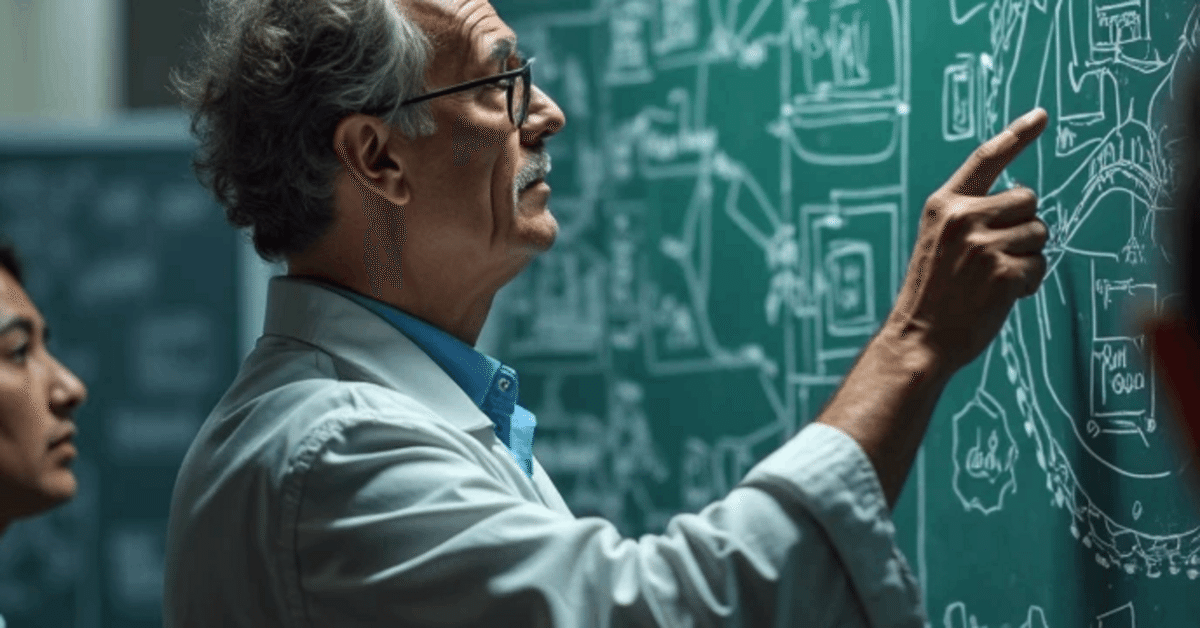
💡数式の解答過程をアニメーション化🤖
数式の解答過程をアニメーション化することは可能です。例えば、二次方程式の解法や微分・積分の計算過程などをステップバイステップでアニメーションGIFにできます。
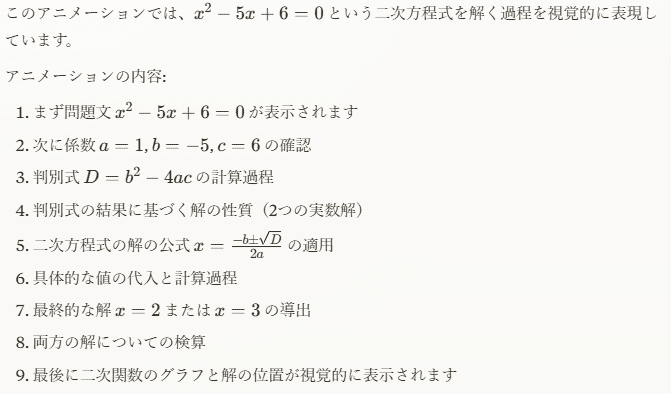
このようなアニメーションは、他の数学的問題(積分、微分、行列計算など)にも応用可能です。例えば、微分の計算過程や、連立方程式の解法なども同様にステップバイステップでアニメーション化できます。
また、より複雑な数式や計算過程も表現可能です。特定の数学の例題があれば、それに合わせたアニメーションを作成することもできます。
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
from matplotlib import rc
from IPython.display import HTML
# LaTeXの設定
rc('text', usetex=False)
rc('font', family='serif')
# アニメーションのフレーム数
n_frames = 10
# 図の初期化
fig, ax = plt.subplots(figsize=(10, 6))
ax.set_axis_off() # 軸を非表示
fig.patch.set_facecolor('#f9f9f9') # 背景色を設定
# タイトルの設定
plt.suptitle('二次方程式の解法: $ax^2 + bx + c = 0$', fontsize=16)
# 解くべき例題(二次方程式)
a, b, c = 1, -5, 6 # x^2 - 5x + 6 = 0
# 解法のステップを定義
steps = [
r"問題: $x^2 - 5x + 6 = 0$ を解け",
r"Step 1: 係数を確認する \\ $a = 1, b = -5, c = 6$",
r"Step 2: 判別式 $D = b^2 - 4ac$ を計算 \\ $D = (-5)^2 - 4 \cdot 1 \cdot 6 = 25 - 24 = 1$",
r"Step 3: $D > 0$ なので、2つの実数解がある",
r"Step 4: 二次方程式の解の公式を適用 \\ $x = \frac{-b \pm \sqrt{D}}{2a}$",
r"Step 5: 値を代入 \\ $x = \frac{5 \pm \sqrt{1}}{2 \cdot 1} = \frac{5 \pm 1}{2}$",
r"Step 6: 2つの解を計算 \\ $x_1 = \frac{5 + 1}{2} = 3$ \\ $x_2 = \frac{5 - 1}{2} = 2$",
r"解答: $x = 2$ または $x = 3$",
r"検算: $x = 2$ の場合 \\ $1 \cdot 2^2 - 5 \cdot 2 + 6 = 4 - 10 + 6 = 0$ ✓",
