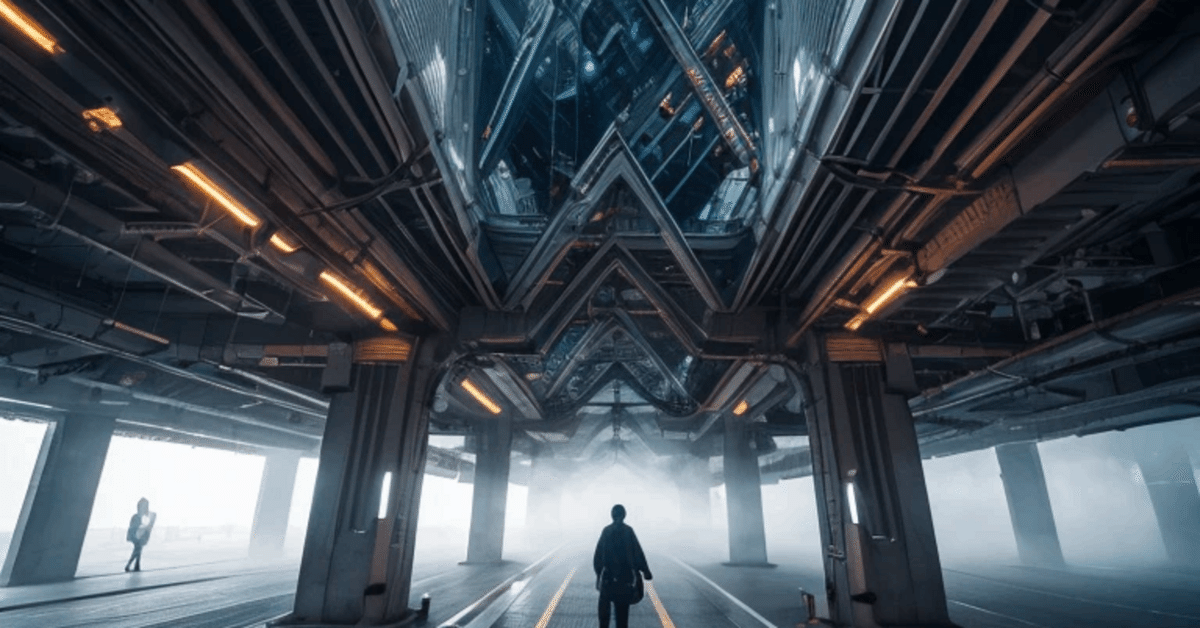
無限構造体の街のプロンプト
いきなりのタイトルですみません。
元々、自分はAmazonで、
・近未来多構造体イラスト集!! Vol.1~3
・近未来光合成都市イラスト集!!
・多重構造体の街 多階層構造体の街
・宇宙図書館: 工学生命体と知の媒介人による情報の集積
と、上記の書籍を出版しております。
生成AIを使用した事も有り、まだまだ改良の余地が有るイラスト集ですが、
今後も精進致します。
近未来多構造体イラスト集!! Vol.1~3
近未来光合成都市イラスト集!!
近未来ベジタリアン・ヴィーガン都市イラスト集!!
多重構造体の街 多階層構造体の街
宇宙図書館: 工学生命体と知の媒介人による情報の集積
今回、無限構造体の街: Python イラスト集ですが、出版する運びとなりました。下図になります。
昔から多重構造体、多階層構造体の表現が好きで、それが乗じてイラスト化しておりました。生成AIの力を借りて何とか制作できております。
色々な漫画家先生の方々から着想を得ておりました。
無限構造体の街の制作にあたり、弐瓶勉先生のBLAME! Noise、吾峠呼世晴先生の映画の次回作、鬼滅の刃 無限城、つくみず先生の少女終末旅行を参考に致しました。兼ねて感謝致します。※リンク先は直リンクになります。私はアフィリエイトはやっておりません。
因みに少女週末旅行は、最初に見たのはアニメのほうからです。なので↑にはDVDも載せておきます。非常に良い出来なので、アニメのほうもおススメです。遅くなりましたが、
ChatGPTに入力するべきプロンプトは、
A futuristic cityscape inspired by a massive, infinite grid-like structure, featuring modular, symmetrical designs with intricate metallic details. The city extends endlessly into the horizon, creating a vanishing point where light bursts through, emphasizing depth and infinity. The architecture showcases geometric patterns, glowing accents, and suspended pathways, with layers of complex structures stacked vertically. The lighting is dramatic, with soft ambient light complemented by glowing highlights in blue and white, casting reflections and shadows that enhance the atmosphere. The perspective draws attention to the endless repetition and vast scale of the city. Created using: high-detail sci-fi design, modular architecture, glowing accents, dynamic perspective, atmospheric mist, geometric patterns, industrial aesthetic, and 4K quality.
