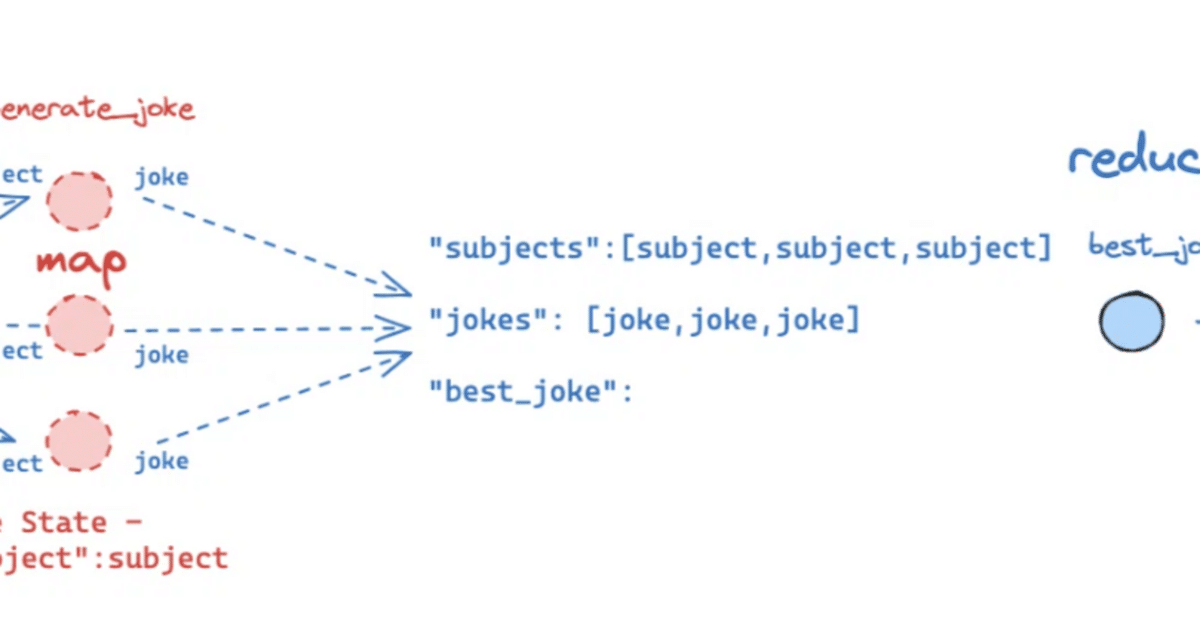
LangGraphによるMap-Reduce実装:柔軟に並列実行用ブランチを作成する方法
今回は以下のlangchainの記事を紹介しながら、以前紹介したTree of thoughのアルゴリズムをmap-reduceを用いたlanngarphで実装してみます。
map-reduce操作は、効率的なタスク分解と並列処理を実現するための重要な手法です。この方法では、大きなタスクを小さなサブタスクに分割し、それらを並列で処理した後、結果を集約します。しかし、この実装には主に二つの課題があります。一つは、グラフ設計時にサブタスクの数が不明な場合があること、もう一つは各サブタスクに異なる入力状態が必要なことです。
これらの課題に対して、LangGraphは`Send` APIを用いた解決策を提供しています。この APIは条件付きエッジを活用し、異なる状態を複数のノードインスタンスに配布することができます。さらに、送信される状態がコアグラフの状態と異なることを許容するため、柔軟で動的なワークフロー管理が可能となります。
このアプローチにより、複雑な並列処理タスクを効率的に実行することができ、大規模なデータ処理や複雑な計算タスクなどに適用できます。LangGraphの`Send` APIは、map-reduce操作の実装における柔軟性と効率性を大幅に向上させる革新的なソリューションと言えるでしょう。
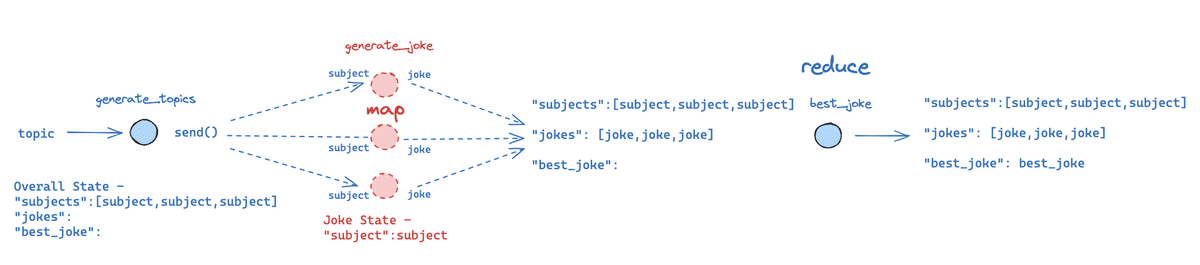
利用例(Tree of thought
これから紹介するコードは、LangGraphを使用してMap-Reduce パターンを実装し、成長する都市の公共交通機関改善のための解決策を生成、評価、分析するプロセスを自動化しています。Sendの使用方法を含め、主要な部分を説明します:
全体の流れ:
解決策の生成
各解決策の評価
各評価の深堀り分析
最終的なランキング
StateGraphの使用:
コードはStateGraphを使用してワークフローを定義しています。これにより、異なるステップ間でのデータの流れを管理します。Sendの使用: Sendは、Map-Reduceパターンを実装する上で重要な役割を果たしています。 a. continue_to_evaluation 関数:
def continue_to_evaluation(state: OverallState):
return [Send("evaluate_solution", {"solution": s}) for s in state["solutions"]]
この関数は、生成された各解決策に対して「evaluate_solution」ノードを呼び出します。各解決策は別々に評価されます。 b. continue_to_deep_thought 関数:
def continue_to_deep_thought(state: OverallState):
return [Send("deepen_thought", {"solution": r}) for r in state["reviews"]]
Map-Reduce の実装:
Map: 解決策の生成、評価、深堀り分析の各ステップで、個別の項目(解決策や評価)に対して並行処理を行います。
Reduce: 最終的に rank_solutions 関数で、すべての深堀り分析結果を集約してランキングを生成します。
構造化出力:
