eslintでjsコードを整形する
さて今までブワーッとreact + inertia.jsでプログラムを書いてきた。コードのformatもなんとなーく最初から初期のオートデプロイというかスケルトンに従ってはいたのであるが、ここにきてある程度指標を決めたい。
eslintとは
lintなので基本的には文法チェックなのだけどソースコードのフォーマッティイング(整形)も行うツールである。
install
npm install eslint babel-eslint eslint-config-airbnb eslint-plugin-import eslint-plugin-jsx-a11y eslint-plugin-react eslint-plugin-react-hooks --save-dev
ごちゃっとパッケージを入れているが、ともあれ以下のようにして起動できるようになればok
npx eslint --version
v8.50.0
最低限の設定
.eslintrc.json
{
"extends": ["airbnb"],
"settings": {
"import/resolver": {
"alias": {
"map": [["@", "./resources/js"]],
"extensions": [".js", ".jsx"]
}
}
},
"rules": {
"react/react-in-jsx-scope": "off",
"import/no-extraneous-dependencies": "off",
"react/jsx-props-no-spreading": "off",
"react/prop-types": "off",
"jsx-a11y/label-has-associated-control": "off"
},
"env": {
"browser": true,
"node": true,
"es2021": true
},
"parserOptions": {
"ecmaVersion": 2021,
"sourceType": "module"
},
"globals": {
"route": "readonly"
}
}
.babelrc
{ "presets": ["@babel/preset-react"] }
rulesは基本的にエラーを無視しているところが多い。今回はどちらかというとコードフォーマッティングの方を重視していて、そのルールの根拠はairbnbである。起動してみようすると
./vendor/bin/sail npx eslint resources/js/app.jsx
/var/www/html/resources/js/app.jsx
9:1 error More than 1 blank line not allowed no-multiple-empty-lines
13:1 error Expected indentation of 2 spaces but found 4 indent
14:1 error Expected indentation of 2 spaces but found 4 indent
15:1 error Expected indentation of 2 spaces but found 4 indent
16:1 error Expected indentation of 4 spaces but found 8 indent
18:1 error Expected indentation of 4 spaces but found 8 indent
19:13 error Expected indentation of 10 space characters but found 12 react/jsx-indent
20:17 error Expected indentation of 14 space characters but found 16 react/jsx-indent-props
20:24 error Curly braces are unnecessary here react/jsx-curly-brace-presence
21:17 error Expected indentation of 14 space characters but found 16 react/jsx-indent-props
21:32 error Curly braces are unnecessary here react/jsx-curly-brace-presence
22:17 error Expected indentation of 14 space characters but found 16 react/jsx-indent-props
24:17 error Expected indentation of 14 space characters but found 16 react/jsx-indent
25:40 error Missing trailing comma comma-dangle
26:1 error Expected indentation of 4 spaces but found 8 indent
27:1 error Expected indentation of 2 spaces but found 4 indent
28:1 error Expected indentation of 2 spaces but found 4 indent
29:1 error Expected indentation of 4 spaces but found 8 indent
30:1 error Expected indentation of 2 spaces but found 4 indent
✖ 19 problems (19 errors, 0 warnings)
19 errors and 0 warnings potentially fixable with the `--fix` option.
とするとまずエラー、エラーというかコーディング規約に従っていないところが列挙されてくるから
npx eslint resources/js/app.jsx --fix
fixする
% git diff resources/js/app.jsx
diff --git a/resources/js/app.jsx b/resources/js/app.jsx
index 4d47002..4a49cd6 100644
--- a/resources/js/app.jsx
+++ b/resources/js/app.jsx
@@ -6,26 +6,25 @@ import { createInertiaApp } from '@inertiajs/react';
import { resolvePageComponent } from 'laravel-vite-plugin/inertia-helpers';
import { LaravelReactI18nProvider } from 'laravel-react-i18n';
-
const appName = import.meta.env.VITE_APP_NAME || 'Laravel';
createInertiaApp({
- title: (title) => `${title} - ${appName}`,
- resolve: (name) => resolvePageComponent(`./Pages/${name}.jsx`, import.meta.glob('./Pages/**/*.jsx')),
- setup({ el, App, props }) {
- const root = createRoot(el);
+ title: (title) => `${title} - ${appName}`,
+ resolve: (name) => resolvePageComponent(`./Pages/${name}.jsx`, import.meta.glob('./Pages/**/*.jsx')),
+ setup({ el, App, props }) {
+ const root = createRoot(el);
- root.render(
- <LaravelReactI18nProvider
- locale={'ja'}
- fallbackLocale={'en'}
- files={import.meta.glob('/lang/*.json')}
- >
- <App {...props} />
- </LaravelReactI18nProvider>
- );
- },
- progress: {
- color: '#4B5563',
- },
+ root.render(
+ <LaravelReactI18nProvider
+ locale="ja"
+ fallbackLocale="en"
+ files={import.meta.glob('/lang/*.json')}
+ >
+ <App {...props} />
+ </LaravelReactI18nProvider>,
+ );
+ },
+ progress: {
+ color: '#4B5563',
+ },
});
まあ主にはindentが4から2になっていたりする。これに関しては
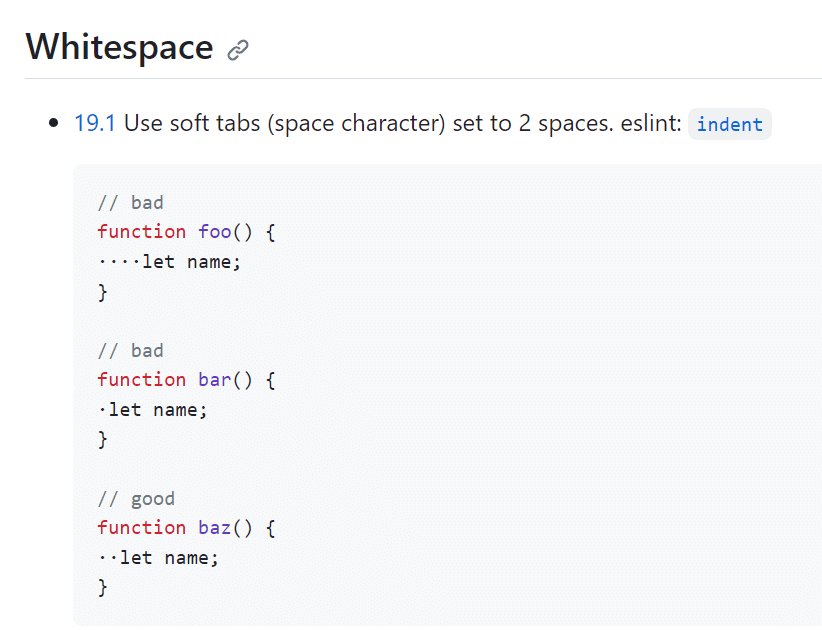
ここにstandard(規約)があるから、見ておくといいかも。
その他の警告
まあ雑にやったので結構出ちゃうんだけど、とりあえずコーディングスタイルは適合してくれる
...
