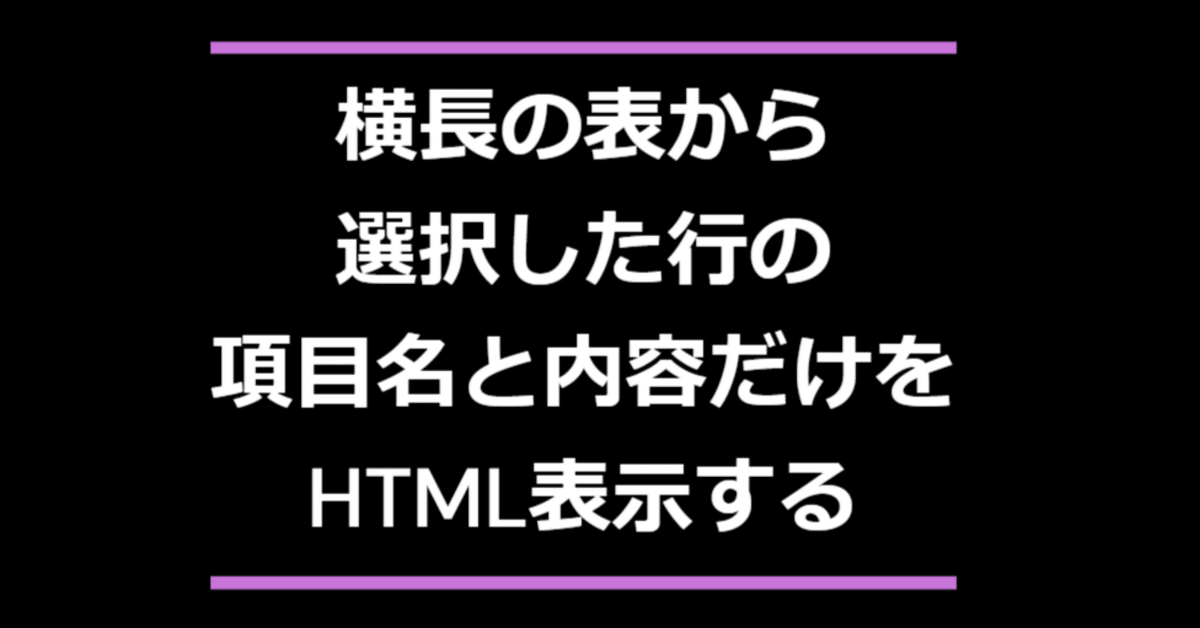
選択したレコードを指定した項目だけHTMLに表示する
この説明は、ChatGPTで作成しています。
このプロシージャは、Excelで選択されたレコードを特定の項目だけ表示するためのHTMLを作成し、そのHTMLをMicrosoft Edgeで表示するものです。以下の手順で動作します。
アクティブなワークシートを取得
最初に、現在アクティブになっているワークシートを取得します。選択範囲を取得
次に、ユーザーが選択したセル範囲を取得します。単一のレコードが選択されているかを確認
ユーザーが複数の行を選択している場合にはエラーメッセージを表示し、プロシージャを終了します。列名を取得
ワークシートの1行目にある列名を取得します。選択したレコードの値を取得
ユーザーが選択した行の値を取得します。表示するカラム名と順番を定義
HTMLに表示するカラム名を定義します。HTMLを準備
HTMLの構造を準備します。指定されたカラム名に基づいて、対応するセルの値をHTMLに追加します。エラーがある場合には「●エラー●」と表示します。また、改行をHTMLの`<br>`タグに変換します。HTMLを一時ファイルに書き込み
作成したHTMLを一時ファイルに保存します。HTMLファイルをMicrosoft Edgeで開く
保存したHTMLファイルをMicrosoft Edgeで開きます。
Sub 選択したレコードを指定した項目だけHTMLに表示する()
' アクティブなワークシートを取得
Dim ws As Worksheet
Set ws = ActiveSheet
' 選択範囲を取得
Dim rng As Range
Set rng = Application.Selection
' 単一のレコードが選択されているかを確認
If rng.Rows.Count <> 1 Then
MsgBox "単一のレコードを選択してください。", vbExclamation
Exit Sub
End If
' 列名を取得
Dim headers As Variant
headers = ws.Rows(1).Value ' すべての列名を取得
' 選択したレコードの値を取得
Dim recordRow As Variant
recordRow = ws.Rows(rng.Row).Value ' 選択したレコードのすべての値を取得
' 表示するカラム名と順番を定義
Dim displayColumns As Variant
displayColumns = Array("タイトル", "応募先会社名", "給与", "仕事内容")
' HTMLを準備
Dim htmlOutput As String
htmlOutput = "<html><body>"
Dim i As Integer, j As Integer
For j = LBound(displayColumns) To UBound(displayColumns)
For i = LBound(headers, 2) To UBound(headers, 2)
If headers(1, i) = displayColumns(j) Then
' エラー表示を代替テキストに置き換え
Dim cellValue As String
If IsError(recordRow(1, i)) Then
cellValue = " ●エラー● "
Else
cellValue = recordRow(1, i)
End If
' 改行をHTMLの<br>タグに変換
cellValue = Replace(cellValue, vbLf, "<br>")
' HTMLに列名とセルの値を追加
htmlOutput = htmlOutput & "<div><strong>" & headers(1, i) & "</strong></div>"
htmlOutput = htmlOutput & "<div>" & cellValue & "</div><br>"
Exit For
End If
Next i
Next j
htmlOutput = htmlOutput & "</body></html>"
' HTMLを一時ファイルに書き込み
Dim tempFilePath As String
tempFilePath = Environ$("temp") & "\temp.html"
Dim fileNum As Integer
fileNum = FreeFile
Open tempFilePath For Output As fileNum
Print #fileNum, htmlOutput
Close fileNum
' HTMLファイルをMicrosoft Edgeで開く
Dim edgePath As String
edgePath = """C:\Program Files (x86)\Microsoft\Edge\Application\msedge.exe"""
Dim shellCommand As String
shellCommand = edgePath & " " & tempFilePath
Shell shellCommand, vbNormalFocus
End Sub
キーワード:
#excel #できること #vba #選択範囲 #HTML表示 #単一レコード #列名取得 #エラー処理 #カラム表示 #一時ファイル #MicrosoftEdge #Web表示 #Excelワークシート #セル値取得 #改行変換 #Edgeブラウザ #Excel自動化 #レコード表示 #応募先会社名 #仕事内容
Display Selected Record in HTML
This explanation is created by ChatGPT.
This procedure generates HTML to display specified columns of a selected record in Excel and opens it in Microsoft Edge. Here’s how it works:
Get Active Worksheet
First, the procedure retrieves the currently active worksheet.Get Selected Range
Next, it gets the range of cells selected by the user.Check if a Single Record is Selected