各ステップで model.with_structured_output() を使用して、AIモデルの出力を構造化しています。これにより、後続の処理が容易になります。
このアプローチにより、複雑な思考プロセスを模倣し、大量の情報を効率的に処理することができます。Sendの使用は、この過程で動的かつ柔軟なワークフロー管理を可能にしています。
実装
import operator
from typing import Annotated, TypedDict
from langchain_core.pydantic_v1 import BaseModel
from langchain_anthropic import ChatAnthropic
from langgraph.constants import Send
from langgraph.graph import END, StateGraph, START
# Define the model
model = ChatAnthropic(model="claude-3-5-sonnet-20240620")
# Define the prompts
step1_prompt = """Step 1: I have a problem related to {input}. Could you brainstorm three distinct solutions? Please consider a variety of factors such as {perfect_factors}"""
step2_prompt = """Step 2: For each of the three proposed solutions, evaluate their potential. Consider their pros and cons, initial effort needed, implementation difficulty, potential challenges, and the expected outcomes. Assign a probability of success and a confidence level to each option based on these factors.
Solutions:
{solutions}"""
step3_prompt = """Step 3: For each solution, deepen the thought process. Generate potential scenarios, strategies for implementation, any necessary partnerships or resources, and how potential obstacles might be overcome. Also, consider any potential unexpected outcomes and how they might be handled.
Evaluation:
{review}"""
step4_prompt = """Step 4: Based on the evaluations and scenarios, rank the solutions in order of promise. Provide a justification for each ranking and offer any final thoughts or considerations for each solution.
