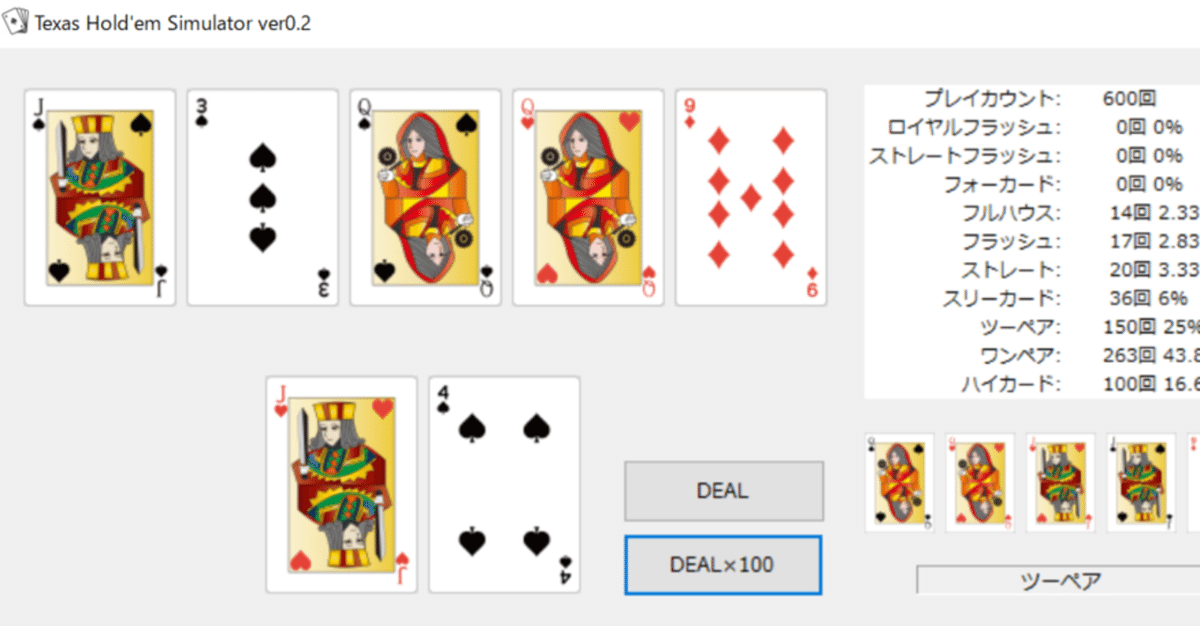
C#ポーカー役判定アルゴリズム
以前、独学でプログラムを組んだことがありまして、めちゃくちゃなコードではありますが、テキサスホールデムポーカーを題材にしたゲームを0から作りたい人はこれを踏み台にすればポーカーアプリ作るのに少しは時間短縮になると思い公開します。
きっと頭の良い人が改良して面白いゲームを作ってくれるはず!
最下部にソースを載せておきますね。こんなので良ければ参考にしてみてください。
(イライラしても責任は負いませんw)
解説
シャッフルと、個人的にめちゃくちゃ苦労した役判定だけ解説しますね。
これはテキサスホールデムを題材にしたアプリです。
カードはJOKER抜きの52枚を使用します。
このアプリではDEALを押すと手札とコミュニティーカード(共通札)が全て開きます。
画面中央に役の成立回数と今回の成立役、
画面右に配られた手札の種類
を表示します。
52枚のトランプは下記の通り表現しています。
1の桁がカードのスートです。(1:スペード 2:ハート 3:ダイア 4:クラブ)
上位2桁はカードの数字です。
(例:123→ダイアのQ)
public class Deck
{
//52枚のデックを構成。
public static int[] deck = new int[52]
{ 11, 21, 31, 41, 51, 61, 71, 81, 91, 101, 111, 121, 131,
12, 22, 32, 42, 52, 62, 72, 82, 92, 102, 112, 122, 132,
13, 23, 33, 43, 53, 63, 73, 83, 93, 103, 113, 123, 133,
14, 24, 34, 44, 54, 64, 74, 84, 94, 104, 114, 124, 134};
}
で、それを時間をシード値にして混ぜます。
public class Dealer
{
//シャッフルメソッド。DEALボタン押下時に1回だけ実行。山札を混ぜます。混ぜたデッキが戻り値です。
public static int[] Shuffle()
{
int seed = Environment.TickCount;
int tmp = 0;
for (int i = 0; i < 52; i++)
{
System.Random r = new System.Random(seed++);
int rnd = r.Next(52);
tmp = Deck.deck[i];
Deck.deck[i] = Deck.deck[rnd];
Deck.deck[rnd] = tmp;
}
return Deck.deck;
}
手札とコミュニティーカード全部で7枚配ります。
//DEALボタン押下時
private void button1_Click(object sender, EventArgs e)
{
label9.Text = "";
int[] judge = new int[7];
int rank = 0;
playcount++;
Clear.ClearCard();
Deck.deck = Dealer.Shuffle();
Dealer.Deal(2);//プリフロップ
pictureBox1.Image = image[0];
pictureBox2.Image = image[1];
rank = Rank.RankHand(Card);
Dealer.Deal(3);//フロップ
pictureBox3.Image = image[2];
pictureBox4.Image = image[3];
pictureBox5.Image = image[4];
Dealer.Deal(1);//ターン
pictureBox6.Image = image[5];
Dealer.Deal(1);//リバー
pictureBox7.Image = image[6];
judge = Judge.JudgeHand(Card);
役判定は下記の流れで行います。
まずは同じスートが5枚以上あるかをチェックし、フラッシュであるかどうかの判定を行います。
YES:ロイヤルフラッシュ判定→ストレートフラッシュ判定→フラッシュ判定
NO:ストレート判定(A2345だけではなく、10JQKAも考慮する必要があります。)
ストレート判定がNOだった場合ペア系の役判定に突入です。
4カードは簡単ですが、厄介なのは3カード成立時に他のペアがある場合はフルハウスになることです。他にペアが無いか判定する必要があります。
そして判定は2ペア、1ペア、ハイカードと続いていきます。
public class Judge
{
static int paircount = 0;
static int suitcount = 0;//スートを数えます。5以上でフラッシュ。フラッシュ判定用。
readonly static int[] hand = new int[6] { 0, 0, 0, 0, 0, 0 };//役とベストハンド。戻り値として扱う。hand[5]は役。
//役判定メソッド。役を判定します。
public static int[] JudgeHand(int[] judgehand)
{
for (int i = 0; i < 6; i++)//役、ベストハンド配列初期化
{
hand[i] = 0;
}
suitcount = 0;
paircount = 0;
if (count >= 5)//グローバル変数 count 配った枚数が5枚以上であればフラッシュ・ストレート判定開始
{
FlushJudge(judgehand);
if (hand[5] == 0)
{
StraightJudge(judgehand);
}
}
if (hand[5] == 0)
PairJudge(judgehand);
if (hand[5] == 0)
{
hand[5] = 10;//ここまで役が該当しなければハイカード確定
Array.Sort(judgehand);
Array.Reverse(judgehand);
int cnt = 0;
if (judgehand[6] / 10 == 1)
{
hand[0] = judgehand[6];
cnt++;
}
for (int i = cnt; i < 5; i++)
{
hand[i] = judgehand[i];
}
}
return hand;//戻り値はhand[0-4]がベストハンドhand[5]が役
}
//フラッシュ役判定。各スートをシーケンシャルで判定
public static int[] FlushJudge(int[] judgehand)
{
int tmp = 0;//ソート用一時的な変数
int bestcount = 0;
int[] flushhand = new int[7] { 999, 999, 999, 999, 999, 999, 999 }; //フラッシュ判定格納用
for (int i = 1; i <= 4; i++)//スペード・ハート・ダイア・クラブを順にiに代入
{
suitcount = 0;
for (int j = 0; j < count; j++)//配られた枚数分スートを確認
{
if (judgehand[j] % 10 == i)//判定用配列よりスートを抽出し、現在参照しているスートと同じか判定
{
flushhand[suitcount] = judgehand[j];//フラッシュ判定用配列に数値を格納
suitcount++;//同スートカウントをインクリメント
}
}
if (suitcount >= 5)//同スートが5枚以上でフラッシュフラグを立てる。スートに対応する数字をフラグに格納
{
hand[5] = 5;//役をフラッシュにセット。
Array.Sort(flushhand);//フラッシュのスートを昇順に並び替え
//ロイヤルフラッシュ判定
if (flushhand[0] / 10 == 1)
for (int cnt = 0; cnt <= 2; cnt++)
{
if (flushhand[1 + cnt] / 10 == 10)
if (flushhand[2 + cnt] / 10 == 11)
if (flushhand[3 + cnt] / 10 == 12)
if (flushhand[4 + cnt] / 10 == 13)
{
tmp = flushhand[0];
for (int m = 0; m < 4; m++)
{
hand[m] = flushhand[cnt + m + 1];
}
hand[4] = tmp;//Aを末尾に格納
hand[5] = 1;//役はロイヤルフラッシュ
}
}
//ストレートフラッシュ判定
if (hand[5] != 1)//ロイヤルフラッシュじゃなければ判定開始
{
for (int cnt = 0; cnt < suitcount - 4; cnt++)//昇順ソートしたフラッシュ配列を始点から+4枚目までを確認し、5連番であればストレートフラッシュ。
{
if (flushhand[cnt] / 10 == (flushhand[cnt + 1] / 10) - 1)
if (flushhand[cnt] / 10 == flushhand[cnt + 2] / 10 - 2)
if (flushhand[cnt] / 10 == flushhand[cnt + 3] / 10 - 3)
if (flushhand[cnt] / 10 == flushhand[cnt + 4] / 10 - 4)
{
for (int m = cnt; m < 5 + cnt; m++)
{
hand[m] = flushhand[m + cnt];
}
hand[5] = 2;
}
}
}
if (hand[5] == 5)//フラッシュ
{
Array.Sort(flushhand);
Array.Reverse(flushhand);//配列をひっくり返して降順に
if (flushhand[6] / 10 == 1)//Aハイフラッシュかどうかを判定
{
