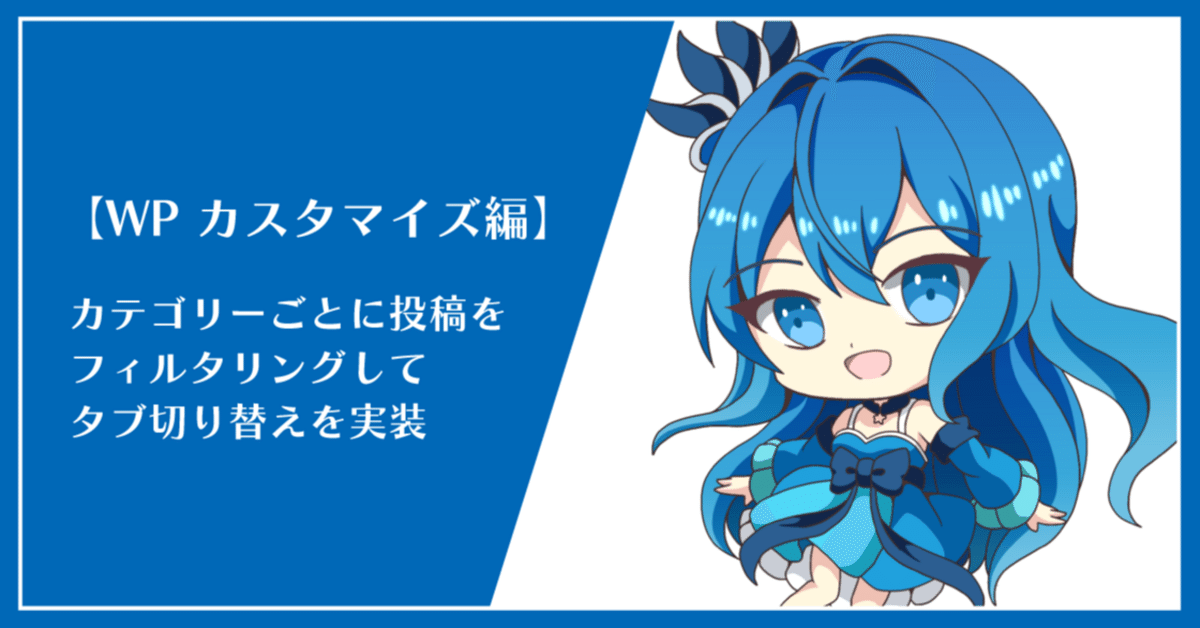
WordPressでカテゴリーごとに投稿をフィルタリングしてタブ切り替えを実装
ども。
WordPressで記事一覧をタブで切り替えるような仕組みを作りたい。
そんな方もいるかと思います。
今回は、WordPressで記事一覧をタブで切り替える方法について説明したいと思います。
まず、タブ一覧の作成。
タクソノミー名はここでは「sample_category」とします。
PHP
<ul class="category-list">
<li class="item" data-category="all"><span class="tab">すべて</span></li>
<?php
$categories = get_terms('sample_category');
foreach ($categories as $category) {
echo '<li class="item" data-category="' . esc_attr($category->slug) . '"><span class="tab">' . esc_html($category->name) . '</span></li>';
}
?>
</ul>
次に記事一覧。
<div class="article-list">
<?php $paged = get_query_var('paged') ? get_query_var('paged') : 1; ?>
<?php
$args = array(
'posts_per_page' => wp_is_mobile() ? 6 : 9,
'post_type' => 'sample',
'order' => 'DESC',
'orderby' => 'modified',
'paged' => $paged
);
$query = new WP_Query($args);
if ($query->have_posts()) : while ($query->have_posts()) : $query->the_post();
$eyecatch_id = get_post_thumbnail_id();
$eyecatch_img = wp_get_attachment_image_src($eyecatch_id, 'large');
$eyecatch = $eyecatch_img[0];
$categories = get_the_terms($post->ID, 'sample_category');
?>
<div class="article">
<div class="article-in">
<div class="article-thumb">
<a href="<?php the_permalink(); ?>">
<?php if ($eyecatch) : ?>
<img src="<?php echo esc_url($eyecatch); ?>" alt="<?php the_title(); ?>">
<?php else : ?>
<img src="<?php echo esc_url(get_template_directory_uri() . '/img/noimage.jpg'); ?>" alt="<?php the_title(); ?>">
<?php endif; ?>
</a>
</div>
<div class="article-info">
<p class="article-date"><?php the_modified_date('Y.m.d') ?></p>
<h3 class="article-title"><?php the_title(); ?></h3>
<ul class="article-cat-list">
<?php foreach ($categories as $category) : ?>
<?php
$cat_id = $category->term_id;
$cat_name = $category->name;
$cat_custom_id = 'category_' . $cat_id;
$cat_color = get_field('category_color', $cat_custom_id);
$cat_color_style = $cat_color ? 'background-color:' . esc_attr($cat_color) . ';' : '';
?>
<li class="item"><a style="<?php echo esc_attr($cat_color_style); ?>" href="<?php echo esc_url(get_category_link($cat_id)); ?>"><?php echo esc_html($cat_name); ?></a></li>
<?php endforeach; ?>
</ul>
</div>
</div>
</div>
<?php endwhile; endif; wp_reset_postdata(); ?>
</div>
JavaScript
そしてクリックイベント用のJavaScript。
これで選択されたタブに基づいて記事をフィルタリングします。
document.addEventListener('DOMContentLoaded', function() {
const tabs = document.querySelectorAll('.category-list .item');
const articles = document.querySelectorAll('.article-list .article');
tabs.forEach(tab => {
tab.addEventListener('click', function() {
const selectedCategory = this.getAttribute('data-category');
articles.forEach(article => {
const articleCategories = article.querySelectorAll('.article-cat-list .item a');
// 該当カテゴリーのチェック
let isVisible = selectedCategory === 'all'; // "すべて"タブの条件
articleCategories.forEach(category => {
if (category.getAttribute('href').includes(selectedCategory)) {
isVisible = true;
}
});
// 表示/非表示の切り替え
if (isVisible) {
article.style.display = 'block';
} else {
article.style.display = 'none';
}
});
});
});
});
これで、カテゴリータブをクリックすることで、対応する投稿だけが表示されるようになります。
各部分のコードは、必要に応じてカスタマイズしてください。
この記事が気に入ったらサポートをしてみませんか?