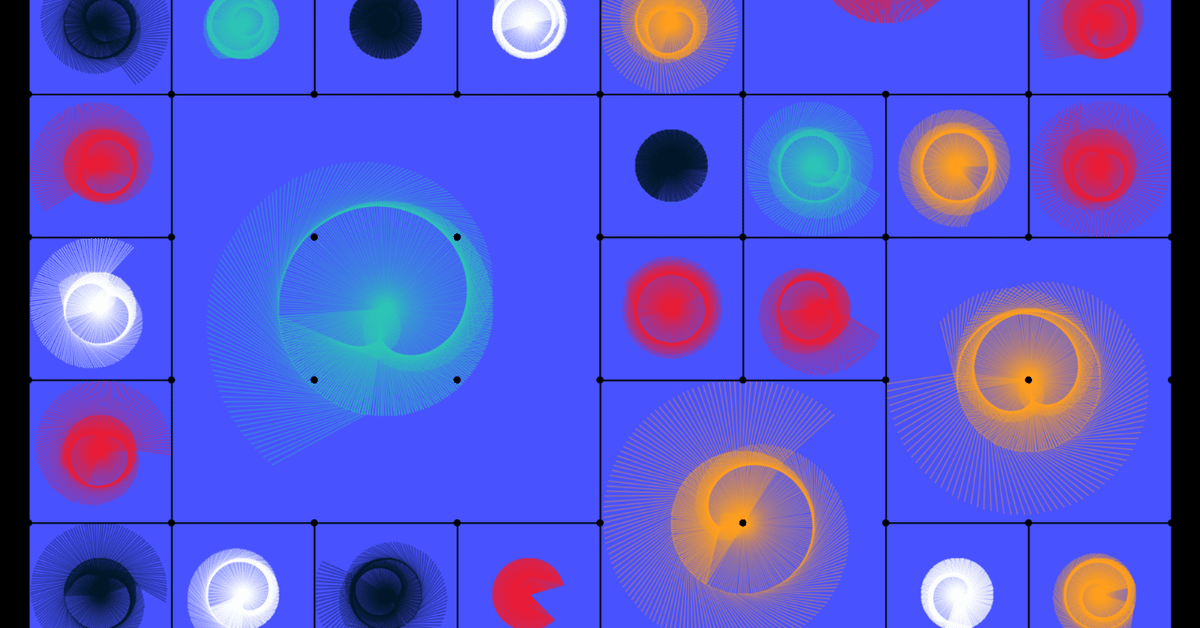
Generative art #103
Code
ArrayList<Form> forms;
ArrayList<Pendulum> pendulums;
Grid grid;
int drawMode = 1;
void setup() {
size(840, 840);
pixelDensity(2);
noLoop();
grid = new Grid(20, 20, 800, 800, 100);
newForms();
}
void draw() {
background(0);
if (drawMode == 1) {
blendMode(BLEND);
}
if (drawMode == 2) {
blendMode(ADD);
}
for (int i = 0; i < forms.size(); i ++) {
Form f = forms.get(i);
f.show();
Pendulum p = pendulums.get(i);
stroke(getCol(), 90);
for (int j = 0; j < 300; j ++) {
p.run();
}
}
grid.show();
}
void newForms() {
forms = new ArrayList<Form>();
pendulums = new ArrayList<Pendulum>();
boolean useGrid[][] = new boolean[grid.cw][grid.cw];
int emptys = grid.cw*grid.cw;
while (emptys > 0) {
float t = grid.t;
int w = int(random(1, grid.cw));
int x = int(random(grid.cw-w+1));
int y = int(random(grid.ch-w+1));
boolean colision = true;
for (int j = 0; j < w; j++) {
for (int i = 0; i < w; i++) {
if (useGrid[x+i][y+j]) {
colision = false;
break;
}
}
}
if (colision) {
for (int j = 0; j < w; j ++) {
for (int i = 0; i < w; i ++) {
useGrid[x+i][y+j] = true;
}
}
forms.add(new Form(grid.x + x*t, grid.y+y*t, w*t, w*t));
pendulums.add(new Pendulum(grid.x + x*t + (w*t)/2, grid.y+y*t + (w*t)/2, (w*t)/2));
emptys -= w*w;
}
}
}
int[] colors = {#011627, #FDFFFC, #2EC4B6, #E71D36, #FF9F1C};
int getCol() {
return colors[(int)random(colors.length)];
}
void mousePressed() {
newForms();
redraw();
}
void keyPressed() {
if (key == 's')saveFrame("####.png");
if (key == '1')drawMode = 1;
if (key == '2')drawMode = 2;
}
//---------------------------------------------------------------
class Form {
color col;
float x, y, w, h;
Form(float x, float y, float w, float h) {
this.x = x;
this.y = y;
this.w = w;
this.h= h;
}
void show() {
stroke(255);
fill(0);
if (drawMode == 1) {
stroke(0);
fill(#4852FF);
}
rect(x, y, w, h);
}
}
//---------------------------------------------------------------
class Grid {
int cw, ch;
float x, y, w, h, t;
Grid(float x, float y, float w, float h, float t) {
this.x = x;
this.y = y;
this.w = w;
this.h = h;
this.t = t;
cw = int(w/t);
ch = int(h/t);
}
void show() {
noStroke();
fill(0);
for (float j = y; j <= y+h; j+=t) {
for (float i = x; i <= x+w; i+=t) {
circle(i, j, 5);
}
}
}
}
//---------------------------------------------------------------
class Pendulum {
float x, y;
float len1, len2;
float angle1, angle2;
float aStep1, aStep2;
Pendulum(float x, float y, float l) {
this.x = x;
this.y = y;
len1 = l/2;
len2 = l-len1;
angle1 = random(PI);
angle2 = random(PI);
aStep1 = radians(random(1,3));
aStep2 = radians(random(1));
}
void run() {
show();
move();
}
void show() {
pushMatrix();
translate(x, y);
rotate(angle1);
line(0, 0, len1, 0);
push();
translate(len1, 0);
rotate(angle2);
line(0, 0, len2, 0);
pop();
popMatrix();
}
void move() {
angle1 += aStep1;
angle2 += aStep2;
}
}
正方形を充填させる形。
そこに二重振り子的な形を置いて、アクセサリーケースのようなイメージで。
しばらくblendMode()で光らせてなかったなと思ったから使いたかった。
Happy coding!
応援してくださる方!いつでもサポート受け付けてます!