hand[0] = flushhand[6];
bestcount++;
}
for (int cnt = 0; cnt < 7; cnt++)
{
if (flushhand[cnt] != 999)
{
hand[bestcount] = flushhand[cnt];
bestcount++;
}
if (bestcount == 5)
break;
}
}
if (hand[5] != 0)
break;//フラッシュフラグが立ったらループを抜ける。他のスートは見ない。
}
}
return hand;
}
//ストレート判定
public static int[] StraightJudge(int[] judgehand)
{
int[] numcount = new int[13] { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
int bestcount = 0;
for (int cnt = 0; cnt < 7; cnt++)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (judgehand[cnt] / 10 == i + 1) //数字毎に枚数をカウント
{
numcount[i]++;
}
}
}
if (numcount[0] > 0)//Aハイストレート判定
{
if (numcount[12] > 0)
if (numcount[11] > 0)
if (numcount[10] > 0)
if (numcount[9] > 0)
{
hand[5] = 6;
for (int i = 9; i < 13; i++)
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
break;
}
}
if (bestcount == 5)
break;
}
Array.Sort(judgehand);//昇順ソートでjudgehand[0]にAを格納
hand[bestcount] = judgehand[0];//Aをhand[0]に格納
bestcount++;
}
}
if (hand[5] == 0)
{
for (int i = 12; i >= 4; i--)
{
bestcount = 0;
if (numcount[i] > 0)
if (numcount[i - 1] > 0)
if (numcount[i - 2] > 0)
if (numcount[i - 3] > 0)
if (numcount[i - 4] > 0)
{
hand[5] = 6;
for (int end = i; end >= i - 4; end--)
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == end + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
break;
}
}
if (bestcount == 5)
break;
}
}
}
}
return hand;
}
//1ペア・2ペア・3カード・4カード・フルハウス判定
public static int[] PairJudge(int[] judgehand)
{
int[] numcount = new int[13] { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
int bestcount = 0;
for (int cnt = 0; cnt < 7; cnt++)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (judgehand[cnt] / 10 == i + 1) //数字毎に枚数をカウント
{
numcount[i]++;
}
}
}
if (numcount[0] == 4)//Aの4カードが含まれるなら
{
hand[5] = 3;//4カード確定
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
if (hand[5] == 0)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (numcount[i] == 4)//同じ札が4枚あるか
{
hand[5] = 3;//フォーカード確定
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列に4カードの札を格納
bestcount++;
}
}
break;
}
}
}
if (hand[5] != 0)
{
if (numcount[0] != 4)//Aの4カードじゃなければ
{
if (numcount[0] > 0)
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列にAを格納
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
if (bestcount < 5)//上の処理をしていなければ
{
for (int m = 12; m >= 0; m--)//キッカー判定。一番大きい数字かつ4カード以外の数字をnumcount1以上の数字から探索
{
if (numcount[m] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != m + 1)//最大の数字が4カードの数字と同じじゃなければ5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == m + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
break;
}
}
}
if (bestcount == 5)
break;
}
}
}
}
//フルハウス・3カード判定
if (hand[5] == 0)
{
if (numcount[0] == 3)//Aの3カードなら
{
hand[5] = 7;//3カードフラグ
for (int j = 0; j < count; j++)
{
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
}
if (hand[5] == 0)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (numcount[i] == 3)
{
hand[5] = 7;//3カードフラグ
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列に3カードの札を格納
bestcount++;
}
}
if (bestcount == 3)
break;
}
}
}
if (hand[5] == 7)//3カードフラグは立っているか
{
if (numcount[0] == 2)//Aのペアが含まれるなら
{
hand[5] = 4;//3カードからフルハウスにランクアップ
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//3カードの数字がAじゃなければ4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
}
if (bestcount != 5)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (bestcount == 5)
break;
if (hand[0] / 10 != i + 1)//最大の数字が3カードの数字と同じじゃなければペアの札を見る(便宜上配列の一番最初[0]を参照)
if (numcount[i] >= 2)
{
for (int j = 0; j < count; j++)
{
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列にペアの札を格納
bestcount++;
}
}
}
hand[5] = 4;//3カードからフルハウスにランクアップ
break;
}
}
}
if (hand[5] == 7)//3カードキッカー判定
{
if (numcount[0] > 0)//Aが1枚でも含まれていればAキッカー判定
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//3カードの数字がAじゃなければ4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
for (int i = 12; i >= 0; i--)//キッカー判定。一番大きい数字かつ3カード以外の数字をnumcount1以上の数字から探索
{
if (bestcount == 5)
break;
if (numcount[i] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの4,5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != i + 1)//最大の数字が3カードの数字と同じじゃなければ4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
}
}
}
}
if (hand[5] == 0)//2ペア・1ペア判定
{
if (numcount[0] == 2)//Aのペアなら
{
paircount++;
for (int j = 0; j < count; j++)
{
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
}
for (int i = 12; i >= 1; i--)//高い数字からシーケンシャル探索
{
if (numcount[i] == 2)
{
paircount++;
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列にペアの札を格納
