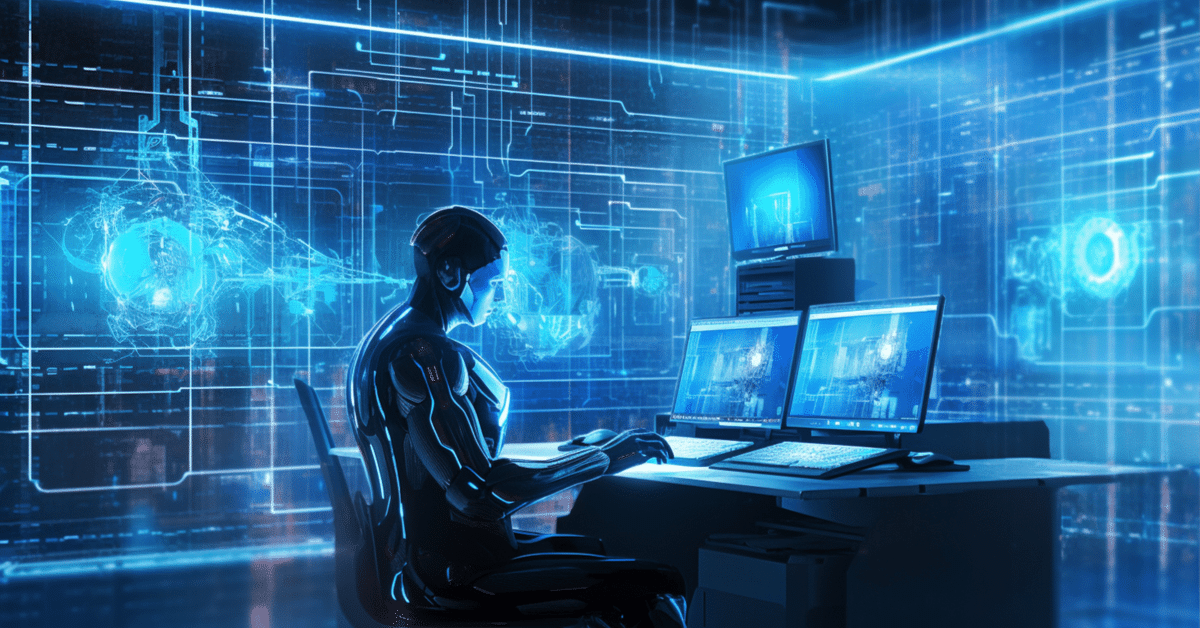
HTML5 レベル2 過去問まとめ(WebブラウザにおけるJavaScript API)
イベント
ページ上で発生する様々な操作、表所の変更など
イベントハンドラ / イベントリスナ
イベントハンドラ:
onload プロパティを使用して、
イベントが発生したときに実行される関数を設定する
1つのHTML要素に1つのイベントハンドラしか設定できない
イベントリスナー:
addEventListener メソッドを使用して、
イベントが発生したときに実行される関数を追加する
1つのHTML要素に複数のリスナーを設定できる
メモ: 設定済のイベントハンドラ、イベントリスナは削除できる
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Onload Event Handler Example</title>
</head>
<body>
<h1>Onload Event Handler Example</h1>
<script>
window.onload = function() {
console.log('Page fully loaded');
};
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Onload Event Listener Example</title>
</head>
<body>
<h1>Onload Event Listener Example</h1>
<script>
window.addEventListener('load', function() {
console.log('Page fully loaded - listener 1');
});
window.addEventListener('load', function() {
console.log('Page fully loaded - listener 2');
});
</script>
</body>
</html>
例) ボタンクリック時にfoo()関数を呼び出すイベントリスナ
button.addEventListener('click', foo, true);
イベントハンドラ/イベントリスナの関数の設定の書き方
・イベントハンドラとイベントリスナーの両方で、
関数名を記載
関数の呼び出しを記載
のやり方を書ける
・関数名を記載する場合は、イベントが発生したときに関数が実行される
・関数の呼び出しを記載する場合は、その場で関数が実行され、その戻り値がイベントハンドラやイベントリスナーに設定される
イベントハンドラ - 関数名を記載
<button id="myButton">Click me</button>
<script>
var button = document.getElementById('myButton');
function handleClick() {
console.log('Button clicked!');
}
// 関数名をイベントハンドラに設定
button.onclick = handleClick;
</script>
イベントハンドラ - 関数の呼び出しを記載
<button id="myButton">Click me</button>
<script>
var button = document.getElementById('myButton');
function handleClick() {
console.log('Button clicked!');
}
// 関数の呼び出しをイベントハンドラに設定(意図しない動作)
button.onclick = handleClick(); // handleClickが即座に実行され、その戻り値がonclickに設定される
</script>
イベントリスナ - 関数名を記載
<button id="myButton">Click me</button>
<script>
var button = document.getElementById('myButton');
function handleClick() {
console.log('Button clicked!');
}
// 関数名をイベントリスナーに設定
button.addEventListener('click', handleClick);
</script>
イベントリスナ - 関数の呼び出しを記載
<button id="myButton">Click me</button>
<script>
var button = document.getElementById('myButton');
function handleClick() {
console.log('Button clicked!');
}
// 関数の呼び出しをイベントリスナーに設定(意図しない動作)
button.addEventListener('click', handleClick()); // handleClickが即座に実行され、その戻り値がイベントリスナーに設定される
</script>
onloadイベント
起動タイミング: DOM, 画像を含むページ全体、HTML要素の読み込みが完了した時に起動する
・Documentオブジェクトはonloadイベントハンドラをプロパティとして持つ
・Windowオブジェクトのloadイベント起動時には、ページ内の全ての画像は読み込まれている
・Windowオブジェクトのloadイベント起動時には、ページ内の全てのDOMは読み込まれている
注意: loadイベントはページがキャッシュから読み込まれた場合は起動しない
フォームに関するイベント
blur
フォーカスが外れた時に発生する
change
入力要素の値が変更されたとき
contextmenu
右クリックメニューが表示されたとき
focus
入力要素がフォーカスを受けた時
input
入力要素の値が入力/変更されたとき
invalid
