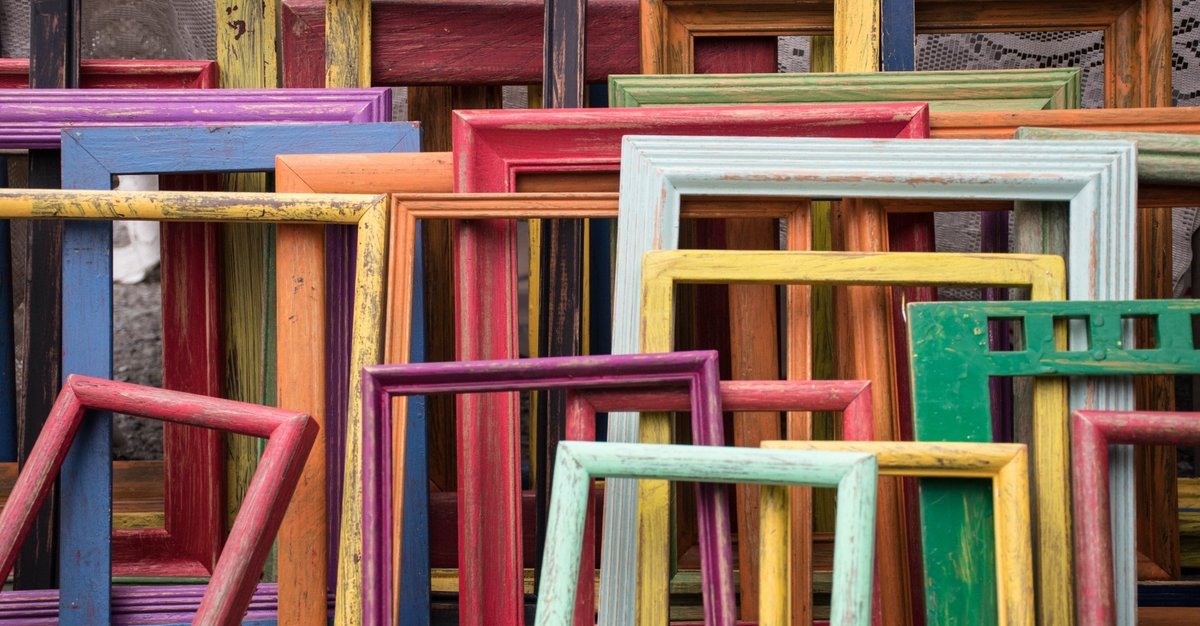
C++(タロット占い)
おはようございます。JavaもPythonも裏ではC++が動いているらしい
JavaとC++の違いについて勉強していきます。
各プログラミング言語
JAVA
・ほとんどのOSで動かすことができる汎用プログラミング言語。
・大企業の業務システムや基幹システム、金融機関の会計システムなど
のWEBアプリ開発にも使われている。
・大手ネットショップや銀行のシステム。
・アクセスが集中する大規模WEBサービスにも適している。
C++
・C言語から派生した汎用プログラミング言語。
・C言語の機能や特徴を継承しながら、オブジェクト指向プログラミング
手続き型プログラミング、データ抽象、ジェネリックプログラミング
などのプログラミングパラダイムを複数組み合わせられています。
・コンパイル型言語です。
・「ポインター」と「オブジェクト指向」が特徴。
・処理速度が速い。
Microsoft Visual Studioで作ってみよう
・新しいプロジェクトの作成
新しいプロジェクトの作成する
C++ Windows コンソール
#include <iostream>
int main()
{
std::cout << "Hello world" << std::endl;
}
Hello world
using namespace std;
これはJavaでいうところのpackageと同じ、名前空間。
C++ではC言語の書き方しても認識してくれる。
➤ローカルWindowsデバッガーで実行する
cout << "Hello C++ World!" << endl;
「cout」は出力(ストリーム出力演算子)
「endl」は改行
#include <iostream>
using namespace std;
int main()
{
cout << "Hello C++ World!" << endl;
cout << 5 << "という数字出力" << endl;
printf("Hello C World\n");
}
cont << ① << ② << endl;
①を出力して②を出力して改行。(前から順番に出力する。)
#include
using namespace std; //namespace名前空間(javaでいうpackageと同じ)
int main()
{
//コンソールに文字を出力
//※<<演算子(通常は美っとシフト演算子)がオーバーロードされている
//ストリーム出力演算子
//前から順に演算(評価)し結果を出す
cout << "Hello C++ World!" << endl;
cout << 5 << "という数字出力" << endl;
//C言語では↓の記述
printf("Hello C World\n");
//printf("%s 文字列を入れる", 5);//やばい
}
cout << 5 << "という数字出力\n" << endl;
👇(std::をつける)
std::cout << 5 << "という数字出力\n" << std::endl;
#include <iostream>
int main()
{
std::cout << "Hello world" << std::endl;
}
Hello world
「std::」をつけると仮想空間になる為、「using namespace std;」がなくてもいける。
#include <iostream>
using namespace std; //namespace名前空間(javaでいうpackageと同じ)
int main()
{
//コンソールに文字を出力
//※<<演算子(通常は美っとシフト演算子)がオーバーロードされている
//ストリーム出力演算子
//前から順に演算(評価)し結果を出す
cout << "Hello C++ World!" << endl;
std::cout << 5 << "という数字出力" << std::endl;
//C言語では↓の記述
printf("Hello C World\n");
//printf("%s 文字列を入れる", 5);//やばい
}
同じ結果になる
#include <iostream>
using namespace std; //namespace名前空間(javaでいうpackageと同じ)
int main()
{
//コンソールに文字を出力
//※<<演算子(通常は美っとシフト演算子)がオーバーロードされている
//ストリーム出力演算子
//前から順に演算(評価)し結果を出す
cout << "Hello C++ World! << endl;
std::cout << 5 << "という数字出力" << std::endl;
//C言語では↓の記述
printf("Hello C World\n");
//printf("%s 文字列を入れる", 5);//やばい
}
