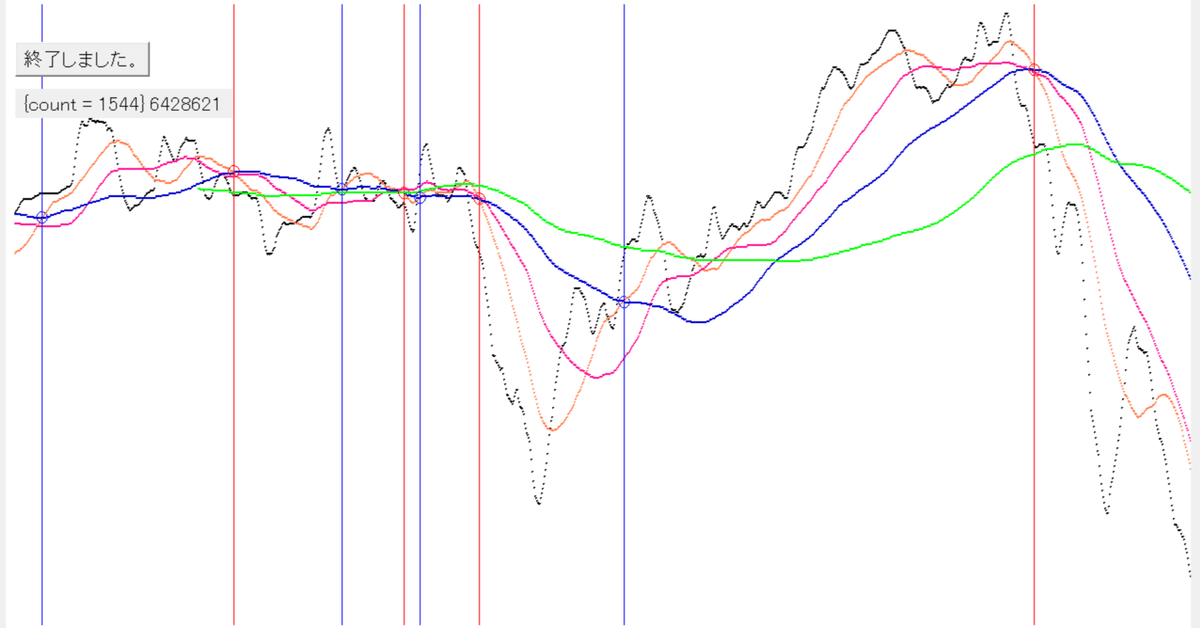
BOT開発、ゴールデンクロスとデッドクロスを検出する。暗号資産・販売所ザイフ API python
ZaifAPIを使用したBOT開発のサンプル・プログラムです。
from zaifapi import ZaifPublicApi
zaif_public = ZaifPublicApi()
import time
import threading
import tkinter
import math
import requests
import json
loop = 1260
hei = 680
root = tkinter.Tk()
root.geometry("1280x715") #note = 1210x710" desk = 1600x830
ca = tkinter.Canvas(width=loop, height=hei, bg="white") #note = height=680 desk = height=800
ca.pack()
me = tkinter.Message(root, text="銭の生る樹", font=("Times New ZRoman", 16), width=500)
me.place(x=20, y=100)
flag = "off"
histry1s = []
histry10s = []
histry1m = []
histry2m = []
histry4m = []
histry8m = []
stance = []
market = "bitflyer" # "zaif" "bitflyer"
entry = "ok"
count = -1
trend_new = 0
trend_old = 0
def func1():
global flag
global ca
global count
global loop
global trend_new
global trend_old
global histry1s
global histry10s
global histry1m
global histry2m
global histry4m
global histry8m
global stance
while True:
time.sleep(0.001)
count = count + 1
if market == "bitflyer":
# bitflyer
#res = requests.get('https://api.zaif.jp/api/1/last_price/btc_jpy')
res = requests.get('https://api.bitflyer.jp/v1/getboard?product_code=BTC_JPY')
if res.status_code != 200:
raise Exception('return status code is {}'.format(res.status_code))
price = json.loads(res.text)
graph_y = int(price["mid_price"])
else:
# zaif
res = zaif_public.last_price('btc_jpy') #
graph_y = int(res["last_price"]) #
me["text"] = "count = {}".format(count),str(graph_y)
index = len(histry1s)
if index < loop:
histry1s.append(graph_y)
else:
for i in range(1,loop):
histry1s[i-1] = histry1s[i]
histry1s[loop - 1] = graph_y
if count >= 10:
total = 0
for s in range(len(histry1s) - 10,len(histry1s)):
total = total + histry1s[s]
heikin10s = total // 10
if len(histry10s) < loop:
histry10s.append(heikin10s)
else:
for i in range(1,loop):
histry10s[i-1] = histry10s[i]
histry10s[loop - 1] = heikin10s
if count >= 60:
total = 0
for s in range(len(histry1s) - 60,len(histry1s)):
total = total + histry1s[s]
heikin1m = total // 60
if len(histry1m) < loop:
histry1m.append(heikin1m)
else:
for i in range(1,loop):
histry1m[i-1] = histry1m[i]
histry1m[loop - 1] = heikin1m
if count >= 120:
total = 0
for s in range(len(histry1s) - 120,len(histry1s)):
total = total + histry1s[s]
heikin2m = total // 120
if len(histry2m) < loop:
histry2m.append(heikin2m)
else:
for i in range(1,loop):
histry2m[i-1] = histry2m[i]
histry2m[loop - 1] = heikin2m
if count >= 240:
total = 0