入力要素が無効な値を持つとき
select
テキストが選択されたとき
submit
フォームが送信されたとき
formchange
フォームの内容が変更されたとき
forminput
フォームの入力が発生した時
注意: unfocusというイベントはない
document.addEventListener('DOMContentLoaded', function() {
var form = document.getElementById('myForm');
var nameInput = document.getElementById('name');
var ageInput = document.getElementById('age');
var commentsTextarea = document.getElementById('comments');
nameInput.addEventListener('blur', function() {
console.log('Name input lost focus');
});
ageInput.addEventListener('change', function() {
console.log('Age input value changed');
});
form.addEventListener('contextmenu', function(event) {
event.preventDefault();
console.log('Context menu opened on form');
});
nameInput.addEventListener('focus', function() {
console.log('Name input gained focus');
});
commentsTextarea.addEventListener('input', function() {
console.log('Comments textarea input event');
});
nameInput.addEventListener('invalid', function() {
console.log('Name input is invalid');
});
commentsTextarea.addEventListener('select', function() {
console.log('Text selected in comments textarea');
});
form.addEventListener('submit', function(event) {
event.preventDefault();
console.log('Form submitted');
});
form.addEventListener('formchange', function() {
console.log('Form change event');
});
form.addEventListener('forminput', function() {
console.log('Form input event');
});
});
キーボードのイベント
keydown
キーが押されたとき
keypress
キーが押されている時
keyup
押されたキーが離されたとき
注意: keyleaveというイベントはない
マウス操作のイベント
click
クリックした時
dbclick
ダブルクリックした時
drag
要素やテキストをドラッグしている時
dragend
要素やテキストのドラッグ操作が終了した時
dragenter
ドラッグされた要素やテキストがドロップ対象に入った時
dragleave
ドラッグされた要素やテキストがドロップ対象から離されたとき
dragover
ドラッグされた要素やテキストがドロップ対象にドラッグされている時
dragstart
要素やテキストのドラッグ操作を開始した時
drop
ドラッグされた要素やテキストがドロップされたとき
mousedown
要素上でマウスボタンが押されたとき
mousemove
マウスポインタが要素上を移動している時
mouseout
マウスポインタが要素上から外れた時
mouseover
マウスポインタが要素に入った時
mouseup
要素上で押されたマウスボタンが離されたとき
mousewheel
マウスホイールを操作した時
scroll
ページや要素がスクロールされたとき
タッチスクリーンのイベント
touchstart
要素に指がおかれたとき
touchmove
要素に沿って指を移動した時
touchend
要素から指が離れた時
入れ子構造になっている要素にイベントを追加した場合
イベントのバブリング(またはバブルアップ)
イベントのバブリング(またはバブルアップ)
イベントが発生した要素から親要素へと順次伝播する仕組み
2つのフェーズがある。
1) キャプチャリングフェーズ
イベントリスナーを設定する際に第三引数に true を指定する
親要素 -> ターゲット要素 の順にイベント実行
2) バブリングフェーズ
デフォルトでは、イベントリスナーはバブリングフェーズで呼び出す
ターゲット要素 -> 親要素 の順にイベント実行
例1) バブリングフェーズ
イベントのバブリング(またはバブルアップ)
イベントが発生した要素から親要素へと順次伝播する仕組み
#inner 要素がクリックされた場合、
1) まず #inner のイベントハンドラが実行される
2) その後に #outer のイベントハンドラが実行される
以下が表示される
INNER is clicked
OUTER is clicked
<!DOCTYPE html>
<html>
<body>
<div id="outer">
OUTER
<p id="inner">INNER</p>
</div>
<script type="text/javascript">
const outer = document.getElementById("outer");