Detailed analysis:
{deepen_thought_process}"""
# Define the output structures
class Solutions(BaseModel):
solutions: list[str]
class Review(BaseModel):
review: str
class DeepThought(BaseModel):
deep_thought: str
class RankedSolutions(BaseModel):
ranked_solutions: str
# Define the overall state
class OverallState(TypedDict):
input: str
perfect_factors: str
solutions: Annotated[list[str], operator.add]
reviews: Annotated[list[str], operator.add]
deep_thoughts: Annotated[list[str], operator.add]
ranked_solutions: str
# Define the state for individual solution processing
class SolutionState(TypedDict):
solution: str
# Graph components
def generate_solutions(state: OverallState):
prompt = step1_prompt.format(input=state["input"], perfect_factors=state["perfect_factors"])
response = model.with_structured_output(Solutions).invoke(prompt)
return {"solutions": response.solutions}
def evaluate_solution(state: SolutionState):
prompt = step2_prompt.format(solutions=state["solution"])
response = model.with_structured_output(Review).invoke(prompt)
return {"reviews": [response.review]}
def deepen_thought(state: SolutionState):
prompt = step3_prompt.format(review=state["solution"])
response = model.with_structured_output(DeepThought).invoke(prompt)
return {"deep_thoughts": [response.deep_thought]}
def rank_solutions(state: OverallState):
deep_thoughts = "\n\n".join(state["deep_thoughts"])
prompt = step4_prompt.format(deepen_thought_process=deep_thoughts)
response = model.with_structured_output(RankedSolutions).invoke(prompt)
return {"ranked_solutions": response.ranked_solutions}
# Define the mapping logic
def continue_to_evaluation(state: OverallState):
return [Send("evaluate_solution", {"solution": s}) for s in state["solutions"]]
def continue_to_deep_thought(state: OverallState):
return [Send("deepen_thought", {"solution": r}) for r in state["reviews"]]
# Construct the graph
graph = StateGraph(OverallState)
graph.add_node("generate_solutions", generate_solutions)
graph.add_node("evaluate_solution", evaluate_solution)
graph.add_node("deepen_thought", deepen_thought)
graph.add_node("rank_solutions", rank_solutions)
graph.add_edge(START, "generate_solutions")
graph.add_conditional_edges("generate_solutions", continue_to_evaluation, ["evaluate_solution"])
graph.add_conditional_edges("evaluate_solution", continue_to_deep_thought, ["deepen_thought"])
graph.add_edge("deepen_thought", "rank_solutions")
graph.add_edge("rank_solutions", END)
app = graph.compile()
# Call the graph
for s in app.stream({
"input": "improving public transportation in a growing city",
"perfect_factors": "cost, efficiency, environmental impact, and user experience"
}):
print(s)
実行結果
{'generate_solutions': {'solutions': ['Implement a Bus Rapid Transit (BRT) system', 'Develop a comprehensive bike-sharing program', 'Introduce an integrated smart card payment system']}}
{'evaluate_solution': {'reviews': ["\nI will evaluate the proposed solution: Develop a comprehensive bike-sharing program\n\nPros:\n1. Environmentally friendly transportation option\n2. Reduces traffic congestion and parking issues\n3. Promotes physical activity and public health\n4. Enhances urban mobility and accessibility\n5. Potential to attract tourists and boost local economy\n\nCons:\n1. High initial investment for infrastructure and bikes\n2. Ongoing maintenance costs\n3. Potential for vandalism or theft of bikes\n4. Weather-dependent usage\n5. May face resistance from car-centric communities\n\nInitial effort needed:\n1. Comprehensive city planning and feasibility studies\n2. Securing funding (public, private, or mixed)\n3. Developing partnerships with bike manufacturers and technology providers\n4. Creating a user-friendly app and payment system\n5. Establishing docking stations and bike lanes\n\nImplementation difficulty: Moderate to High\n- Requires coordination between multiple stakeholders (city planners, transportation departments, private partners)\n- Infrastructure changes needed (bike lanes, docking stations)\n- Technology integration for bike tracking and user management\n\nPotential challenges:\n1. Balancing bike availability across different areas of the city\n2. Ensuring safety for riders in mixed traffic environments\n3. Overcoming potential public resistance or skepticism\n4. Adapting the program for different seasons and weather conditions\n5. Competing with existing transportation options (e.g., ride-sharing services)\n\nExpected outcomes:\n1. Increased use of sustainable transportation\n2. Reduced carbon emissions from personal vehicles\n3. Improved public health due to increased physical activity\n4. Enhanced city image as a progressive, eco-friendly destination\n5. Potential reduction in traffic congestion\n\nProbability of success: 70%\nThe bike-sharing program has a good chance of success, especially if implemented thoughtfully and with strong community engagement. Many cities worldwide have successfully adopted similar programs, proving their viability.