オーバーロードされているか検索できる
#include <iostream>
using namespace std; //namespace名前空間(javaでいうpackageと同じ)
int main()
{
//コンソールに文字を出力
//※<<演算子(通常は美っとシフト演算子)がオーバーロードされている
//ストリーム出力演算子
//前から順に演算(評価)し結果を出す
cout << "Hello C++ World!" << endl;
std::cout << 5 << "という数字出力" << std::endl;
int i = 0;
int j = 0;
string text;
//コンソールからデータを入力
cin >> i >> text >> j;
//取得した情報を表示
cout << "1回目は" << i << "2回目は" << j << endl;
}
・ヘッダーファイルを作る
ヘッダーファイルはクラスの宣言・定義をするところ
右クリックで「追加」「新しい項目」
「VisualC++」「ヘッダーファイル」「名前をclass1.hにする」「開く」
「class1.h」ができている。
#pragma once
class Point{
private:
int x;
int y;
public:
int getX() const;
int getY() const;
void setX(int x);
void setY(int y);
};
Pointクラスを作る。メソッドは基本的にはpublic、メンバ変数は基本的にはprivateとする場合が多いらしい。
private:(ここからはプライベートと言う意味)
プライベートでx、yの変数を定義する。
public:(ここからはパブリックだよという意味)
パブリックで、getX()getY()を定数として、setX(),setY()も作る。
#include <iostream>
//class1.hを組み込む
#include "class1.h"
using namespace std;
int Point::getX() const {
return x;
}
int Point::getY() const {
return x;
}
void Point::setX(int _x) {
x = _x;
}
void Point::setY(int _y) {
y = _y;
}
int main()
{
cout << "Hello C++ World!" << endl;
std::cout << 5 << "という数字出力" << std::endl;
int i = 0;
int j = 0;
string text;
//コンソールからデータを入力
cin >> i >> text >> j;
//取得した情報を表示
cout << "1回目は" << i << "2回目は" << j << endl;
//Point型変数を新規作成
Point* p = new Point();
p->setX(i);
p->setY(j);
cout << "" << p->getX()
<< "" << p->getY()
<< endl;
}
まだ完全ではない。借りたものは返さないといけない。
※ 自分で使ったメモリは解放する必要がある。(Javaは自動で開放する)
newしたpを開放する。⬇️
//割り当てられたメモリの開放
delete p;
#include <iostream>
#include "class1.h"
using namespace std;
int Point::getX() const {
return x;
}
int Point::getY() const {
return x;
}
void Point::setX(int _x) {
x = _x;
}
void Point::setY(int _y) {
y = _y;
}
int main()
{
cout << "Hello C++ World!" << endl;
std::cout << 5 << "という数字出力" << std::endl;
int i = 0;
int j = 0;
string text;
//コンソールからデータを入力
cin >> i >> text >> j;
//取得した情報を表示
cout << "1回目は" << i << "2回目は" << j << endl;
//Point型変数を新規作成
Point* p = new Point();
p->setX(i);
p->setY(j);
cout << "" << p->getX()
<< "" << p->getY()
<< endl;
//割り当てられたメモリの開放
delete p;
}
「class1.h 」ヘッダーには継承の仕方が3種類ある。
private:
public:
:private
#pragma once
class Point{
//プライベート①