const inner = document.getElementById("inner");
outer.onclick = function() {
console.log("OUTER is clicked");
}
inner.onclick = function() {
console.log("INNER is clicked");
}
</script>
</body>
</html>
例2) キャプチャリングフェーズ
下記はイベントリスナの第3引数にtrueをしているので、キャプチャリングフェーズとなる。
親要素からイベント実行する
以下が表示される
A is clicked
B is clicked
<!DOCTYPE html>
<html>
<body>
<div id="a">
<p>A</p>
<p id="b">B</p>
</div>
<script type="text/javascript">
const a = document.getElementById("a");
const b = document.getElementById("b");
a.addEventListener('click', function() {
console.log("A is clicked");
}, true);
b.addEventListener('click', function() {
console.log("B is clicked");
}, true);
</script>
</body>
</html>
カスタムイベント
例1)
・ボタンをクリックすると、まず現在日時を表示する
・10秒ごとに現在日時を表示する
<!DOCTYPE html>
<html>
<body>
<input type="button" value="現在日時取得" id="btn">
<span id="time"></span>
<script type="text/javascript">
const button = document.getElementById("btn");
button.addEventListener('click', displayTime);
var event = new Event("click");
window.setInterval(function() {
button.dispatchEvent(event);
}, 10000);
function displayTime() {
var now = new Date();
document.getElementById("time").textContent = now.toLocaleString();
}
</script>
</body>
</html>
例2) Eventインタフェースのプロパティを使う
Eventインタフェースの主なプロパティ/メソッド
target
イベント発生元のオブジェクトの参照を取得
timestamp
イベントが生成された時刻をミリ秒単位で取得
1000ミリ秒 = 1秒
type
発生したイベントの方を取得
preventDefault()
既定のイベント処理をキャンセルする
submitボタンの処理に記載したら、リクエストをサーバに送信されなくなる
・テキストボックスからフォーカスが外れるとイベントが発生する
・イベント発生時、テキストボックスが未入力ならダイアログが表示される
<!DOCTYPE html>
<html>
<body>
<form method="post" action="/hoge">
<label for="foo">Text:</label>
<input type="text" id="foo">
<button>OK</button>
</form>
<script type="text/javascript">
document.getElementById("foo").addEventListener('blur', function(e) {
if(e.target.value === "") {
alert("no input");
}
}, false);
</script>
</body>
</html>
フォーム送信に関するメソッド
submit()
form要素の内容を送信する
reset()
フォーム内容のinput要素の入力をリセットする
注意: send(), cancel() はform要素のオブジェクトにはない
キーボードイベントの入力情報の取得
keyboardEventオブジェクトの主なプロパティ
code
キーコードを取得する
key
キーの値を取得する
注意: valueというプロパティはない
HTML文書を読み込み際に発生するイベント順
要素.onload
img要素などを読み込む
document.addEventListener('DOMContentLoaded'
DOMツリーの構築が完了すると起動する
window.onload
DOM、画像を含むページ全体の読み込みが完了した時に起動する
下記の読み込む順は、
DOMContent, image, pageとなる
<img id="img" src="text.jpg">
<script type="text/javascript">
var img = document.getElementById('img');
img.onload = function() {
alert('image');
};
document.addEventListener('DOMContentLoaded', function() {
alert('DOMContent');
});
window.onload = function() {
alert('page');
};
</script>
DOM
・HTML要素を生成、追加できる
・W3Cが仕様を策定している
・XMLの属性を操作できる
・windowオブジェクトのdocumentプロパティ経由で操作する
(グローバルオブジェクト経由じゃない)
fomr要素の取得
document.formsプロパティを使う