bestcount++;
}
}
if (paircount == 2)
break;
}
}
}
switch (paircount)
{
case 1:
hand[5] = 9;//1ペア確定
if (numcount[0] > 0)//Aが1枚でも含まれていればAキッカー判定
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//ペアの数字がAじゃなければ3,4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
for (int i = 12; i >= 0; i--)//キッカー判定。一番大きい数字かつペア以外の数字をnumcount1以上の数字から探索
{
if (bestcount == 5)
break;
if (numcount[i] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの3,4,5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != i + 1)//最大の数字がペアの数字と同じじゃなければ3,4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
}
break;
case 2:
hand[5] = 8;//2ペア確定
if (numcount[0] > 0)//Aが1枚でも含まれていればAキッカー判定
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//ペアの数字がAじゃなければ5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
if (bestcount != 5)//5枚格納されていなければ以下処理を行う
for (int i = 12; i >= 0; i--)//キッカー判定。一番大きい数字かつペア以外の数字をnumcount1以上の数字から探索
{
if (bestcount == 5)
break;
if (numcount[i] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != i + 1)//最大の数字がペアの数字と同じじゃなければ5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (hand[2] / 10 != i + 1)//最大の数字がペアの数字と同じじゃなければ5枚目に格納(便宜上2ペアの数字(配列の3番目)を参照)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
}
}
break;
}
}
return hand;
}
}
ただただポーカーが好きな素人が組んだ物なので、考え方だけ参考にしていただけたらと思います。
微力ながら、誰かの助けになれれば幸いです!
全ソース
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
Deck.deck = Dealer.Shuffle();
}
static int playcount = 0;//DEALした回数。役や手札の確率を求める際に使用
static int count;//配られたのは何枚目か
static int[] Card = new int[7];//配列0,1が手札。2~6は共通札。
static Image[] image = new Image[7];//0~1が手札、2~6がボード画像
static Image[] resultimage = new Image[5];//役判定後の5枚を表示する
static double[] handcount = new double[10];//出た役の回数
static double[] handpro = new double[10];//出た役の確率。playcount/handcountで算出
static double[] rankcount = new double[17];//出た手札(スーテッド等)の回数
static double[] rankpro = new double[17];//出た手役の確率。playcount/rankcountで算出
static double pocketcount = 0;
static double pocketpro = 0;
//DEALボタン押下時
private void button1_Click(object sender, EventArgs e)
{
label9.Text = "";
int[] judge = new int[7];
int rank = 0;
playcount++;
Clear.ClearCard();
Deck.deck = Dealer.Shuffle();
Dealer.Deal(2);//プリフロップ
pictureBox1.Image = image[0];
pictureBox2.Image = image[1];
rank = Rank.RankHand(Card);
Dealer.Deal(3);//フロップ
pictureBox3.Image = image[2];
pictureBox4.Image = image[3];
pictureBox5.Image = image[4];
Dealer.Deal(1);//ターン
pictureBox6.Image = image[5];
Dealer.Deal(1);//リバー
pictureBox7.Image = image[6];
judge = Judge.JudgeHand(Card);
RankCounter.RankCount(rank);
if (rank <= 13)
{
pocketcount++;
pocketpro = Math.Round(pocketcount / playcount * 100, 2, MidpointRounding.AwayFromZero);
}
HandCounter.HandCount(judge[5]);
label11.Text = string.Format(" プレイカウント:{0,9}回", playcount);
label10.Text = string.Format(" ロイヤルフラッシュ:{0,9}回 {10}%\r" +
"ストレートフラッシュ:{1,9}回 {11}%\r" +
" フォーカード:{2,9}回 {12}%\r" +
" フルハウス:{3,9}回 {13}%\r" +
" フラッシュ:{4,9}回 {14}%\r" +
" ストレート:{5,9}回 {15}%\r" +
" スリーカード:{6,9}回 {16}%\r" +
" ツーペア:{7,9}回 {17}%\r" +
" ワンペア:{8,9}回 {18}%\r" +
" ハイカード:{9,9}回 {19}%\r"
, handcount[0], handcount[1], handcount[2], handcount[3], handcount[4],
handcount[5], handcount[6], handcount[7], handcount[8], handcount[9],
handpro[0], handpro[1], handpro[2], handpro[3], handpro[4], handpro[5],
handpro[6], handpro[7], handpro[8], handpro[9]);
label12.Text = string.Format(
" AA:{0,9}回 {17}%\r" +
" KK:{1,9}回 {18}%\r" +
" QQ:{2,9}回 {19}%\r" +
" JJ:{3,9}回 {20}%\r" +
" TT:{4,9}回 {21}%\r" +
" 99:{5,9}回 {22}%\r" +
" 88:{6,9}回 {23}%\r" +
" 77:{7,9}回 {24}%\r" +
" 66:{8,9}回 {25}%\r" +
" 55:{9,9}回 {26}%\r" +
" 44:{10,9}回 {27}%\r" +
" 33:{11,9}回 {28}%\r" +
" 22:{12,9}回 {29}%\r" +
" ポケットペア:{34,9}回 {35}%\r" +
" スーテッドコネクタ:{13,9}回 {30}%\r" +
" スーテッド:{14,9}回 {31}%\r" +
" コネクタ:{15,9}回 {32}%\r" +
" その他:{16,9}回 {33}%\r"
, rankcount[0], rankcount[12], rankcount[11], rankcount[10], rankcount[9],
rankcount[8], rankcount[7], rankcount[6], rankcount[5], rankcount[4],
rankcount[3], rankcount[2], rankcount[1], rankcount[13], rankcount[14],
rankcount[15], rankcount[16],
rankpro[0], rankpro[12], rankpro[11], rankpro[10], rankpro[9], rankpro[8], rankpro[7], rankpro[6], rankpro[5],
rankpro[4], rankpro[3], rankpro[2], rankpro[1], rankpro[13], rankpro[14], rankpro[15], rankpro[16], pocketcount, pocketpro);
for (int i = 0; i < judge.Length - 1; i++)
{
Display.CardDisplay(judge[i],i);
}
pictureBox8.Image = resultimage[0];
pictureBox9.Image = resultimage[1];
