FullStackOpen Part2-c Getting data from server メモ
json-serverを使ってjsonファイルを提供する
npx json-server --port 3001 --watch db.json
アクセスするときはhttp://localhost:3001/notes
jsonの中身:
{
"notes": [
{
"id": 1,
"content": "HTML is easy",
"important": true
},
{
"id": 2,
"content": "Browser can execute only JavaScript",
"important": false
},
{
"id": 3,
"content": "GET and POST are the most important methods of HTTP protocol",
"important": true
}
]
}
The browser as a runtime environment
昔はXHR(XMLHttpRequest)を使ってサーバからデータを取っていた。
xhttpオブジェクトのイベントハンドラーを登録し、状態の変化により実行する形
const xhttp = new XMLHttpRequest()
xhttp.onreadystatechange = function(){
if(this.readyState == 4 && this.status == 200){
const data = JSON.parse(this.responseText)
//handle the response
}
}
xhttp.open('GET', '/data.json', true)
xhttp.send()
Javascriptでは非同期的にHTTPリクエストを処理する
npm
Node Package Managerの略
ブラウザからサーバのデータを取得する際にはaxiosライブラリを使用する
npm install axiosでインストール(プロジェクトのルートディレクトリで実行する)
npm install json-server --save-devでjson-serverをインストール
package.jsonに以下を追加しておくとnpm run serverで起動できるようになる
{
// ...
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject",
"server": "json-server -p3001 --watch db.json" }, //これ
}
--save-devというオプションはプログラム自体にはjson-serverは必要ないが、開発依存関係として追加するという意味
Axios and Promises
axiosはimport axios from 'axios'で使用
Promiseとは非同期処理の結果(成功、失敗)を表すオブジェクトである
promiseには3つのstateがあり、Pending, Fulfilled, Rejectedである
Promoseの結果にアクセスするにはthen()を使う
const promise = axios.get('http://localhost:3001/notes')
promise.then(response => {
console.log(response)
})
responseにはデータのほかにヘッダやリクエストに関する情報がオブジェクトとして入っている
response.dataとしてアクセスデキル
Effect-hooks
副作用フックは関数コンポーネントに対して副次的な処理を実行できる
例えばデータの取得、サブスクリプションの設定、ReactコンポーネントのDOMの手動変更などに便利
特にサーバーからデータをフェッチするのに適したツールである
以下のような感じ
import { useState, useEffect } from 'react'
const hook = () => {
console.log('effect')
axios
.get('http://localhost:3001/notes')
.then(res => {
console.log('promise fulfilled')
setNotes(res.data)
})
}
useEffect(hook, [])
return (
<>
...
</>
)
useEffectは引数を2つ取り、1つ目はイベントハンドラ、2つ目は配列を取る
配列が空欄の場合はコンポーネントのレンダリング実行後に実行される
そのため上記コードの場合、以下のような順で実行される
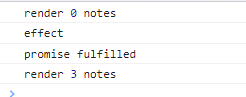