private:
int x;
int y;
//パブリック②
public:
//メンバ変数
int getX() const;
int getY() const;
void setX(int x);
void setY(int y);
};
//派生型の宣言
class Roctangle
:private Point //プライベート継承③
{
private:
int w; //幅
int h; //高
public:
Roctangle(int x , int y , int w , int h)
:w(w), h(h) //メンバ変数への初期値の代入
{
setX(x);
setY(y);
}
int getLeft() const;
int getRightt() const;
int getTop() const;
int getBottom() const;
};
Pointの方にもポインター変数を設定できる
#pragma once
class Point{
//プライベート①
private:
int x;
int y;
//パブリック②
public:
Point(int x, int y)
:x(x), y(y)
{
}
//メンバ変数
int getX() const;
int getY() const;
void setX(int x);
void setY(int y);
};
//派生型の宣言
class Roctangle
:private Point //プライベート継承③
{
private:
int w; //幅
int h; //高
public:
Roctangle(int x , int y , int w , int h)
:Point(x,y),w(w),h(h)
{
setX(x);
setY(y);
}
int getLeft() const;
int getRight() const;
int getTop() const;
int getBottom() const;
};
#include <iostream>
#include "class1.h"
using namespace std;
int Point::getX() const {
return x;
}
int Point::getY() const {
return x;
}
void Point::setX(int _x) {
x = _x;
}
void Point::setY(int _y) {
y = _y;
}
int Roctangle::getLeft() const {
return getX();
}
int Roctangle::getRight() const {
return getX() + w;
}
int Roctangle::getTop() const {
return getY();
}
int Roctangle::getBottom() const {
return getY() + h;
}
int main()
{
cout << "Hello C++ World!" << endl;
std::cout << 5 << "という数字出力" << std::endl;
int i = 0;
int j = 0;
string text;
//コンソールからデータを入力
cin >> i >> text >> j;
//取得した情報を表示
cout << "1回目は" << i << "2回目は" << j << endl;
Point* p = new Point(i,j);
//p->setX(i);
//p->setY(j);
cout << " x= " << p->getX()
<< " y= " << p->getY()
<< endl;
//割り当てられたメモリの開放
delete p;
//Roctangle型変数の新規作成
Roctangle* r = new Roctangle(10, 25, 100, 10);
cout << "短形型情報の"
<< "左:" << r->getLeft()
<< "右:" << r->getRight()
<< "上:" << r->getTop()
<< "下;" << r->getBottom()
<< endl;
//割り当てられたメモリの開放
delete r;
do {
Roctangle r2(20, 40, 100, 100);
cout << "短形型情報の"
<< "左:" << r2.getLeft()
<< "右:" << r2.getRight()
<< "上:" << r2.getTop()
<< "下;" << r2.getBottom()
<< endl;
} while (true);
}
newしてなければdeleteはいらない
うまく動作してるのかよくわからないけど。また復習するねー。
いいなと思ったら応援しよう!
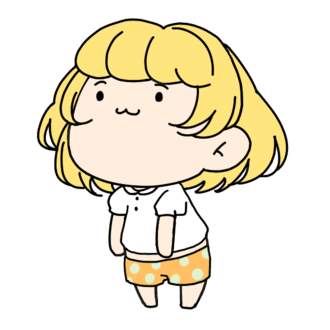