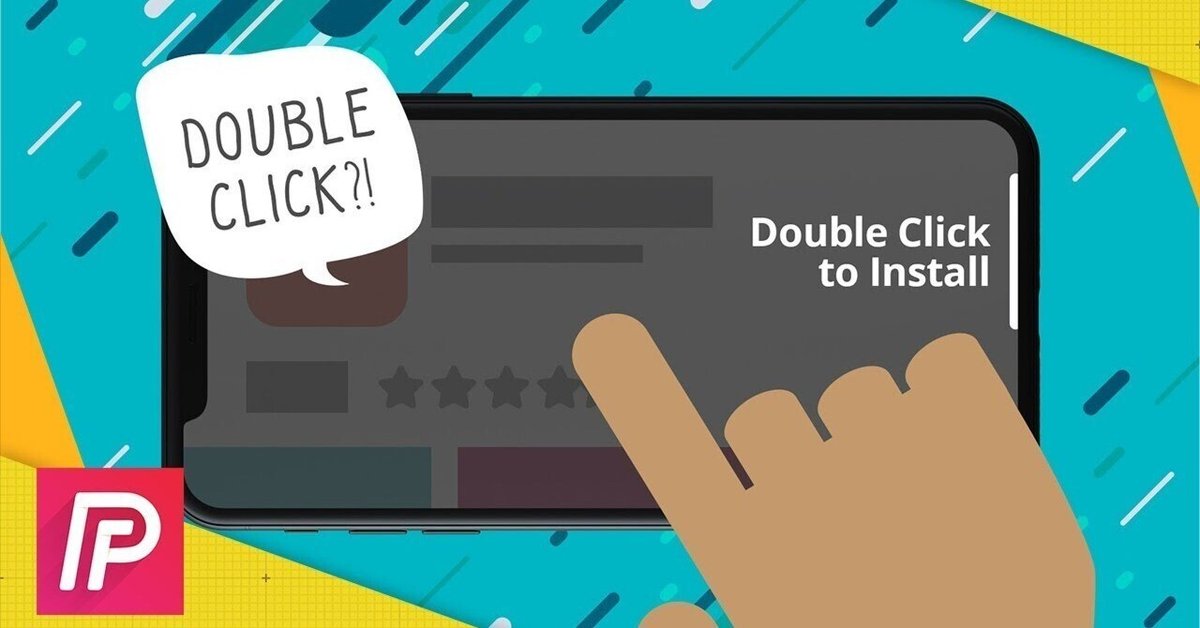
How to prevent all button double click on your web application
Recently, I have some issue about double click on a web application. I think that sometime exist on your project.
In my case, I have applied the following simple code to resolving the problems. Hope it help you on your projects.
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Testing double click</title>
</head>
<body>
<input type="button" value="Button" id="btn-submit">
<a href="#!">Link 1</a>
<input type="submit" value="Submit" id="btn-submit">
</body>
<script type="text/javascript" src="https://code.jquery.com/jquery-3.2.1.min.js"></script>
<script type="text/javascript">
// Stand-in for something that takes time
function doSomethingThatTakesTime() {
return new Promise(resolve => setTimeout(resolve, 2000));
}
// Code triggered by the button
let running = 0;
function doOperation(e) {
if (running > 0) {
console.log("Call ignored, already running");
return;
}
++running;
updateUI(e);
console.log("Started running");
doSomethingThatTakesTime()
.finally(() => {
console.log("Done running");
--running;
updateUI(e);
});
}
// Update the UI to match our current state
function updateUI(e) {
isDisable = running > 0;
if (e.target.localName == 'a') {
isDisable ? e.target.style.pointerEvents = "none" : e.target.style.pointerEvents = ""
} else {
e.target.disabled = isDisable;
}
}
// Handle clicks on the button
$(document).on('click', 'a, :button, :submit', (e) => {doOperation(e)});
</script>
</html>
この記事が気に入ったらサポートをしてみませんか?