↑になります。コピペしてそのまま貼り付けて下さい。それからアレンジはいくらでも可能だと思うので、英語を翻訳しながらでもChatGPTにそれぞれ入力をお願い致します。
無限構造体の街を生成する為のPythonコード
import matplotlib.pyplot as plt
import numpy as np
def generate_infinite_city(grid_size=10, spacing=1.0, layers=3):
fig, ax = plt.subplots(figsize=(10, 10))
ax.set_aspect('equal')
# 配列のグリッドを生成
for layer in range(layers):
layer_spacing = spacing * (1.5 ** layer)
for x in range(-grid_size, grid_size + 1):
for y in range(-grid_size, grid_size + 1):
# 中心点
center = (x * layer_spacing, y * layer_spacing)
# 四角形の建物を描画
square_size = layer_spacing * 0.4
rect = plt.Rectangle(
(center[0] - square_size / 2, center[1] - square_size / 2),
square_size, square_size,
color="steelblue", alpha=0.6 - layer * 0.2
)
ax.add_patch(rect)
# 発光エフェクト
ax.plot(center[0], center[1], 'o', color='cyan', alpha=0.6 - layer * 0.2)
# 軸の範囲と装飾を設定
ax.set_xlim(-grid_size * spacing * 2, grid_size * spacing * 2)
ax.set_ylim(-grid_size * spacing * 2, grid_size * spacing * 2)
ax.axis("off")
plt.title("Infinite Modular Cityscape", fontsize=14, color="white")
plt.gca().set_facecolor("black")
plt.show()
# 無限構造体の街を描画
generate_infinite_city(grid_size=15, spacing=1.5, layers=4)
無限構造体の街の住民が光合成を行う要素
import m無限構造体の街atplotlib.pyplot as plt
import numpy as np
class PhotosyntheticHuman:
def __init__(self, energy=100, efficiency=0.05, consumption_rate=10):
"""
人間の光合成シミュレーション
:param energy: 初期エネルギー (kcal)
:param efficiency: 光合成効率 (光エネルギー -> 食物エネルギーの変換率)
:param consumption_rate: エネルギー消費率 (kcal/h)
"""
self.energy = energy # 初期エネルギー
self.efficiency = efficiency # 光合成効率
self.consumption_rate = consumption_rate # エネルギー消費率
self.energy_history = [] # エネルギーの履歴
def photosynthesize(self, sunlight_intensity, hours):
"""
光合成によるエネルギー生成
:param sunlight_intensity: 太陽光の強度 (W/m^2)
:param hours: 光合成に費やす時間 (h)
"""
sunlight_energy = sunlight_intensity * hours * 3.6 # 光エネルギー (kcal)
generated_energy = sunlight_energy * self.efficiency
self.energy += generated_energy
def consume_energy(self, hours):
"""
活動によるエネルギー消費
:param hours: 活動時間 (h)
"""
consumed_energy = self.consumption_rate * hours
self.energy -= consumed_energy
def simulate_day(self, sunlight_schedule):
"""
1日のエネルギー変動をシミュレーション
:param sunlight_schedule: 時間ごとの太陽光強度 (リスト: W/m^2)
"""
for sunlight_intensity in sunlight_schedule:
self.photosynthesize(sunlight_intensity, 1) # 1時間ごとに光合成
self.consume_energy(1) # 1時間ごとにエネルギー消費
self.energy_history.append(max(self.energy, 0)) # エネルギーが0未満にならないようにする
# シミュレーションパラメータ
sunlight_schedule = [200, 400, 600, 800, 1000, 800, 600, 400, 200, 0, 0, 0, 0, 0, 0, 0, 200, 400, 600, 800, 1000, 800, 600, 400] # 1時間ごとの太陽光強度
