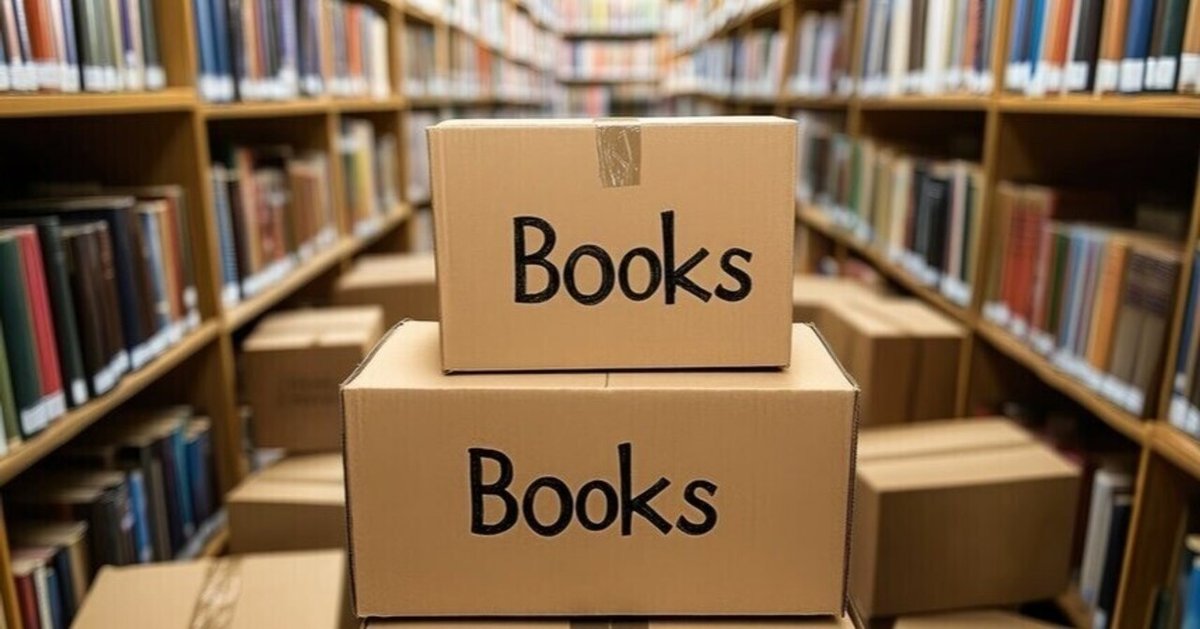
本の重さ+宅配便の箱数計算機.html : made by perplexity & ChatGPT4o
update2024_10_10
斤量の表をビルトインすることに成功しました。
レイアウトも少し変更
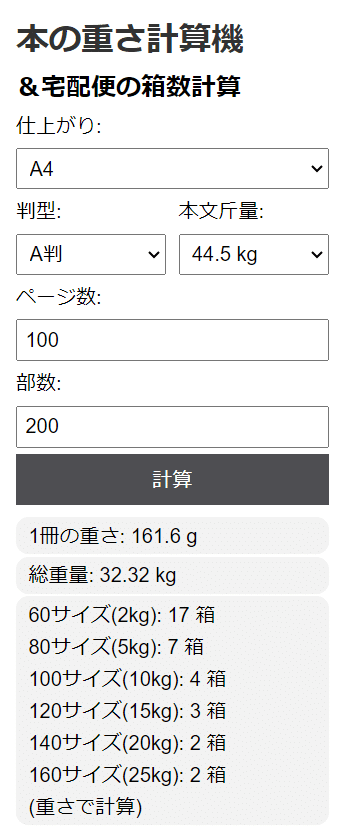
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>本の重さと宅急便箱数計算機</title>
<style>
body { font-family: Arial, sans-serif; line-height: 1.6; margin: 0; padding: 20px; }
.container { display: flex; }
.left-column { flex: 0 0 250px; }
.right-column { width: 250px; margin-left: 20px; }
h1 { color: #333; font-size: 26px; margin-top: 0; margin-bottom: 1px; }
h2 { font-size: 20px; margin-top: 0; margin-bottom: 2px; }
form { margin-bottom: 5px; }
label { display: block; margin-bottom: 5px; }
input, select, button {
width: 100%;
margin-bottom: 5px;
box-sizing: border-box;
padding: 6px;
font-size: 16px;
}
.flex-container {
display: flex;
gap: 10px; /* フィールド間のスペースを調整 */
}
.half-width {
flex: 1;
box-sizing: border-box;
}
button {
background-color: #4e4f52;
color: white;
border: none;
cursor: pointer;
padding: 10px;
font-size: 16px;
}
button:hover { background-color: #45a049; }
#result, #totalWeight, #bookWeight {
background-color: #f2f2f2;
padding: 2px;
padding-left: 10px; /* 右側の空きを調整 */
border-radius: 10px;
margin-top: 2px; /* 上のスペースを調整 */
margin-bottom: 2px; /* 下のスペースを調整 */
height: auto;
}
@media screen and (max-width: 767px) {
body {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
}
.container {
width: 100%;
max-width: 250px;
}
.left-column {
flex: 1;
}
}
</style>
</head>
<body>
<div class="container">
<div class="left-column">
<h1>本の重さ計算機</h1>
<h2>&宅配便の箱数計算</h2>
<form id="calculatorForm">
<label for="bookSize">仕上がり:</label>
<select id="bookSize" required>
<option value="A4">A4</option>
<option value="B5">B5</option>
<option value="A5">A5</option>
</select>
<div class="flex-container">
<div class="half-width">
<label for="paperSize">判型:</label>
<select id="paperSize" required>
<option value="A判">A判</option>
<option value="菊判">菊判</option>
<option value="四六判">四六判</option>
</select>
</div>
<div class="half-width">
<label for="weight">本文斤量:</label>
<select id="weight" required></select>
</div>
</div>