\n\nConfidence level: 75%\nWhile there are challenges to overcome, the potential benefits and growing global trend towards sustainable urban transportation support a relatively high confidence in this solution's potential success.\n"]}}
{'evaluate_solution': {'reviews': ["To evaluate the proposed solution of introducing an integrated smart card payment system, let's consider its potential, pros and cons, implementation factors, and expected outcomes:\n\n1. Potential:\nThe potential for an integrated smart card payment system is significant, as it can streamline transactions, improve customer experience, and potentially increase revenue for the transit system.\n\n2. Pros:\n- Convenient for users: One card for multiple transit modes\n- Faster transactions: Reduces queuing time\n- Data collection: Provides valuable insights on travel patterns\n- Reduced cash handling: Improves security and reduces costs\n- Flexible fare structures: Enables easy implementation of discounts and loyalty programs\n\n3. Cons:\n- High initial investment: Requires significant upfront costs\n- Technical complexity: Needs robust infrastructure and software\n- Privacy concerns: Handling of personal and financial data\n- Adoption challenges: Some users may resist the change\n\n4. Initial effort needed:\nThe initial effort required is substantial, including:\n- System design and planning\n- Hardware procurement and installation\n- Software development and integration\n- Staff training\n- Public education and marketing campaigns\n\n5. Implementation difficulty:\nImplementation difficulty is relatively high due to:\n- Complex integration with existing systems\n- Need for coordination across multiple transit agencies\n- Potential technical challenges and compatibility issues\n- Requirement for extensive testing and gradual rollout\n\n6. Potential challenges:\n- Technical glitches during initial deployment\n- Resistance from some user groups (e.g., elderly, low-income)\n- Ensuring system security and data protection\n- Maintaining system reliability and minimizing downtime\n\n7. Expected outcomes:\n- Improved efficiency in fare collection\n- Enhanced user experience and satisfaction\n- Better data for transit planning and optimization\n- Potential increase in ridership due to convenience\n- Long-term cost savings in fare collection and management\n\n8. Probability of success:\nGiven the track record of similar systems in other cities and the potential benefits, I would assign a probability of success of 75%.\n\n9. Confidence level:\nBased on the available information and the complexity of the project, I would assign a confidence level of 80% to this evaluation.\n\nIn conclusion, while introducing an integrated smart card payment system presents significant challenges and requires substantial initial investment, its potential benefits and long-term advantages make it a promising solution for improving the transit system. The relatively high probability of success and confidence level suggest that this option is worth serious consideration, provided that the implementation is well-planned and executed."]}}
{'evaluate_solution': {'reviews': ["Based on the given task, I will evaluate the potential of implementing a Bus Rapid Transit (BRT) system as a solution to improve urban transportation. I'll consider various factors and assign a probability of success and confidence level.\n\nEvaluation of Bus Rapid Transit (BRT) system:\n\nPros:\n1. Cost-effective compared to rail-based transit systems\n2. Faster implementation time than subway or light rail systems\n3. Increased passenger capacity compared to regular bus service\n4. Dedicated lanes reduce travel times and improve reliability\n5. Flexible and scalable - can be expanded or modified as needed\n6. Potential to reduce traffic congestion and carbon emissions\n\nCons:\n1. May require reallocation of road space, potentially reducing lanes for private vehicles\n2. Initial resistance from car users and businesses affected by lane changes\n3. Less capacity than heavy rail systems\n4. May not be as attractive to riders as rail-based options\n\nInitial effort needed:\n1. Comprehensive urban planning and traffic studies\n2. Design of dedicated bus lanes and stations\n3. Procurement of specialized BRT vehicles\n4. Installation of intelligent transportation systems (ITS) for real-time tracking and signal priority\n5. Public outreach and education campaigns\n\nImplementation difficulty: Moderate\n- While easier to implement than rail-based systems, BRT still requires significant infrastructure changes and coordination between various city departments.