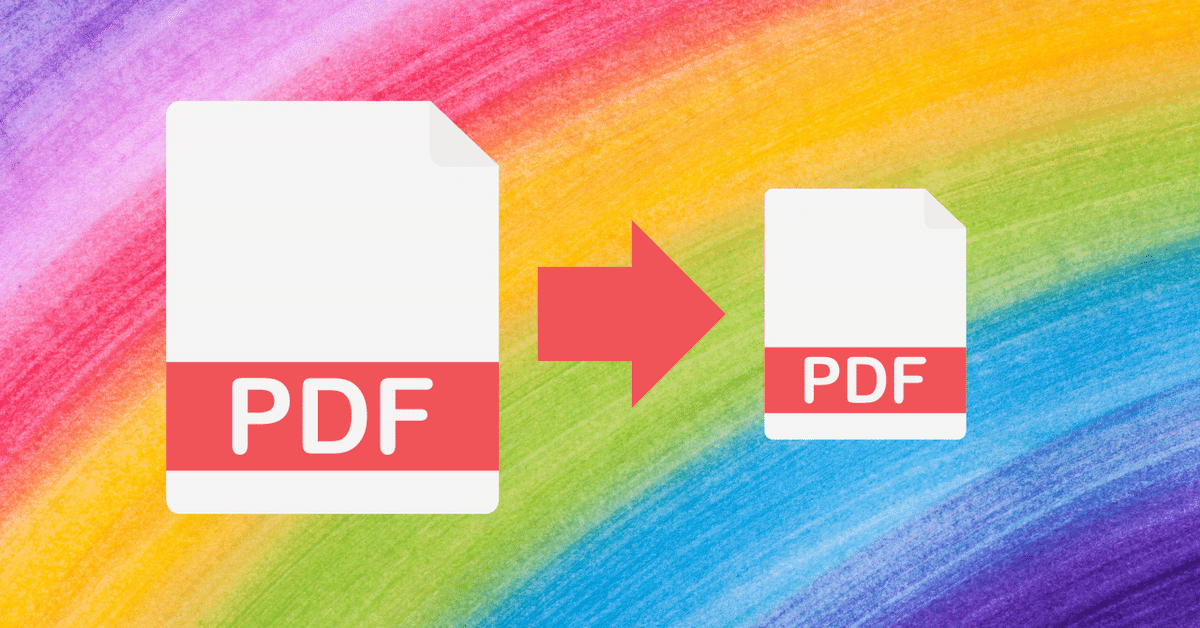
PDFをA4サイズに変更するwebアプリの作成(Google Apps Script)
Google Apps Script(GAS)を使って、PDFをA4サイズに変更するwebアプリを作成したいと思います。
PDFの操作を行うのは、pdf-libというJavascript用のPDFライブラリを使用していきたいと思います。
※なぜこれを作ったかと言うと、FigmaでPDFをエクスポートした時に、PDFのサイズを選べなかったので、必要に迫られてです。
コード.gs
function doGet(e) {
const template = HtmlService.createTemplateFromFile('index');
return template.evaluate()
.setTitle("PDFをA4サイズに変更する")
}
index.html
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PDFをA4にリサイズ</title>
<script src="https://cdn.jsdelivr.net/npm/pdf-lib@1.17.1/dist/pdf-lib.min.js"></script>
</head>
<body>
<h1>PDFをA4サイズにリサイズ</h1>
<input type="file" id="uploadPdf" accept="application/pdf">
<button onclick="resizeToA4()">PDFをA4サイズにリサイズ</button>
<script>
const pageSizes = {
a4: {
width: 595.28, // A4サイズ (210mm x 297mm) をポイントに換算
height: 841.89,
}
};
// A4にリサイズする関数
const resizeToA4 = async () => {
const fileInput = document.getElementById('uploadPdf');
const file = fileInput.files[0];
if (!file) {
alert('PDFファイルを選択してください。');
return;
}
// PDFファイルをバイナリとして読み込む
const arrayBuffer = await file.arrayBuffer();
const resizedPdfBytes = await resizeToPageFormat(new Uint8Array(arrayBuffer), 'a4');
// リサイズしたPDFをダウンロードする
const blob = new Blob([resizedPdfBytes], { type: 'application/pdf' });
const link = document.createElement('a');
link.href = URL.createObjectURL(blob);
link.download = 'resized_to_a4.pdf';
link.click();
};
// PDFのページサイズを指定されたフォーマットに変更する関数
const resizeToPageFormat = async (snapshot, paper_format) => {
// PDFファイルを読み込む
const pdfDoc = await PDFLib.PDFDocument.load(snapshot);
const new_size = pageSizes[paper_format || 'a4'];
const new_size_ratio = Math.round((new_size.width / new_size.height) * 100);
// 全てのページを取得
const pages = pdfDoc.getPages();
pages.forEach(page => {
const { width, height } = page.getMediaBox();
const size_ratio = Math.round((width / height) * 100);
// 元のページと新しいフォーマットの比率が1%以上違う場合
if (Math.abs(new_size_ratio - size_ratio) > 1) {
// ページサイズをA4に変更
page.setSize(new_size.width, new_size.height);
const scale_content = Math.min(new_size.width / width, new_size.height / height);
// 内容をスケール
page.scaleContent(scale_content, scale_content);
const scaled_diff = {
width: Math.round(new_size.width - scale_content * width),
height: Math.round(new_size.height - scale_content * height),
};
// 新しいページフォーマットで内容を中央に配置
page.translateContent(Math.round(scaled_diff.width / 2), Math.round(scaled_diff.height / 2));
} else {
// 比率が近い場合はそのままスケール
page.scale(new_size.width / width, new_size.height / height);
}
});
// 修正されたPDFをバイナリデータとして保存
return pdfDoc.save();
};
</script>
</body>
</html>
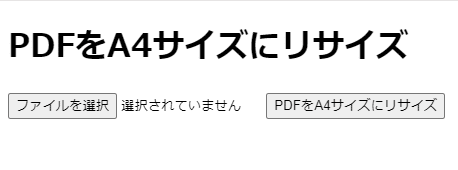