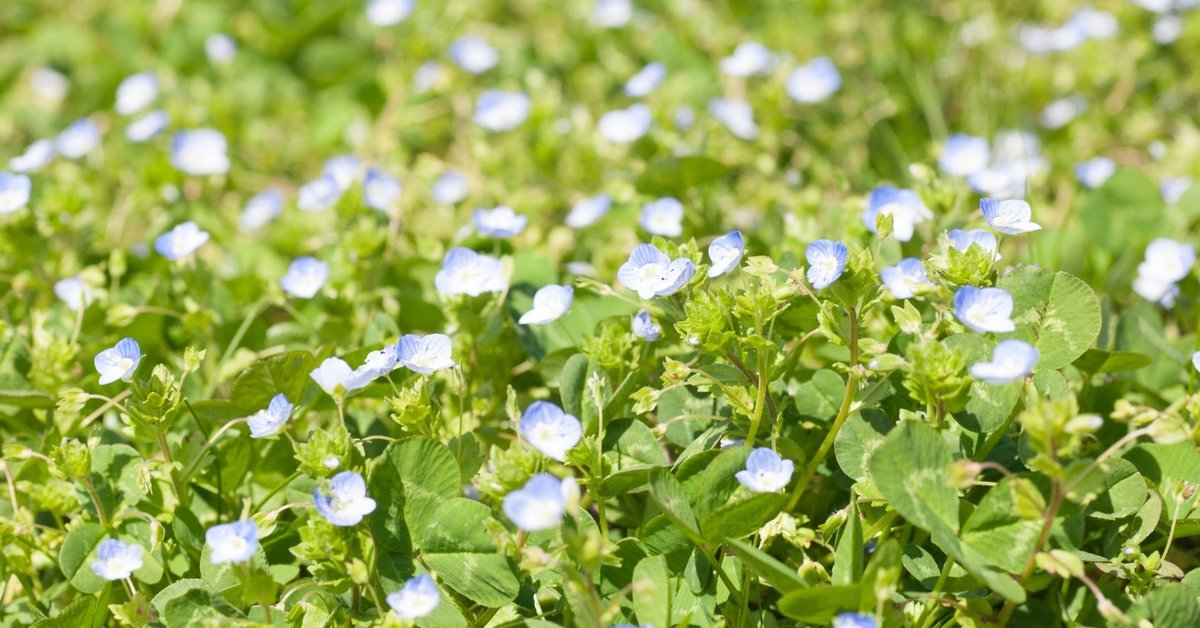
ML.netでModel Builderを使ってみる その10 ロギングも不足しています
log4netを使ってロギングが出来るようになりました。
そこで、個人的にConsumeModel.csでもロギングしようとしたのですが、結構手間取りました。
自動生成されるソースコードなので、AssemblyInfo.cs が出力されないんですよね。
そこで、サンプルソースコードを作ることにしました。
基本的に自動生成されたソースコードの部分、例外エラーだけロギングできれば十分だと思います。
想定される例外エラーは、判定する画像ファイルかMLModel.zipが存在しないかだけなので。
このソースコードを使って、指定するファイル名を変更するなど例外エラーが発生する状況を作ってみてください。
例外エラーの発生がロギングされているので、効果が実感できると思います。
前回作ったソースコードを改良してみました。
自動生成されるソースコードでも、例外エラーが発生した場合にロギングされます。
このコード、以下のWebアドレスに掲載されているものを基本にしています。
https://docs.microsoft.com/ja-jp/dotnet/machine-learning/
印刷して学びたい場合もPDFファイルがあるので参考になります。
https://docs.microsoft.com/ja-jp/dotnet/opbuildpdf/machine-learning/toc.pdf?branch=live
#学習 #勉強 #AI #機械学習 #プログラミング #Visual #CSharp #BASIC #ソースコード #Windows #自動判定
#画像 #判定 #検査
C#で作ったクラスライブラリーのソースコードです。
using System;
using System.Collections.Generic;
using System.Linq;
using System.IO;
using Microsoft.ML;
using static Microsoft.ML.DataOperationsCatalog;
using Microsoft.ML.Vision;
using System.Reflection;
using log4net;
namespace ClassLibrary1
{
public class Class1
{
public bool SampleMachineLearning(string SpecifyFileToInputPhoto,ref string JudgmentResult, ref float Probability,string LocationOfLearningData)
{
//ロギングを開始する、namespaceを超えるので、付加情報は関数ごとに付ける
ILog logger = LogManager.GetLogger("");
//MlTestML.ModelのConsumeModelでも、ロガーの処理を実行させるため、インターフェースを受け渡す
MlTestML.Model.ConsumeModel.loggerObj = logger;
//付加情報
logger.Info(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType +":"+ MethodBase.GetCurrentMethod().Name);
//関数の起動時の引数を保存する
logger.Info("起動時引数一覧");
logger.Info("SpecifyFileToInputPhoto:" + SpecifyFileToInputPhoto);
logger.Info("LocationOfLearningData:" + LocationOfLearningData);
try
{
// Create single instance of sample data from first line of dataset for model input
MlTestML.Model.ModelInput sampleData = new MlTestML.Model.ModelInput()
{
ImageSource = SpecifyFileToInputPhoto,
};
//学習データの位置を指定する
MlTestML.Model.ConsumeModel.modelPath = LocationOfLearningData;
// Make a single prediction on the sample data and print results
var predictionResult = MlTestML.Model.ConsumeModel.Predict(sampleData);
Console.WriteLine("Using model to make single prediction -- Comparing actual Label with predicted Label from sample data...\n\n");
Console.WriteLine($"ImageSource: {sampleData.ImageSource}");
Console.WriteLine($"\n\nPredicted Label value {predictionResult.Prediction} \nPredicted Label scores: [{String.Join(",", predictionResult.Score)}]\n\n");
Console.WriteLine("=============== End of process, hit any key to finish ===============");
