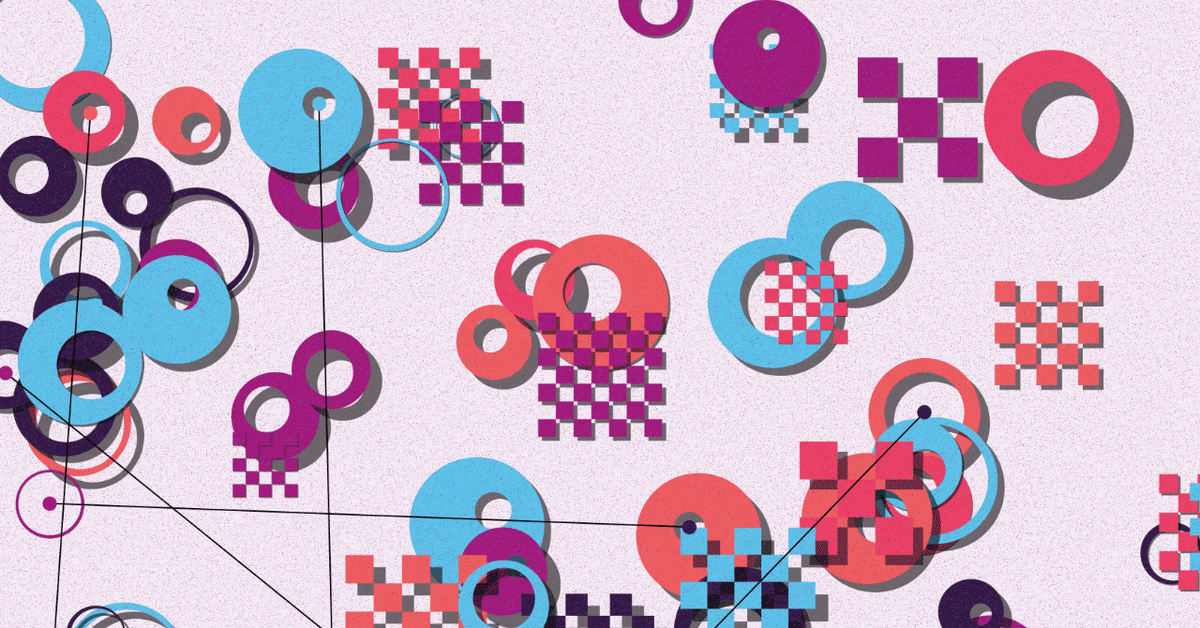
Generative Art #121
Code
Form[] form = new Form[100];
ArrayList<PVector> points;
void setup() {
size(840, 840);
pixelDensity(2);
noLoop();
colorMode(HSB, 360, 100, 100, 100);
newForm();
}
void draw() {
points = new ArrayList();
background(#F5EBF5);
for (int i = 0; i < form.length; i ++) {
form[i].show();
}
node();
grain();
}
void grain() {
for (int i = 0; i < 500000; i ++) {
stroke(getCol(), 50);
strokeWeight(0.4);
point(random(width), random(height));
}
}
void node(){
strokeWeight(1);
for(int i = 0; i < 6; i ++){
PVector p1 = points.get((int)random(points.size()));
PVector p2 = points.get((int)random(points.size()));
stroke(0);
line(p1.x, p1.y, p2.x, p2.y);
noStroke();
fill(getCol());
circle(p1.x, p1.y, 10);
fill(getCol());
circle(p2.x, p2.y, 10);
}
}
void mousePressed() {
redraw();
newForm();
}
void newForm() {
for (int i = 0; i < form.length; i ++) {
form[i] = new Form(random(width), random(height), random(40, 100));
}
}
void keyPressed() {
if (key == 's')saveFrame("####.png");
}
int[] colors = {#5BC3EB, #ef5d60, #ec4067, #a01a7d, #311847};
int getCol() {
return colors[(int)random(colors.length)];
}
//------------------------------------------------------------
class Form {
float x, y, s;
float r, shiftX, shiftY;
int tileCount;
float shdwPos;
color shdwCol;
Form(float x, float y, float s) {
this.x = x;
this.y = y;
this.s = s;
r = random(s*0.1, s*0.48);
shiftX = random(-((s/2) - r)/2, ((s/2) - r)/2);
shiftY = random(-((s/2) - r)/2, ((s/2) - r)/2);
tileCount = (int)random(3, 8);
shdwPos = random(10);
shdwCol = color(20, 60);
}
void show() {
noStroke();
fill(getCol());
if (random(1) < 0.7) {
push();
translate(shdwPos, shdwPos);
fill(shdwCol);
hoop();
pop();
hoop();
} else {
push();
translate(shdwPos, shdwPos);
fill(shdwCol);
tile();
pop();
tile();
}
}
void hoop() {
noStroke();
pushMatrix();
translate(x, y);
beginShape();
for (float a = 0; a < TAU; a += TAU/360) {
float posX = s/2 * cos(a);
float posY = s/2 * sin(a);
vertex(posX, posY);
}
beginContour();
for (float a = TAU; a > 0; a -= TAU/360) {
float posX = shiftX + r * cos(a);
float posY = shiftY + r * sin(a);
vertex(posX, posY);
}
endContour();
endShape();
popMatrix();
points.add(new PVector(x + shiftX, y + shiftY));
}
void tile() {
noStroke();
pushMatrix();
translate(x, y);
float w = s / tileCount;
for (int j = 0; j < tileCount; j+=2) {
for (int i = 0; i < tileCount; i+=2) {
square((i * w) - (w / 2), (j * w) - (w / 2), w);
}
}
for (int j = 1; j < tileCount; j+=2) {
for (int i = 1; i < tileCount; i+=2) {
square((i * w) - (w / 2), (j * w) - (w / 2), w);
}
}
popMatrix();
}
}
主役は円を円でくり抜いた形。
Happy coding!
応援してくださる方!いつでもサポート受け付けてます!