pictureBox10.Image = resultimage[2];
pictureBox11.Image = resultimage[3];
pictureBox12.Image = resultimage[4];
label9.Text = Display.HandDisplay(judge[5]);
}
//DEAL×100ボタン押下時
private void button2_Click(object sender, EventArgs e)
{
label9.Text = "";
int[] judge = new int[7];
int rank = 0;
for (int cnt = 0; cnt < 100; cnt++)
{
playcount++;
Clear.ClearCard();
Deck.deck = Dealer.Shuffle();
Dealer.Deal(2);//プリフロップ
pictureBox1.Image = image[0];
pictureBox2.Image = image[1];
rank = Rank.RankHand(Card);
Dealer.Deal(3);//フロップ
pictureBox3.Image = image[2];
pictureBox4.Image = image[3];
pictureBox5.Image = image[4];
Dealer.Deal(1);//ターン
pictureBox6.Image = image[5];
Dealer.Deal(1);//リバー
pictureBox7.Image = image[6];
judge = Judge.JudgeHand(Card);
RankCounter.RankCount(rank);
if (rank <= 13)
{
pocketcount++;
pocketpro = Math.Round(pocketcount / playcount * 100, 2, MidpointRounding.AwayFromZero);
}
HandCounter.HandCount(judge[5]);
}
label11.Text = string.Format(" プレイカウント:{0,9}回", playcount);
label10.Text = string.Format(" ロイヤルフラッシュ:{0,9}回 {10}%\r" +
"ストレートフラッシュ:{1,9}回 {11}%\r" +
" フォーカード:{2,9}回 {12}%\r" +
" フルハウス:{3,9}回 {13}%\r" +
" フラッシュ:{4,9}回 {14}%\r" +
" ストレート:{5,9}回 {15}%\r" +
" スリーカード:{6,9}回 {16}%\r" +
" ツーペア:{7,9}回 {17}%\r" +
" ワンペア:{8,9}回 {18}%\r" +
" ハイカード:{9,9}回 {19}%\r"
, handcount[0], handcount[1], handcount[2], handcount[3], handcount[4],
handcount[5], handcount[6], handcount[7], handcount[8], handcount[9],
handpro[0], handpro[1], handpro[2], handpro[3], handpro[4], handpro[5],
handpro[6], handpro[7], handpro[8], handpro[9]);
label12.Text = string.Format(
" AA:{0,9}回 {17}%\r" +
" KK:{1,9}回 {18}%\r" +
" QQ:{2,9}回 {19}%\r" +
" JJ:{3,9}回 {20}%\r" +
" TT:{4,9}回 {21}%\r" +
" 99:{5,9}回 {22}%\r" +
" 88:{6,9}回 {23}%\r" +
" 77:{7,9}回 {24}%\r" +
" 66:{8,9}回 {25}%\r" +
" 55:{9,9}回 {26}%\r" +
" 44:{10,9}回 {27}%\r" +
" 33:{11,9}回 {28}%\r" +
" 22:{12,9}回 {29}%\r" +
" ポケットペア:{34,9}回 {35}%\r" +
" スーテッドコネクタ:{13,9}回 {30}%\r" +
" スーテッド:{14,9}回 {31}%\r" +
" コネクタ:{15,9}回 {32}%\r" +
" その他:{16,9}回 {33}%\r"
, rankcount[0], rankcount[12], rankcount[11], rankcount[10], rankcount[9],
rankcount[8], rankcount[7], rankcount[6], rankcount[5], rankcount[4],
rankcount[3], rankcount[2], rankcount[1], rankcount[13], rankcount[14],
rankcount[15], rankcount[16],
rankpro[0], rankpro[12], rankpro[11], rankpro[10], rankpro[9], rankpro[8], rankpro[7], rankpro[6], rankpro[5],
rankpro[4], rankpro[3], rankpro[2], rankpro[1], rankpro[13], rankpro[14], rankpro[15], rankpro[16], pocketcount, pocketpro);
for (int i = 0; i < judge.Length - 1; i++)
{
Display.CardDisplay(judge[i], i);
}
pictureBox8.Image = resultimage[0];
pictureBox9.Image = resultimage[1];
pictureBox10.Image = resultimage[2];
pictureBox11.Image = resultimage[3];
pictureBox12.Image = resultimage[4];
label9.Text = Display.HandDisplay(judge[5]);
}
public class Clear
{
//カード初期化
public static void ClearCard()
{
count = 0;
for (int i = 0; i < 7; i++)
{
Card[i] = 0;
image[i] = null;
}
}
}
public class Deck
{
//52枚のデックを構成。1の位がスート 1:スペード 2:ハート 3:ダイア 4:クラブ 上位2桁は数字。
public static int[] deck = new int[52]
{ 11, 21, 31, 41, 51, 61, 71, 81, 91, 101, 111, 121, 131,
12, 22, 32, 42, 52, 62, 72, 82, 92, 102, 112, 122, 132,
13, 23, 33, 43, 53, 63, 73, 83, 93, 103, 113, 123, 133,
14, 24, 34, 44, 54, 64, 74, 84, 94, 104, 114, 124, 134};
}
public class Dealer
{
//シャッフルメソッド。DEALボタン押下時に1回だけ実行。山札を混ぜます。混ぜたデッキが戻り値です。
public static int[] Shuffle()
{
int seed = Environment.TickCount;
int tmp = 0;
for (int i = 0; i < 52; i++)
{
System.Random r = new System.Random(seed++);
int rnd = r.Next(52);
tmp = Deck.deck[i];
Deck.deck[i] = Deck.deck[rnd];
Deck.deck[rnd] = tmp;
}
return Deck.deck;
}
//カードを引数で得た数字分配ります
public static void Deal(int DealCount)
{
for (int i = 0; i < DealCount; i++)
{
Card[count] = Deck.deck[count];//デッキから1枚手札orボードに配る
//カード表示用配列imageにresourcesから得た画像を格納
switch (Card[count])
{
case 11:
{
image[count] = Properties.Resources.s01;
break;
}
case 21:
{
image[count] = Properties.Resources.s02;
break;
}
case 31:
{
image[count] = Properties.Resources.s03;
break;
}
case 41:
{
image[count] = Properties.Resources.s04;
break;
}
case 51:
{
image[count] = Properties.Resources.s05;
break;
}
case 61:
{
image[count] = Properties.Resources.s06;
break;
}
case 71:
{
image[count] = Properties.Resources.s07;
break;
}
case 81:
{
image[count] = Properties.Resources.s08;
break;
}
case 91:
{
image[count] = Properties.Resources.s09;
break;
}
case 101:
{
image[count] = Properties.Resources.s10;
