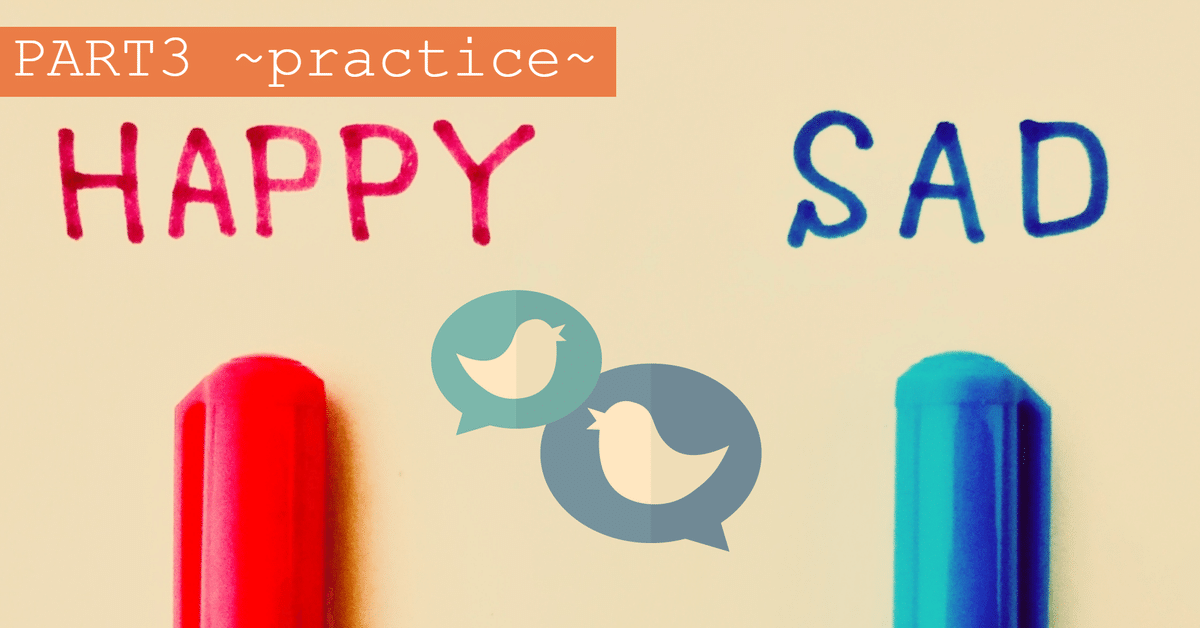
twitterのツイートを使って感情分析をしてみよう(初級者向け)~実践編~
前回は、感情分析のための環境構築についてご紹介しました。
確認までに前回のおさらいをします。
・twitter APIを用いてツイートを自動的に取得する
・ツイートを形態素解析する
・感情辞書(極性辞書)を用いてツイートの感情をスコアリングする
・CentOS release 6
・Apache 2.2
・PHP 5.6
・mecab本体とipa辞書のインストール
・mecab-phpのインストール
・感情辞書(極性辞書)のインストール
・twitter API の申請およびAPIキー等の取得
・abraham/twitteroauth のインストール
本記事は、実際にプログラムを動かして感情分析をする方法をご紹介いたします。
はじめに
githubにてソースを公開しておりますので、こちらのソースに沿ってご説明をしていきます。
config.php の中に
・API key
・API secret key
・Access token
・Access token secret
がありますので、ここにtwitter API の登録後に取得したAPI key とトークンを入力してください。
1. 分析手順
初期画面で、最近のツイートの中から感情分析したいキーワードを入力します。
「analysis」を選択すると、分析結果が表示されます。
scoreが正だとポジティブ、負だとネガティブと判定されます。
2.プログラムの説明
それでは、実際にプログラムを見ながら分析方法をご紹介していきます。
初期画面
最初の画面が表示されます。
ここで調べたいキーワードを入力して、「analysis」を選択すると、main.phpが呼び出されて分析が始まります。
感情分析のロジック
それでは実際にmain.phpを見ていきます。
<?php
require_once('./config.php');
require_once('./feelings.php');
$dir = str_replace('batch', 'func', dirname(__FILE__));
require_once '../../vendor/autoload.php';
use Abraham\TwitterOAuth\TwitterOAuth;
// create instance of TwitterOAuth .
$obj = new TwitterOAuth(TWITTER_API_KEY, TWITTER_API_SECRET, ACCESS_TOKEN, ACCESS_SECRET);
// get keyword.
$keyword = $_GET["keyword"];
// q is search word, count is search number, lang is written language. result_type is wheather recent or not. count is number for serching.
