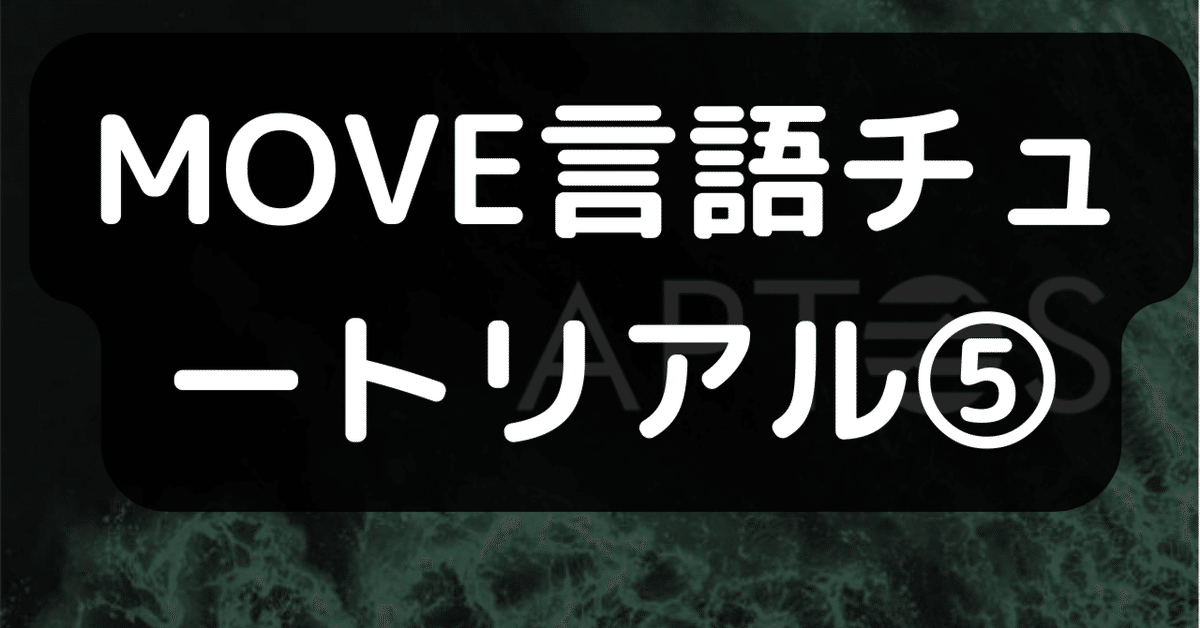
MOVE言語 チュートリアルStep_5 モジュールでのユニットテストの追加と使用
どうも!TABEです!
今回もMOVE言語のチュートリアルやっていきます。
前回の記事
今回はモジュールのユニットテスト
環境構築していない方は以下の公式URLを参考に進めてください。
https://github.com/move-language/move/tree/main/language/documentation/tutorial
では早速作業する為に
ディレクトリに移動します。
cd <moveフォルダをダウンロードしたディレクトリ>/move/language/documentation/tutorial/step_5/BasicCoin
test コマンドを入力します
move test
全てPASSすればOKみたいです。
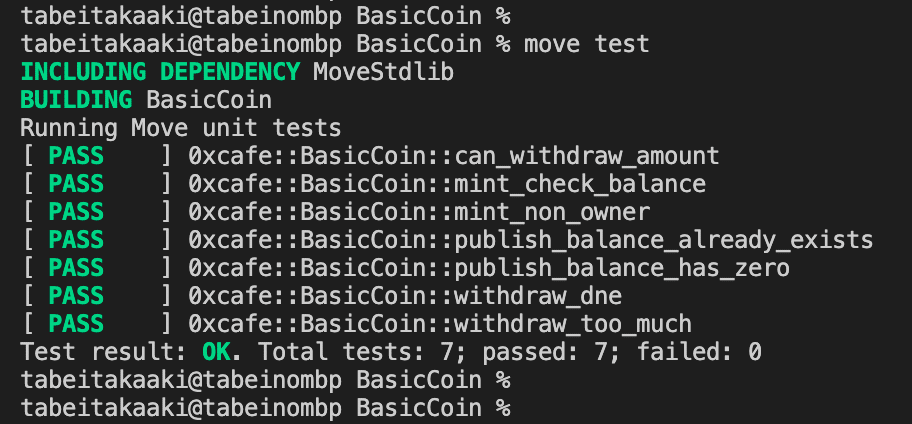
以下ユニットテストの部分に自分なりにコメントを入れました。
※前回と同じ部分はコメントしていません。
※日本語でコメントを入れるとmove testを実行した時にエラーになるため、リリースするソースのコメントは英語にしないといけないみたいです。
/// This module defines a minimal Coin and Balance.
module NamedAddr::BasicCoin {
use std::signer;
/// Address of the owner of this module
const MODULE_OWNER: address = @NamedAddr;
/// Error codes
const ENOT_MODULE_OWNER: u64 = 0;
const EINSUFFICIENT_BALANCE: u64 = 1;
const EALREADY_HAS_BALANCE: u64 = 2;
struct Coin has store {
value: u64
}
/// Struct representing the balance of each address.