document.forms[インデックス]
document.forms.フォーム名
document.forms["フォーム名"]
document.forms[0])
document.forms.inputForm
document.forms["inputForm"]
<form action="foo" method="GET" name="inputForm">
<input type="text" name="input" value="bar">
<input type="submit" value="Submit">
</form>
<script type="text/javascript">
console.log(document.forms[0]); // <form action="foo" method="GET" name="inputForm"></form>
console.log(document.forms.inputForm); // <form action="foo" method="GET" name="inputForm"></form>
console.log(document.forms["inputForm"]);// <form action="foo" method="GET" name="inputForm"></form>
</script>
formのinputを取得する
document.getElementById("foge")
document.forms.inputForm.bar
document.forms[0][0]
document.forms["inputForm"]["bar"]
<form action="foo" method="GET" name="inputForm">
<input type="text" name="bar" value="foge">
</form>
console.log(document.forms.inputForm.bar);
console.log(document.forms[0][0]);
console.log(document.forms["inputForm"]["bar"]);
innerHTMLでHTML要素を追加する例
要素.innerHTML += 文字列、タグなど
+=と書かないといけない
= だと上書きされて、その値しか入らない
<ul id="list"></ul>
var list = document.getElementById('list');
list.innerHTMl += "<li>Item 1</li>";
list.innerHTMl += "<li>Item 2</li>";
list.innerHTMl += "<li>Item 3</li>";
document.createElementでHTML要素を生成、操作
document.createElement()
document.getElementById()
で生成、取得したHTML要素を操作できる
children:
子要素を取得
innerHTML:
HTML要素のテキストを設定
parentElement:
親要素を取得
style:
HTML要素のスタイルを設定
textContent:
HTML要素のテキストコンテンツを設定
value:
HTML要素の入力値を取得
appendChild():
新しい子要素を追加
hasAttribute():
属性の存在を確認
insertBefore():
新しい要素を既存の要素の前に挿入
remove():
要素を削除
removeAttribute():
属性を削除
setAttribute():
属性を設定
setAttributeNode():
Attr ノードを設定
createAttribute()
属性に値を設定する
document.addEventListener('DOMContentLoaded', function() {
// 要素を取得
var container = document.getElementById('container');
var myInput = document.getElementById('myInput');
var myParagraph = document.getElementById('myParagraph');
// children: 子要素の取得
console.log(container.children); // 出力: HTMLCollection(2) [input#myInput, p#myParagraph]
// innerHTML: HTMLコンテンツの設定
myParagraph.innerHTML = 'Updated <strong>paragraph</strong> content.';
console.log(myParagraph.innerHTML); // 出力: "Updated <strong>paragraph</strong> content."
// parentElement: 親要素の取得
console.log(myInput.parentElement); // 出力: <div id="container">
// style: インラインスタイルの設定
myParagraph.style.color = 'blue';
myParagraph.style.fontSize = '20px';
console.log(myParagraph.style.cssText); // 出力: "color: blue; font-size: 20px;"
// textContent: テキストコンテンツの設定
myParagraph.textContent = 'Updated text content only.';
console.log(myParagraph.textContent); // 出力: "Updated text content only."
