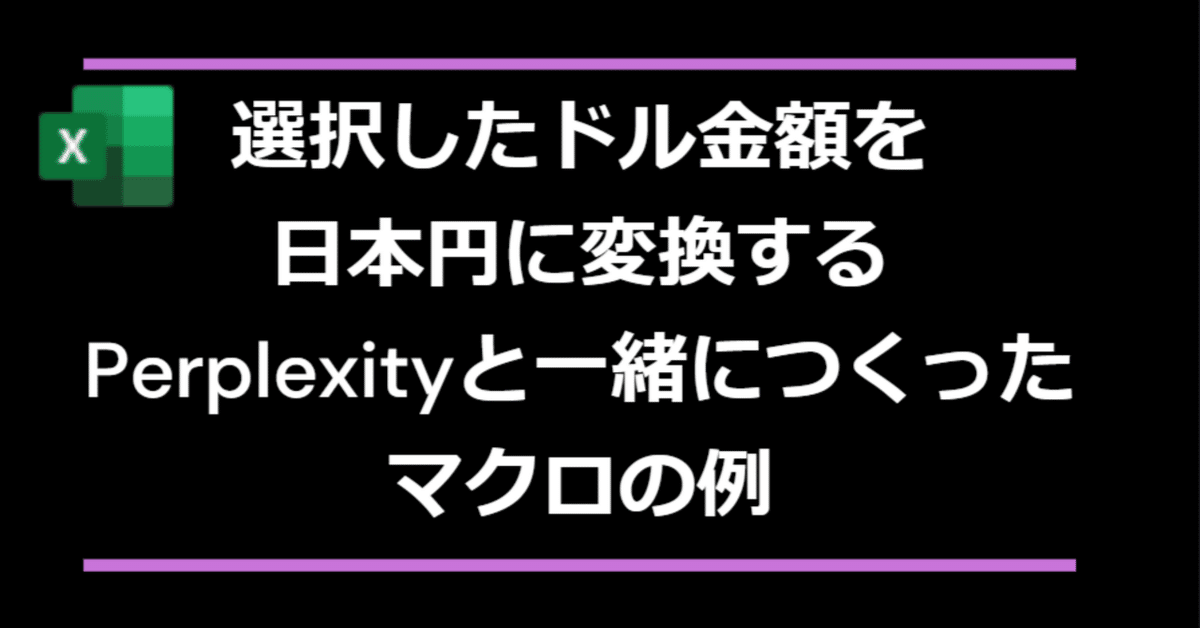
選択したドル金額を日本円に変換するPerplexityと一緒に作ったやつ
この説明は、ChatGPTで作成しています。
このVBAプロシージャは、選択したセルに入力されたドル金額を、日本円に変換して隣のセルに表示するものです。インターネットを通じて最新の為替レートを取得し、それに基づいて円に換算する仕組みになっています。順を追って説明していきますね。
プロシージャの動き
為替レートの取得:
インターネットを利用して、ドルから円への最新の為替レートを取得します。
"https://api.excelapi.org/currency/rate?pair=usd-jpy" というURLから為替レートを取得し、それをコード内で使えるようにしています。
もし為替レートの取得に失敗した場合、メッセージが表示されて終了します。
選択されたセルのデータを処理:
まず、選択されたセルの中身を1つずつ確認します。
セルが空でなく、数値として認識できる場合のみ処理が進みます。
ドルの金額を読み取り、先ほど取得した為替レートを使って日本円に変換します。
変換結果の出力:
変換した結果は、元のセルの右隣に表示されます。
また、為替レートも元のセルの隣のセルに表示されます。
表示された円の金額は、四捨五入されて小数点なしの金額になります。
エラー処理:
万が一エラーが発生した場合は、エラーメッセージを表示して、プロシージャが安全に終了するようになっています。
使い方の手順
ドルの金額を変換したいセルを選びます。
プロシージャを実行します。
各セルの右隣に為替レート、さらにその隣に変換された円の金額が表示されます。
例
例えば、セル A1 に「100」と入力されていたとします。プロシージャを実行すると、
B1 には現在の為替レート(例えば「150.25」)が表示され、
C1 には「15025」(= 100ドル × 150.25円)のように円での金額が表示されます。
実際のコードのポイント
`MSXML2.XMLHTTP` という機能を使ってインターネットから為替レートを取得しています。これは、プログラムの中でWebページにアクセスするための方法です。
選択したセルだけを処理するようにしているので、他のセルに影響を与える心配はありません。
Sub 選択したドル金額を日本円に変換するPerplexityと一緒に作ったやつ()
Dim nowCell As Range
Dim usd, jpy, rate As Double
Dim url As String
Dim xmlHttp As Object
' エラー処理
On Error GoTo Err
' 為替レートを取得
url = "https://api.excelapi.org/currency/rate?pair=usd-jpy"
Set xmlHttp = CreateObject("MSXML2.XMLHTTP")
With xmlHttp
.Open "GET", url, False
.Send
If .Status = 200 Then
rate = CDbl(.responseText)
Else
MsgBox "為替レートの取得に失敗しました。", vbExclamation
Exit Sub
End If
End With
Set xmlHttp = Nothing
' レート取得に失敗した場合
If rate <= 0 Then
MsgBox "為替レートの取得に失敗しました。", vbExclamation
Exit Sub
End If
' 選択された各セルに対して処理を実行
For Each nowCell In Selection
' セルが空でなく、数値として解釈できる場合のみ処理
If Not IsEmpty(nowCell) And IsNumeric(nowCell.Value) Then
' ドル金額を取得
usd = CDbl(nowCell.Value)
' 円に変換
jpy = usd * rate
nowCell.Offset(0, 1).Value = rate
nowCell.Offset(0, 1).NumberFormatLocal = "0.00"
' 結果を隣のセルに出力(小数点以下を四捨五入)
nowCell.Offset(0, 2).Value = Round(jpy, 0)
' 表示形式を通貨形式に設定
nowCell.Offset(0, 2).NumberFormatLocal = "\#,##0"
End If
Next nowCell
Exit Sub
Err:
MsgBox "エラーが発生しました: " & Err.Description, vbCritical
End Sub
キーワード
#excel #できること #vba #為替レート #ドルから円 #通貨変換 #選択したセル #自動計算 #インターネット接続 #XMLHTTP #エラー処理 #リアルタイム #数値変換 #プログラミング初心者 #金融計算 #通貨換算 #セルの操作 #業務効率化 #初心者向けVBA #プロシージャ解説
英語版
Sub Convert Selected Dollar Amounts to Japanese Yen (Created with Perplexity)
