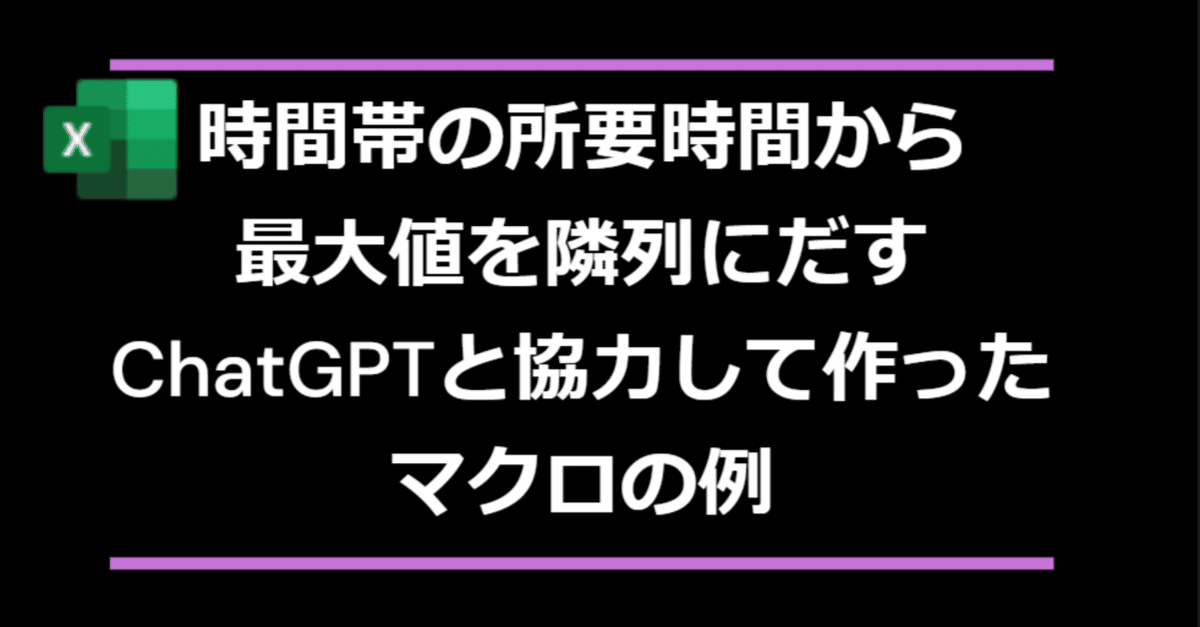
時間帯の所要時間から最大値を隣列にだすChatGPTと一緒につくったやつ
この説明は、ChatGPTで作成しています。
このVBAコードは、選択したセルに記載されている時間帯(例:「12:30~14:00」)を解析し、その時間帯の所要時間を計算します。そして、そのセルの隣の列にその中での最大の所要時間(時間単位)を表示します。
仕組みの説明
正規表現を使った時間の抽出
コードの最初では、正規表現を設定し、セルの中に「開始時間~終了時間」の形式で書かれている部分を探します。例えば、「09:00~12:00」といった時間帯がセルにあった場合、これを取り出して処理します。時間帯の解析
時間帯が見つかった場合、開始時間と終了時間を取り出し、それらを元に所要時間を計算します。もし終了時間が「24:00」になっている場合は、次の日の「00:00」として扱います。時間が「マイナス」になる場合(例えば、22:00~02:00のような場合)、それを24時間制に補正します。最大所要時間の計算
各時間帯の所要時間を計算し、複数の時間帯があった場合、その中で一番長い時間(最大所要時間)を保持します。結果を隣の列に表示
各セルで見つけた最大の所要時間を、選択したセルの隣の列に「○時間」の形式で表示します。所要時間は少数点以下2桁まで表示されます。
例えば、セルに「09:00~12:00, 14:00~16:30」とあった場合、2つの時間帯のうち長い方(2時間30分)の時間が隣のセルに「2.50」として表示されます。
コードの流れのポイント
ScreenUpdating: 画面の更新を一時停止して処理を速くし、終了後に再開します。
正規表現: 「~」で区切られた時間帯を見つけるために使用します。
時間の計算: TimeValue 関数を使って、文字列で表現された時間を時間データに変換します。
所要時間の最大値を計算: すべての時間帯の中で最長のものを見つけ、隣のセルに出力します。
まとめ
このコードは、時間帯を扱う場面で非常に便利です。例えば、1日の中で複数の時間帯を管理しているときに、どの時間帯が最も長いかを自動的に計算して隣の列に表示してくれます。Excelシート上で時間データを使う作業を効率的に行うためのツールとして役立ちます。
Sub 時間帯の所要時間から最大値を隣列にだすChatGPTと一緒につくったやつ()
Application.ScreenUpdating = False
Dim reg As Object
Set reg = CreateObject("VBScript.RegExp") 'オブジェクト作成
Dim myRng As Range
Dim txt, totalDuration As String
Dim matches, match As Object
Dim startTime, endTime As Date
Dim duration, maxDuration As Double
' 正規表現パターンの設定
With reg
.Pattern = "\b([01]?[0-9]|2[0-3]):[0-5][0-9]~((24:00)|([01]?[0-9]|2[0-3]):[0-5][0-9])\b"
.IgnoreCase = True
.Global = True
End With
On Error Resume Next
' セル範囲のループ処理
For Each myRng In Selection
txt = myRng.Value
totalDuration = "" ' 初期化
maxDuration = 0 ' 最大所要時間を初期化
If reg.Test(txt) Then
Set matches = reg.Execute(txt)
' マッチした場合
For Each match In matches
' 時間帯の解析
Dim times() As String
times = Split(match.Value, "~")
' 開始時間と終了時間を変数に格納
startTime = TimeValue(times(0))
' 終了時間が「24:00」の場合の処理
If times(1) = "24:00" Then
endTime = TimeValue("00:00") + 1 ' 24時間を表す
Else
endTime = TimeValue(times(1))
End If
' 所要時間を計算
duration = endTime - startTime
' 所要時間が負の場合、24時間経過とみなす
If duration < 0 Then
duration = duration + 1 ' 1は24時間を表す
End If
' 最大所要時間を更新
If duration > maxDuration Then
maxDuration = duration
End If
Next match
' 最大所要時間を隣列に出力する(単位を時間に変換して出力)
myRng.Offset(0, 1).Value = Format(maxDuration * 24, "0.00")
End If
Next myRng
Application.ScreenUpdating = True
End Sub
#excel #vba #できること #正規表現 #時間管理 #所要時間 #最大値 #時間計算 #開始時間 #終了時間 #時間解析 #24時間対応 #データ処理 #隣列出力 #自動化 #範囲指定 #TimeValue #セル操作 #フォーマット #画面更新 #VBAオブジェクト