// value: 入力値の取得と設定
console.log(myInput.value); // 出力: "Hello"
myInput.value = 'Updated value';
console.log(myInput.value); // 出力: "Updated value"
// appendChild(): 子要素の追加
var newElement = document.createElement('div');
newElement.textContent = 'I am a new element';
container.appendChild(newElement);
console.log(container.children); // 出力: HTMLCollection(3) [input#myInput, p#myParagraph, div]
// hasAttribute(): 属性の存在確認
console.log(myInput.hasAttribute('value')); // 出力: true
console.log(myInput.hasAttribute('placeholder')); // 出力: false
// insertBefore(): 要素の挿入
var anotherElement = document.createElement('span');
anotherElement.textContent = 'Inserted before input';
container.insertBefore(anotherElement, myInput);
console.log(container.children); // 出力: HTMLCollection(4) [span, input#myInput, p#myParagraph, div]
// remove(): 要素の削除
newElement.remove();
console.log(container.children); // 出力: HTMLCollection(3) [span, input#myInput, p#myParagraph]
// removeAttribute(): 属性の削除
myInput.removeAttribute('value');
console.log(myInput.hasAttribute('value')); // 出力: false
// setAttribute(): 属性の設定
myInput.setAttribute('placeholder', 'Enter text here');
console.log(myInput.getAttribute('placeholder')); // 出力: "Enter text here"
// setAttributeNode(): Attrノードの設定
var newAttr = document.createAttribute('data-custom');
newAttr.value = 'customValue';
myInput.setAttributeNode(newAttr);
console.log(myInput.getAttribute('data-custom')); // 出力: "customValue"
});
例1) createElement, insertBeforeで要素の前に配置する
<!DOCTYPE html>
<html>
<body>
<div id="container">
<span id="target">FLM</span>
</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
const spanElement = document.createElement('span');
spanElement.textContent = 'SE';
const divElement = document.getElementById('container');
const targetElement = document.getElementById('target');
divElement.insertBefore(spanElement, targetElement);
});
</script>
</body>
</html>
例2) createElement, appendChild で要素の前に配置する
<!DOCTYPE html>
<html>
<body>
<div id="container">
<span id="target">FLM</span>
</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
const spanElement = document.createElement('span');
spanElement.textContent = 'SE';
const divElement = document.getElementById('container');
divElement.appendChild(spanElement);
});
</script>
</body>
</html>
Elementオブジェクトのvalueでinput要素の入力値を取得
document.getEkementById("input1").addEventListener("submit", function(e){
for(var ele of e.target.children) {
if(ele.value === "") {
alert("Please fill out all fields");
e.preventDefault();
}
}
});
HTML要素の非表示の設定
非表示にすると言ってもまた、後で表示する場合もあれば、表示/非表示を切り替えたいだけということもある
方法としては、
1)
setAttribute("class", "hidden")
2)
style = "display: none";
document.getElementById('item').setAttribute("class", "hidden");
document.getElementById('item').style = "display: none";
WIndowオブジェクト
・ブラウザ上でのグローバルオブジェクト
ブラウザの操作機能のためのオブジェクト
・windowsオブジェクトのプロパティにアクセスする際、window. を省略できる
・ブラウザのウィンドウを操作できる
・windowオブジェクト経由でDocumentオブジェクトにアクセスできる
Windowオブジェクトを通してDocumentオブジェクト、Historyオブジェクトなどにアクセスできる
windowオブジェクトのプロパティ
console
デベロッパーツールのコンソールにアクセスする
メッセージ出力する
document
DOMにアクセスする
HTML操作ができる
indexedDB
Indexed Databaseにアクセスする
データベースを開き、オブジェクトストアを作成できる
location
Locationオブジェクトにアクセスする
localStorage
sessionStorage
Web Storageにアクセスする
navigator
Navigatorオブジェクトにアクセスする
worker
Workerコンストラクタにアクセスする
例)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Window Object Properties Example</title>
