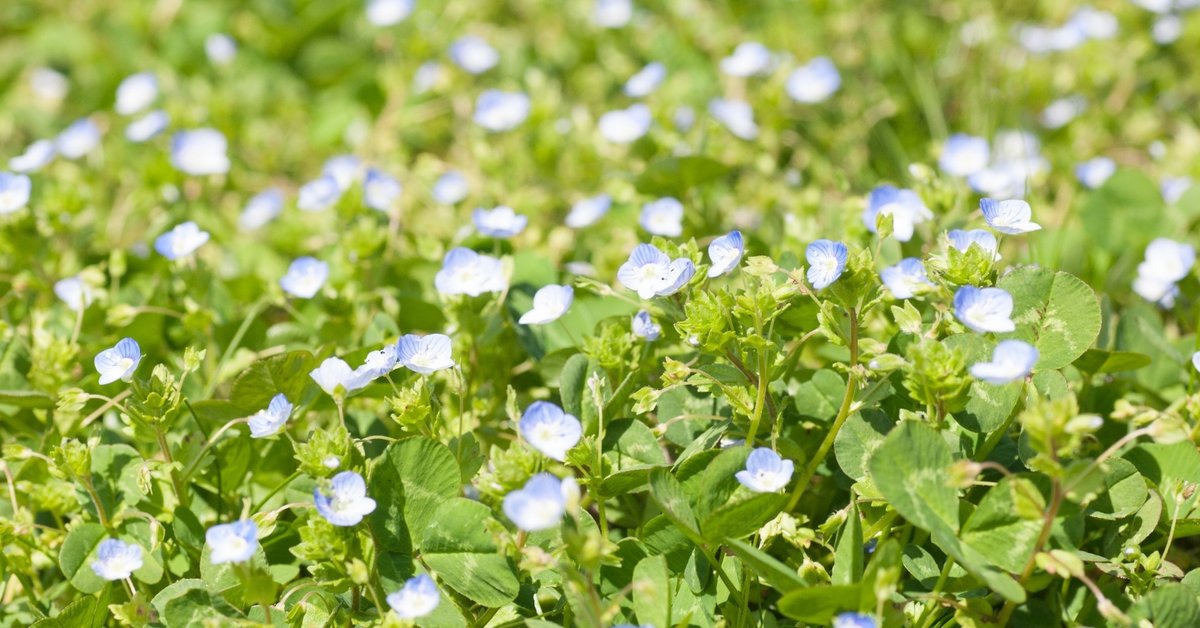
ML.netでModel Builderを使ってみる その7 C#でロギングを使ってみる
今まで自作の関数を使っていたのですが、そのまま公開するのも色々な問題があります。
そこで、標準的なロギングのlog4netを使ってみます。
私は初見ですので、詳しい設定などはインターネット検索してください。
NuGetでlog4netをインストールします。
C#でクラスライブラリーを書いているプロジェクトに、新しい項目の追加を選択します。
アプリケーション構成ファイルを選び、ファイル名をlog4net.configにします。
log4net.configの中身、適当にネットで出てきたものをコピペします。
(私が書くより詳しく書いている人、沢山居ますので)
一旦、Visual Studioを保存して終了し、C#で書いたクラスライブラリーのPropertiesフォルダを表示します。
AssemblyInfo.csをエディタで開き、using定義している下の行あたりに、以下を追記します。
[assembly: log4net.Config.XmlConfigurator(ConfigFile="Log4net.Config", Watch=true)]
(なんでVisual Studioから直接編集できないんかな)
あとは、ロギングを出力する処理を追加します。
自分でロギングの関数を作って使っているので、業界標準のロギングが便利か、良くわからないです。
このコード、以下のWebアドレスに掲載されているものを基本にしています。
https://docs.microsoft.com/ja-jp/dotnet/machine-learning/
印刷して学びたい場合もPDFファイルがあるので参考になります。
https://docs.microsoft.com/ja-jp/dotnet/opbuildpdf/machine-learning/toc.pdf?branch=live
#学習 #勉強 #AI #機械学習 #プログラミング #Visual #CSharp #BASIC #ソースコード #Windows #自動判定
#画像 #判定 #検査
C#で書いたクラスライブラリーのソースコードを記載します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.IO;
using Microsoft.ML;
using static Microsoft.ML.DataOperationsCatalog;
using Microsoft.ML.Vision;
using System.Reflection;
using log4net;
namespace ClassLibrary1
{
public class Class1
{
public void SampleMachineLearning(string SpecifyFileToInputPhoto,ref string JudgmentResult, ref float Probability,string LocationOfLearningData)
{
//ロギングを開始する
var logger = LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
//関数の起動時の引数を保存する
logger.Info("起動時引数一覧");
logger.Info("SpecifyFileToInputPhoto:" + SpecifyFileToInputPhoto);
logger.Info("LocationOfLearningData:" + LocationOfLearningData);
// Create single instance of sample data from first line of dataset for model input
MlTestML.Model.ModelInput sampleData = new MlTestML.Model.ModelInput()
{
ImageSource = SpecifyFileToInputPhoto,
};
//学習データの位置を指定する
MlTestML.Model.ConsumeModel.modelPath = LocationOfLearningData;
// Make a single prediction on the sample data and print results
var predictionResult = MlTestML.Model.ConsumeModel.Predict(sampleData);
Console.WriteLine("Using model to make single prediction -- Comparing actual Label with predicted Label from sample data...\n\n");
Console.WriteLine($"ImageSource: {sampleData.ImageSource}");
Console.WriteLine($"\n\nPredicted Label value {predictionResult.Prediction} \nPredicted Label scores: [{String.Join(",", predictionResult.Score)}]\n\n");
Console.WriteLine("=============== End of process, hit any key to finish ===============");
//Console.ReadKey();
JudgmentResult = predictionResult.Prediction;
Probability = 0;
for (int i = 0; i < predictionResult.Score.Length; i++)
{
if (Probability < predictionResult.Score[i])
{
Probability = predictionResult.Score[i];
}
}
//関数の終了時の戻り値を保存する
logger.Info("終了時引数一覧");
logger.Info("JudgmentResult:" + JudgmentResult);
logger.Info("Probability:" + Probability);
}
}
}