break;
}
case 111:
{
image[count] = Properties.Resources.s11;
break;
}
case 121:
{
image[count] = Properties.Resources.s12;
break;
}
case 131:
{
image[count] = Properties.Resources.s13;
break;
}
case 12:
{
image[count] = Properties.Resources.h01;
break;
}
case 22:
{
image[count] = Properties.Resources.h02;
break;
}
case 32:
{
image[count] = Properties.Resources.h03;
break;
}
case 42:
{
image[count] = Properties.Resources.h04;
break;
}
case 52:
{
image[count] = Properties.Resources.h05;
break;
}
case 62:
{
image[count] = Properties.Resources.h06;
break;
}
case 72:
{
image[count] = Properties.Resources.h07;
break;
}
case 82:
{
image[count] = Properties.Resources.h08;
break;
}
case 92:
{
image[count] = Properties.Resources.h09;
break;
}
case 102:
{
image[count] = Properties.Resources.h10;
break;
}
case 112:
{
image[count] = Properties.Resources.h11;
break;
}
case 122:
{
image[count] = Properties.Resources.h12;
break;
}
case 132:
{
image[count] = Properties.Resources.h13;
break;
}
case 13:
{
image[count] = Properties.Resources.d01;
break;
}
case 23:
{
image[count] = Properties.Resources.d02;
break;
}
case 33:
{
image[count] = Properties.Resources.d03;
break;
}
case 43:
{
image[count] = Properties.Resources.d04;
break;
}
case 53:
{
image[count] = Properties.Resources.d05;
break;
}
case 63:
{
image[count] = Properties.Resources.d06;
break;
}
case 73:
{
image[count] = Properties.Resources.d07;
break;
}
case 83:
{
image[count] = Properties.Resources.d08;
break;
}
case 93:
{
image[count] = Properties.Resources.d09;
break;
}
case 103:
{
image[count] = Properties.Resources.d10;
break;
}
case 113:
{
image[count] = Properties.Resources.d11;
break;
}
case 123:
{
image[count] = Properties.Resources.d12;
break;
}
case 133:
{
image[count] = Properties.Resources.d13;
break;
}
case 14:
{
image[count] = Properties.Resources.c01;
break;
}
case 24:
{
image[count] = Properties.Resources.c02;
break;
}
case 34:
{
image[count] = Properties.Resources.c03;
break;
}
case 44:
{
image[count] = Properties.Resources.c04;
break;
}
case 54:
{
image[count] = Properties.Resources.c05;
break;
}
case 64:
{
image[count] = Properties.Resources.c06;
break;
}
case 74:
{
image[count] = Properties.Resources.c07;
break;
}
case 84:
{
image[count] = Properties.Resources.c08;
break;
}
case 94:
{
image[count] = Properties.Resources.c09;
break;
}
case 104:
{
image[count] = Properties.Resources.c10;
break;
}
case 114:
{
image[count] = Properties.Resources.c11;
break;
}
case 124:
{
image[count] = Properties.Resources.c12;
break;
}
case 134:
{
image[count] = Properties.Resources.c13;
break;
}
}
count++;
}
}
}
public class HandCounter
{
//役をカウントします。handpro[]には役が出た確率を格納します
public static void HandCount(int hand)
{
switch (hand)
{
case 1:
{
handcount[0]++;
break;
}
case 2:
{
handcount[1]++;
break;
}
case 3:
{
handcount[2]++;
break;
}
case 4:
{
handcount[3]++;
break;
}
case 5:
{
handcount[4]++;
break;
}
case 6:
{
handcount[5]++;
break;
}
case 7:
{
handcount[6]++;
break;
}
case 8:
{
handcount[7]++;
break;
}
case 9:
{
handcount[8]++;
break;
}
case 10:
{
handcount[9]++;
break;
}
}
for (int i = 0; i < 10; i++)
{
handpro[i] = Math.Round(handcount[i] / playcount * 100, 2, MidpointRounding.AwayFromZero);
}
}
}
public class RankCounter//手札の種類をカウントします。rankpro[]には確率を格納します
{
public static void RankCount(int rank)
{
switch (rank)
{
case 1:
{
rankcount[0]++;
break;
}
case 2:
{
rankcount[1]++;
break;
}
case 3:
{
rankcount[2]++;
break;
}
case 4:
{
rankcount[3]++;
break;
}
case 5:
{
rankcount[4]++;
break;
}
case 6:
{
rankcount[5]++;
break;
}
case 7:
{
rankcount[6]++;
break;
}
case 8:
{
rankcount[7]++;
break;
}
case 9:
{
rankcount[8]++;
break;
}
case 10:
{
rankcount[9]++;
break;
}
case 11:
{
rankcount[10]++;
break;
}
case 12:
{
rankcount[11]++;
break;
}
case 13:
{
rankcount[12]++;
break;
}
case 20:
{
rankcount[13]++;
break;
}
case 30:
{
rankcount[14]++;
break;
}
case 40:
{
rankcount[15]++;
break;
}
case 50:
{
rankcount[16]++;
break;
}
}
for (int i = 0; i < 17; i++)
{
rankpro[i] = 0;
rankpro[i] = Math.Round(rankcount[i] / playcount * 100, 2, MidpointRounding.AwayFromZero);
}
}
}
public class Judge
{
static int paircount = 0;
static int suitcount = 0;//スートを数えます。5以上でフラッシュ。フラッシュ判定用。
readonly static int[] hand = new int[6] { 0, 0, 0, 0, 0, 0 };//役とベストハンド。戻り値として扱う。hand[5]は役。
//役判定メソッド。役を判定します。
public static int[] JudgeHand(int[] judgehand)
{
for (int i = 0; i < 6; i++)//役、ベストハンド配列初期化
{
hand[i] = 0;
}
suitcount = 0;
paircount = 0;
