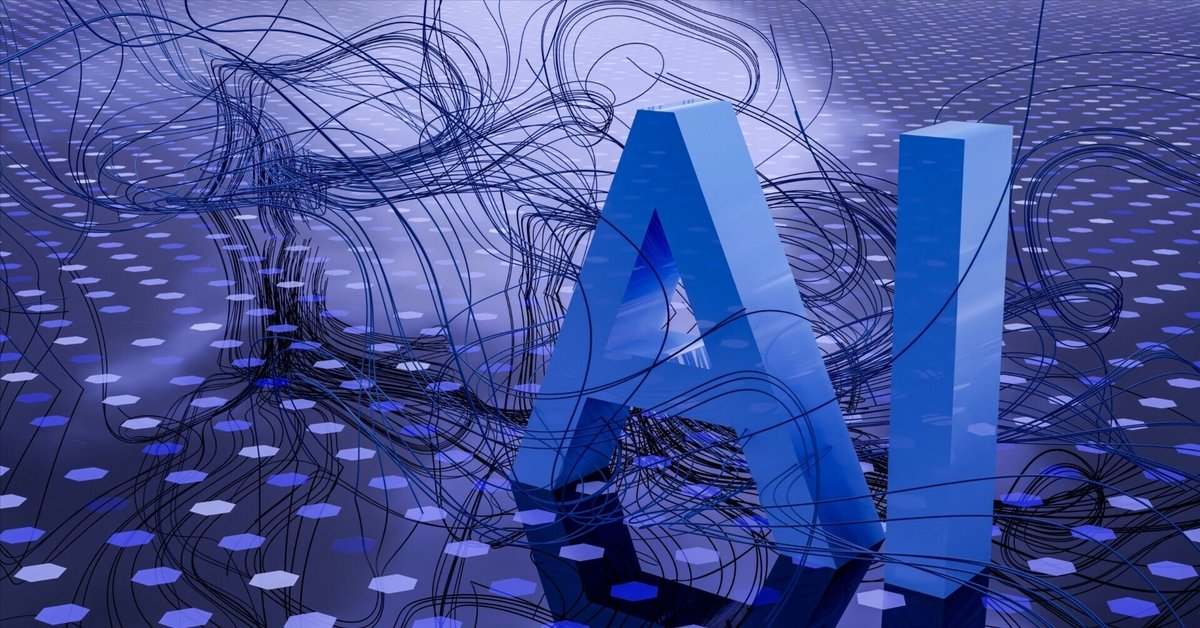
Pythonでエントリーシートに記載されている頻出単語を集計・分析するスクリプト
AIって単語を聞かない日がなくなった
最近、どんなことに生成AIを使ったら、自分の仕事が効率的になるかなぁと考えています。
特に人事のお仕事では、求人票作成なんかは生成AIで書くようになるのだろうなぁと….。その時、ふと思いついたのが、自社に関心を寄せてくれて、なおかつエントリーシートまで書いてくれた人がどんなキーワードを使用しているか、頻出単語を調べられたら面白そうだと思い、Pythonで実装してみました。
最終的には、頻出単語を使用した求人票をChat GPTに書いてもらうというのがゴールになります。SEO対策なんかも加味してくれるとVery Goodだなと。
実行環境
Windows 11
Visual Studio Code
Pythonスクリプト 頻出単語の集計・吐き出し
import os
import pandas as pd
import matplotlib.pyplot as plt
import glob
from janome.tokenizer import Tokenizer
from collections import Counter
# 名詞のみを抽出する関数を定義
def extract_nouns(text):
nouns = [token.surface for token in Tokenizer().tokenize(text) if '名詞' in token.part_of_speech]
print(f"{nouns}\n")
return nouns
entrysheet_file_path = r'c:/Users/user/Desktop/**********/entrysheet.csv'
df = pd.read_csv(entrysheet_file_path)
print(df.columns)
target_columns = ['志望理由をお答えください', '会社選びの軸について']
df_selected = df[target_columns]
# 空白行を削除する
df_selected = df_selected.dropna(how='any')
print(df_selected)
print(len(df_selected))
print(df_selected.tail())
nouns_list = []
for index, row in df_selected.iterrows():
for column in df_selected.columns:
text = row[column]
nouns_list.extend(extract_nouns(text))
print(f"Index: {index}, Column: {column}\n")
print(f"{text}\n\n")
# 頻出単語を集計
counter = Counter(nouns_list)
# 頻出単語を降順でソート
sorted_counter = sorted(counter.items(), key=lambda x: x[1], reverse=True)
# 頻出単語をDataFrameに変換
df_counter = pd.DataFrame(sorted_counter, columns=['word', 'count'])
# CSVに出力
results_file_path = r'c:\Users\user\Desktop\*********\frequent_words.csv'
df_counter.to_csv(results_file_path, index=False)
Chat GPT部分
OPEN_AI_KEYの部分は、KEYの取得と環境変数への登録が必要です。
import os
from openai import OpenAI
from datetime import datetime
client = OpenAI(
api_key=os.environ.get('OPENAI_API_KEY'),
)
# Chat GPTのAPIを呼び出す
def call_chatgpt(prompt, debug=False):
chat_completion = client.chat.completions.create(
messages=[
{'role': 'system', 'content': 'あなたは新卒採用の担当者です。魅力的な求人票を作成し採用計画を達成する責務を負っています。'},
{'role': 'user', 'content': prompt}
],
model='gpt-4o',
)
# Chat GPTからの応答内容を全部表示
print('生成された内容\n\n')
if debug: print(chat_completion)
content = chat_completion.choices[0].message.content
# トークン使用量を表示する
usage = chat_completion.usage
print(usage)
return content
# プロンプトが記載されているファイルパスを指定
file_path = r'C:\Users\user\Desktop\******\job_recruting_prompt.md'
with open(file_path, 'r', encoding='utf-8') as file:
# ファイルの内容を読み込む
prompt = file.read()
print('\n-------------------------------------------------------------\n\n')
print(prompt)
print('\n\nChat GPTからの応答をお待ちください。\n')
result = call_chatgpt(prompt, debug=False)
print(result)
current_time = datetime.now()
date_string = current_time.strftime("%Y_%m%d_%H%M")
result_file_path = fr'C:\Users\user\Desktop\************\newgraduate_chatgpt_{date_string}.md'
with open(result_file_path, 'w', encoding='utf-8') as new_file:
new_file.write(result)
print(f"結果は新規マークダウンファイルに保存されました: {result_file_path}")