<label for="pages">ページ数:</label>
<input type="number" id="pages" required min="1">
<label for="copies">部数:</label>
<input type="number" id="copies" required min="1">
<button type="button" id="calculateButton">計算</button>
</form>
<div id="bookWeight"></div>
<div id="totalWeight"></div>
<div id="result"></div>
</div>
</div>
<script>
const weightOptions = {
'四六判': [45, 55, 62, 67.5, 68, 70, 72.5, 73, 90, 110, 135, 160, 180],
'菊判': [31, 38, 39.5, 47, 48.5, 50.5, 62.5, 76.5, 93.5, 111, 125],
'A判': [28.5, 35, 36.5, 40, 43, 43.5, 44.5, 46.5, 57.5, 70.5, 86.5]
};
const baseSize = {
'四六判': [788, 1091],
'菊判': [636, 939],
'A判': [625, 880]
};
function updateWeightOptions() {
const paperSize = document.getElementById('paperSize').value;
const weightSelect = document.getElementById('weight');
weightSelect.innerHTML = '';
weightOptions[paperSize].forEach(weight => {
const option = document.createElement('option');
option.value = weight;
option.textContent = weight + ' kg';
weightSelect.appendChild(option);
});
}
function calculateResults() {
const bookSize = document.getElementById('bookSize').value;
const paperSize = document.getElementById('paperSize').value;
const paperWeight = parseFloat(document.getElementById('weight').value);
let pages = parseInt(document.getElementById('pages').value);
const copies = parseInt(document.getElementById('copies').value);
const sizes = {
"A4": [210, 297],
"A5": [148, 210],
"B5": [182, 257]
};
if (!bookSize || isNaN(paperWeight) || isNaN(pages) || isNaN(copies)) {
alert("全てのフィールドに値を入力してください。");
return;
}
// ページ数を偶数に調整
if (pages % 2 !== 0) {
pages++;
document.getElementById('pages').value = pages;
}
const [width, height] = sizes[bookSize];
const [baseWidth, baseHeight] = baseSize[paperSize];
const sheetWeight = paperWeight / (baseWidth * baseHeight) * (width * height);
const book_weight = sheetWeight * pages / 2;
const total_weight = book_weight * copies / 1000;
document.getElementById('bookWeight').innerHTML = `1冊の重さ: ${book_weight.toFixed(1)} g`;
document.getElementById('totalWeight').innerHTML = `総重量: ${total_weight.toFixed(2)} kg`;
const yamato = [
