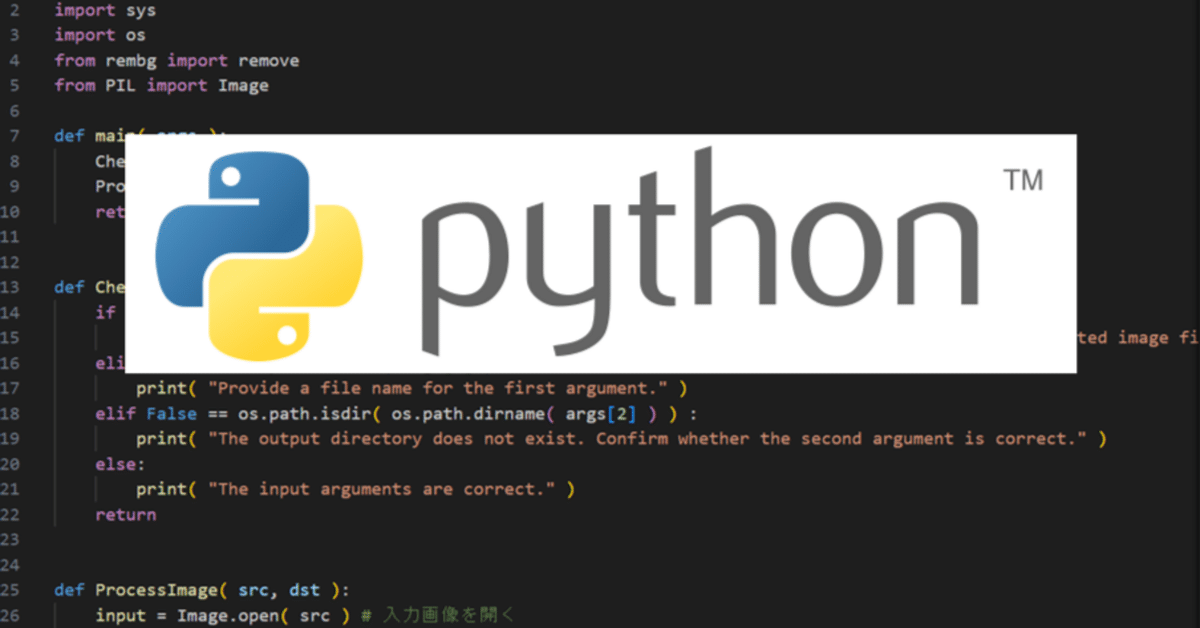
Pythonで複数PDF文書を1つにまとめる
Pythonプログラム
ファイル名は「con_pdfs.py」とした。
#!python3.12
import glob
import os
import sys
from pypdf import PdfReader, PdfWriter
#---------*---------*---------*---------*---------*---------*
# main
#
# 指定したフォルダにあるPDF文書ファイルを全部まとめて
# 1つのPDF文書(C)にする。
# PDFファイル(C)は、con_pdfs.pyのあるフォルダに作成される。
#
# 使い方
# con_pdfs.py [合体するpdfのあるフォルダ] [PDFファイル(C)のファイル名]
#---------*---------*---------*---------*---------*---------*
def main( args ):
# 引数チェック
checked = CheckArguments( args )
if checked == False :
return
# 指定したフォルダ内のPDFファイルを列挙
files = GetFilesInDirectory( args[1], "pdf" )
if len( files ) < 2 :
print( "There is no pdf file or only one pdf file in the specified directoy." )
return
# PDFファイル全部を1つのファイルにまとめる
ConcatenatePdfFiles( files, args[2] )
print( f"{len(files)}個のPDFファイルを1つのファイルにまとめました。: {args[2]}" )
return
#---------*---------*---------*---------*---------*---------*
# 入力引数チェック
#---------*---------*---------*---------*---------*---------*
def CheckArguments( args ):
if len( args ) != 3 :
print( "The number of arguments is incorrect. Input an directory path containing target pdf files and an result pdf file name." )
return False
elif False == os.path.isdir( args[1] ) :
print( f"Provide a directoy name for the first argument. The specified value is {args[1]}." )
return False
elif True == os.path.isfile( args[2] ) :
print( f"The file with specified name already exists. Confirm whether the second argument is correct. The specified value is {args[2]}." )
return False
else:
print( "The input arguments are correct." )
return True
#---------*---------*---------*---------*---------*---------*
# 指定したフォルダ内にある、指定した拡張子のファイルを列挙する
#---------*---------*---------*---------*---------*---------*
def GetFilesInDirectory( directoryPath, extension ):
searchPattern = os.path.join( directoryPath, f"*.{extension}" )
files = glob.glob( searchPattern )
return files
#---------*---------*---------*---------*---------*---------*
# 指定した任意の数のPDF文書ファイルを1つの文書ファイルにまとめる
#---------*---------*---------*---------*---------*---------*
def ConcatenatePdfFiles( pdfPaths, mergedPdfPath ):
writer = PdfWriter()
for pdfPath in pdfPaths:
# PDFリーダーオブジェクトの作成
reader = PdfReader( pdfPath )
# PDFの各ページを新しい文書ファイルに追加していく
for page in reader.pages:
writer.add_page( page )
# 結合したPDFを保存
with open( mergedPdfPath, "wb" ) as outputFile:
writer.write( outputFile )
return
#---------*---------*---------*---------*---------*
#
#---------*---------*---------*---------*---------*
if __name__ == "__main__":
args = sys.argv
main( args )
使用例
準備
PDF文書を扱うため、pypdfパッケージのインストールが必要。
> py -3.12 -m pip install pypdf
コマンド実行した時に以下のようなメッセージが出れば成功。
Collecting pypdf
Downloading pypdf-5.3.0-py3-none-any.whl.metadata (7.2 kB)
Downloading pypdf-5.3.0-py3-none-any.whl (300 kB)
Installing collected packages: pypdf
Successfully installed pypdf-5.3.0
文書合体実行
> py -3.12 con_pdfs.py .\ combined_result.pdf
現在のフォルダにあるpdfファイルを合体して、combined_result.pdfという名前のファイルを作る。
実行環境について
Python のインストールが必要。
上のプログラムは、Windows 10 上で動作確認済み。
Pythonのバージョン:3.12.9
必要なライブラリ:pypdf