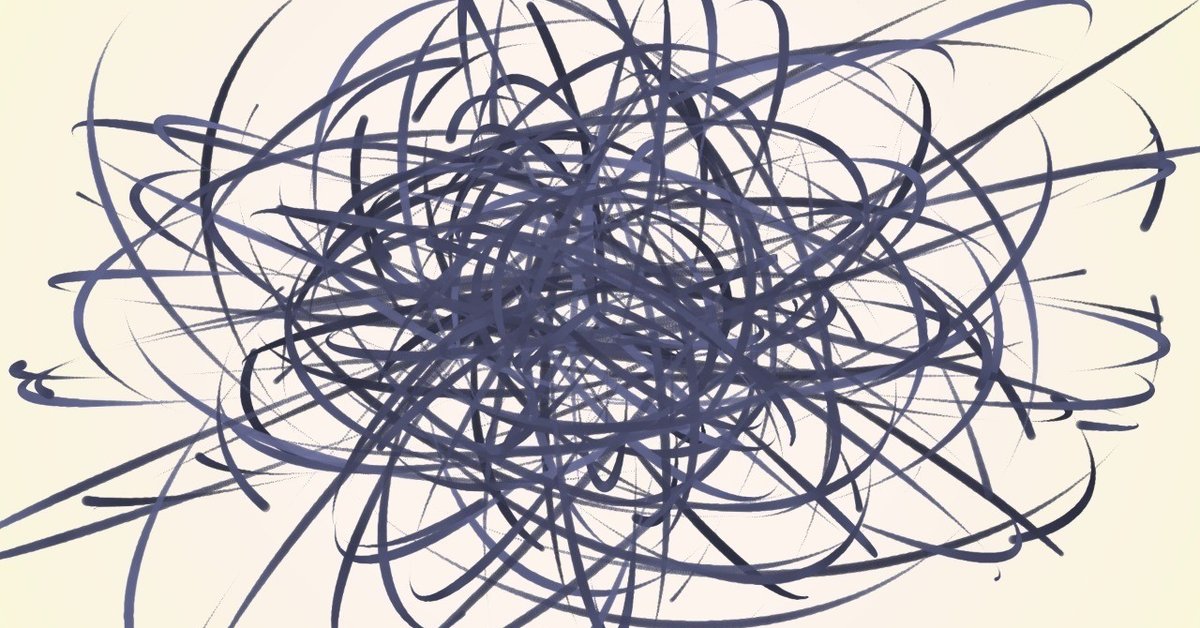
ProcessingによるGenerative art作品#7
中心に引力が働いていて、太陽を中心に公転する惑星のように回っています。
シンプルに軌跡だけを描画してもつまらなかったのでsinでstrokeWeightを増減させました。
書籍Nature of codeを参考にしました。
読んでいるとシミュレートできることがどんどん増えていっているのでワクワクします。
Code
Mover[] m = new Mover[50];
Attractor a;
void setup() {
size(960, 540);
pixelDensity(2);
smooth();
colorMode(HSB, 360, 100, 100, 100);
background(360);
for (int i = 0; i < m.length; i++) {
m[i] = new Mover(random(10), random(0, width), random(0, height));
}
a = new Attractor();
}
void draw() {
if (mousePressed) {
background(360);
}
for (int i = 0; i < m.length; i++) {
PVector force = a.attract(m[i]);
m[i].applyForce(force);
m[i].display();
m[i].update();
}
}
void keyPressed() {
if (key == ' ') {
saveFrame("####.png");
}
}
//--------------------------------------------------------
class Mover {
PVector location;
PVector velocity;
PVector acceleration;
float mass;
float colnoise;
float t;
Mover(float m, float x, float y) {
mass = m;
location = new PVector(x, y);
velocity = new PVector(random(-1, 1), random(-1, 1));
acceleration = new PVector(0, 0);
t = random(360);
}
void applyForce(PVector force) {
PVector f = PVector.div(force, mass);
acceleration.add(f);
}
void update() {
velocity.add(acceleration);
location.add(velocity);
acceleration.mult(0);
t += 1;
colnoise += 0.007;
}
void display() {
color col = color(map(noise(colnoise), 0, 1, 0, 250), 50);
stroke(col);
strokeWeight((4*sin(radians(t)))+4);
point(location.x, location.y);
}
}
//--------------------------------------------------------
class Attractor {
float mass;
float G;
PVector location;
Attractor() {
location = new PVector(width/2, height/2);
mass = 20;
G = 0.5;
}
PVector attract(Mover m) {
PVector force = PVector.sub(location, m.location);
float d = force.mag();
d = constrain(d, 5.0, 25.0);
force.normalize();
float strength = (G * mass * m.mass) / (d * d * 2);
force.mult(strength);
return force;
}
void display() {
strokeWeight(4);
stroke(0);
point(location.x, location.y);
}
}
Happy coding!
応援してくださる方!いつでもサポート受け付けてます!