\n\nPotential challenges:\n1. Securing funding and political support\n2. Overcoming resistance from car-centric communities\n3. Ensuring seamless integration with existing transit systems\n4. Maintaining dedicated lanes and preventing unauthorized use\n5. Achieving high-quality service standards to attract ridership\n\nExpected outcomes:\n1. Improved public transit travel times and reliability\n2. Increased public transit ridership\n3. Reduced traffic congestion in BRT corridors\n4. Improved air quality and reduced carbon emissions\n5. Enhanced urban mobility and accessibility\n6. Potential for transit-oriented development along BRT corridors\n\nProbability of success: 75%\nThe relatively high probability of success is based on the proven track record of BRT systems in various cities worldwide, its cost-effectiveness, and the flexibility it offers in implementation and operation.\n\nConfidence level: 80%\nThe high confidence level is due to the extensive data available on BRT implementations globally, the clear benefits observed in many cities, and the adaptability of the system to various urban contexts. However, local factors and potential resistance could still impact the success of the project, hence not a higher confidence level.\n\nOverall, implementing a Bus Rapid Transit system appears to be a promising solution for improving urban transportation. Its cost-effectiveness, relatively quick implementation time, and proven success in other cities make it a strong contender. However, careful planning, strong political will, and effective public engagement will be crucial to overcome potential challenges and ensure its success."]}}
{'deepen_thought': {'deep_thoughts': ["\nTo deepen the thought process for the integrated smart card payment system solution, let's consider potential scenarios, implementation strategies, partnerships, resources, obstacle management, and unexpected outcomes:\n\n1. Potential Scenarios:\n\na) Phased rollout:\n- Scenario: Implement the system in stages, starting with a single transit mode (e.g., buses) and gradually expanding to others.\n- Strategy: Begin with a pilot program in a specific area or route to test the system and gather feedback before full implementation.\n\nb) Multi-city collaboration:\n- Scenario: Partner with neighboring cities to create a regional smart card system.\n- Strategy: Form a consortium of transit authorities to share costs, expertise, and resources for a more comprehensive and widely-adopted system.\n\nc) Public-private partnership:\n- Scenario: Collaborate with a technology company to develop and maintain the system.\n- Strategy: Leverage private sector expertise while maintaining public oversight and control over fare policies.\n\n2. Implementation Strategies:\n\na) Stakeholder engagement:\n- Conduct extensive user research and involve various stakeholder groups in the design process.\n- Create a dedicated project team with representatives from different transit agencies and departments.\n\nb) Technology selection:\n- Evaluate multiple technology providers and select a solution that offers flexibility and scalability.\n- Ensure the chosen technology can integrate with existing systems and accommodate future innovations.\n\nc) Gradual transition:\n- Maintain existing payment methods alongside the new system during a transition period.\n- Offer incentives (e.g., discounts, loyalty points) for early adopters of the smart card system.