</head>
<body>
<button id="reloadButton">Reload Page</button>
<div id="storageExample">Storage Example</div>
<div id="locationExample">Location Example</div>
<div id="navigatorExample">Navigator Example</div>
<script src="script.js"></script>
</body>
</html>
document.addEventListener('DOMContentLoaded', function() {
// console
console.log('This is a message logged to the console');
// document
const storageDiv = document.getElementById('storageExample');
storageDiv.textContent = 'LocalStorage and SessionStorage example';
// indexedDB
if ('indexedDB' in window) {
let request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
let db = event.target.result;
db.createObjectStore('myObjectStore', { keyPath: 'id' });
};
request.onsuccess = function(event) {
console.log('IndexedDB opened successfully');
};
request.onerror = function(event) {
console.error('IndexedDB error:', event.target.errorCode);
};
} else {
console.error('IndexedDB is not supported in this browser');
}
// location
const locationDiv = document.getElementById('locationExample');
locationDiv.textContent = `Current URL: ${window.location.href}`;
document.getElementById('reloadButton').addEventListener('click', function() {
window.location.reload();
});
// localStorage
localStorage.setItem('myKey', 'myValue');
console.log('LocalStorage item:', localStorage.getItem('myKey'));
// sessionStorage
sessionStorage.setItem('sessionKey', 'sessionValue');
console.log('SessionStorage item:', sessionStorage.getItem('sessionKey'));
// navigator
const navigatorDiv = document.getElementById('navigatorExample');
navigatorDiv.textContent = `User Agent: ${navigator.userAgent}`;
// worker
if (window.Worker) {
const worker = new Worker('worker.js');
worker.onmessage = function(event) {
console.log('Message from worker:', event.data);
};
worker.postMessage('Hello, worker!');
} else {
console.error('Web Workers are not supported in this browser');
}
});
self.onmessage = function(event) {
console.log('Message received in worker:', event.data);
self.postMessage('Hello from the worker!');
};
Windowオブジェクトのメソッド
alert()
警告ダイアログを表示する
confirm()
OK, Cancelボタンを持つ確認ダイアログを表示する
prompt()
入力ダイアログを表示する
入力値を返す
open()
指定したURLのwebページを開く
postMessage()
windowオブジェクト間でクロスオリジン通信を行うためのメソッド
注意: post(), send(), sendMessage()というメソッドはない
window.alert("アラート");
window.confirm("確認ダイアログ");
window.prompt("この質問はどうする?入力値を返す");
window.open("next.html", "next");
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Window Object Methods Example</title>
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', function() {
const alertButton = document.getElementById('alertButton');
const confirmButton = document.getElementById('confirmButton');
const promptButton = document.getElementById('promptButton');
const openButton = document.getElementById('openButton');
// alert(): 警告ダイアログを表示する
alertButton.addEventListener('click', function() {
window.alert("アラート");
});
// confirm(): OK, Cancelボタンを持つ確認ダイアログを表示する
confirmButton.addEventListener('click', function() {
window.confirm("確認ダイアログ");
});
// prompt(): 入力ダイアログを表示する
promptButton.addEventListener('click', function() {
window.prompt("この質問はどうする?入力値を返す");
});
// open(): 新しいウィンドウを開く
openButton.addEventListener('click', function() {
window.open("next.html", "next");
});
});
</script>
</head>
<body>
<button id="alertButton">Show Alert</button>
