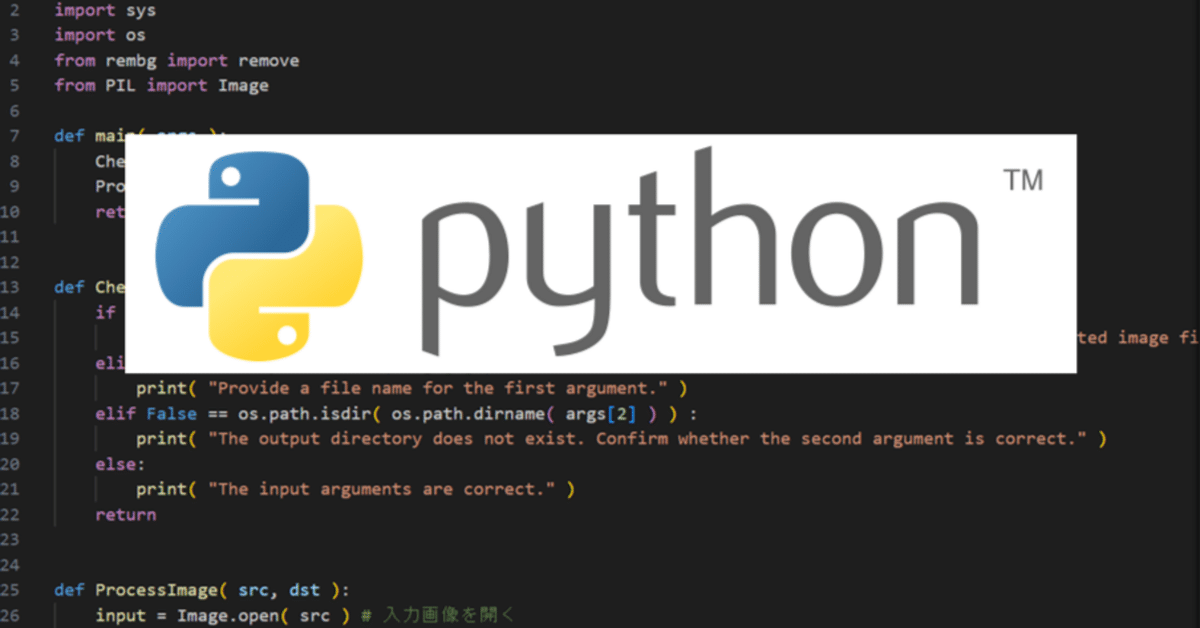
Pythonで複数CSVファイルを1つにまとめる
Pythonプログラム
ファイル名は「con_csvs.py」とした。
#!python3.12
import glob
import os
import sys
import pandas
#---------*---------*---------*---------*---------*---------*
# main
#
# 指定したフォルダにあるCSVファイルを全部まとめて
# 1つのCSVファイル(C)にする。
# CSVファイル(C)は、con_csvs.pyのあるフォルダに作成される。
#
# 使い方
# con_csvs.py [合体するCSVのあるフォルダ] [CSVファイル(C)のファイル名]
#---------*---------*---------*---------*---------*---------*
def main( args ):
# 引数チェック
checked = CheckArguments( args )
if checked == False :
return
# 指定したフォルダ内のCSVファイルを列挙
csvFiles = GetFilesInDirectory( args[1], "csv" )
if len( csvFiles ) < 2 :
print( "There is no csv file or only one csv file in the specified directoy." )
return
# CSVファイルの encoding
csvEncoding = 'shift-jis'
# CSVファイルを読み込み、リストに格納
dataFrames = []
for file in csvFiles:
# skiprows=7でファイル先頭7行を読み込まない
# skipfooter=4でファイル末尾4行を読み込まない
dataFrame = pandas.read_csv( file, encoding=csvEncoding, header = None, skiprows = 7, skipfooter = 4 )
dataFrames.append( dataFrame )
# CSVファイル全部を1つのファイルにまとめる
resultFile = pandas.concat( dataFrames, ignore_index = True )
resultFile.to_csv( args[2], encoding = csvEncoding, index = False )
print( f"{len( csvFiles )}個のCSVファイルを1つのファイルにまとめました。: {args[2]}" )
return
#---------*---------*---------*---------*---------*---------*
# 入力引数チェック
#---------*---------*---------*---------*---------*---------*
def CheckArguments( args ):
if len( args ) != 3 :
print( "The number of arguments is incorrect. Input an directory path containing target pdf files and an result pdf file name." )
return False
elif False == os.path.isdir( args[1] ) :
print( f"Provide a directoy name for the first argument. The specified value is {args[1]}." )
return False
elif True == os.path.isfile( args[2] ) :
print( f"The file with specified name already exists. Confirm whether the second argument is correct. The specified value is {args[2]}." )
return False
else:
print( "The input arguments are correct." )
return True
#---------*---------*---------*---------*---------*---------*
# 指定したフォルダ内にある、指定した拡張子のファイルを列挙する
#---------*---------*---------*---------*---------*---------*
def GetFilesInDirectory( directoryPath, extension ):
