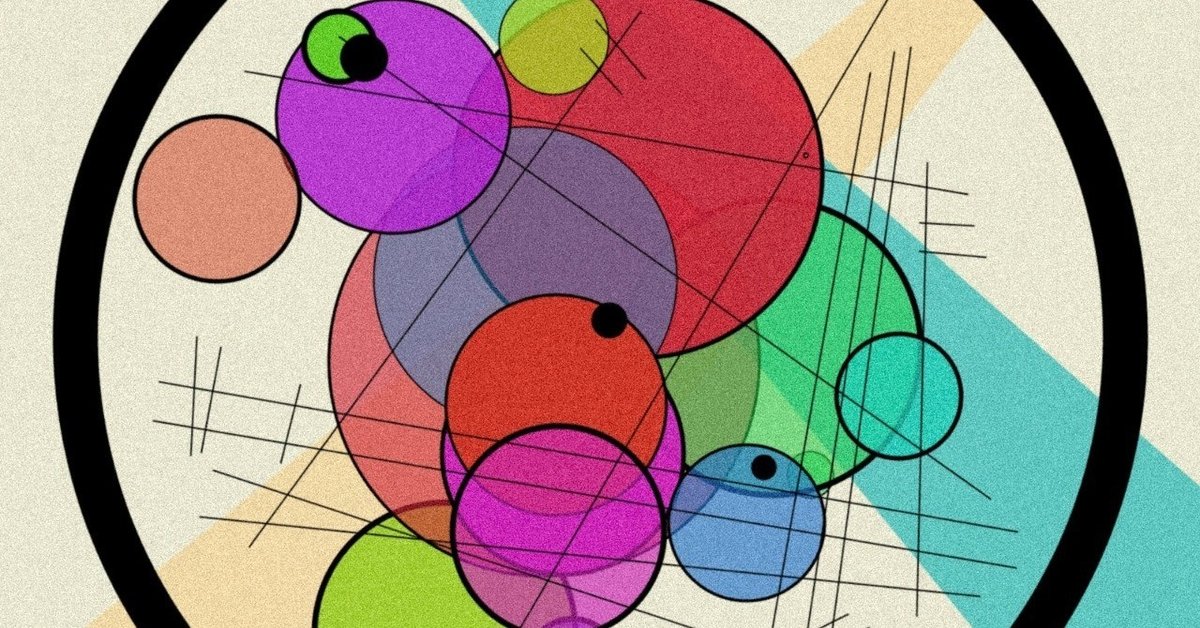
ProcessingでGenerative art #79
lineは全部print(mouseX, mouseY)で座標を調べて打ちました。
Code
int count = 20;
Circle[] circles = new Circle[count];
color[] col = new color[count];
int[] smalCircleDimeter = new int[count];
float largeCircleDiameter = 700;
int largeCircleStrokeW = 30;
void setup() {
size(800, 800);
pixelDensity(2);
colorMode(HSB, 360, 100, 100, 100);
smooth();
for (int i = 0; i < count; i ++) {
smalCircleDimeter[i] = (int)random(map(i, 0, count, 350, 10));
circles[i] = new Circle(smalCircleDimeter[i]);
if (i >= count - 3) {
col[i] = color(0);
} else {
col[i] = color(random(360), 80, 80, random(50, 100));
}
}
}
void draw() {
background(58, 10, 91);
noStroke();
fill(40, 36, 95, 80);
triangle(-200, height, 100, height + 100, width + 10, -10);
fill(175, 52, 78);
triangle(width, height - 20, width, height - 300, 0, - 200);
stroke(0);
strokeWeight(largeCircleStrokeW);
noFill();
ellipse(width/2, height/2, largeCircleDiameter, largeCircleDiameter);
stroke(0);
for (int i = 0; i < count; i ++) {
fill(col[i]);
circles[i].run();
}
drawLine();
}
void drawLine() {
stroke(0);
strokeWeight(1);
line(163, 223, 645, 305);
line(102, 456, 677, 554);
line(595, 169, 297, 679);
line(607, 207, 547, 652);
line(618, 284, 611, 586);
line(142, 489, 582, 634);
line(427, 183, 166, 540);
line(226, 200, 660, 508);
line(375, 658, 228, 670);
line(262, 637, 374, 717);
line(414, 238, 387, 208);
line(430, 228, 397, 189);
line(613, 324, 649, 326);
line(613, 343, 657, 347);
line(133, 520, 653, 573);
line(596, 210, 531, 643);
line(580, 224, 509, 627);
line(590, 606, 502, 651);
line(503, 634, 579, 594);
line(497, 619, 565, 585);
line(678, 530, 106, 430);
line(127, 479, 131, 400);
line(134, 477, 147, 407);
line(187, 484, 200, 432);
}
void mousePressed() {
for (int i = 0; i < count; i ++) {
smalCircleDimeter[i] = (int)random(map(i, 0, count, 350, 10));
circles[i] = new Circle(smalCircleDimeter[i]);
if (i >= count - 3) {
col[i] = color(0);
} else {
col[i] = color(random(360), 80, 80, random(50, 100));
}
}
}
void keyPressed() {
if (key == 's')saveFrame("####.png");
}
//----------------------------------------------------------------
class Circle {
PVector postion;
PVector velocity ;
int diameter;
int strokeW;
Circle(int d) {
postion = new PVector(width/2, height/2);
velocity = new PVector(random(-3, 3), random(-3, 3));
diameter = d;
strokeW = (int)random(1, 5);
}
void run() {
display();
move();
checkHit();
}
void display() {
strokeWeight(strokeW);
ellipse(postion.x, postion.y, diameter, diameter);
}
void move() {
postion.add(velocity);
}
void checkHit() {
if (dist(postion.x, postion.y, width/2, height/2) > (largeCircleDiameter - diameter - largeCircleStrokeW)/2) {
velocity.mult(random(-1, -2));
}
}
}
Happy coding!
応援してくださる方!いつでもサポート受け付けてます!