human = PhotosyntheticHuman(energy=500, efficiency=0.01, consumption_rate=50) # 初期エネルギー500kcal, 効率1%
# 1日をシミュレート
human.simulate_day(sunlight_schedule)
# 結果の可視化
time = np.arange(24) # 24時間
plt.figure(figsize=(10, 6))
plt.plot(time, human.energy_history, label="Energy Level (kcal)", marker='o')
plt.axhline(0, color='red', linestyle='--', label="Energy Depletion")
plt.title("Photosynthetic Human Energy Levels Over a Day")
plt.xlabel("Time (hours)")
plt.ylabel("Energy (kcal)")
plt.legend()
plt.grid(True)
plt.show()
無限構造体の街に必要な資材をどのように調達し、無限に近い供給をシミュレーションする
import matplotlib.pyplot as plt
import numpy as np
class InfiniteStructureResourceModel:
def __init__(self, initial_resources=1000, recycling_rate=0.8, mining_rate=50, usage_rate=100):
"""
資材モデルの初期化
:param initial_resources: 初期資材量
:param recycling_rate: リサイクル率(0~1)
:param mining_rate: 宇宙資源採掘による資材供給量/ターン
:param usage_rate: 都市建設に必要な資材消費量/ターン
"""
self.resources = initial_resources
self.recycling_rate = recycling_rate
self.mining_rate = mining_rate
self.usage_rate = usage_rate
self.history = []
def simulate_turn(self):
"""
1ターンの資材サイクルをシミュレート
"""
# 資材のリサイクル
recycled = self.usage_rate * self.recycling_rate
# 新規資材の採掘
new_resources = self.mining_rate
# 消費と供給
self.resources += recycled + new_resources - self.usage_rate
# 資材量を記録
self.history.append(self.resources)
def simulate(self, turns=100):
"""
複数ターンのシミュレーション
:param turns: シミュレーションするターン数
"""
for _ in range(turns):
self.simulate_turn()
def plot_results(self):
"""
結果をプロット
"""
plt.figure(figsize=(10, 6))
plt.plot(self.history, label="Resources Available")
plt.axhline(0, color="red", linestyle="--", label="Depletion Line")
plt.title("Resource Dynamics in an Infinite Structure City")
plt.xlabel("Turns")
plt.ylabel("Resources")
plt.legend()
plt.grid()
plt.show()
# モデルパラメータ
initial_resources = 1000 # 初期資材量
recycling_rate = 0.85 # リサイクル率(85%)
mining_rate = 150 # 宇宙資源採掘による資材供給
usage_rate = 200 # 都市建設による消費
# モデルの初期化とシミュレーション
model = InfiniteStructureResourceModel(
initial_resources=initial_resources,
recycling_rate=recycling_rate,
mining_rate=mining_rate,
usage_rate=usage_rate
)
# シミュレーション実行
model.simulate(turns=200)
# 結果をプロット
model.plot_results()
コードの説明
InfiniteStructureResourceModel クラス
資材供給と消費を管理します。
初期資材、リサイクル率、宇宙採掘率、消費量を設定。
資材のリサイクルと採掘
消費資材の一定割合をリサイクル。
採掘による新規資材を加算。
資材量のシミュレーション
simulate() メソッドで、指定ターン数の資材量の変動を計算。
結果の可視化
時間経過に伴う資材量の変化をグラフ化。
資材が枯渇しないかどうかを確認。
実行結果の例:
資材が枯渇しない場合
リサイクル率や採掘量が消費量を上回っているため、資材は安定。
資材が枯渇する場合
リサイクル率や採掘量が低いと資材が枯渇し、無限構造体の建設が維持できなくなる。
カスタマイズポイント
リサイクル率
環境技術の進化を考慮して変動させる。
採掘量
宇宙資源の供給量を調整することで持続可能性を模索。
消費量
都市の拡張スピードや人口増加を反映して調整。
このモデルを使えば、無限構造体の街の資材供給を現実的な範囲でシミュレーション可能です。