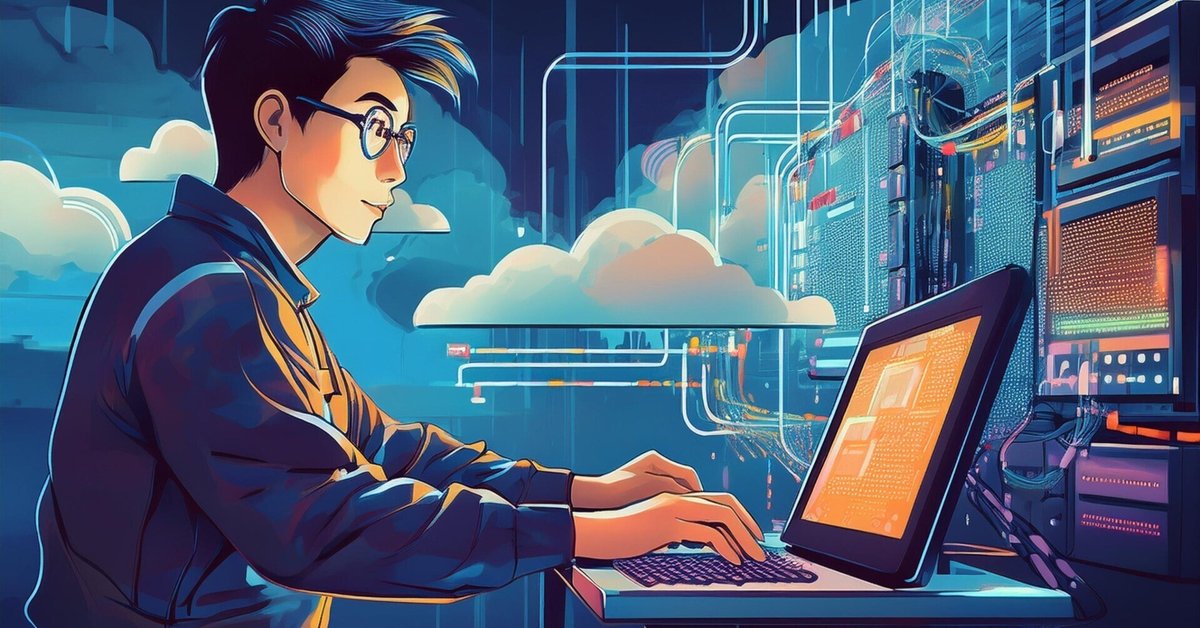
課題概要: 不動産物件管理APIの構築をする課題(架空のプロジェクト)
課題概要: 不動産物件管理APIの構築
目的
不動産物件情報を管理するAPIをGoで作成し、CRUD操作(Create, Read, Update, Delete)ができるバックエンドを構築します。ユーザーは新しい物件を登録し、物件リストを閲覧、編集、削除できるようにします。
課題ステップ
1. 環境セットアップ
Goのインストール
フレームワークとしてGinまたはFiberを使用
MySQLやPostgreSQLなどのリレーショナルデータベースを使用し、データを管理する
2. APIの設計
RESTful APIを設計し、以下のエンドポイントを実装します。
`POST /properties`: 新しい物件を登録する(Create)
`GET /properties`: すべての物件のリストを取得する(Read)
`GET /properties/{id}`: 特定の物件の詳細を取得する(Read)
`PUT /properties/{id}`: 既存の物件情報を更新する(Update)
`DELETE /properties/{id}`: 物件を削除する(Delete)
3. データベース設計
データベースに物件テーブルを作成し、以下のカラムを持たせます:
`id` (整数型, 主キー)
`name` (文字列型, 物件の名前)
`address` (文字列型, 物件の住所)
`price` (浮動小数点型, 物件の価格)
`size` (整数型, 物件の広さ)
`description` (文字列型, 物件の詳細)
4. リポジトリパターンの実装
データベースとAPIの間にリポジトリ層を設け、データベース操作を抽象化します。リポジトリ層がデータベースへのCRUD操作を実行します。
5. セキュリティ
**JWT(JSON Web Token)**を使ってAPIに認証を追加し、物件の登録、更新、削除操作には認証を要求します。
脆弱性防止対策として、SQLインジェクションや**クロスサイトスクリプティング(XSS)**を防ぐための対策を実装します。
6. エラーハンドリング
各エンドポイントでのエラーハンドリングを実装し、適切なHTTPステータスコード(400, 404, 500など)を返します。
7. テスト
Postmanを使ってAPIエンドポイントをテストし、正しく動作することを確認します。
Goのユニットテストフレームワークを使用し、各機能のテストケースを作成します。
8. デプロイ
アプリケーションをDockerでコンテナ化し、デプロイ可能な状態にします。
AWSやHerokuを使って、デプロイして本番環境で動作するAPIを構築します。
9. バージョン管理
GitHubを使ってプロジェクトをバージョン管理します。
主要な機能実装ごとに適切なコミットメッセージを書き、バージョン管理を徹底します。
課題のゴール
Goでのバックエンド開発の流れを理解し、実際にAPIを構築するスキルを身につける。
データベースとの連携や、セキュリティ対策、デプロイまでのフルサイクルを経験する。
RESTful API設計、認証システム、エラーハンドリング、バージョン管理のベストプラクティスを習得する。
この課題を通じて、Goを使ったバックエンド開発の基本から応用までを学べるはずです。進める中で不明点や新たなチャレンジが出てきたら、その都度調べたり学んだりしながら成長していくことが大切です。
Task Overview: Building a Real Estate Management API
Objective
Create a backend API in Go that manages real estate property information, supporting CRUD operations (Create, Read, Update, Delete). Users will be able to register new properties, view the property list, edit, and delete properties.
Task Steps
1. Environment Setup
Install Go
Use the Gin or Fiber framework
Use a relational database such as MySQL or PostgreSQL to manage the data
2. API Design
Design a RESTful API and implement the following endpoints:
POST /properties: Register a new property (Create)
GET /properties: Retrieve the list of all properties (Read)
