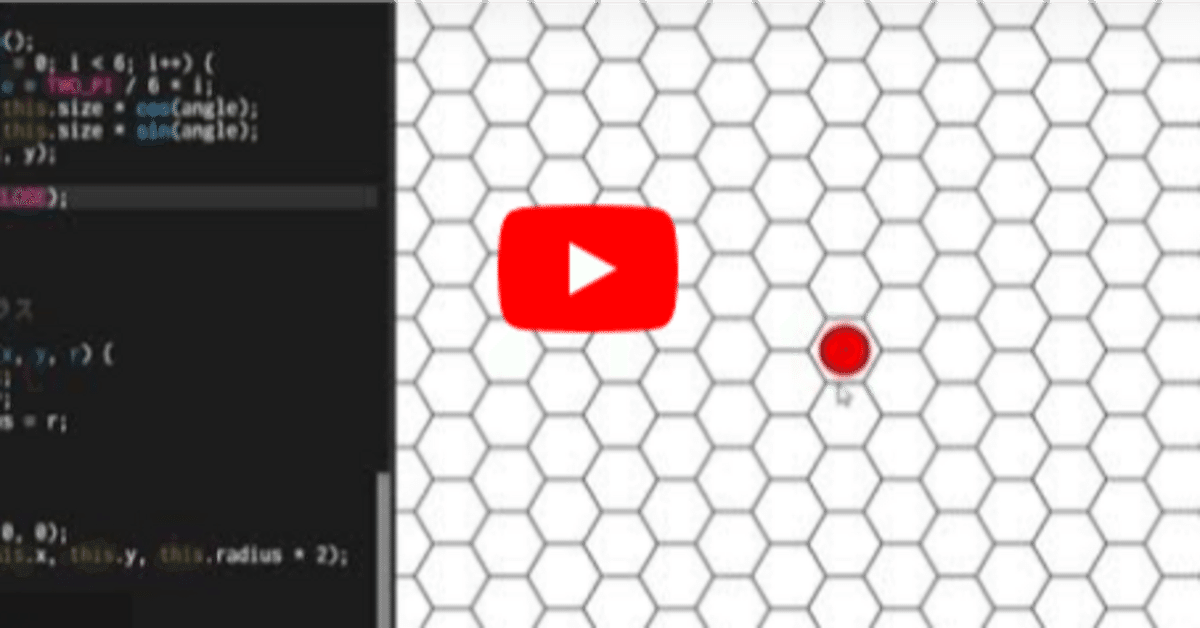
🐢p5.jsでhex map
let cols, rows;
let hexSize = 30;
let hexagons = []; // ヘックスグリッドを格納する配列
let units = []; // ユニットを格納する配列
function setup() {
createCanvas(800, 600);
cols = int(width / (hexSize * 3/2));
rows = int(height / (hexSize * sqrt(3)));
// ヘックスグリッドの生成
for (let r = 0; r < rows; r++) {
for (let c = 0; c < cols; c++) {
let x = hexSize * 3/2 * c;
let y = hexSize * sqrt(3) * (r + 0.5 * (c % 2));
hexagons.push(new Hexagon(x, y, hexSize));
}
}
// 初期ユニットの位置を設定
units.push(new Unit(hexagons[0].x, hexagons[0].y, 20));
}
function draw() {
background(255);
for (let hex of hexagons) {
hex.show();
}
for (let unit of units) {
unit.show();
}
}
function mousePressed() {
// クリックされた位置に最も近いヘックスを見つける
let closestHex = null;
let minDist = Infinity;
for (let hex of hexagons) {
let d = dist(mouseX, mouseY, hex.x, hex.y);
if (d < minDist) {
closestHex = hex;
minDist = d;
}
}
if (closestHex !== null) {
units[0].moveTo(closestHex.x, closestHex.y);
}
}
// ヘックスクラス
class Hexagon {
constructor(x, y, s) {
this.x = x;
this.y = y;
this.size = s;
}
show() {
push();
translate(this.x, this.y);
stroke(0);
noFill();
beginShape();
for (let i = 0; i < 6; i++) {
let angle = TWO_PI / 6 * i;
let x = this.size * cos(angle);
let y = this.size * sin(angle);
vertex(x, y);
}
endShape(CLOSE);
pop();
}
}
// ユニットクラス
class Unit {
constructor(x, y, r) {
this.x = x;
this.y = y;
this.radius = r;
}
show() {
push();
fill(255, 0, 0);
ellipse(this.x, this.y, this.radius * 2);
pop();
}
moveTo(x, y) {
this.x = x;
this.y = y;
}
}
cols と rows は、キャンバスに描画するヘックスの列数と行数を保存します。
hexSize はヘックスの大きさ(半径)を定義します。
hexagons は、生成された全ヘックスオブジェクトを格納する配列です。
units は、画面上のユニットを管理する配列です。
いいなと思ったら応援しよう!
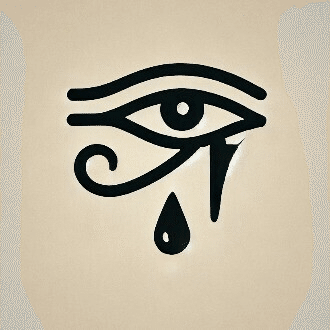