{ name: '60サイズ(2kg)', max: 60, maxWeight: 2000 },
{ name: '80サイズ(5kg)', max: 80, maxWeight: 5000 },
{ name: '100サイズ(10kg)', max: 100, maxWeight: 10000 },
{ name: '120サイズ(15kg)', max: 120, maxWeight: 15000 },
{ name: '140サイズ(20kg)', max: 140, maxWeight: 20000 },
{ name: '160サイズ(25kg)', max: 160, maxWeight: 25000 }
];
const boxVolume = width + height + 10;
let result = '';
let weightCalculation = '';
for (const size of yamato) {
const booksPerBoxByVolume = Math.floor(size.max / boxVolume);
const booksPerBoxByWeight = Math.floor(size.maxWeight / book_weight);
const booksPerBox = Math.min(booksPerBoxByVolume, booksPerBoxByWeight);
let boxesNeeded;
if (booksPerBox > 0) {
boxesNeeded = Math.ceil(copies / booksPerBox);
result += `${size.name}: ${boxesNeeded} 箱(1箱あたり最大${booksPerBox}冊)<br>`;
} else {
boxesNeeded = Math.ceil(total_weight * 1000 / size.maxWeight);
result += `${size.name}: ${boxesNeeded} 箱<br>`;
weightCalculation = '(重さで計算)';
}
}
document.getElementById('result').innerHTML = result + weightCalculation;
}
document.getElementById('calculateButton').addEventListener('click', calculateResults);
document.getElementById('paperSize').addEventListener('change', updateWeightOptions);
window.onload = updateWeightOptions;
document.getElementById('pages').addEventListener('keydown', function(event) {
if (event.key === 'Enter') {
event.preventDefault();
let pages = parseInt(this.value);
if (pages % 2 !== 0) {
pages++;
this.value = pages;
}
document.getElementById('copies').focus();
}
});
document.getElementById('copies').addEventListener('keydown', function(event) {
if (event.key === 'Enter') {
event.preventDefault();
document.getElementById('calculateButton').click();
}
});
</script>
</body>
</html>
今回は本の重さ計算機の第二弾、普段の業務でサクッと使えるよう、本気出してみました(笑)
重さと宅配の箱が何箱になるかワンポチで出してくれる憎いやつw しかも箱サイズ60サイズ~160サイズ全バリエーション対応❗
これマジでしょっちゅう電卓弾いてるんですよ^^;
まぁ私の場合、背幅計算して20~30cmくらいの箱で何箱?って感じでやってるんですけど、封入業者とかって聞いてみると、見積りは重さでやってますよね。この流れで「+パレット数計算機」も追加してみよかしら🤣
実はこの二段組レイアウトにするのに今回、perplexityで無限ループにはまり2時間以上かかっちゃいましたヨ😂 何度言っても分からんちんなのでCopilot@MSとEdge、Geminiにも浮気しに行ったけど正直お話になりませんでした。
やはり、現状私個人的にはperplexityとchatGPTです。的を得ているのは。
