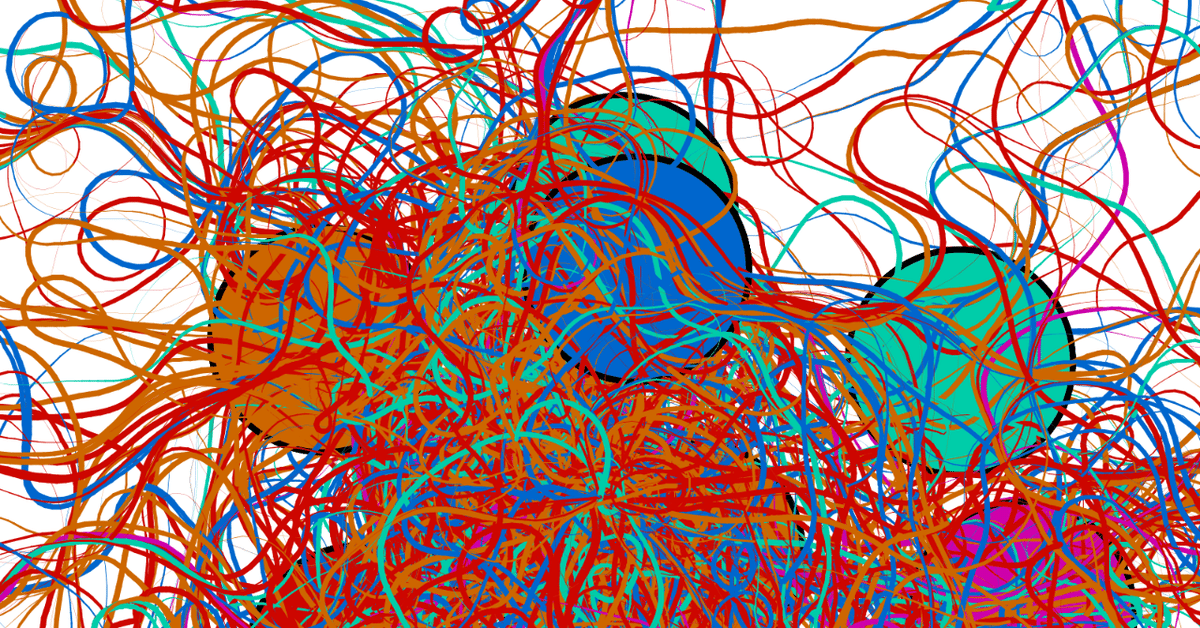
ProcessingでGenerative art #71
Code
Particle[] particles = new Particle[300];
int actRandomSeed = 0;
float noiseScale = 800, noiseStrength = 30;
float pAlpha = 100, strokeWidth = 4;
int drawMode = 1;
void setup() {
size(800, 800);
pixelDensity(2);
colorMode(HSB, 360, 100, 100, 100);
background(360);
for (int i=0; i<particles.length; i++) {
particles[i] = new Particle();
}
}
void draw() {
randomSeed(actRandomSeed);
for (int i=0; i<particles.length; i++) {
particles[i].run();
}
if (mousePressed) {
strokeWeight(4);
stroke(0);
ellipse(mouseX, mouseY, 150, 150);
}
}
void keyPressed() {
if (key == '1')drawMode = 1;
if (key == '2')drawMode = 2;
if (key == '3')drawMode = 3;
if (key == '4')drawMode = 1;
if (key == 's')saveFrame("####.png");
if (key == BACKSPACE)background(360);
if (key == ' ') {
int newNoiseSeed = (int)random(10000);
noiseSeed(newNoiseSeed);
}
}
void mousePressed() {
actRandomSeed = (int)random(10000);
}
//-----------------------------------------------------------------
class Particle {
PVector pos, posOld;
float stepSize, angle;
boolean isOutside = false;
color col;
float t;
float noiseZ;
Particle() {
pos = new PVector(width/2, height/2);
posOld = new PVector(pos.x, pos.y);
stepSize = random(1, 7);
t = random(10);
noiseZ = random(10);
}
void update() {
angle = noise(pos.x/noiseScale, pos.y/noiseScale, noiseZ) * noiseStrength;
pos.x += cos(angle) * stepSize;
pos.y += sin(angle) * stepSize;
if (pos.x<-10) isOutside = true;
else if (pos.x>width+10)isOutside = true;
else if (pos.y<-10)isOutside = true;
else if (pos.y>height+10)isOutside = true;
if (isOutside) {
pos.x = width/2;
pos.y = height/2;
posOld.set(pos);
}
noiseZ += 0.01;
t += 0.05;
}
void draw() {
changeDrawMode();
stroke(col, pAlpha);
fill(col);
strokeWeight(map(sin(t), -1, 1,0 , strokeWidth));
line(posOld.x, posOld.y, pos.x, pos.y);
posOld.set(pos);
isOutside = false;
}
void run() {
update();
draw();
}
void changeDrawMode(){
if(drawMode == 1){
if(random(1) > 0.66)col = color(5, 100, 100);
else if(random(1) > 0.33) col = color(360);
else col = color(0);
}
if(drawMode == 2){
if(random(1) > 0.66)col = color(305, 59, 100);
else if(random(1) > 0.33)col = color(120, 75, 59);
else col = color(random(360));
}
if(drawMode == 3){
if(random(1) > 0.8)col = color(210, 100, 100);
else if(random(1) > 0.6)col = color(2, 100, 100);
else if(random(1) > 0.4)col = color(30, 100, 100);
else if(random(1) > 0.2)col = color(170, 100, 100);
else col = color(310, 100, 80);
}
}
}
Happy coding!!
応援してくださる方!いつでもサポート受け付けてます!