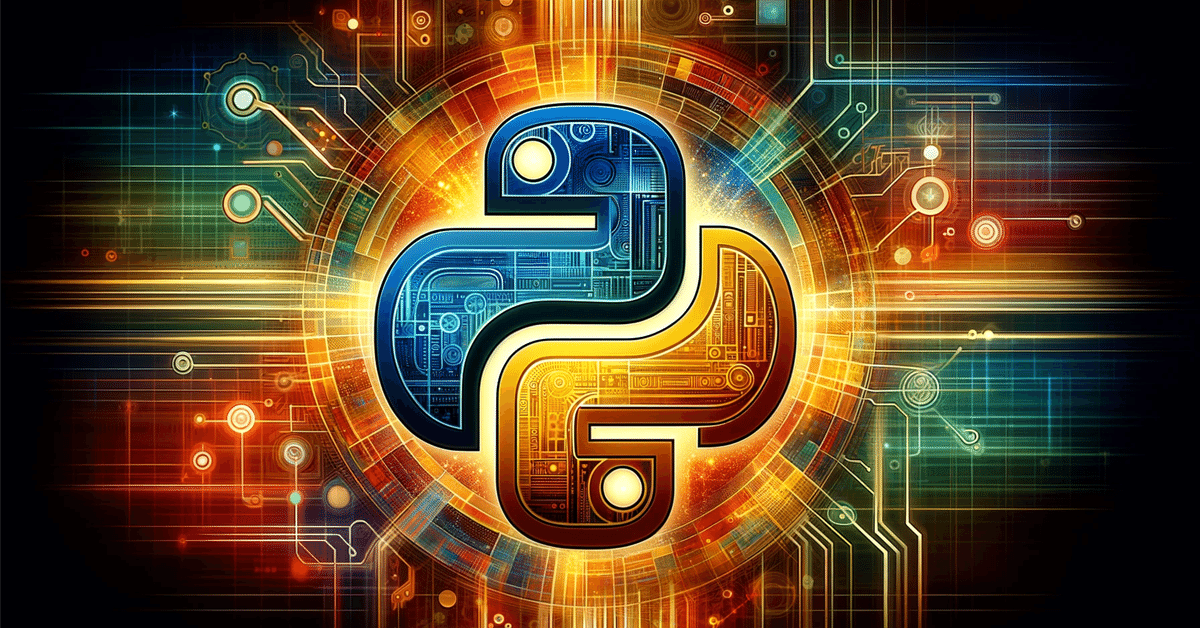
Pythonプログラム 類似画像を削除
生成AIを活用していると、重複する画像をダウンロードしたり、エラーで同じ画像が生成されたりします。
そんなときに指定したフォルダの中にある、重複する画像を削除するPythonコードを作成しました。
import os
import hashlib
from PIL import Image, UnidentifiedImageError # UnidentifiedImageErrorをインポート
def get_image_hash(img_path):
#画像ファイルのハッシュ値を計算する関数
try:
with Image.open(img_path) as img:
# 画像を一定のサイズにリサイズして、ハッシュ計算の変動を減少させる
img = img.resize((8, 8), Image.ANTIALIAS).convert('L')
pixels = list(img.getdata())
avg_pixel = sum(pixels) / len(pixels)
bits = "".join(['1' if (px > avg_pixel) else '0' for px in pixels])
hex_representation = hex(int(bits, 2))
return hex_representation
except UnidentifiedImageError:
print(f"Unable to identify image: {img_path}")
return None
def remove_duplicate_images(directory):
#指定されたディレクトリ内の重複画像を削除する関数
image_hashes = {}
deleted_count = 0
for dirpath, dirnames, filenames in os.walk(directory):
for filename in filenames:
if filename.lower().endswith(('.png', '.jpg', '.jpeg', '.gif', '.bmp')):
filepath = os.path.join(dirpath, filename)
image_hash = get_image_hash(filepath)
if image_hash in image_hashes:
print(f"Deleting duplicate image: {filepath}")
os.remove(filepath)
deleted_count += 1
else:
image_hashes[image_hash] = filepath
print(f"Total deleted images: {deleted_count}")
# 実行部分
directory = input("Enter the directory path: ")
remove_duplicate_images(directory)
print("作業が終了しました。")
importは各自で行ってください。
白黒画像も削除
以下は白黒画像を削除→重複した画像を削除するPythonコードです。
# 白黒画像削除版
import os
from PIL import Image, UnidentifiedImageError
from PIL import Image, UnidentifiedImageError # UnidentifiedImageErrorをインポート
def get_image_hash(img_path):
try:
with Image.open(img_path) as img:
img = img.resize((8, 8), Image.ANTIALIAS).convert('L')
pixels = list(img.getdata())
avg_pixel = sum(pixels) / len(pixels)
bits = "".join(['1' if (px > avg_pixel) else '0' for px in pixels])
hex_representation = hex(int(bits, 2))
return hex_representation
except UnidentifiedImageError:
print(f"Unable to identify image: {img_path}")
return None
def is_grayscale(img_path):
with Image.open(img_path) as img:
for pixel in img.getdata():
if pixel[0] != pixel[1] != pixel[2]: # R, G, Bの値が異なる場合はカラー画像
return False
return True
def remove_duplicate_images(directory):
image_hashes = {}
deleted_count = 0
for dirpath, dirnames, filenames in os.walk(directory):
for filename in filenames:
if filename.lower().endswith(('.png', '.jpg', '.jpeg', '.gif', '.bmp')):
filepath = os.path.join(dirpath, filename)
if is_grayscale(filepath):
print(f"Deleting grayscale image: {filepath}")
os.remove(filepath)
deleted_count += 1
else:
image_hash = get_image_hash(filepath)
if image_hash in image_hashes:
print(f"Deleting duplicate image: {filepath}")
os.remove(filepath)
deleted_count += 1
else:
image_hashes[image_hash] = filepath
print(f"Total deleted images: {deleted_count}")
# 実行部分
directory = input("Enter the directory path: ")
remove_duplicate_images(directory)
print("作業が終了しました。")
この記事が気に入ったらサポートをしてみませんか?