<button id="confirmButton">Show Confirm</button>
<button id="promptButton">Show Prompt</button>
<button id="openButton">Open Window</button>
</body>
</html>
postMessageを使った例
var nextPage = window.open("next.html", "next");
nextPage.postMessage("message", "http://localhost:8080");
MessageEventオブジェクトのプロパティ
data
postMessage()で送信されたデータを取得する
origin
messageイベントを発生させたwindowオブジェクトのオリジンを取得
source
messageイベントを発生させたwindowオブジェクトを取得
Windowオブジェクトの主なイベント
change
webページ上のDOMが変更されると起動
devicemotion
デバイスの傾きが変更されると起動
deviceorientation
デバイスの加速度センサの方向が変更されたら起動
hashchange
URLのハッシュが変化すると起動
load
webページがロードされたら起動
message
postMessage()を受信すると起動
popstate
履歴が変更されるたびに起動
resize
ブラウザのサイズを変更すると起動
scroll
スクロールすると起動
storage
web storageの保存領域に変更が発生した場合に起動
ブラウザの座標情報のプロパティ/メソッド
innerHeight
スクロールバーを含むブラウザviewportの高さ
innerWidth
スクロールバーを含むブラウザviewportの幅
outerHeight
ブラウザ全体の高さ
outerWidth
ブラウザ全体の幅
pageXOffset
水平スクロールの位置
pageYOffset
垂直スクロールの位置
screeX
スクリーンの左端からのブラウザ位置
screenY
スクリーンの上端からのブラウザ位置
scrollBy(x軸方向, y軸方向)
scrollTo(x軸方向, y軸方)
scrollBy は現在のスクロール位置から引数で指定分スクロールする
scrollToは現在の位置に関わらず、指定された位置にスクロールする
windowオブジェクトのブラウザ操作
close()
ウィンドウを閉じる
moveBy()
ウィンドウを相対値で移動する
moveTo()
ウィンドウを絶対値で移動する
open()
ウィンドウを開く
reseizeBy()
ウィンドウを相対値でサイズ変更する
reseizeTo()
ウィンドウを絶対値でサイズ変更する
setInterval(), setTimeout, clearTimeout(), clearTimeInterval()
setInterval()
指定した時間間隔で繰り返し関数を実行
setTimeout()
指定した遅延時間後に関数を1回だけ実行
clearTimeout()
setTimeout() によって設定されたタイマーをキャンセル
clearTimeInterval()
setInterval() によって設定された繰り返しタイマーをキャンセル
document.querySelector()
・id属性名、class属性名、要素名でHTML要素を取得できる
・一致する要素がない場合はnullを返す
エラーは発生しない
注意: ID属性名を指定する場合は、#ID名と書く
document.getElementByIdと指定が違う
間違った例)
document.querySelector(".input") は最初に見つかった class="input" の要素を取得する
しかし、最初のinputにvalueが設定されてない
出力結果: コンソールに何も表示されない
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Window Object Methods Example</title>
<script type="text/javascript">
document.addEventListener('DOMContentLoaded', function() {
var element = document.querySelector(".input");
console.log(element.value);
});
</script>
</head>
<body>
<input type="text" class="input">
<input type="button" class="input" value="click">
</body>
</html>
document.querySelectorAll
・セレクタに一致する複数の要素を取得できる
・1つの要素を指定するには、
document.querySelectorAll("要素")[0]
というように配列のように添え字で指定して書く
( xxx.name みたいに書けない)
・forEach(), length などが使える
要素の指定のやり方
document.getElementByIdは指定したID属性の値と一致する要素を取得する
セレクタのように「#」をつけない
正しい例
<input type="text" name="secret" id="secret">
document.querySelectorAll("#secret")[0]
document.querySelector("#secret")
document.querySelector("[name='secret']")
document.getElementById('secret')
間違いの例
<input type="text" name="secret" id="secret">
document.getElementById('#secret')
Historyオブジェクト
ブラウザの履歴を操作するためのオブジェクト
履歴の追加、変更、ページの移動が出来る
Historyオブジェクトのプロパティ、メソッド
length
履歴数の取得
state
履歴の状態を取得
go()
指定した位置の履歴に移動
back()
履歴を1つ戻る
forward()
履歴を1つ進む
pushState()
履歴を追加する
replaceState()
現在の履歴を修正する
pushStateの例
第3引数でパス名を指定することでURLが決まる
history.pushState({data: "foo"}, "foo", "foo");
新しい履歴エントリが追加される
URLが http://localhost/foo に変更される
history.pushState({data: "bar"}, "bar", "bar");
新しい履歴エントリが追加される
URLが http://localhost/bar に変更される
history.pushState({data: "foge"}, "foge", "foge");
新しい履歴エントリが追加される
URLが http://localhost/foge に変更される
URL: http://localhost/index
window.onload = function() {
history.pushState({data: "foo"}, "foo", "foo");
history.pushState({data: "bar"}, "bar", "bar");
history.pushState({data: "foge"}, "foge", "foge");