struct Balance has key {
coin: Coin
}
/// Publish an empty balance resource under `account`'s address. This function must be called before
/// minting or transferring to the account.
public fun publish_balance(account: &signer) {
let empty_coin = Coin { value: 0 };
assert!(!exists<Balance>(signer::address_of(account)), EALREADY_HAS_BALANCE);
move_to(account, Balance { coin: empty_coin });
}
/// Mint `amount` tokens to `mint_addr`. Mint must be approved by the module owner.
public fun mint(module_owner: &signer, mint_addr: address, amount: u64) acquires Balance {
// Only the owner of the module can initialize this module
assert!(signer::address_of(module_owner) == MODULE_OWNER, ENOT_MODULE_OWNER);
// Deposit `amount` of tokens to `mint_addr`'s balance
deposit(mint_addr, Coin { value: amount });
}
/// Returns the balance of `owner`.
public fun balance_of(owner: address): u64 acquires Balance {
borrow_global<Balance>(owner).coin.value
}
/// Transfers `amount` of tokens from `from` to `to`.
public fun transfer(from: &signer, to: address, amount: u64) acquires Balance {
let check = withdraw(signer::address_of(from), amount);
deposit(to, check);
}
/// Withdraw `amount` number of tokens from the balance under `addr`.
fun withdraw(addr: address, amount: u64) : Coin acquires Balance {
let balance = balance_of(addr);
// balance must be greater than the withdraw amount
assert!(balance >= amount, EINSUFFICIENT_BALANCE);
let balance_ref = &mut borrow_global_mut<Balance>(addr).coin.value;
*balance_ref = balance - amount;
Coin { value: amount }
}
/// Deposit `amount` number of tokens to the balance under `addr`.
fun deposit(addr: address, check: Coin) acquires Balance{
let balance = balance_of(addr);
let balance_ref = &mut borrow_global_mut<Balance>(addr).coin.value;
let Coin { value } = check;
*balance_ref = balance + value;
}
以下ユニットテストです。
ーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーーー
#[test(account = @0x1)]
#[expected_failure]
/// このアノテーションは、そのテストがエラーを発生させることが期待されていることを意味します。
fun mint_non_owner(account: signer) acquires Balance {
/// 選択したアドレスがモジュールと一致しないことを確認する
/// オーナーアドレス
publish_balance(&account);
/// 入ってきたアドレスがモジュールのオーナーか確認
assert!(signer::address_of(&account) != MODULE_OWNER, 0);
mint(&account, @0x1, 10);
}
#[test(account = @NamedAddr)]
fun mint_check_balance(account: signer) acquires Balance {
let addr = signer::address_of(&account);
publish_balance(&account);
mint(&account, @NamedAddr, 42);
// ミントした数が42であることを確認
assert!(balance_of(addr) == 42, 0);
}
#[test(account = @0x1)]
fun publish_balance_has_zero(account: signer) acquires Balance {
let addr = signer::address_of(&account);
publish_balance(&account);
// ミントしていないので、balanceが0であることを確認
assert!(balance_of(addr) == 0, 0);
}
#[test(account = @0x1)]
// テストはabort_code = 2に等しい中止コードで中止されなければなりません。
// 構文の部分を検索して上記書いたのですが、、、意味がわからず、、また使っていく中で理解します。
#[expected_failure(abort_code = 2)]
fun publish_balance_already_exists(account: signer) {
publish_balance(&account);
publish_balance(&account);
}
#[test]
#[expected_failure]
// publish_balanceを呼び出していないからエラーになる?
fun balance_of_dne() acquires Balance {
balance_of(@0x1);
}
#[test]
#[expected_failure]
fun withdraw_dne() acquires Balance {
// コインがリソースであるため、コインを解凍する必要がある。
Coin { value: _ } = withdraw(@0x1, 0);
}
#[test(account = @0x1)]
#[expected_failure] // This test should fail
fun withdraw_too_much(account: signer) acquires Balance {
let addr = signer::address_of(&account);
publish_balance(&account);
// withdrawするCoinがなくてエラーになる?
Coin { value: _ } = withdraw(addr, 1);
}
#[test(account = @NamedAddr)]
// 1000コインを発行しても問題ないことをかくにんしている。
fun can_withdraw_amount(account: signer) acquires Balance {
publish_balance(&account);
let amount = 1000;
let addr = signer::address_of(&account);
mint(&account, addr, amount);
let Coin { value } = withdraw(addr, amount);
assert!(value == amount, 0);
}
}
今回新たに出てきた
expected_failureのアノテーションについて
この後ユニットテストをガチャガチャ動かして
どんなふうになるのか検証していこうと思います!
では引き続きコツコツやっていきましょう!
よろしくお願いします!
この記事が気に入ったらサポートをしてみませんか?