4:16 error 'Link' is defined but never used no-unused-vars
/var/www/html/resources/js/Pages/Users/Index.jsx
2:16 error 'Link' is defined but never used no-unused-vars
13:3 error 'VscInfo' is defined but never used no-unused-vars
20:9 error 'dayjs' is assigned a value but never used no-unused-vars
23:9 error Unexpected use of 'confirm' no-restricted-globals
23:9 warning Unexpected confirm no-alert
71:28 error Do not use Array index in keys react/no-array-index-key
78:1 error This line has a length of 107. Maximum allowed is 100 max-len
97:27 error Missing an explicit type attribute for button react/button-has-type
/var/www/html/resources/js/Pages/Users/Show.jsx
2:16 error 'Link' is defined but never used no-unused-vars
15:9 error 'dayjs' is assigned a value but never used no-unused-vars
18:9 error Unexpected use of 'confirm' no-restricted-globals
18:9 warning Unexpected confirm no-alert
42:1 error This line has a length of 101. Maximum allowed is 100 max-len
/var/www/html/resources/js/Pages/Welcome.jsx
215:28 error `'` can be escaped with `'`, `‘`, `'`, `’` react/no-unescaped-entities
✖ 42 problems (39 errors, 3 warnings)
% git status
On branch breeze-inertia-react-usercrud
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: package-lock.json
modified: package.json
modified: resources/js/Components/AppLink.jsx
modified: resources/js/Components/ApplicationLogo.jsx
modified: resources/js/Components/Breadcrumbs.jsx
modified: resources/js/Components/Checkbox.jsx
modified: resources/js/Components/DangerButton.jsx
modified: resources/js/Components/Dropdown.jsx
modified: resources/js/Components/EmailWithStatus.jsx
modified: resources/js/Components/InputError.jsx
modified: resources/js/Components/InputLabel.jsx
modified: resources/js/Components/LastLoginDisplay.jsx
modified: resources/js/Components/Modal.jsx
modified: resources/js/Components/NavLink.jsx
modified: resources/js/Components/PaginationNav.jsx
modified: resources/js/Components/PrimaryButton.jsx
modified: resources/js/Components/ResponsiveNavLink.jsx
modified: resources/js/Components/RoleDisplay.jsx
modified: resources/js/Components/SecondaryButton.jsx
modified: resources/js/Components/TextInput.jsx
modified: resources/js/Layouts/AuthenticatedLayout.jsx
modified: resources/js/Layouts/GuestLayout.jsx
modified: resources/js/Pages/Auth/ConfirmPassword.jsx
modified: resources/js/Pages/Auth/ForgotPassword.jsx
modified: resources/js/Pages/Auth/Login.jsx
modified: resources/js/Pages/Auth/Register.jsx
modified: resources/js/Pages/Auth/ResetPassword.jsx
modified: resources/js/Pages/Auth/VerifyEmail.jsx
modified: resources/js/Pages/Dashboard.jsx
modified: resources/js/Pages/Profile/Edit.jsx
modified: resources/js/Pages/Profile/Partials/DeleteUserForm.jsx
modified: resources/js/Pages/Profile/Partials/UpdatePasswordForm.jsx
modified: resources/js/Pages/Profile/Partials/UpdateProfileInformationForm.jsx
modified: resources/js/Pages/Users/ActivityLog.jsx
modified: resources/js/Pages/Users/Create.jsx
modified: resources/js/Pages/Users/Edit.jsx
modified: resources/js/Pages/Users/Index.jsx
modified: resources/js/Pages/Users/Partials/UserForm.jsx
modified: resources/js/Pages/Users/Show.jsx
modified: resources/js/Pages/Welcome.jsx
modified: resources/js/app.jsx
まあこれはこれでcommitしちゃっていいと思う
vimがjsonとかをindent: 4で取りにいく問題
さて今、全体的にindent:2に変更されたが依然としてindent :4で取りにいっているのを何とかしたいような。これは.editorconfigに記述できるというかそれで十分。vscodeも多分何とかなるはず。
[*.{js,jsx,json}]
charset = utf-8
end_of_line = lf
indent_size = 2
indent_style = space
insert_final_newline = true
trim_trailing_whitespace = true
ここまでで
一連の認証を見たりusersのcrudを作ったりするのはおしまい。次回はこれをsurvey.jsに統合したりする。これはマガジンになる予定。