};
上記を以下のような変更にする場合
http://localhost/index
-> http://localhost/index/foo
-> http://localhost/index/foo/bar
-> http://localhost/index/foo/bar/foge
window.onload = function() {
// URLを `http://localhost/index/foo` に変更し、履歴エントリを追加
history.pushState({data: "foo"}, "foo", "index/foo");
// URLを `http://localhost/index/foo/bar` に変更し、履歴エントリを追加
history.pushState({data: "bar"}, "bar", "index/foo/bar");
// URLを `http://localhost/index/foo/bar/foge` に変更し、履歴エントリを追加
history.pushState({data: "foge"}, "foge", "index/foo/bar/foge");
};
history.pushState({data: "foo"}, "foo", "foo");
URLが http://localhost/foo に変更される
history.pushState({data: "bar"}, "bar", "bar");
URLが http://localhost/bar に変更される
history.pushState({data: "foge"}, "foge", "foge");
URLが http://localhost/foge に変更される
history.replaceState({data: "foo"}, "foo", "piyo");
現在の履歴エントリを新しいエントリで置き換え
URLが http://localhost/piyo に変更される
history.go(2);
現在のURL: http://localhost/piyo の状態から2つ進むため、履歴が変わらず http://localhost/piyo のまま
履歴スタックの最後のエントリにいるため、URLは変わらない
history.back(2)
現在のURL: http://localhost/piyo から2つ前に戻る
履歴エントリ: http://localhost/index
-> http://localhost/foo
-> http://localhost/bar
-> http://localhost/piyo
2つ前のエントリは http://localhost/foo です。
URL: http://localhost/index
window.onload = function() {
history.pushState({data: "foo"}, "foo", "foo");
history.pushState({data: "bar"}, "bar", "bar");
history.pushState({data: "foge"}, "foge", "foge");
history.pushState({data: "foo"}, "foo", "piyo");
history.go(2);
};
popstateイベントは履歴が変更されるたびに起動する
pushStateメソッドなどで履歴を追加しても起動しない
history.goなどで起動する
pushStateだけだとコンソールに何も表示しない
window.onload = function() {
history.pushState({data: "foo"}, "foo", "foo");
history.pushState({data: "bar"}, "bar", "bar");
};
window.onpopstate = function() {
console.log(history.state);
};
Locationオブジェクト
Locationオブジェクトのプロパティ、メソッド
href
URL全体を取得
protocol
「:」まで含めたスキーム
host
ポート番号を含むホスト名
hostname
ホスト名
port
ポート番号
pathname
URLのパス
search
「?」を含むクエリ文字列
hash
「#」を含むハッシュ
assign()
URLのリソースを読み込む
現在のURLに履歴が残る
replace()
URLのリソースを読み込む
現在のURLに履歴が残らない
reload()
webページを再読み込みする
location.assign() と location.replace() の違い
初期状態: http://localhost/index1.html
location.assign("page2.html"): http://localhost/page2.html
現在のページを履歴に残す
history.pushState(null, "", "page3.html"): http://localhost/page3.html
history.pushState(null, "", "page4.html"): http://localhost/page4.html
location.replace("page5.html"): http://localhost/page5.html
現在の履歴エントリを新しいURLで置き換える
http://localhost/page4.html が
http://localhost/page5.html に置き換えられる
この時点で履歴は以下になる
http://localhost/index1.html
http://localhost/page2.html
http://localhost/page3.html
http://localhost/page5.html
history.back(): http://localhost/page3.html
1つ前の履歴に戻る
page1.htm lの URL は http://localhost/index1.html とする
location.assign("page2.html");
history.pushState(null, "", page3.html);
history.pushState(null, "", page4.html);
location.replace("page5.html");
history.back(); // page3.html に戻る
// この時点での履歴は page1.html -> page2.html -> page3.html となっている
Consoleオブジェクト
assert()
第1引数がfalseならコンソールに情報を出力する
clear()
コンソールをクリア
debug()
log()と同じ
dir()
オブジェクトを階層構造で出力
dirxml()
HTML要素をツリー形式で出力
error()
エラーメッセージを出力
info()
情報を出力
log()
ログを出力
profile()
プロファイラを開始
profleEnd()
プロファイラを終了
trace()
スタックトレースを出力
プログラムの実行家庭の記録を出力できる
warn()
警告を出力
ブラウザのプロファイラ
各処理の実行時間などを記録し、パフォーマンスの改善のためのツール
出来ること:
・各処理の実行時間などを記録し、パフォーマンスの改善に役に立つ
出来ないこと:
・web storageなどで保存したデータを確認する
・JavaScriptのコードをデバッグする
・セキュリティ情報を確認する