r"検算: $x = 3$ の場合 \\ $1 \cdot 3^2 - 5 \cdot 3 + 6 = 9 - 15 + 6 = 0$ ✓"
]
# テキストオブジェクトを初期化
text = ax.text(0.5, 0.5, '', ha='center', va='center', fontsize=14,
transform=ax.transAxes, linespacing=2.0)
# グラフを描画する関数(最後のフレームで表示)
def draw_graph(frame):
if frame == n_frames - 1:
# xの範囲を設定
x = np.linspace(0, 5, 1000)
# 二次関数 f(x) = ax^2 + bx + c
y = a * x**2 + b * x + c
# グラフの描画エリアを作成(テキストの下部)
graph_ax = fig.add_axes([0.2, 0.1, 0.6, 0.3])
graph_ax.plot(x, y, 'b-', linewidth=2)
graph_ax.axhline(y=0, color='k', linestyle='-', alpha=0.3)
graph_ax.axvline(x=0, color='k', linestyle='-', alpha=0.3)
# 解の位置をプロット
graph_ax.plot([2, 3], [0, 0], 'ro', markersize=8)
graph_ax.annotate('x = 2', xy=(2, 0), xytext=(2, 5),
arrowprops=dict(facecolor='black', shrink=0.05))
graph_ax.annotate('x = 3', xy=(3, 0), xytext=(3, 5),
arrowprops=dict(facecolor='black', shrink=0.05))
graph_ax.set_title('$f(x) = x^2 - 5x + 6$')
graph_ax.grid(True, alpha=0.3)
# アニメーションの更新関数
def update(frame):
# ステップ数に基づいてフレームをマッピング
step_idx = min(frame, len(steps) - 1)
text.set_text(steps[step_idx])
# 最後のフレームでグラフを表示
if frame == n_frames - 1:
draw_graph(frame)
return text,
# アニメーションの作成
ani = animation.FuncAnimation(fig, update, frames=n_frames, interval=1200, blit=True)
# GIFとして保存
ani.save('equation_solving_animation.gif', writer='pillow', fps=0.8, dpi=100)
print("数式解法のアニメーションGIFが保存されました: equation_solving_animation.gif")
↓別の解法になります。