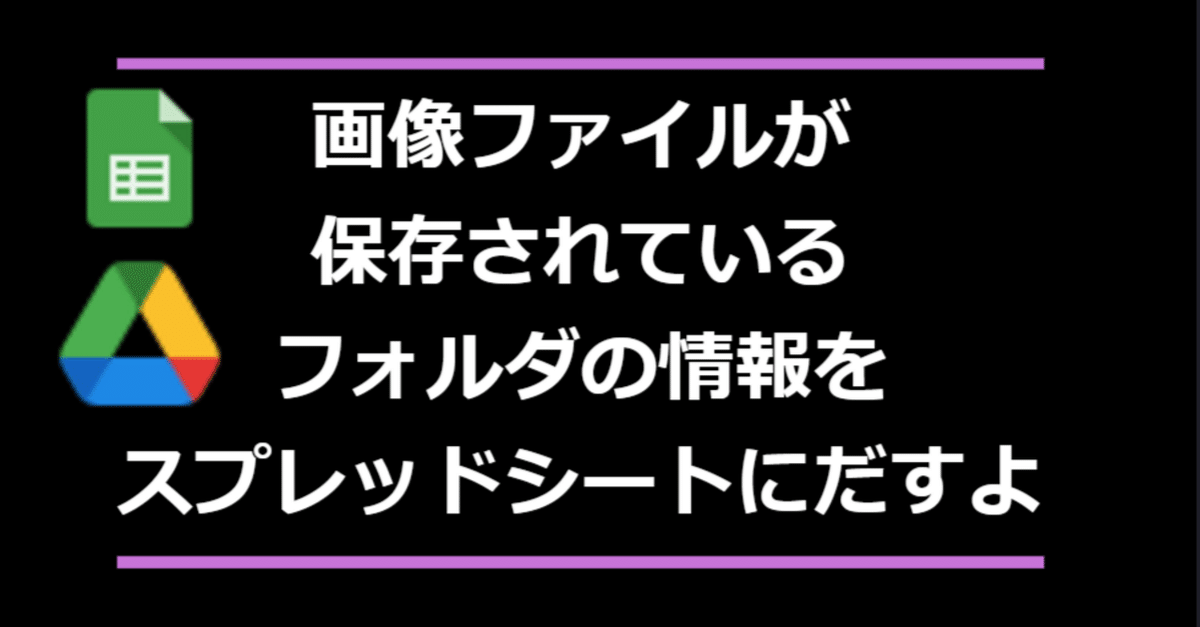
画像ファイルが保存されているフォルダの情報をスプレッドシートにだすGoogle Apps Scriptの例
この説明は、ChatGPTで作成しています。
以下は、提供されたスクリプト「フォルダ内の画像情報をスプレッドシートに出力するスクリプト」についての解説です。
このスクリプトの目的
このスクリプトは、指定したGoogle Driveのフォルダ内に保存されている画像ファイルの情報を取得し、それをGoogleスプレッドシートに記録するものです。画像の情報として、ファイル名、ファイルサイズ、画像のURL、解像度(幅×高さ)、プレビューを記録します。
動作の仕組み
フォルダとスプレッドシートの設定
スクリプトの初めに、画像が保存されているフォルダと、情報を記録するスプレッドシートを指定します。
FOLDER_ID と SPREADSHEET_ID に、それぞれフォルダIDとスプレッドシートIDを設定する必要があります。
フォルダID を取得するには:
Google Driveで対象のフォルダを開く。
URLのhttps://drive.google.com/drive/folders/の後ろに続く文字列がフォルダIDです。
スプレッドシートID を取得するには:
スプレッドシートをChromeで開く。
URLのhttps://docs.google.com/spreadsheets/d/の後ろに続く文字列がスプレッドシートIDです。
末尾に/editが含まれている場合、それを除いた部分がIDとなります。
ヘッダーを設定
スプレッドシートの1行目に、以下のヘッダーを設定します:
ファイル名
ファイルサイズ
URL
画素数
プレビュー
画像ファイルの取得
指定したフォルダ内のすべてのファイルを取得し、その中から画像ファイルのみを処理します。画像ファイルの判定は、ファイルのMIMEタイプ(image/で始まるかどうか)で行います。
画像の解像度取得
一時的にGoogle Docsに画像を貼り付けることで、画像の幅(横幅)と高さ(縦幅)を取得します。この処理は一時的なものなので、使用後はGoogle Docsファイルを削除します。
スプレッドシートへの出力
スプレッドシートに、画像の情報を1行ずつ記録します。各画像のダウンロードリンクを使用して、IMAGE関数で画像プレビューを挿入します。
列幅や行高さを自動調整して、見やすいレイアウトに整えます。
実行時間の記録
スクリプトの開始時刻と終了時刻を記録して、処理時間を表示します。
エラーハンドリング
スクリプト実行中にエラーが発生した場合は、エラーメッセージと発生時刻をポップアップで通知します。
使い方
Googleスプレッドシートを開き、「拡張機能」→「Apps Script」を選択してエディターを開きます。
提供されたスクリプトをエディターにコピー&ペーストします。
FOLDER_ID と SPREADSHEET_ID を正しい値に置き換えます。
スクリプトを保存し、実行します。
注意点
初めてスクリプトを実行する際は、Googleからの認証を求められる場合があります。指示に従い、権限を許可してください。
対象フォルダ内のファイルが多い場合、処理に時間がかかることがあります。
リンク
function getImageFilesInfo() {
// 開始時刻を記録
const startTime = Utilities.formatDate(new Date(), Session.getScriptTimeZone(), "HH:mm:ss");
try {
// 画像が格納されているフォルダと各種データを出力するスプレッドシートを指定する
const FOLDER_ID = '●●●ここにフォルダIDをいれてください●●●';
const SPREADSHEET_ID = '●●●ここにスプレッドシートIDをいれてください●●●';
const folder = DriveApp.getFolderById(FOLDER_ID);
const spreadsheet = SpreadsheetApp.openById(SPREADSHEET_ID);
const sheet = spreadsheet.getActiveSheet();
const headers = [['ファイル名', 'ファイルサイズ', 'URL', '画素数', 'プレビュー']];
sheet.getRange(1, 1, 1, 5).setValues(headers);
sheet.setRowHeight(2, 100);
const files = folder.getFiles();
const imageData = [];
let row = 2;
while (files.hasNext()) {
const file = files.next();
const mimeType = file.getMimeType();
if (mimeType.indexOf('image/') === 0) {
const tempDoc = DocumentApp.create('temp');
const blob = file.getBlob();