//Console.ReadKey();
JudgmentResult = predictionResult.Prediction;
Probability = 0;
for (int i = 0; i < predictionResult.Score.Length; i++)
{
if (Probability < predictionResult.Score[i])
{
Probability = predictionResult.Score[i];
}
}
//関数の終了時の戻り値を保存する
logger.Info("終了時引数一覧");
logger.Info("JudgmentResult:" + JudgmentResult);
logger.Info("Probability:" + Probability);
}
catch (System.Exception ex)
{
logger.Error("HResult:" + ex.HResult);
logger.Error("Exception:"+ex.Message);
return false;
}
//正常終了
return true;
}
}
}
ConsumeModel.csのソースコードです。
// This file was auto-generated by ML.NET Model Builder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using Microsoft.ML;
using MlTestML.Model;
using static Microsoft.ML.DataOperationsCatalog;
using Microsoft.ML.Vision;
using System.Reflection;
using log4net;
namespace MlTestML.Model
{
public class ConsumeModel
{
// ClassLibrary1のClass1から、ロガーの処理を実行させるため、インターフェースを教えてもらう
public static ILog loggerObj;
private static Lazy<PredictionEngine<ModelInput, ModelOutput>> PredictionEngine = new Lazy<PredictionEngine<ModelInput, ModelOutput>>(CreatePredictionEngine);
// For more info on consuming ML.NET models, visit https://aka.ms/mlnet-consume
// Method for consuming model in your app
public static ModelOutput Predict(ModelInput input)
{
//付加情報
loggerObj.Info(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType + ":" + MethodBase.GetCurrentMethod().Name);
//戻り値の初期化
ModelOutput result = null;
try
{
result = PredictionEngine.Value.Predict(input);
}
catch (System.Exception ex)
{
loggerObj.Error("HResult:" + ex.HResult);
loggerObj.Error("Exception:" + ex.Message);
}
return result;
}
//学習結果のMLModel.zipが格納されている場所を指示する。
public static string modelPath;
public static PredictionEngine<ModelInput, ModelOutput> CreatePredictionEngine()
{
//付加情報
loggerObj.Info(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType + ":" + MethodBase.GetCurrentMethod().Name);
//戻り値の初期化
PredictionEngine<ModelInput, ModelOutput> predEngine=null;
try
{
// Create new MLContext
MLContext mlContext = new MLContext();
// Load model & create prediction engine
//string modelPath = System.AppDomain.CurrentDomain.BaseDirectory + "MLModel.zip";
ITransformer mlModel = mlContext.Model.Load(modelPath, out var modelInputSchema);
predEngine = mlContext.Model.CreatePredictionEngine<ModelInput, ModelOutput>(mlModel);
}
catch(System.Exception ex)
{
loggerObj.Error("HResult:" + ex.HResult);
loggerObj.Error("Exception:" + ex.Message);
}
return predEngine;
}
}
}