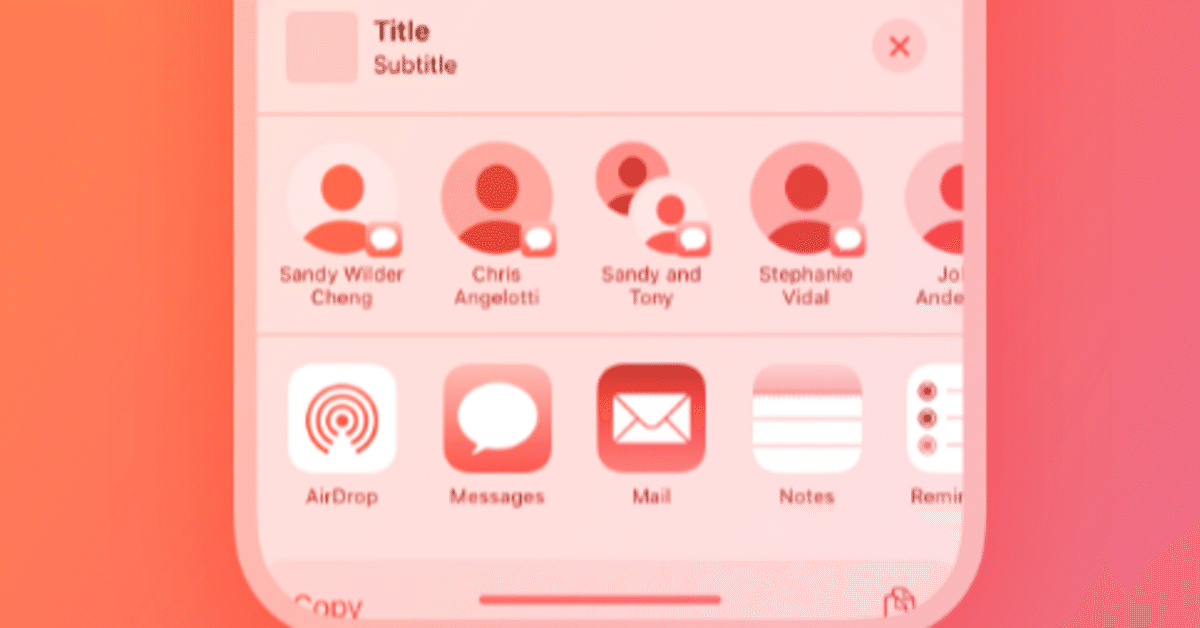
iOSアプリでディープリンク(アプリ内へのスクリーンへのリンク)を発行する
アプリの中で共有ボタンを押すと、LINEやInstagram、Airdropなど共有オプションが表示され、友達などにリンクを送信できたりしますよね
今回の記事ではそちらの実装の仕方を記載したいと思います
(iOSモバイルアプリの実装になります)
1. URLスキームを設定する
XcodeのアプリのターゲットからInfoタブに移動します
URL Typesで+ボタンを押します
URL Schemesに任意の文字を入力します。アプリ名が一番良さそうです
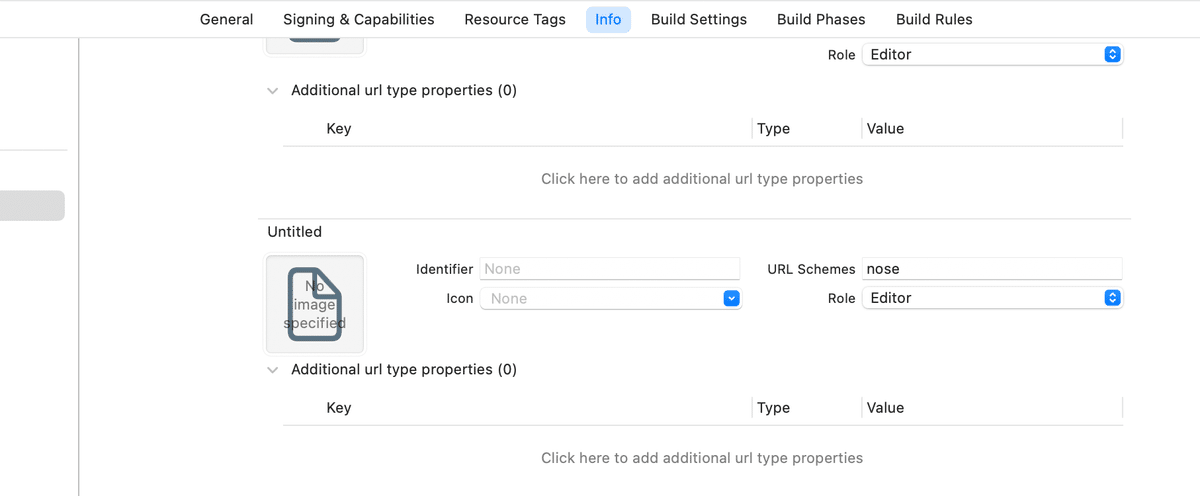
2. AppDelegate.swiftの設定
以下のようにアップデートします (参考例)
今回は自分のアプリでブックマークのリストがあるのですが、その一つに対してIDを払出す形にしています。のでブックマークの部分は必要に応じて変更してください
import UIKit
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
return true
}
// ディープリンクのハンドリング
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey: Any] = [:]) -> Bool {
guard let components = URLComponents(url: url, resolvingAgainstBaseURL: false),
let host = components.host else {
return false
}
// bookmarkの部分は書き換えてください
if host == "bookmark" {
if let queryItems = components.queryItems,
let id = queryItems.first(where: { $0.name == "id" })?.value {
navigateToBookmarkList(withId: id)
return true
}
}
return false
}
// 共有したいアイテムのIDへ移動させる
private func navigateToBookmarkList(withId id: String) {
guard let navigationController = window?.rootViewController as? UINavigationController else {
return
}
let savedBookmarksVC = SavedBookmarksViewController()
navigationController.pushViewController(savedBookmarksVC, animated: true)
// Delay to allow the view controller to load
DispatchQueue.main.asyncAfter(deadline: .now() + 0.5) {
savedBookmarksVC.showBookmarkList(withId: id)
}
}
}
3. リンク発行の対象にIDの付与
今回は以下のようにブックマークに対してIDを付与するようにしました
import Foundation
struct BookmarkList: Equatable {
let id: String
let name: String
var bookmarks: [BookmarkedPOI]
init(name: String, bookmarks: [BookmarkedPOI] = []) {
self.id = UUID().uuidString
self.name = name
this.bookmarks = bookmarks
}
static func == (lhs: BookmarkList, rhs: BookmarkList) -> Bool {
return lhs.id == rhs.id
}
}
4. リンク発行の対象となるViewControllerの設定
例として以下のように設定します
- myappの部分は1で設定したURLスキームに変更してください
private func shareBookmarkList(_ list: BookmarkList) {
let shareText = "Check out my bookmark list: \(list.name)\nmyapp://bookmark?id=\(list.id)" // myappの部分はURLスキームに合わせて変更する
let activityViewController = UIActivityViewController(activityItems: [shareText], applicationActivities: nil)
present(activityViewController, animated: true, completion: nil)
}