\n\n3. Necessary Partnerships and Resources:\n\na) Partnerships:\n- Technology providers for hardware and software solutions\n- Financial institutions for payment processing\n- Local businesses for potential cross-promotions and expanded use of the smart card\n- Community organizations to assist with outreach and education\n\nb) Resources:\n- Funding: Secure a combination of government grants, transit authority budgets, and possibly private investment\n- Technical expertise: Hire or contract specialists in smart card technology, data security, and system integration\n- Training resources: Develop comprehensive training programs for staff and educational materials for the public\n\n4. Overcoming Potential Obstacles:\n\na) Technical issues:\n- Implement rigorous testing protocols and have a dedicated technical support team\n- Develop contingency plans for system outages, including backup offline verification methods\n\nb) User adoption:\n- Launch a comprehensive marketing and education campaign\n- Provide assistance kiosks and staff at major transit hubs to help users transition\n- Offer a grace period for accidental underpayment or system misuse\n\nc) Privacy concerns:\n- Implement strong data protection measures and clearly communicate privacy policies\n- Offer anonymous card options for users who prefer not to link their personal information\n\nd) Equity issues:\n- Ensure accessibility for unbanked individuals by offering cash loading options at various locations\n- Provide subsidized or free cards for low-income users\n\n5. Potential Unexpected Outcomes and Management:\n\na) Unexpectedly high adoption rate:\n- Outcome: System overload or card shortages\n- Management: Have scalable infrastructure and a flexible supply chain for cards\n\nb) Unforeseen security vulnerability:\n- Outcome: Data breach or fraudulent card use\n- Management: Establish a rapid response team and protocol for security incidents; implement regular security audits\n\nc) Integration challenges with certain transit modes:\n- Outcome: Delays in full system implementation\n- Management: Develop mode-specific solutions and be prepared to extend timelines if necessary\n\nd) Shift in travel patterns:\n- Outcome: Unexpected changes in transit usage due to the new system\n- Management: Utilize data analytics to quickly identify trends and adjust service accordingly\n\ne) Expanded use beyond transit:\n- Outcome: Increased demand for card use in other city services or local businesses\n- Management: Develop partnerships and protocols for expanded use while ensuring the primary transit function remains efficient\n\nBy considering these scenarios, strategies, and potential outcomes, the transit authority can create a more robust and adaptable implementation plan for the integrated smart card payment system. This deeper analysis helps in anticipating challenges, leveraging opportunities, and ultimately increasing the likelihood of successful deployment and adoption of the new system.\n"]}}
{'deepen_thought': {'deep_thoughts': ["Let's explore the implementation of a Bus Rapid Transit (BRT) system in more depth, considering various scenarios, strategies, partnerships, resources, and potential obstacles:\n\n1. Scenario Planning:\na) Best-case scenario: The BRT system is implemented smoothly, gaining widespread public support. It significantly reduces travel times, increases ridership, and sparks transit-oriented development along its corridors.\nb) Moderate scenario: The BRT system faces initial resistance but gradually gains acceptance. It improves transit times and reliability but takes longer than expected to reach ridership goals.\nc) Worst-case scenario: The BRT system faces strong opposition, experiences significant delays in implementation, and struggles to attract riders away from private vehicles.\n\n2. Implementation Strategies:\na) Phased approach: Start with a pilot corridor to demonstrate benefits and iron out issues before expanding to a larger network.\nb) Comprehensive redesign: Implement the BRT system as part of a larger urban mobility plan, including bike lanes, pedestrian improvements, and transit-oriented development policies.\nc) Public-private partnership: Collaborate with private sector partners for funding, operation, and maintenance to reduce the burden on public resources.\n\n3. Necessary Partnerships and Resources:\na) Government agencies: Transportation department, urban planning department, environmental agency, and public works department.\nb) Private sector: Bus manufacturers, ITS providers, construction companies.\nc) NGOs and community organizations: For public outreach and education.\nd) Academic institutions: For research support and impact studies.\ne) International organizations: For technical assistance and best practices (e.g., ITDP, World Bank).\n\n4. Overcoming Obstacles:\na) Public resistance: Implement a comprehensive public engagement campaign, showcasing benefits through virtual reality simulations and study tours to successful BRT cities.