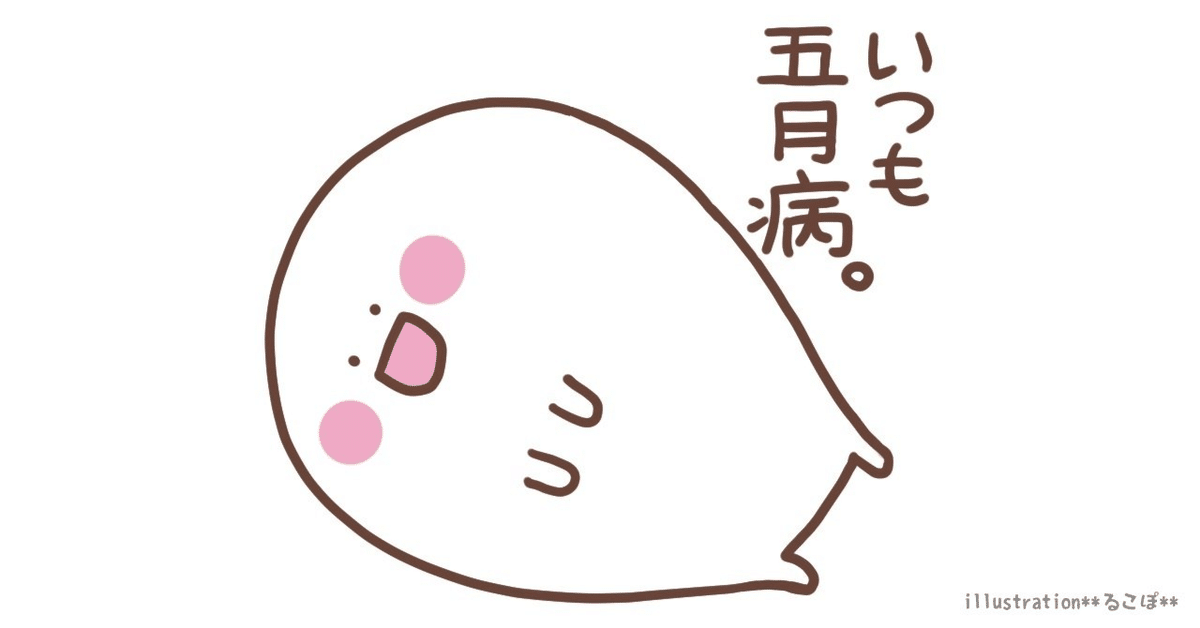
Photo by
lcopo
143 BeautifulSoupのチートシート #自分のための備忘録
BeautifulSoupのチートシートを作成しました。これを参考にして、さまざまスクレイピングタスクを試してみてください!
1. 基本操作
インポートとセットアップ
from bs4 import BeautifulSoup
import requests
# ウェブページを取得
url = 'https://www.example.com'
response = requests.get(url)
# BeautifulSoupオブジェクトを作成
soup = BeautifulSoup(response.content, 'html.parser')
2. HTML解析
HTMLの整形
print(soup.prettify())
HTMLのタイトルを取得
soup.title.string
print(title)
3. 要素の検索
タグ名で検索
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
クラス名で検索
links = soup.find_all('a', class_='myClass')
for link in links:
print(link.get('href'))
IDで検索
element = soup.find(id='myId')
print(element.text)
CSSセレクタで検索
items = soup.select('.myClass #myId')
for item in items:
print(item.text)
4. 要素の属性の操作
属性の取得
link = soup.find('a')
href = link['href']
print(href)
属性の設定
link['href'] = 'https://www.newurl.com'
5. ナビゲーション
親要素の取得
parent = element.parent
print(parent)
子要素の取得
children = element.children
for child in children:
print(child)
兄弟要素の取得
next_sibling = element.next_sibling
previous_sibling = element.previous_sibling
print(next_sibling, previous_sibling)
6. 出力と保存
テキストの抽出
text = soup.get_text()
print(text)
HTMLの保存
with open('output.html', 'w', encoding='utf-8') as file:
file.write(str(soup))
7. 便利な関数
要素の検索と抽出を同時に行う
for link in soup.find_all('a', href=True):
print(link['href'])
コメントの抽出
comments = soup.find_all(string=lambda text: isinstance(text, Comment))
for comment in comments:
print(comment)
条件に一致する要素の検索
def has_class_but_no_id(tag):
return tag.has_attr('class') and not tag.has_attr('id')
elements = soup.find_all(has_class_but_no_id)
for element in elements:
print(element)
参考
このチートシートを使って、BeautifulSoupでさまざまなウェブスクレイピングのタスクに挑戦してみてください!さらに知りたいことや質問があれば教えてくださいね。✨📄💻