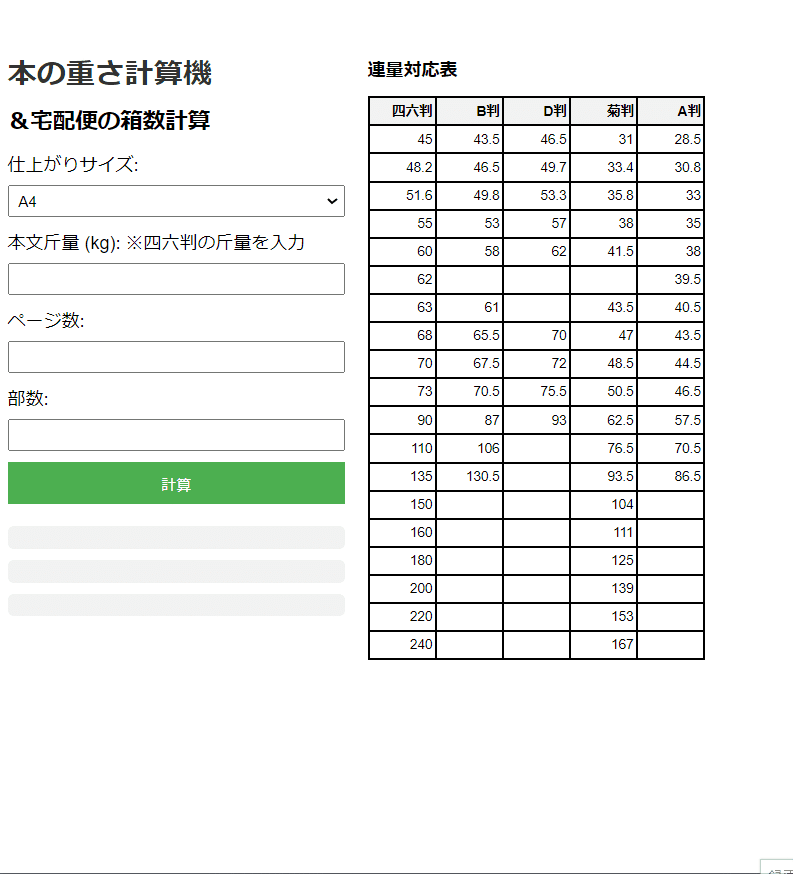
ご注意:計算でやっているので、1gオーバーしても箱数が1箱増えてしまいます。ただ表紙に厚紙を使っているようなケースでは逆に丁度良かったりすると思いますので、まぁその辺は臨機応変で±1箱くらいは想定してくださいな😆
htmlは下記になります。
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>本の重さ計算機</title>
<style>
body { font-family: Arial, sans-serif; line-height: 1.6; margin: 0; padding: 20px; }
.container { display: flex; }
.left-column { flex: 0 0 300px; }
.right-column { width: 300px; margin-left: 20px; }
h1 { color: #333; font-size: 26px; margin-bottom: 5px; }
h2 { font-size: 20px; margin-top: 0; margin-bottom: 10px; }
form { margin-bottom: 10px; }
label { display: block; margin-bottom: 5px; }
input, select, button {
width: 100%;
margin-bottom: 10px;
box-sizing: border-box;
padding: 5px;
}
button {
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
padding: 10px;
}
button:hover { background-color: #45a049; }
#result, #totalWeight, #bookWeight {
background-color: #f2f2f2;
padding: 10px;
border-radius: 5px;
margin-bottom: 10px;
height: auto;
}
#table-container {
width: 100%;
margin-top: 22px; /* 表全体を下げるためにマージンを増やす */
}
#table-container h2 { font-size: 16px; margin-top: 0; }
table {
width: 100%;
border-collapse: collapse;
font-size: 12px;
table-layout: fixed;
border: 2px solid #000; /* 表全体の罫線を太くする */
}
th, td {
border: 2px solid #000; /* セルの罫線を太くする */
padding: 2px;
text-align: right;
width: 20%;
}
th { background-color: #f2f2f2; }
</style>
</head>
<body>
<div class="container">
<div class="left-column">
<h1>本の重さ計算機</h1>
<h2>&宅配便の箱数計算</h2>
<form id="calculatorForm">
<label for="bookSize">仕上がりサイズ:</label>
<select id="bookSize" required>
<option value="A4">A4</option>
<option value="B5">B5</option>
<option value="A5">A5</option>
</select>
<label for="weight">本文斤量 (kg): ※四六判の斤量を入力</label>
<input type="number" id="weight" step="0.1" required min="0.1">
<label for="pages">ページ数:</label>
<input type="number" id="pages" required min="1">
<label for="copies">部数:</label>
<input type="number" id="copies" required min="1">
<button type="button" id="calculateButton">計算</button>
</form>
<div id="bookWeight"></div>
<div id="totalWeight"></div>
<div id="result"></div>