searchPattern = os.path.join( directoryPath, f"*.{extension}" )
files = glob.glob( searchPattern )
return files
#---------*---------*---------*---------*---------*
#
#---------*---------*---------*---------*---------*
if __name__ == "__main__":
args = sys.argv
main( args )
使用例
準備
pandasパッケージのインストールが必要。
> py -3.12 -m pip install pandas
コマンド実行した時に以下のようなメッセージが出れば成功。
>py -3.12 -m pip install pandas
Collecting pandas
Downloading pandas-2.2.3-cp312-cp312-win_amd64.whl.metadata (19 kB)
Collecting numpy>=1.26.0 (from pandas)
Downloading numpy-2.2.3-cp312-cp312-win_amd64.whl.metadata (60 kB)
Collecting python-dateutil>=2.8.2 (from pandas)
Downloading python_dateutil-2.9.0.post0-py2.py3-none-any.whl.metadata (8.4 kB)
Collecting pytz>=2020.1 (from pandas)
Downloading pytz-2025.1-py2.py3-none-any.whl.metadata (22 kB)
Collecting tzdata>=2022.7 (from pandas)
Downloading tzdata-2025.1-py2.py3-none-any.whl.metadata (1.4 kB)
Collecting six>=1.5 (from python-dateutil>=2.8.2->pandas)
Downloading six-1.17.0-py2.py3-none-any.whl.metadata (1.7 kB)
Downloading pandas-2.2.3-cp312-cp312-win_amd64.whl (11.5 MB)
---------------------------------------- 11.5/11.5 MB 6.5 MB/s eta 0:00:00
Downloading numpy-2.2.3-cp312-cp312-win_amd64.whl (12.6 MB)
---------------------------------------- 12.6/12.6 MB 7.2 MB/s eta 0:00:00
Downloading python_dateutil-2.9.0.post0-py2.py3-none-any.whl (229 kB)
Downloading pytz-2025.1-py2.py3-none-any.whl (507 kB)
Downloading tzdata-2025.1-py2.py3-none-any.whl (346 kB)
Downloading six-1.17.0-py2.py3-none-any.whl (11 kB)
Installing collected packages: pytz, tzdata, six, numpy, python-dateutil, pandas
Successfully installed numpy-2.2.3 pandas-2.2.3 python-dateutil-2.9.0.post0 pytz-2025.1 six-1.17.0 tzdata-2025.1
[notice] A new release of pip is available: 24.3.1 -> 25.0.1
[notice] To update, run: python.exe -m pip install --upgrade pip
ファイル合体実行
> py -3.12 con_csvs.py .\ combined_result.csv
現在のフォルダにあるpdfファイルを合体して、combined_result.csvという名前のファイルを作る。
実行環境について
Python のインストールが必要。
上のプログラムは、Windows 10 上で動作確認済み。
Pythonのバージョン:3.12.9
必要なライブラリ:pandas
その他
プログラムは以下の記事にあるコードとよく似ている。
関数CheckArgumentsとGetFilesInDirectoryは同じもの。