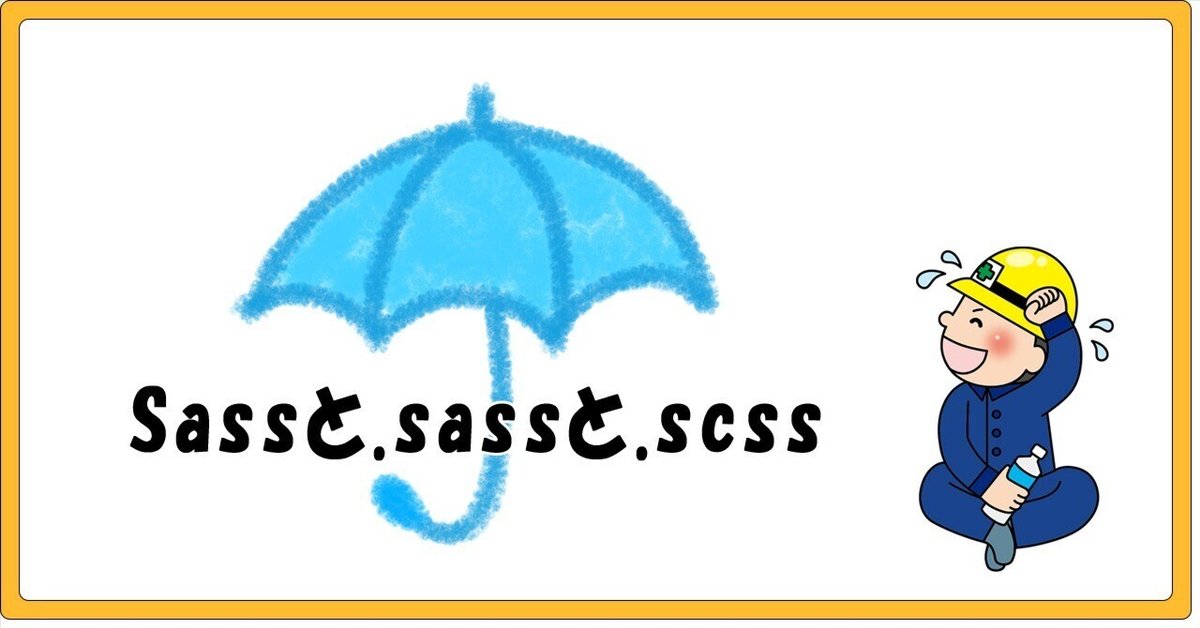
Sassと.sassと.scss
Sass
CSSを便利に簡単に効率よく記述するためのメタ言語。
.sassと.scss
Sassの記法により拡張子が変わる。
.sass : {}や;を使わず、インデントで階層を表現する 記法。
$primary-color: #3498db
.button
background-color: $primary-color
color: white
padding: 10px 20px
border-radius: 5px
.scss :CSSとほぼ同じ書き方なので、CSSからの移行がしやすい。
Sassといえば.scssを指すのが一般的。
$primary-color: #3498db;
.button {
background-color: $primary-color;
color: white;
padding: 10px 20px;
border-radius: 5px;
}
使い方(.scss)
ネスト
&(アンパサンド)を使った親セレクタの参照
.button {
background-color: #3498db;
color: white;
padding: 10px 20px;
border-radius: 5px;
&:hover {
background-color: #2980b9; // ホバー時に色を変更
}
&.active {
background-color: #2ecc71; // アクティブ時のスタイル
}
}
cssに変換(コンパイル)すると
.button {
background-color: #3498db;
color: white;
padding: 10px 20px;
border-radius: 5px;
}
.button:hover {
background-color: #2980b9;
}
.button.active {
background-color: #2ecc71;
}
メディアクエリのネスト
.container {
width: 80%;
margin: 0 auto;
@media (max-width: 768px) {
width: 100%;
}
}
cssに変換(コンパイル)すると
.container {
width: 80%;
margin: 0 auto;
}
@media (max-width: 768px) {
.container {
width: 100%;
}
}
変数
先頭に $ を付けて定義し、: の後ろに値。
// 変数の定義
$primary-color: #3498db;
$secondary-color: #2ecc71;
$font-size-large: 20px;
// 変数の使用
.button {
background-color: $primary-color;
color: white;
font-size: $font-size-large;
padding: 10px 20px;
border-radius: 5px;
&:hover {
background-color: $secondary-color;
}
}
cssに変換(コンパイル)すると
.button {
background-color: #3498db;
color: white;
font-size: 20px;
padding: 10px 20px;
border-radius: 5px;
}
.button:hover {
background-color: #2ecc71;
}
calc()なしで演算できる
$base-size: 16px;
.container {
font-size: $base-size;
padding: $base-size * 2; // 32px
margin: $base-size / 2; // 8px
}
カラーのバリエーションを作る
darken lightenは組み込み関数。
$primary-color: #3498db;
.button {
background-color: $primary-color;
color: white;
&:hover {
background-color: darken($primary-color, 10%); // 少し暗くする(#217dbb)
}
&:active {
background-color: lighten($primary-color, 10%); // 少し明るくする(#5dade2)
}
}
グローバル変数
スコープ外で変数を使用したい場合は !global
$global-var: red !global;
パーシャル
_(アンダースコア) をつけて作成する部分的なスタイルファイル
SCSSファイルを分割して管理しやすく なる
/scss
├── _variables.scss // 変数の定義
├── _mixins.scss // Mixinの定義
├── _header.scss // ヘッダーのスタイル