for s in range(len(histry1s) - 240,len(histry1s)):
total = total + histry1s[s]
heikin4m = total // 240
if len(histry4m) < loop:
histry4m.append(heikin4m)
else:
for i in range(1,loop):
histry4m[i-1] = histry4m[i]
histry4m[loop - 1] = heikin4m
if count >= 480:
total = 0
for s in range(len(histry1s) - 480,len(histry1s)):
total = total + histry1s[s]
heikin8m = total // 480
if len(histry8m) < loop:
histry8m.append(heikin8m)
else:
for i in range(1,loop):
histry8m[i-1] = histry8m[i]
histry8m[loop - 1] = heikin8m
if count >= 240:
signal = "non"
if histry1m[len(histry1m) - 2] < histry4m[len(histry4m) - 2] and histry1m[len(histry1m) - 1] > histry4m[len(histry4m) - 1]:
signal = "golden"
print("old 1m({}) > 4m({})".format(histry1m[len(histry1m) - 2],histry4m[len(histry4m) - 2]))
print("golden 1m({}) < 4m({})".format(histry1m[len(histry1m) - 1],histry4m[len(histry4m) - 1]))
if histry1m[len(histry1m) - 2] > histry4m[len(histry4m) - 2] and histry1m[len(histry1m) - 1] < histry4m[len(histry4m) - 1]:
signal = "ded"
print("old 1m({}) < 4m({})".format(histry1m[len(histry1m) - 2],histry4m[len(histry4m) - 2]))
print("ded 1m({}) > 4m({})".format(histry1m[len(histry1m) - 1],histry4m[len(histry4m) - 1]))
index = len(stance)
if index < loop:
stance.append(0)
else:
for i in range(1,len(stance)):
stance[i-1] = stance[i]
if signal == "non":
stance[len(stance) - 1] = 0
if signal == "ded":
stance[len(stance) - 1] = -1
print("stance[len(stance) - 1] = {}".format(stance[len(stance) - 1]))
if signal == "golden":
stance[len(stance) - 1] = 1
print("stance[len(stance) - 1] = {}".format(stance[len(stance) - 1]))
if count < loop + 10:
min_price = int(min(histry1s) / 100)
max_price = int(max(histry1s) / 100)
if count > loop + 10:
min_price = int(min(min(histry1s, histry10s, key=min)) / 100)
max_price = int(max(max(histry1s, histry10s, key=max)) / 100)
if count > loop + 60:
min_price = int(min(min(histry1s, histry10s, histry1m, key=min)) / 100)
max_price = int(max(max(histry1s, histry10s, histry1m, key=max)) / 100)
if count > loop + 120:
min_price = int(min(min(histry1s, histry10s, histry1m, histry2m, key=min)) / 100)
max_price = int(max(max(histry1s, histry10s, histry1m, histry2m, key=max)) / 100)
if count > loop + 240:
min_price = int(min(min(histry1s, histry10s, histry1m, histry2m, histry4m, key=min)) / 100)
max_price = int(max(max(histry1s, histry10s, histry1m, histry2m, histry4m, key=max)) / 100)
if count > loop + 480:
min_price = int(min(min(histry1s, histry10s, histry1m, histry2m, histry4m, histry8m, key=min)) / 100)