if (count >= 5)//グローバル変数 count 配った枚数が5枚以上であればフラッシュ・ストレート判定開始
{
FlushJudge(judgehand);
if (hand[5] == 0)
{
StraightJudge(judgehand);
}
}
if (hand[5] == 0)
PairJudge(judgehand);
if (hand[5] == 0)
{
hand[5] = 10;//ここまで役が該当しなければハイカード確定
Array.Sort(judgehand);
Array.Reverse(judgehand);
int cnt = 0;
if (judgehand[6] / 10 == 1)
{
hand[0] = judgehand[6];
cnt++;
}
for (int i = cnt; i < 5; i++)
{
hand[i] = judgehand[i];
}
}
return hand;//戻り値はhand[0-4]がベストハンドhand[5]が役
}
//フラッシュ役判定。各スートをシーケンシャルで判定
public static int[] FlushJudge(int[] judgehand)
{
int tmp = 0;//ソート用一時的な変数
int bestcount = 0;
int[] flushhand = new int[7] { 999, 999, 999, 999, 999, 999, 999 }; //フラッシュ判定格納用
for (int i = 1; i <= 4; i++)//スペード・ハート・ダイア・クラブを順にiに代入
{
suitcount = 0;
for (int j = 0; j < count; j++)//配られた枚数分スートを確認
{
if (judgehand[j] % 10 == i)//判定用配列よりスートを抽出し、現在参照しているスートと同じか判定
{
flushhand[suitcount] = judgehand[j];//フラッシュ判定用配列に数値を格納
suitcount++;//同スートカウントをインクリメント
}
}
if (suitcount >= 5)//同スートが5枚以上でフラッシュフラグを立てる。スートに対応する数字をフラグに格納
{
hand[5] = 5;//役をフラッシュにセット。
Array.Sort(flushhand);//フラッシュのスートを昇順に並び替え
//ロイヤルフラッシュ判定
if (flushhand[0] / 10 == 1)
for (int cnt = 0; cnt <= 2; cnt++)
{
if (flushhand[1 + cnt] / 10 == 10)
if (flushhand[2 + cnt] / 10 == 11)
if (flushhand[3 + cnt] / 10 == 12)
if (flushhand[4 + cnt] / 10 == 13)
{
tmp = flushhand[0];
for (int m = 0; m < 4; m++)
{
hand[m] = flushhand[cnt + m + 1];
}
hand[4] = tmp;//Aを末尾に格納
hand[5] = 1;//役はロイヤルフラッシュ
}
}
//ストレートフラッシュ判定
if (hand[5] != 1)//ロイヤルフラッシュじゃなければ判定開始
{
for (int cnt = 0; cnt < suitcount - 4; cnt++)//昇順ソートしたフラッシュ配列を始点から+4枚目までを確認し、5連番であればストレートフラッシュ。
{
if (flushhand[cnt] / 10 == (flushhand[cnt + 1] / 10) - 1)
if (flushhand[cnt] / 10 == flushhand[cnt + 2] / 10 - 2)
if (flushhand[cnt] / 10 == flushhand[cnt + 3] / 10 - 3)
if (flushhand[cnt] / 10 == flushhand[cnt + 4] / 10 - 4)
{
for (int m = cnt; m < 5 + cnt; m++)
{
hand[m] = flushhand[m + cnt];
}
hand[5] = 2;
}
}
}
if (hand[5] == 5)//フラッシュ
{
Array.Sort(flushhand);
Array.Reverse(flushhand);//配列をひっくり返して降順に
if (flushhand[6] / 10 == 1)//Aハイフラッシュかどうかを判定
{
hand[0] = flushhand[6];
bestcount++;
}
for (int cnt = 0; cnt < 7; cnt++)
{
if (flushhand[cnt] != 999)
{
hand[bestcount] = flushhand[cnt];
bestcount++;
}
if (bestcount == 5)
break;
}
}
if (hand[5] != 0)
break;//フラッシュフラグが立ったらループを抜ける。他のスートは見ない。
}
}
return hand;
}
//ストレート判定
public static int[] StraightJudge(int[] judgehand)
{
int[] numcount = new int[13] { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
int bestcount = 0;
for (int cnt = 0; cnt < 7; cnt++)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (judgehand[cnt] / 10 == i + 1) //数字毎に枚数をカウント
{
numcount[i]++;
}
}
}
if (numcount[0] > 0)//Aハイストレート判定
{
if (numcount[12] > 0)
if (numcount[11] > 0)
if (numcount[10] > 0)
if (numcount[9] > 0)
{
hand[5] = 6;
for (int i = 9; i < 13; i++)
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
break;
}
}
if (bestcount == 5)
break;
}
Array.Sort(judgehand);//昇順ソートでjudgehand[0]にAを格納
hand[bestcount] = judgehand[0];//Aをhand[0]に格納
bestcount++;
}
}
if (hand[5] == 0)
{
for (int i = 12; i >= 4; i--)
{
bestcount = 0;
if (numcount[i] > 0)
if (numcount[i - 1] > 0)
if (numcount[i - 2] > 0)
if (numcount[i - 3] > 0)
if (numcount[i - 4] > 0)
{
hand[5] = 6;
for (int end = i; end >= i - 4; end--)
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == end + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
break;
}
}
if (bestcount == 5)
break;
}
}
}
}
return hand;
}
//1ペア・2ペア・3カード・4カード・フルハウス判定
public static int[] PairJudge(int[] judgehand)
{
int[] numcount = new int[13] { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
int bestcount = 0;
for (int cnt = 0; cnt < 7; cnt++)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (judgehand[cnt] / 10 == i + 1) //数字毎に枚数をカウント
{
numcount[i]++;
}
}
}
if (numcount[0] == 4)//Aの4カードが含まれるなら
{
hand[5] = 3;//4カード確定
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
if (hand[5] == 0)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (numcount[i] == 4)//同じ札が4枚あるか
{
hand[5] = 3;//フォーカード確定
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列に4カードの札を格納
bestcount++;
}
}
break;
}
}
}
if (hand[5] != 0)
{
if (numcount[0] != 4)//Aの4カードじゃなければ
{
if (numcount[0] > 0)
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列にAを格納
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
if (bestcount < 5)//上の処理をしていなければ
{
for (int m = 12; m >= 0; m--)//キッカー判定。一番大きい数字かつ4カード以外の数字をnumcount1以上の数字から探索
{