const image = tempDoc.getBody().appendImage(blob);
const width = image.getWidth();
const height = image.getHeight();
const fileUrl = file.getUrl();
const match = fileUrl.match(/https:\/\/drive\.google\.com\/file\/d\/(.*)\/view(.*)/);
const fileId = match ? match[1] : null;
const downloadUrl = fileId ? `https://drive.google.com/uc?export=download&id=${fileId}` : '';
// imageDataには'プレビュー'列を含めない
imageData.push([
file.getName(),
formatFileSize(file.getSize()),
file.getUrl(),
`${width} x ${height}`
]);
// IMAGE関数を別途設定
if (downloadUrl) {
sheet.getRange(row, 5).setFormula(`=IMAGE("${downloadUrl}", 2)`);
}
DriveApp.getFileById(tempDoc.getId()).setTrashed(true);
row++;
}
}
if (imageData.length > 0) {
// 最初の4列のみデータを設定
sheet.getRange(2, 1, imageData.length, 4).setValues(imageData);
}
sheet.autoResizeColumns(1, 4);
sheet.setColumnWidth(5, 150);
// 完了時刻を記録(ミリ秒単位)
const endTime = Utilities.formatDate(new Date(), Session.getScriptTimeZone(), "HH:mm:ss");
SpreadsheetApp.getUi().alert(
'実行が完了しました!\n' +
`開始時間:${startTime}\n` +
`終了時間:${endTime}`
);
// エラー処理
} catch (error) {
// エラー発生時刻を記録
const errorTime = Utilities.formatDate(new Date(), Session.getScriptTimeZone(), "HH:mm:ss");
// エラーメッセージを表示
SpreadsheetApp.getUi().alert(
'エラー発生のため実行中断しました!\n' +
`所要時間:${errorTime}\n` +
`エラー内容:${error.toString()}`
);
}
}
// ファイルサイズフォーマット用の関数(既存のまま)
function formatFileSize(bytes) {
if (bytes < 1024) return bytes + ' B';
if (bytes < 1024 * 1024) return (bytes / 1024).toFixed(2) + ' KB';
if (bytes < 1024 * 1024 * 1024) return (bytes / (1024 * 1024)).toFixed(2) + ' MB';
return (bytes / (1024 * 1024 * 1024)).toFixed(2) + ' GB';
}
ハッシュタグ
#GoogleAppsScript #GoogleDrive #スプレッドシート #画像情報 #プレビュー表示 #フォルダ内検索 #ファイルサイズ取得 #解像度取得 #自動化 #スクリプト初心者 #エラー処理 #スクリプト実行 #GASチュートリアル #GoogleDocs #画像一覧作成 #スクリプト活用 #AppsScript活用 #初心者向けGAS #ファイル管理 #DriveAPI
英訳
This explanation is created using ChatGPT.
Below is an explanation of the provided script "Script to Output Image Information in a Folder to a Spreadsheet."
Purpose of this Script
This script retrieves information about image files stored in a specified Google Drive folder and records it in a Google Spreadsheet. The recorded information includes file name, file size, image URL, resolution (width x height), and a preview.
How it Works
Setting the Folder and Spreadsheet