max_price = int(max(max(histry1s, histry10s, histry1m, histry2m, histry4m, histry8m, key=max)) / 100)
min_price = min_price - 1
max_price = max_price + 1
hi_range = max_price - min_price
bai = hei / hi_range
ca.delete("all")
barA_x = 10
j1s = -1
j10s = -1
j1m = -1
j2m = -1
j4m = -1
j8m = -1
for i in range(loop):
if len(histry10s) > 0:
if i > loop - len(histry10s):
j10s = j10s + 1
hi_10s = max_price - int(histry10s[j10s] /100)
barO_y = hi_10s * bai
ca.create_line(barA_x, barO_y, barA_x + 1, barO_y + 1, fill="black",width=2)
if len(histry1m) > 0:
if i > loop - len(histry1m):
j1m = j1m + 1
hi_1m = max_price - int(histry1m[j1m] /100)
barO_y = hi_1m * bai
ca.create_line(barA_x, barO_y, barA_x + 1, barO_y + 1, fill="coral",width=2)
if len(histry2m) > 0:
if i > loop - len(histry2m):
j2m = j2m + 1
hi_2m = max_price - int(histry2m[j2m] /100)
barM_y = hi_2m * bai
ca.create_line(barA_x, barM_y, barA_x + 1, barM_y + 1, fill="deeppink",width=2)
if len(histry4m) > 0:
if i > loop - len(histry4m):
j4m = j4m + 1
hi_4m = max_price - int(histry4m[j4m] /100)
barB_y = hi_4m * bai
ca.create_line(barA_x, barB_y, barA_x + 1, barB_y + 1, fill="mediumblue",width=2)
if stance[j4m] == -1 and j4m != 0:
ca.create_line(barA_x, 10, barA_x, hei - 10, fill="red", width=1)
ca.create_oval(barA_x - 6, barB_y - 6, barA_x + 6, barB_y + 6, width=1,outline="red")
if stance[j4m] == 1 and j4m != 0:
ca.create_line(barA_x, 10, barA_x, hei - 10, fill="blue",width=1)
ca.create_oval(barA_x - 6, barB_y - 6, barA_x + 6, barB_y + 6, width=1,outline="blue")
if len(histry8m) > 0:
if i > loop - len(histry8m):
j8m = j8m + 1
hi_8m = max_price - int(histry8m[j8m] /100)
barG_y = hi_8m * bai
ca.create_line(barA_x, barG_y, barA_x + 1, barG_y + 1, fill="lime",width=2)
if i > loop - len(histry1s):
j1s = j1s + 1
hi_1s = max_price - int(histry1s[j1s] /100)
barS_y = hi_1s * bai
#ca.create_line(barA_x, barS_y, barA_x + 1, barS_y + 1, fill="black",width=2)
#if stance[j1s] == -1 and j1s != 0:
#ca.create_oval(barA_x - 4, barS_y - 4, barA_x + 4, barS_y + 4, fill="red", width=2,outline="red")
#if stance[j1s] == 1 and j1s != 0:
#ca.create_oval(barA_x - 4, barS_y - 4, barA_x + 4, barS_y + 4, fill="blue",width=2,outline="blue")
barA_x = barA_x + 1
if flag == "on":
flag = "off"
break
print("loop end")
def btn_on2():
global flag
global bu2
bu2["text"] = "終了しました。"
flag = "on"
bu2 = tkinter.Button(root, text="ループ終了", font=("Times New ZRoman", 16), command=btn_on2)
bu2.place(x=20, y=50)
thread_1 = threading.Thread(target=func1)
thread_1.start()
root.mainloop()