if (numcount[m] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != m + 1)//最大の数字が4カードの数字と同じじゃなければ5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == m + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
break;
}
}
}
if (bestcount == 5)
break;
}
}
}
}
//フルハウス・3カード判定
if (hand[5] == 0)
{
if (numcount[0] == 3)//Aの3カードなら
{
hand[5] = 7;//3カードフラグ
for (int j = 0; j < count; j++)
{
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
}
if (hand[5] == 0)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (numcount[i] == 3)
{
hand[5] = 7;//3カードフラグ
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列に3カードの札を格納
bestcount++;
}
}
if (bestcount == 3)
break;
}
}
}
if (hand[5] == 7)//3カードフラグは立っているか
{
if (numcount[0] == 2)//Aのペアが含まれるなら
{
hand[5] = 4;//3カードからフルハウスにランクアップ
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//3カードの数字がAじゃなければ4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
}
if (bestcount != 5)
{
for (int i = 12; i >= 0; i--)//高い数字からシーケンシャル探索
{
if (bestcount == 5)
break;
if (hand[0] / 10 != i + 1)//最大の数字が3カードの数字と同じじゃなければペアの札を見る(便宜上配列の一番最初[0]を参照)
if (numcount[i] >= 2)
{
for (int j = 0; j < count; j++)
{
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列にペアの札を格納
bestcount++;
}
}
}
hand[5] = 4;//3カードからフルハウスにランクアップ
break;
}
}
}
if (hand[5] == 7)//3カードキッカー判定
{
if (numcount[0] > 0)//Aが1枚でも含まれていればAキッカー判定
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//3カードの数字がAじゃなければ4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
for (int i = 12; i >= 0; i--)//キッカー判定。一番大きい数字かつ3カード以外の数字をnumcount1以上の数字から探索
{
if (bestcount == 5)
break;
if (numcount[i] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの4,5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != i + 1)//最大の数字が3カードの数字と同じじゃなければ4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
}
}
}
}
if (hand[5] == 0)//2ペア・1ペア判定
{
if (numcount[0] == 2)//Aのペアなら
{
paircount++;
for (int j = 0; j < count; j++)
{
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
}
for (int i = 12; i >= 1; i--)//高い数字からシーケンシャル探索
{
if (numcount[i] == 2)
{
paircount++;
{
for (int j = 0; j < count; j++)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];//ベストハンド配列にペアの札を格納
bestcount++;
}
}
if (paircount == 2)
break;
}
}
}
switch (paircount)
{
case 1:
hand[5] = 9;//1ペア確定
if (numcount[0] > 0)//Aが1枚でも含まれていればAキッカー判定
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//ペアの数字がAじゃなければ3,4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
for (int i = 12; i >= 0; i--)//キッカー判定。一番大きい数字かつペア以外の数字をnumcount1以上の数字から探索
{
if (bestcount == 5)
break;
if (numcount[i] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの3,4,5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != i + 1)//最大の数字がペアの数字と同じじゃなければ3,4,5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
}
break;
case 2:
hand[5] = 8;//2ペア確定
if (numcount[0] > 0)//Aが1枚でも含まれていればAキッカー判定
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != 1)//ペアの数字がAじゃなければ5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (judgehand[j] / 10 == 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
}
}
if (bestcount != 5)//5枚格納されていなければ以下処理を行う
for (int i = 12; i >= 0; i--)//キッカー判定。一番大きい数字かつペア以外の数字をnumcount1以上の数字から探索
{
if (bestcount == 5)
break;
if (numcount[i] > 0)//大きい数字から探索し1枚でも引っかかったらベストハンドの5枚目に格納
{
for (int j = 0; j < count; j++)
{
if (hand[0] / 10 != i + 1)//最大の数字がペアの数字と同じじゃなければ5枚目に格納(便宜上配列の一番最初[0]を参照)
{
if (hand[2] / 10 != i + 1)//最大の数字がペアの数字と同じじゃなければ5枚目に格納(便宜上2ペアの数字(配列の3番目)を参照)
{
if (judgehand[j] / 10 == i + 1)
{
hand[bestcount] = judgehand[j];
bestcount++;
}
if (bestcount == 5)
break;
}
}
}
}
}
break;
}
}
return hand;
}
}
public class Rank
{
public static int RankHand(int[] judgehand)
{
int rank = 0;
//手札判定(Rank)
//1~13:ポケット
//20:スーテッドコネクタ
//30:スーテッド
//40:コネクタ
//50:その他
if (judgehand[0] / 10 == judgehand[1] / 10)
{
rank = judgehand[0] / 10;//ポケットの数字を格納
}
else
{
if (judgehand[0] / 10 == 1)//手札1枚目がAなら2枚目Kでコネクタ。スートが同じならスーテッドコネクタ。
{
if (judgehand[1] / 10 == 13)
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 20;
}
else
{
rank = 40;
}
}
else
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 30;
}
else
{
rank = 50;
}
}
}
if (rank == 0)
{
if (judgehand[1] / 10 == 1)//手札2枚目がAなら1枚目Kでコネクタ。スートが同じならスーテッドコネクタ。
{
if (judgehand[0] / 10 == 13)
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 20;
}
else
{
rank = 40;
}
}
else
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 30;
}
else
{
rank = 50;
}
}
}
}
if (rank == 0) //ポケットではない・Aが含まれない場合、手札の数字が1差であればコネクタ
{
if (judgehand[0] / 10 + 1 == judgehand[1] / 10)