This explanation is created using ChatGPT.
This VBA procedure is designed to convert dollar amounts entered in selected cells to Japanese yen and display the result in adjacent cells. It fetches the latest exchange rate via the internet and uses that rate to perform the conversion. Let me explain step by step.
How the Procedure Works
Fetching the Exchange Rate:
The procedure uses the internet to fetch the latest USD to JPY exchange rate.
It retrieves the rate from the URL "https://api.excelapi.org/currency/rate?pair=usd-jpy" and makes it available in the code.
If fetching the rate fails, a message will be displayed, and the procedure will terminate.
Processing Data in Selected Cells:
It goes through each selected cell one by one.
It only processes the cell if it's not empty and can be interpreted as a number.
The procedure reads the dollar amount, uses the previously fetched exchange rate, and converts it to Japanese yen.
Outputting the Conversion Result:
The converted result is displayed to the right of the original cell.
The exchange rate is shown in the cell next to the original cell.
The yen amount displayed is rounded off to a whole number.
Error Handling:
If any error occurs, an error message is displayed, and the procedure safely ends.
Usage Steps
Select the cells with dollar amounts you want to convert.
Run the procedure.
The exchange rate will appear in the cell to the right of each selected cell, and the converted yen amount will be shown in the next cell.
Example
Suppose cell A1 contains "100." When the procedure runs:
B1 will show the current exchange rate (e.g., "150.25"),
C1 will display "15025" (= 100 dollars × 150.25 yen), the converted yen amount.
Key Points of the Code
It uses `MSXML2.XMLHTTP` to fetch the exchange rate from the internet. This is a way to access a web page within the program.
It processes only the selected cells, ensuring that no other cells are affected.
Keywords
#excel #whatspossible #vba #exchangerate #usdtojpy #currencyconversion #selectedcells #automaticcalculation #internetconnection #XMLHTTP #errorhandling #realtime #numberconversion #programmingforbeginners #financialcalculation #currencyexchange #celloperation #workefficiency #vbaforbeginners #procedureexplanation