\nb) Funding challenges: Explore innovative financing mechanisms like value capture, carbon credits, and public-private partnerships.\nc) Technical issues: Establish a dedicated project management office with experienced professionals and international advisors.\nd) Political opposition: Build a coalition of supporters including businesses, environmental groups, and community organizations to advocate for the project.\n\n5. Unexpected Outcomes and Mitigation:\na) Gentrification along BRT corridors: Implement inclusive zoning policies and affordable housing requirements in transit-oriented developments.\nb) Reduced ridership due to autonomous vehicles: Integrate BRT with emerging mobility technologies, possibly using autonomous buses or creating dedicated lanes for shared autonomous vehicles.\nc) Cybersecurity threats to ITS: Develop robust security protocols and redundancy systems to protect against potential attacks or system failures.\nd) Climate change impacts: Design infrastructure to be resilient to extreme weather events and rising temperatures.\n\n6. Long-term Sustainability:\na) Establish a dedicated funding stream for ongoing maintenance and upgrades, possibly through a percentage of fare revenues or special assessment districts.\nb) Create a continuous improvement program to adapt the system to changing urban needs and technological advancements.\nc) Develop a skills training program to ensure a steady supply of qualified personnel for system operation and maintenance.\nd) Implement a comprehensive data collection and analysis system to monitor performance and inform future improvements.\n\nBy considering these deeper aspects of BRT implementation, cities can better prepare for challenges, capitalize on opportunities, and create a more resilient and adaptive urban transportation system. This approach increases the likelihood of long-term success and maximizes the positive impact on urban mobility and quality of life."]}}
{'deepen_thought': {'deep_thoughts': ["Let's delve deeper into the bike-sharing program solution, considering potential scenarios, implementation strategies, partnerships, resources, obstacles, and unexpected outcomes:\n\n1. Potential Scenarios:\na) Rapid Adoption: The program sees quick uptake, with residents and tourists embracing the new transportation option. This leads to a need for rapid expansion and additional funding.\nb) Slow Start: Initial adoption is slower than expected, requiring increased marketing efforts and potential adjustments to pricing or accessibility.\nc) Seasonal Fluctuations: Usage spikes during good weather and plummets during harsh conditions, necessitating adaptive strategies for off-peak seasons.\nd) Tech Integration Success: The program's app becomes a model for smart city initiatives, integrating with other transportation options for seamless mobility.\n\n2. Implementation Strategies:\na) Phased Rollout: Start with a pilot program in high-traffic areas, then expand based on data and feedback.\nb) Public-Private Partnership: Collaborate with a experienced bike-share operator to leverage their expertise and technology.\nc) Community Engagement: Involve local communities in planning station locations and gather continuous feedback for improvements.\nd) Integration with Public Transit: Ensure seamless integration with existing public transportation for last-mile connectivity.\ne) Adaptive Pricing: Implement dynamic pricing to encourage balanced bike distribution and off-peak usage.\n\n3. Necessary Partnerships and Resources:\na) Local Government: For permits, funding, and integration with city planning.\nb) Bike Manufacturers: To supply and maintain a fleet of durable, user-friendly bikes.\nc) Tech Companies: For developing and maintaining the app, payment systems, and IoT integration.\nd) Urban Planners and Traffic Engineers: To design safe bike lanes and optimal station locations.\ne) Local Businesses: For sponsorships and potential station hosts.\nf) Health Insurance Companies: Potential partners for promoting health benefits and offering user incentives.\n\n4. Overcoming Potential Obstacles:\na) Vandalism/Theft: Implement GPS tracking, require user registration, and design theft-resistant bikes and docking stations.\nb) Safety Concerns: Provide free safety training, distribute helmets, and invest in protected bike lanes.\nc) Uneven Distribution: Use incentives (e.g., credits for returning bikes to less popular stations) and employ staff to redistribute bikes.