</div>
<div class="right-column">
<div id="table-container">
<h2>連量対応表</h2>
<table>
<tr><th>四六判</th><th>B判</th><th>D判</th><th>菊判</th><th>A判</th></tr>
<tr><td>45</td><td>43.5</td><td>46.5</td><td>31</td><td>28.5</td></tr>
<tr><td>48.2</td><td>46.5</td><td>49.7</td><td>33.4</td><td>30.8</td></tr>
<tr><td>51.6</td><td>49.8</td><td>53.3</td><td>35.8</td><td>33</td></tr>
<tr><td>55</td><td>53</td><td>57</td><td>38</td><td>35</td></tr>
<tr><td>60</td><td>58</td><td>62</td><td>41.5</td><td>38</td></tr>
<tr><td>62</td><td></td><td></td><td></td><td>39.5</td></tr>
<tr><td>63</td><td>61</td><td></td><td>43.5</td><td>40.5</td></tr>
<tr><td>68</td><td>65.5</td><td>70</td><td>47</td><td>43.5</td></tr>
<tr><td>70</td><td>67.5</td><td>72</td><td>48.5</td><td>44.5</td></tr>
<tr><td>73</td><td>70.5</td><td>75.5</td><td>50.5</td><td>46.5</td></tr>
<tr><td>90</td><td>87</td><td>93</td><td>62.5</td><td>57.5</td></tr>
<tr><td>110</td><td>106</td><td></td><td>76.5</td><td>70.5</td></tr>
<tr><td>135</td><td>130.5</td><td></td><td>93.5</td><td>86.5</td></tr>
<tr><td>150</td><td></td><td></td><td>104</td><td></td></tr>
<tr><td>160</td><td></td><td></td><td>111</td><td></td></tr>
<tr><td>180</td><td></td><td></td><td>125</td><td></td></tr>
<tr><td>200</td><td></td><td></td><td>139</td><td></td></tr>
<tr><td>220</td><td></td><td></td><td>153</td><td></td></tr>
<tr><td>240</td><td></td><td></td><td>167</td><td></td></tr>
</table>
</div>
</div>
</div>
<script>
function calculateResults() {
const bookSize = document.getElementById('bookSize').value;
const paperWeight = parseFloat(document.getElementById('weight').value);
const pages = parseInt(document.getElementById('pages').value);
const copies = parseInt(document.getElementById('copies').value);
const sizes = {
"A4": [210, 297],
"A5": [148, 210],
"B5": [182, 257]
};
const [width, height] = sizes[bookSize];
const book_weight = paperWeight / (1091 * 788) * (width * height) * pages / 2;
const total_weight = book_weight * copies / 1000;
document.getElementById('bookWeight').innerHTML = `1冊の重さ: ${book_weight.toFixed(1)}g`;