English Version:
Time Interval Duration and Max Value Extraction with VBA
This explanation is created with ChatGPT.
This VBA code analyzes time intervals written in a selected cell (e.g., "12:30~14:00"), calculates the duration of those intervals, and outputs the longest duration to the adjacent column.
How It Works:
Extracting Time Intervals Using Regular Expressions:
At the start, the code sets up a regular expression to find patterns like "start time~end time" in the cell. For instance, if the cell contains "09:00~12:00," the code extracts this time period for processing.Parsing Time Intervals:
If a match is found, the code separates the start and end times. It then calculates the duration between them. If the end time is "24:00," it handles this as "00:00" on the next day. If the time calculation results in a negative value (for example, from "22:00 to 02:00"), the code adjusts it to a 24-hour format.Calculating Maximum Duration:
The code calculates the duration of each time interval, and if multiple intervals are present, it tracks the longest duration.Displaying Results in Adjacent Column:
The longest duration is then displayed in the adjacent column as hours with two decimal places.
For instance, if a cell contains "09:00~12:00, 14:00~16:30," the longest duration (2 hours 30 minutes) will be displayed in the adjacent cell as "2.50."
Key Points of the Code:
ScreenUpdating: Temporarily pauses screen updates to speed up the process, and resumes updates after completion.
Regular Expressions: Used to detect time intervals separated by "~."
Time Calculations: The TimeValue function converts string-based time values into actual time data.
Finding Maximum Duration: The code finds the longest duration among all intervals and outputs the result in the adjacent cell.
Summary:
This code is useful in scenarios where you need to manage multiple time intervals and automatically calculate which one is the longest. It can be a helpful tool for tasks involving time data in Excel sheets, making time management more efficient.
#excel #vba #time_management #duration_calculation #regular_expression #start_time #end_time #24_hour_format #data_processing #adjacent_column #automation #TimeValue #cell_operations #formatting #screen_update #VBA_object #maximum_duration #time_parsing #interval_analysis
この記事が気に入ったらサポートをしてみませんか?