If multiple rows are selected, it displays an error message and exits the procedure.Retrieve Column Names
The column names in the first row of the worksheet are retrieved.Retrieve Values of Selected Record
The values in the selected row are retrieved.Define Columns to Display
The columns to display in HTML are defined.Prepare HTML
The HTML structure is prepared. The corresponding cell values for the specified columns are added to the HTML. Errors are displayed as "●Error●", and line breaks are converted to `<br>` tags.Write HTML to Temporary File
The created HTML is saved to a temporary file.Open HTML File in Microsoft Edge
The saved HTML file is opened in Microsoft Edge.
Sub DisplaySelectedRecordInHTML()
' Get Active Worksheet
Dim ws As Worksheet
Set ws = ActiveSheet
' Get Selected Range
Dim rng As Range
Set rng = Application.Selection
' Check if a Single Record is Selected
If rng.Rows.Count <> 1 Then
MsgBox "Please select a single record.", vbExclamation
Exit Sub
End If
' Retrieve Column Names
Dim headers As Variant
headers = ws.Rows(1).Value
' Retrieve Values of Selected Record
Dim recordRow As Variant
recordRow = ws.Rows(rng.Row).Value
' Define Columns to Display
Dim displayColumns As Variant
displayColumns = Array("Title", "Company Name", "Salary", "Job Description")
' Prepare HTML
Dim htmlOutput As String
htmlOutput = "<html><body>"
Dim i As Integer, j As Integer
For j = LBound(displayColumns) To UBound(displayColumns)
For i = LBound(headers, 2) To UBound(headers, 2)
If headers(1, i) = displayColumns(j) Then
Dim cellValue As String
If IsError(recordRow(1, i)) Then
cellValue = " ●Error● "
Else
cellValue = recordRow(1, i)
End If
cellValue = Replace(cellValue, vbLf, "<br>")
htmlOutput = htmlOutput & "<div><strong>" & headers(1, i) & "</strong></div>"
htmlOutput = htmlOutput & "<div>" & cellValue & "</div><br>"
Exit For
End If
Next i
Next j
htmlOutput = htmlOutput & "</body></html>"
' Write HTML to Temporary File
Dim tempFilePath As String
tempFilePath = Environ$("temp") & "\temp.html"
Dim fileNum As Integer
fileNum = FreeFile
Open tempFilePath For Output As fileNum
Print #fileNum, htmlOutput
Close fileNum
' Open HTML File in Microsoft Edge
Dim edgePath As String
edgePath = """C:\Program Files (x86)\Microsoft\Edge\Application\msedge.exe"""
Dim shellCommand As String
shellCommand = edgePath & " " & tempFilePath
Shell shellCommand, vbNormalFocus
End Sub
[Excel VBA Reference | Microsoft Learn](
https://learn.microsoft.com/en-us/office/vba/api/overview/excel)
Keywords:
#excel #features #vba #selectedrange #htmloutput #singlerecord #getcolumnnames #errorhandling #displaycolumns #tempfile #MicrosoftEdge #webdisplay #worksheet #getcellvalues #linebreakconversion #edgebrowser #excelautomation #displayrecord #companyname #jobdescription