At the beginning of the script, specify the folder containing the images and the spreadsheet where the information will be recorded.
You need to set FOLDER_ID and SPREADSHEET_ID with the respective folder and spreadsheet IDs.
To get the folder ID:
Open the target folder in Google Drive.
The folder ID is the string following https://drive.google.com/drive/folders/ in the URL.
To get the spreadsheet ID:
Open the spreadsheet in Chrome.
The spreadsheet ID is the string following https://docs.google.com/spreadsheets/d/ in the URL.
Exclude /edit at the end if it is present.
Setting the Headers
The script sets the following headers in the first row of the spreadsheet:
File Name
File Size
URL
Resolution
Preview
Retrieving Image Files
It retrieves all files in the specified folder and processes only image files. Image files are identified by their MIME type (those starting with image/).
Getting Image Resolution
It temporarily uploads the image to Google Docs to get the image's width and height. This temporary file is deleted afterward.
Outputting to Spreadsheet
The script writes the image information row by row into the spreadsheet. The IMAGE function is used to insert image previews using their download links.
The column widths and row heights are automatically adjusted for better visibility.
Recording Execution Time
The script records and displays the start and end times of the process to show how long it took.
Error Handling
If an error occurs during execution, a popup displays the error message and the time of occurrence.
Usage
Open a Google Spreadsheet, go to "Extensions" → "Apps Script," and open the editor.
Copy and paste the provided script into the editor.
Replace FOLDER_ID and SPREADSHEET_ID with the correct values.
Save and run the script.
Notes
When running the script for the first time, Google may prompt you for authorization. Follow the instructions to grant the necessary permissions.
Processing may take some time if there are many files in the target folder.
Links
Hashtags
#GoogleAppsScript #GoogleDrive #Spreadsheet #ImageInfo #PreviewDisplay #FolderSearch #FileSizeRetrieval #ResolutionRetrieval #Automation #ScriptBeginner #ErrorHandling #ScriptExecution #GASTutorial #GoogleDocs #ImageListCreation #ScriptUtilization #AppsScriptUtilization #GASForBeginners #FileManagement #DriveAPI