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 20;
}
else
{
rank = 40;
}
}
if (rank == 0)
{
if (judgehand[0] / 10 == judgehand[1] / 10 + 1)
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 20;
}
else
{
rank = 40;
}
}
}
}
if (rank == 0)
{
if (judgehand[0] % 10 == judgehand[1] % 10)
{
rank = 30;
}
else
{
rank = 50;
}
}
}
return rank;
}
}
public class Display
{
//表示するべきカードをimageへ格納
public static void CardDisplay(int Card,int cnt)
{
switch (Card)
{
case 11:
{
resultimage[cnt] = Properties.Resources.s01;
break;
}
case 21:
{
resultimage[cnt] = Properties.Resources.s02;
break;
}
case 31:
{
resultimage[cnt] = Properties.Resources.s03;
break;
}
case 41:
{
resultimage[cnt] = Properties.Resources.s04;
break;
}
case 51:
{
resultimage[cnt] = Properties.Resources.s05;
break;
}
case 61:
{
resultimage[cnt] = Properties.Resources.s06;
break;
}
case 71:
{
resultimage[cnt] = Properties.Resources.s07;
break;
}
case 81:
{
resultimage[cnt] = Properties.Resources.s08;
break;
}
case 91:
{
resultimage[cnt] = Properties.Resources.s09;
break;
}
case 101:
{
resultimage[cnt] = Properties.Resources.s10;
break;
}
case 111:
{
resultimage[cnt] = Properties.Resources.s11;
break;
}
case 121:
{
resultimage[cnt] = Properties.Resources.s12;
break;
}
case 131:
{
resultimage[cnt] = Properties.Resources.s13;
break;
}
case 12:
{
resultimage[cnt] = Properties.Resources.h01;
break;
}
case 22:
{
resultimage[cnt] = Properties.Resources.h02;
break;
}
case 32:
{
resultimage[cnt] = Properties.Resources.h03;
break;
}
case 42:
{
resultimage[cnt] = Properties.Resources.h04;
break;
}
case 52:
{
resultimage[cnt] = Properties.Resources.h05;
break;
}
case 62:
{
resultimage[cnt] = Properties.Resources.h06;
break;
}
case 72:
{
resultimage[cnt] = Properties.Resources.h07;
break;
}
case 82:
{
resultimage[cnt] = Properties.Resources.h08;
break;
}
case 92:
{
resultimage[cnt] = Properties.Resources.h09;
break;
}
case 102:
{
resultimage[cnt] = Properties.Resources.h10;
break;
}
case 112:
{
resultimage[cnt] = Properties.Resources.h11;
break;
}
case 122:
{
resultimage[cnt] = Properties.Resources.h12;
break;
}
case 132:
{
resultimage[cnt] = Properties.Resources.h13;
break;
}
case 13:
{
resultimage[cnt] = Properties.Resources.d01;
break;
}
case 23:
{
resultimage[cnt] = Properties.Resources.d02;
break;
}
case 33:
{
resultimage[cnt] = Properties.Resources.d03;
break;
}
case 43:
{
resultimage[cnt] = Properties.Resources.d04;
break;
}
case 53:
{
resultimage[cnt] = Properties.Resources.d05;
break;
}
case 63:
{
resultimage[cnt] = Properties.Resources.d06;
break;
}
case 73:
{
resultimage[cnt] = Properties.Resources.d07;
break;
}
case 83:
{
resultimage[cnt] = Properties.Resources.d08;
break;
}
case 93:
{
resultimage[cnt] = Properties.Resources.d09;
break;
}
case 103:
{
resultimage[cnt] = Properties.Resources.d10;
break;
}
case 113:
{
resultimage[cnt] = Properties.Resources.d11;
break;
}
case 123:
{
resultimage[cnt] = Properties.Resources.d12;
break;
}
case 133:
{
resultimage[cnt] = Properties.Resources.d13;
break;
}
case 14:
{
resultimage[cnt] = Properties.Resources.c01;
break;
}
case 24:
{
resultimage[cnt] = Properties.Resources.c02;
break;
}
case 34:
{
resultimage[cnt] = Properties.Resources.c03;
break;
}
case 44:
{
resultimage[cnt] = Properties.Resources.c04;
break;
}
case 54:
{
resultimage[cnt] = Properties.Resources.c05;
break;
}
case 64:
{
resultimage[cnt] = Properties.Resources.c06;
break;
}
case 74:
{
resultimage[cnt] = Properties.Resources.c07;
break;
}
case 84:
{
resultimage[cnt] = Properties.Resources.c08;
break;
}
case 94:
{
resultimage[cnt] = Properties.Resources.c09;
break;
}
case 104:
{
resultimage[cnt] = Properties.Resources.c10;
break;
}
case 114:
{
resultimage[cnt] = Properties.Resources.c11;
break;
}
case 124:
{
resultimage[cnt] = Properties.Resources.c12;
break;
}
case 134:
{
resultimage[cnt] = Properties.Resources.c13;
break;
}
default:
{
break;
}
}
}
//表示するべき役を格納(1ロイヤルフラッシュ~10ハイカード)
public static string HandDisplay(int handresult)
{
string handstr = "";
switch (handresult)
{
case 1:
{
handstr = "ロイヤルフラッシュ";
break;
}
case 2:
{
handstr = "ストレートフラッシュ";
break;
}
case 3:
{
handstr = "フォーカード";
break;
}
case 4:
{
handstr = "フルハウス";
break;
}
case 5:
{
handstr = "フラッシュ";
break;
}
case 6:
{
handstr = "ストレート";
break;
}
case 7:
{
handstr = "スリーカード";
break;
}
case 8:
{
handstr = "ツーペア";
break;
}
case 9:
{
handstr = "ワンペア";
break;
}
case 10:
{
handstr = "ハイカード";
break;
}
}
return handstr;
}
}
}
}
この記事が気に入ったらサポートをしてみませんか?