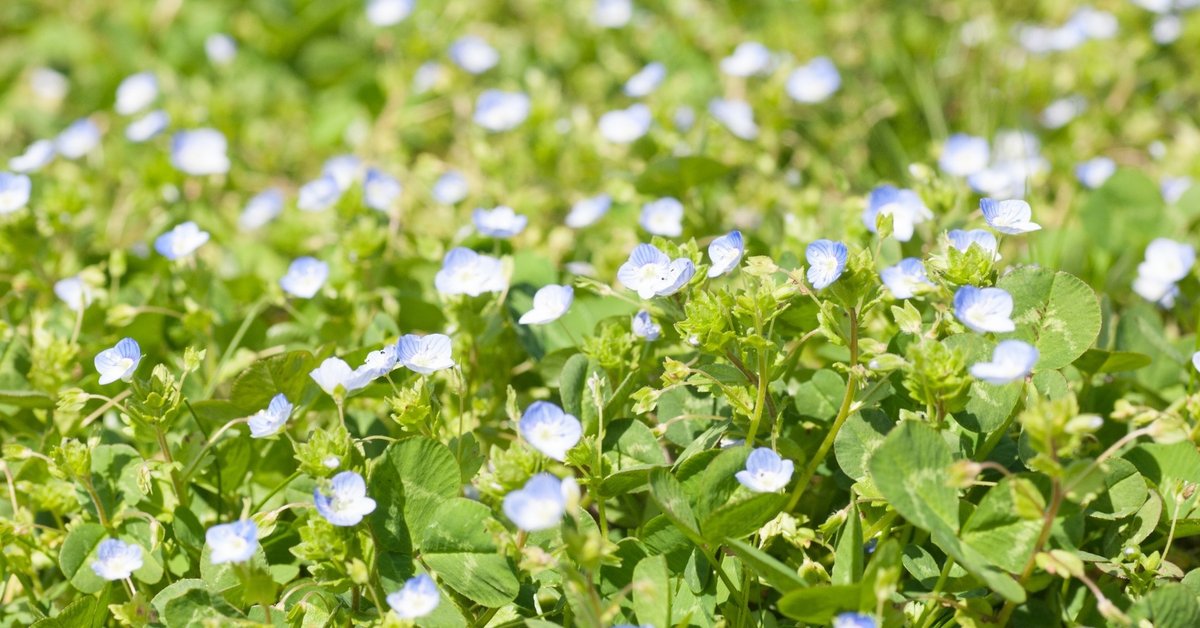
ML.netでModel Builderを使ってみる その5
一歩下がって二歩進むような状況ですが、苦労した分だけ経験と学習する内容が増えるから良いのかなと思います。
Model Builderを使ったコード、特定のファイルを直接指定するようになっています。
これはちょっと駄目なので、プログラムを起動しているフォルダに保存しているZIPファイルを指定するようにします。
ConsumeModel.csで学習結果が保存されているZIPファイルを直接指定している部分を修正します。
// Load model & create prediction engine
string modelPath = System.AppDomain.CurrentDomain.BaseDirectory + "MLModel.zip";
これで、実行ファイルが存在するフォルダと同じ場所にMLModel.zipがあれば、プログラムが動きます。
他にもModelBuilder.csにファイルを直接保存している場所がありますが、こちらは現状では未使用なので、気にしない事にします。
残る修正が必要な場所、それは入力するファイルを指定する部分です。
この指定、VB.net側から指定できるようにしてしまいましょう。
文字列を受け渡しするだけなので、今まで沢山やってきたパターンと変わりません。
一応、ソースコードを掲載しておきます。
このコード、以下のWebアドレスに掲載されているものを基本にしています。
https://docs.microsoft.com/ja-jp/dotnet/machine-learning/
印刷して学びたい場合もPDFファイルがあるので参考になります。
https://docs.microsoft.com/ja-jp/dotnet/opbuildpdf/machine-learning/toc.pdf?branch=live
#学習 #勉強 #AI #機械学習 #プログラミング #Visual #CSharp #BASIC #ソースコード #Windows #自動判定
#画像 #判定 #検査
VB.netのソースコード
Imports System.Reflection
Imports System.Windows.Forms
Imports System.IO
Imports System.Text
Imports Microsoft.VisualBasic.FileIO
Imports System.Globalization
Public Class Form1
Private Sub Form1_Load(sender As Object, e As EventArgs) Handles MyBase.Load
'クラスライブラリーを使う宣言
Dim ClassLibraryObject As Object = New ClassLibrary1.Class1
'写真を入力するフォルダの指定
'このフォルダ指定は自分の環境にあったものにしてください。
Dim SpecifyFileToInputPhoto As String = "C:\Test\assets\CD\7001-115.jpg"
'C#のクラスライブラリーで画像の判定を行わせる
Dim BooleanReturn As Boolean = ClassLibraryObject.SampleMachineLearning(SpecifyFileToInputPhoto)
End Sub
End Class
C#のクラスライブラリーのコードです。
using System;
using System.Collections.Generic;
using System.Linq;
using System.IO;
using Microsoft.ML;
using static Microsoft.ML.DataOperationsCatalog;
using Microsoft.ML.Vision;
namespace ClassLibrary1
{
public class Class1
{
public void SampleMachineLearning(string SpecifyFileToInputPhoto)
{
// Create single instance of sample data from first line of dataset for model input
MlTestML.Model.ModelInput sampleData = new MlTestML.Model.ModelInput()
{
ImageSource = SpecifyFileToInputPhoto,
};
// Make a single prediction on the sample data and print results
var predictionResult = MlTestML.Model.ConsumeModel.Predict(sampleData);
Console.WriteLine("Using model to make single prediction -- Comparing actual Label with predicted Label from sample data...\n\n");
Console.WriteLine($"ImageSource: {sampleData.ImageSource}");
Console.WriteLine($"\n\nPredicted Label value {predictionResult.Prediction} \nPredicted Label scores: [{String.Join(",", predictionResult.Score)}]\n\n");
Console.WriteLine("=============== End of process, hit any key to finish ===============");
//Console.ReadKey();
}
}
}
Model Builderが作ったConsumeModel.csから小改良したソースコードです。
// This file was auto-generated by ML.NET Model Builder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.ML;
using MlTestML.Model;
using static Microsoft.ML.DataOperationsCatalog;
using Microsoft.ML.Vision;
namespace MlTestML.Model
{
public class ConsumeModel
{
private static Lazy<PredictionEngine<ModelInput, ModelOutput>> PredictionEngine = new Lazy<PredictionEngine<ModelInput, ModelOutput>>(CreatePredictionEngine);
// For more info on consuming ML.NET models, visit https://aka.ms/mlnet-consume
// Method for consuming model in your app
public static ModelOutput Predict(ModelInput input)
{
ModelOutput result = PredictionEngine.Value.Predict(input);
return result;
}
public static PredictionEngine<ModelInput, ModelOutput> CreatePredictionEngine()
{
// Create new MLContext
MLContext mlContext = new MLContext();
// Load model & create prediction engine
string modelPath = System.AppDomain.CurrentDomain.BaseDirectory + "MLModel.zip";
ITransformer mlModel = mlContext.Model.Load(modelPath, out var modelInputSchema);
var predEngine = mlContext.Model.CreatePredictionEngine<ModelInput, ModelOutput>(mlModel);
return predEngine;
}
}
}