GET /properties/{id}: Retrieve details of a specific property (Read)
PUT /properties/{id}: Update existing property information (Update)
DELETE /properties/{id}: Delete a property (Delete)
3. Database Design
Create a property table in the database with the following columns:
id (integer, primary key)
name (string, property name)
address (string, property address)
price (float, property price)
size (integer, property size)
description (string, property details)
4. Implement the Repository Pattern
Implement a repository layer between the API and the database to abstract database operations. The repository layer will perform the CRUD operations on the database.
5. Security
Use JWT (JSON Web Token) to add authentication to the API, requiring authentication for property registration, updates, and deletions.
Implement security measures to prevent vulnerabilities such as SQL injection and cross-site scripting (XSS).
6. Error Handling
Implement error handling in each endpoint, returning appropriate HTTP status codes (e.g., 400, 404, 500).
7. Testing
Use Postman to test the API endpoints and ensure everything works correctly.
Use Go's unit testing framework to create test cases for each function.
8. Deployment
Containerize the application using Docker and make it deployable.
Use AWS or Heroku to deploy the API in a production environment.
9. Version Control
Use GitHub to manage the project's version control.
Write appropriate commit messages for each major feature implementation and ensure version control is properly maintained.
Goal of the Task
Understand the flow of backend development in Go and gain the skills to build an API.
Experience the full cycle of database integration, security practices, and deployment.
Learn the best practices for RESTful API design, authentication systems, error handling, and version control.
This task will help you learn both the basics and advanced aspects of backend development using Go. If any questions or new challenges arise during the process, it's important to research and learn as you progress.
いいなと思ったら応援しよう!
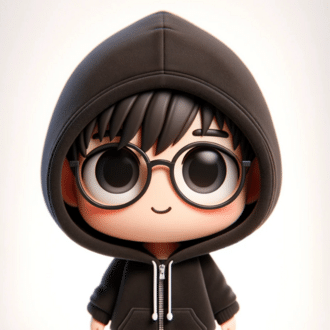