\nd) Weather Issues: Design weather-resistant bikes, provide covered stations, and offer seasonal promotions.\ne) Resistance from Car Users: Launch awareness campaigns about benefits, and gradually implement car-free zones to showcase improvements.\n\n5. Potential Unexpected Outcomes and Handling:\na) Oversaturation: If the program is too successful, it might lead to bike clutter. Solution: Implement strict parking regulations and dynamic caps on bike numbers.\nb) Impact on Local Bike Shops: Program might affect local bike rental businesses. Solution: Partner with these shops for maintenance or offer them a role in the program.\nc) Data Privacy Concerns: User data collection might raise privacy issues. Solution: Implement robust data protection measures and transparent policies.\nd) Micro-mobility Conflicts: Increasing popularity of e-scooters might compete with the program. Solution: Integrate multiple micro-mobility options within the same system.\ne) Gentrification Concerns: Improved bike infrastructure might contribute to gentrification. Solution: Ensure equitable distribution of stations and affordable pricing options.\n\n6. Long-term Sustainability Strategies:\na) Diversification: Expand to include e-bikes or integrate with other shared mobility services.\nb) Corporate Partnerships: Develop B2B offerings for employee wellness programs.\nc) Tourism Integration: Create special passes or tours for tourists, partnering with local attractions.\nd) Data Monetization: Analyze and sell anonymized travel pattern data to urban planners and researchers.\ne) Continuous Innovation: Regularly update bike designs and app features based on user feedback and technological advancements.\n\nBy considering these deeper aspects, the bike-sharing program can be better prepared for various scenarios, increasing its chances of long-term success and positive impact on urban mobility."]}}
{'rank_solutions': {'ranked_solutions': '[\n {\n "rank": 1,\n "solution": "Bus Rapid Transit (BRT) system",\n "justification": "The BRT system offers the most comprehensive and transformative solution to the city\'s transportation challenges. It has the potential to significantly reduce travel times, increase ridership, and spark transit-oriented development. The detailed scenario planning, implementation strategies, and consideration of long-term sustainability make it a robust solution. Its ability to integrate with other transportation modes and adapt to future technologies also gives it an edge."\n },\n {\n "rank": 2,\n "solution": "Integrated smart card payment system",\n "justification": "This solution addresses the efficiency and convenience aspects of public transportation use. It has the potential to streamline operations across various transit modes, improve user experience, and provide valuable data for future planning. The phased implementation approach and consideration of potential obstacles make it a practical and achievable solution. However, it ranks below the BRT system as it doesn\'t directly address capacity and speed issues."\n },\n {\n "rank": 3,\n "solution": "Bike-sharing program",\n "justification": "While the bike-sharing program offers an eco-friendly and health-promoting alternative, it may have limited impact on large-scale commuting patterns compared to the other solutions. It\'s a valuable complement to other transit options but may not be suitable for all users or weather conditions. The detailed implementation strategies and partnership considerations make it a well-thought-out solution, but its overall impact on urban congestion may be less significant than the higher-ranked options."\n }\n]'}}
AIが要約した実行結果
入力(input):"成長中の都市における公共交通機関の改善"
観点 :"コスト、効率性、環境への影響、ユーザー体験"
1. 提案された解決策:
a) バス・ラピッド・トランジット(BRT)システムの導入
b) 包括的な自転車シェアリングプログラムの開発
c) 統合スマートカード決済システムの導入
2. 各解決策の評価:
a) バス・ラピッド・トランジット(BRT)システム
* 成功確率: 75%
* 信頼度: 80%
* 主なメリット: コスト効率、迅速な実装、高い乗客容量、柔軟性
* 主な課題: 道路空間の再配分、初期の抵抗、鉄道システムと比べた魅力度
b) 自転車シェアリングプログラム
* 成功確率: 70%
* 信頼度: 75%
* 主なメリット: 環境に優しい、混雑緩和、健康促進
* 主な課題: 高い初期投資、メンテナンスコスト、天候依存
c) 統合スマートカード決済システム
* 成功確率: 75%
* 信頼度: 80%
* 主なメリット: 利便性向上、取引の迅速化、データ収集
* 主な課題: 高い初期投資、技術的複雑さ、プライバシー懸念
3. 解決策のランキング:
1位: バス・ラピッド・トランジット(BRT)システム
2位: 統合スマートカード決済システム
3位: 自転車シェアリングプログラム
ランキングの根拠: BRTシステムが最も包括的で変革的な解決策とされ、交通時間の短縮、乗客数の増加、交通指向型開発の促進などの可能性が高い
グラフイメージの出力
from IPython.display import Image
Image(app.get_graph().draw_mermaid_png())
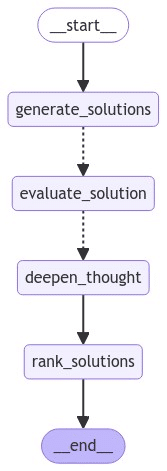
この記事が参加している募集
この記事が気に入ったらサポートをしてみませんか?