document.getElementById('totalWeight').innerHTML = `総重量: ${total_weight.toFixed(2)}kg`;
const yamato = [
{ name: '60サイズ (2kg)', max: 60, maxWeight: 2000 },
{ name: '80サイズ (5kg)', max: 80, maxWeight: 5000 },
{ name: '100サイズ (10kg)', max: 100, maxWeight: 10000 },
{ name: '120サイズ (15kg)', max: 120, maxWeight: 15000 },
{ name: '140サイズ (20kg)', max: 140, maxWeight: 20000 },
{ name: '160サイズ (25kg)', max: 160, maxWeight: 25000 }
];
const boxVolume = width + height + 10;
let result = '';
for (const size of yamato) {
const booksPerBoxByVolume = Math.floor(size.max / boxVolume);
const booksPerBoxByWeight = Math.floor(size.maxWeight / book_weight);
const booksPerBox = Math.min(booksPerBoxByVolume, booksPerBoxByWeight);
let boxesNeeded;
if (booksPerBox > 0) {
boxesNeeded = Math.ceil(copies / booksPerBox);
} else {
boxesNeeded = Math.ceil(total_weight * 1000 / size.maxWeight);
}
result += `${size.name}: ${boxesNeeded}箱`;
if (booksPerBox > 0) {
result += ` (1箱あたり最大${booksPerBox}冊)`;
} else {
result += ` (重さで計算)`;
}
result += '<br>';
}
document.getElementById('result').innerHTML = result;
}
document.getElementById('calculateButton').addEventListener('click', calculateResults);
const inputs = ['bookSize', 'weight', 'pages', 'copies'];
inputs.forEach((id, index) => {
document.getElementById(id).addEventListener('keydown', function(e) {
if (e.key === 'Enter') {
e.preventDefault();
if (index < inputs.length - 1) {
// 次の入力欄にフォーカスを移動
document.getElementById(inputs[index + 1]).focus();
} else {
// 最後の入力欄の場合、計算を実行
calculateResults();
}
}
});
});
// フォーム全体のエンターキー押下時の動作を防止
document.getElementById('calculatorForm').addEventListener('submit', function(e) {
e.preventDefault();
});
</script>
</html>
おまけ:上記htmlのウィンドウ右上にポインタを持っていくとコピーマークが出るので全体をコピーし、メモ帳等にペーストし拡張子.txtを.htmlに書き換えれば使えるようになります。
🔴google driveに置いてiphoneでは見れません。dropboxでは見れました。他のホスティングサービスは試していません(契約していないので)
🔴google driveに置いて物理ドライブ(G)(PC版googledrive,昔で言うドライブファイルストリーム)では普通に開けます。
🔴googole driveに置いて右クリックから.htmlへのハイパーリンを取得し、社内メール等でリンクURLを送って開いたものは一旦webブラウザ内でhtmlコードが開くのでブラウザ上部のGoogleChromeで開くをクリックすれば見れます。(iphoneのchromeとsafariではこれが出て来ませんでした)
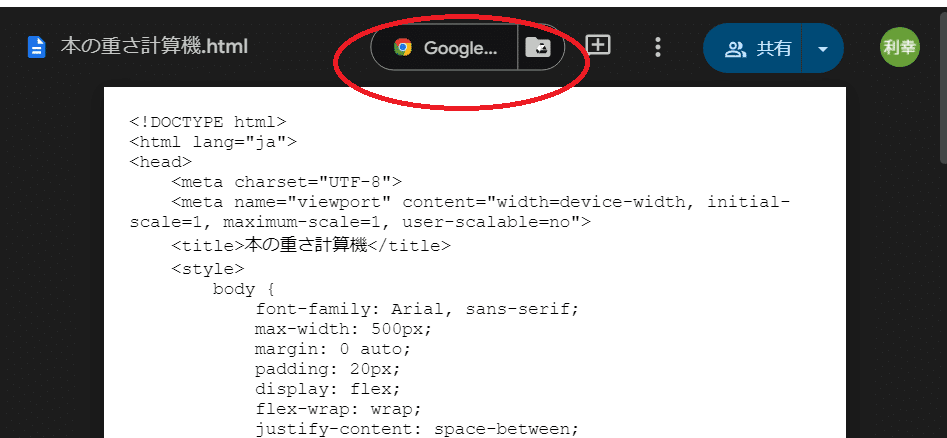