├── _footer.scss // フッターのスタイル
├── style.scss // メインSCSS(ここで全てを読み込む)
//_variables.scss(変数の定義)
$primary-color: #3498db;
$font-size-large: 18px;
//_header.scss(ヘッダーのスタイル)
.header {
background-color: $primary-color;
font-size: $font-size-large;
padding: 10px;
}
//_footer.scss(フッターのスタイル)
.footer {
background-color: darken($primary-color, 10%);
color: white;
padding: 15px;
}
style.scss(メインのSCSSファイルでパーシャルを読み込む)
@use "variables";
@use "header";
@use "footer";
.header {
background-color: variables.$primary-color; // `@use` では変数名の前にファイル名が必要
}
コンパイル後
.header {
background-color: #3498db;
}
.header {
color: white;
font-size: 18px;
}
.footer {
background-color: #217dbb; /* #3498db を 10% 暗く */
color: white;
padding: 15px;
}
Mixin
CSSのスタイルを再利用できる機能
関数のように使え、引数を渡して動的にスタイルを変更できる
1.Mixinの使い方
Mixin の定義
@mixin white-button {
display: inline-block;
padding: 10px 20px;
background-color: #3498db;
color: white;
border-radius: 5px;
text-align: center;
text-decoration: none;
}
Mixin の適用
.button {
@include white-button;
}
コンパイル後
.button {
display: inline-block;
padding: 10px 20px;
background-color: #3498db;
color: white;
border-radius: 5px;
text-align: center;
text-decoration: none;
}
2.引数を使って動的に変更する
@mixin white-button($bg-color, $text-color) {
display: inline-block;
padding: 10px 20px;
background-color: $bg-color;
color: $text-color;
border-radius: 5px;
text-align: center;
text-decoration: none;
}
$bg-color, $text-colorに任意の値を割り当てる
.button-primary {
@include white-button(#3498db, white);
}
.button-danger {
@include white-button(#e74c3c, white);
}
コンパイル後
.button-primary {
display: inline-block;
padding: 10px 20px;
background-color: #3498db;
color: white;
border-radius: 5px;
text-align: center;
text-decoration: none;
}
.button-danger {
display: inline-block;
padding: 10px 20px;
background-color: #e74c3c;
color: white;
border-radius: 5px;
text-align: center;
text-decoration: none;
}
3.デフォルト値を設定する
@mixin white-button($bg-color: #3498db, $text-color: white)
4.メディアクエリをMixinにする
@mixin responsive($breakpoint) {
@media (max-width: $breakpoint) {
@content;
}
}
.box {
width: 600px;
@include responsive(768px) {
width: 100%;
}
}
コンパイル後
.box {
width: 600px;
}
@media (max-width: 768px) {
.box {
width: 100%;
}
}