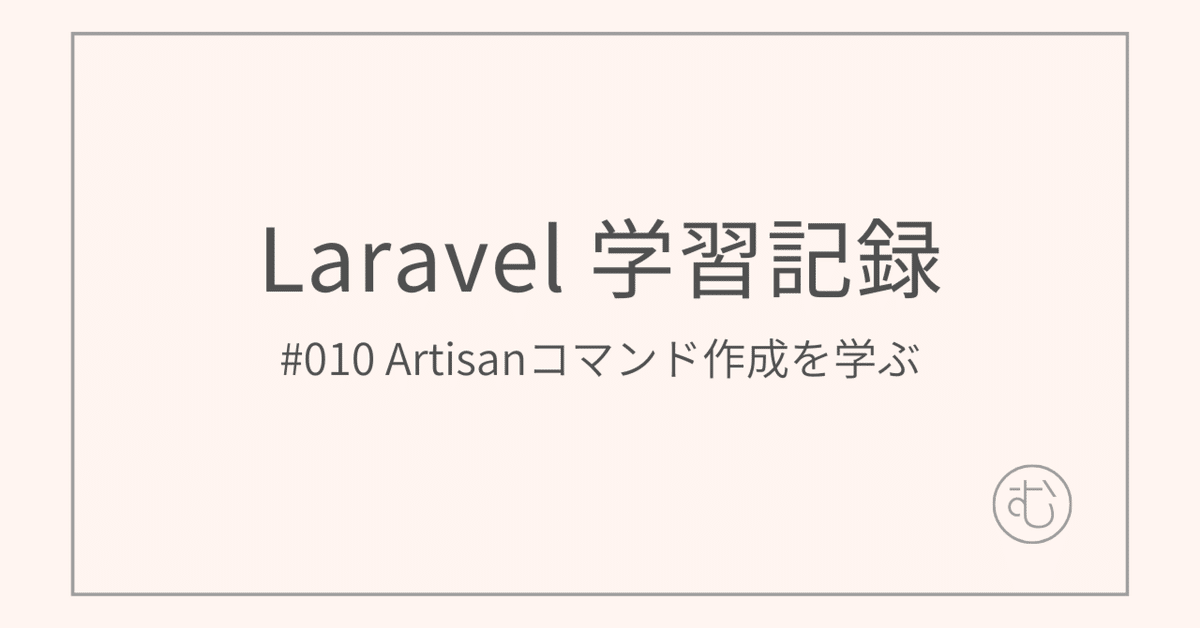
Laravel 学習記録 #010 Artisanコマンド作成を学ぶ
LaravelのArtisanコマンド作成について学習したものをまとめてます。
Artisanコマンドとは
Laravelの専用コマンド
モデルやコントローラーなどの雛形作成、マイグレーションなどができる
コマンドラインから実行できる
他にも機能があって、下記のコマンドで用意されている機能の一覧を確認できます。
php artisan list
開発中に幾度もお世話になるこのArtisanコマンドですが
今回は雛形作成に焦点をあてて、学習しました。
目的は、リクエストクラス、サービスクラスなどの雛形をコマンドで作成できるようにすることです。
Artisanコマンドの作成
作成したいコマンドのファイルを以下のように作成します。
php artisan make:command <作成ファイル名>
例えば サービスクラス用のコマンドを作成するため
php artisan make:command ServiceCommand
とすることで app/Console/Commands配下に
ServiceCommand.php
という名前でファイルが作成されます。
ServiceCommand.php
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class ServiceCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'app:service-command';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Execute the console command.
*/
public function handle()
{
//
}
}
$signature プロパティ:
コマンドを実行する際に使用される名前が指定されています。
上記の場合、php artisan app:service-commandで実行可能になっています。
php artisan make:Service <ファイル名>でサービスクラスの雛形を作成できるようにしたいので、
protected $signature = 'make:Service {filename}' //{filename}をつけることで入力されたファイル名で作成
に変更します。
$description プロパティ:
コマンドの説明です。 php artisan list コマンドを実行した際に表示されるやつです。
handle メソッド:
実際にコマンドが実行されたときに実行されるメソッドです。
ここに、以下のように設定をして、コマンド実行時にサービスクラスの雛形が作成されるようにします。
1.Serviceディレクトリがなければ作成
2.Serviceディレクトリ配下に入力された名前でファイルを作成
3.同名のファイルが存在する場合はコマンドラインでエラー
4.ファイルにはクラスの雛形として、namespaceとクラス名を自動で設定
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class ServiceCommand extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'make:Service {filename}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'サービスクラス作成用コマンド';
/**
* Execute the console command.
*/
public function handle()
{
$filename = $this->argument('filename');
$directory = app_path('Service');
$filePath = "$directory/$filename.php";
// ディレクトリが存在しない場合は作成
if (!file_exists($directory)) {
mkdir($directory, 0755, true);
}
// ファイルが既に存在するかチェック
if (file_exists($filePath)) {
$this->error("Service file $filename already exists. No changes were made.");
return; // ファイルが存在する場合は処理を中断
}
// ファイルを生成し、内容を記述
$content = "<?php\n\nnamespace App\\Service;\n\nclass $filename {\n // Your class content here\n}\n";
file_put_contents($filePath, $content);
$this->info("Service file $filename created successfully in the Service directory with namespace.");
}
}
ファイルを編集したらので、実行してみます。
php artisan make:Service SampleService
結果
app/Service配下にSampleService.phpが作成されていることを確認します。
<?php
namespace App\Service;
class SampleService {
// Your class content here
}
このように作成されているので、OKです。
案外簡単にコマンドの作成ができるようですね。
プロジェクトでよく使うファイルなどはこうして、コマンドで生成できるようにしておきたいです。
この記事が気に入ったらサポートをしてみませんか?