$options = array('q'=>$keyword,'result_type' => 'recent', 'count' => SEARCH_COUNT );
$json = $obj->get("search/tweets", $options);
$statuses = null;
if ($json){
$statuses = $json->statuses; // get status
}
$sum_array = array(
'scores' => 0,
'positive_count' => 0,
'negative_count' => 0,
);
if ($statuses && is_array($statuses)) {
foreach($statuses as $value){
$feelings = new Feelings(PERMIT_ONLY_ADJECTIVE);
$data = $feelings->getAnalizedData($value->text);
$scores = $data['scores'];
$positive_count = $data['positive_count'];
$negative_count = $data['negative_count'];
$sum_array['scores'] = $scores + $sum_array['scores'];
$sum_array['positive_count'] = $positive_count + $sum_array['positive_count'];
$sum_array['negative_count'] = $negative_count + $sum_array['negative_count'];
}
}
?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>test sentiment analysis</title>
</head>
<body>
<?php
echo "keyword : {$keyword}<br>";
echo "score : {$sum_array['scores']} <br>";
echo "positive word count : {$sum_array['positive_count']} <br>";
echo "negative word count : {$sum_array['negative_count']} <br>";
?>
</body>
</html>
composerでインストールしたAbraham\TwitterOAuth\TwitterOAuthを呼び出します。
require_once './vendor/autoload.php';
use Abraham\TwitterOAuth\TwitterOAuth;
twitter APIを呼び出します。ご自身のAPI key、API secret key、Access token、Access token secretをconfig.phpに入力してください。
// create instance of TwitterOAuth .
$obj = new TwitterOAuth(TWITTER_API_KEY, TWITTER_API_SECRET, ACCESS_TOKEN, ACCESS_SECRET);
初期画面で入力したキーワードを分析します。最近のツイートを取得するために、search/tweets というパラメータを指定してStandard search APIを呼び出します。
無料でAPIを使用する場合、リクエスト回数が15分以内で180回までという制限がありますのでお気を付けください。(※2020年5月14日現在)
// q is search word, count is search number, lang is written language. result_type is wheather recent or not. count is number for serching.
$options = array('q'=>$keyword,'result_type' => 'recent', 'count' => SEARCH_COUNT );
$json = $obj->get("search/tweets", $options);
次に取得したツイートを分析します。PERMIT_ONLY_ADJECTIVEは、形容詞のみを対象にするか否かの判定です。今回は形容詞のみ分析対象としました。変更したい場合は、PERMIT_ONLY_ADJECTIVEを0に設定してください。
$feelings = new Feelings(PERMIT_ONLY_ADJECTIVE);
$data = $feelings->getAnalizedData($value->text);
分析はfeelings.phpで行っております。feelings.phpを見ていきましょう。
コンストラクタで、MeCabを呼び出します。IPA_DICTIONARY_PATHでipa辞書のパスを指定してください。
public function __construct($p_permit_only_adjective) {
// Load mecab module
$options = array('-d', IPA_DICTIONARY_PATH);
$this->mecab = new \MeCab\Tagger($options);
$this->permit_only_adjective = $p_permit_only_adjective;
}
$this->mecab->parseToNodeで形態素解析をします。
$node = $this->mecab->parseToNode($p_str);
while($node){
if ( $node->getSurface() ) {
// insert word and type
$text_arr[] = array('type' => explode(',', $node->getFeature())[0], 'word' => $node->getSurface());
}
// next
$node = $node->getNext();
}
形態素解析した単語ごとに、感情辞書(極性辞書)を使ってスコアリングしていきます。出現したポジティブとネガティブの単語の回数も取得していきます。
if ( $rows['type'] === $master[2] && ($rows['word'] === $master[0] || $rows['word'] === $master[1]) ) {
$float_score = (float)trim($master[3]);
$scores += $float_score;
if ( $float_score > 0 ) {
$positive_count++;
$posi_words .= " " . $rows['word'] . $float_score;
}
else {
$negative_count++;
$nega_words .= " " . $rows['word'] . $float_score;
}
}
最後に、main.phpでスコアと回数を集計して画面に表示します。
<?php
echo "keyword : {$keyword}<br>";
echo "score : {$sum_array['scores']} <br>";
echo "positive word count : {$sum_array['positive_count']} <br>";
echo "negative word count : {$sum_array['negative_count']} <br>";
?>
以上で感情分析が行えるようになります。
おわりに
今回は、エンジニア初級者向けということでシンプルな感情分析の手法をご紹介いたしました。
今回の手法は、シンプルであるがゆえに分析の正確さを欠くという問題点もあります。
問題点に関しては、こちらをご覧ください。
本記事をきっかけに、少しでも感情分析にご興味を持っていただけましたら、大変うれしく思います。
最後までお付き合いいただきまして、ありがとうございました。
この記事が気に入ったらサポートをしてみませんか?