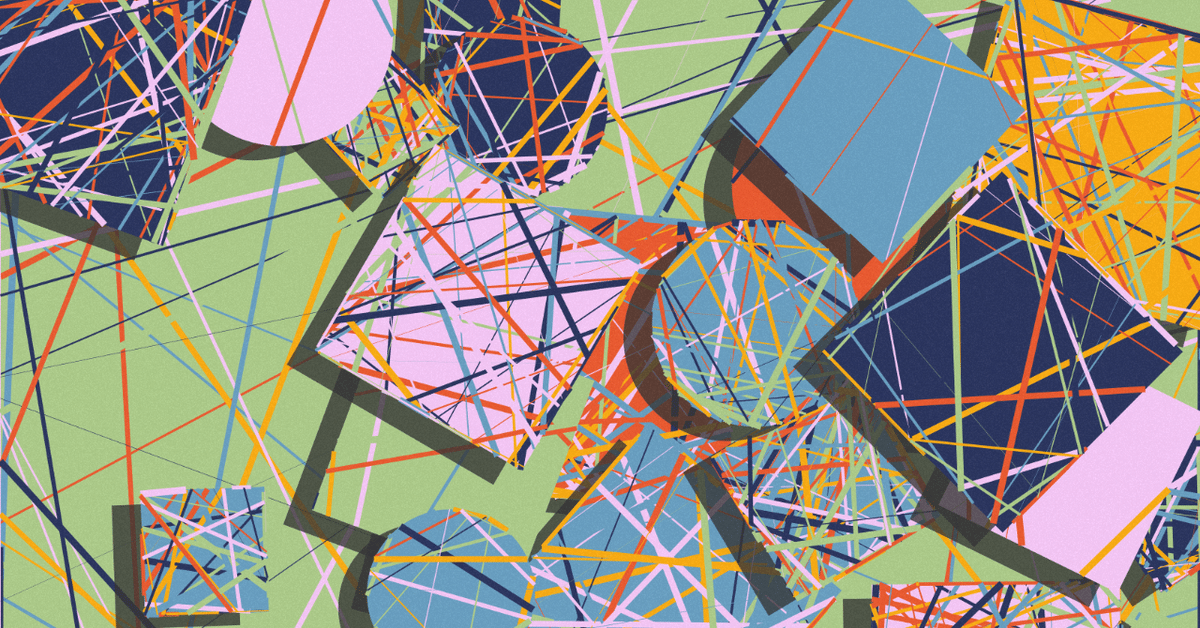
Generative Art #120
Code
Form[] forms = new Form[50];
float maxSize = 200, minSize = 70;
void setup() {
size(840, 840);
pixelDensity(2);
noLoop();
strokeCap(SQUARE);
rectMode(CENTER);
newForm();
}
void draw() {
mySquare(width/2, height/2, width);
for (int i = 0; i < forms.length; i ++) {
forms[i].show();
}
}
void mySquare(float x, float y, float s) {
float hs = s/2;
ArrayList<PVector> points = new ArrayList<PVector>();
for (float i = -s/2; i < s/2; i ++) {
points.add(new PVector(i, -hs));
points.add(new PVector(i, hs));
points.add(new PVector(-hs, i));
points.add(new PVector(hs, i));
}
pushMatrix();
translate(x, y);
noStroke();
fill(getCol());
square(0, 0, s);
for (int i = 0; i < 100; i ++) {
stroke(getCol());
noFill();
strokeWeight(random(5));
PVector p1 = points.get((int)random(points.size()));
PVector p2 = points.get((int)random(points.size()));
line(p1.x, p1.y, p2.x, p2.y);
}
popMatrix();
}
void newForm() {
for (int i = 0; i < forms.length; i ++) {
forms[i] = new Form(random(width), random(height), random(minSize, maxSize));
}
}
void mousePressed() {
redraw();
newForm();
}
void keyPressed() {
if (key == 's')saveFrame("####.png");
}
int[] colors = {#e4572e, #29335c, #f3a712, #a8c686, #669bbc, #efc2f0};
int getCol() {
return colors[(int)random(colors.length)];
}
class Form {
float x, y, s;
float hs;
float angle;
float shiftX, shiftY;
int lineCount;
color shadow;
Form(float x, float y, float s) {
this.x = x;
this.y = y;
this.s = s;
hs = s/2;
angle = random(TAU);
shiftX = -20;
shiftY = 10;
lineCount = (int)random(120);
shadow = color(50, 200);
}
void show() {
if (random(1) < 0.5) {
fill(shadow);
noStroke();
circle(x+shiftX, y+shiftY, s);
myCircle();
} else {
pushMatrix();
translate(x+shiftX, y+shiftY);
rotate(angle);
fill(shadow);
noStroke();
square(0, 0, s);
popMatrix();
pushMatrix();
translate(x, y);
rotate(angle);
mySquare();
popMatrix();
}
}
void myCircle() {
ArrayList<PVector> points = new ArrayList<PVector>();
for (float a = 0; a < 360; a += TAU/360) {
float posX = x + hs * cos(a);
float posY = y + hs * sin(a);
points.add(new PVector(posX, posY));
}
noStroke();
fill(getCol());
circle(x, y, s);
for (int i = 0; i < lineCount; i ++) {
stroke(getCol());
strokeWeight(random(5));
PVector p1 = points.get((int)random(points.size()));
PVector p2 = points.get((int)random(points.size()));
line(p1.x, p1.y, p2.x, p2.y);
}
}
void mySquare() {
ArrayList<PVector> points = new ArrayList<PVector>();
for (float i = -s/2; i < s/2; i ++) {
points.add(new PVector(i, -hs));
points.add(new PVector(i, hs));
points.add(new PVector(-hs, i));
points.add(new PVector(hs, i));
}
noStroke();
fill(getCol());
square(0, 0, s);
for (int i = 0; i < lineCount; i ++) {
stroke(getCol());
noFill();
strokeWeight(random(5));
PVector p1 = points.get((int)random(points.size()));
PVector p2 = points.get((int)random(points.size()));
line(p1.x, p1.y, p2.x, p2.y);
}
}
}
この構造はまず図形のアウトラインの位置(円の場合は360個)をArrayListに入れて
そこからランダムな2点p1,p2を選んで線で結ぶ
これをlineCountの数だけ繰り返す。
そんなふうにできてます。
影はfillを黒にして透明度をあげた図形を先に描画してその上から少しずらして本体を描画する。むしろそのパターンしかできない。
細く伸びる影とか作ってみたい。
Happy coding!!!
応援してくださる方!いつでもサポート受け付けてます!