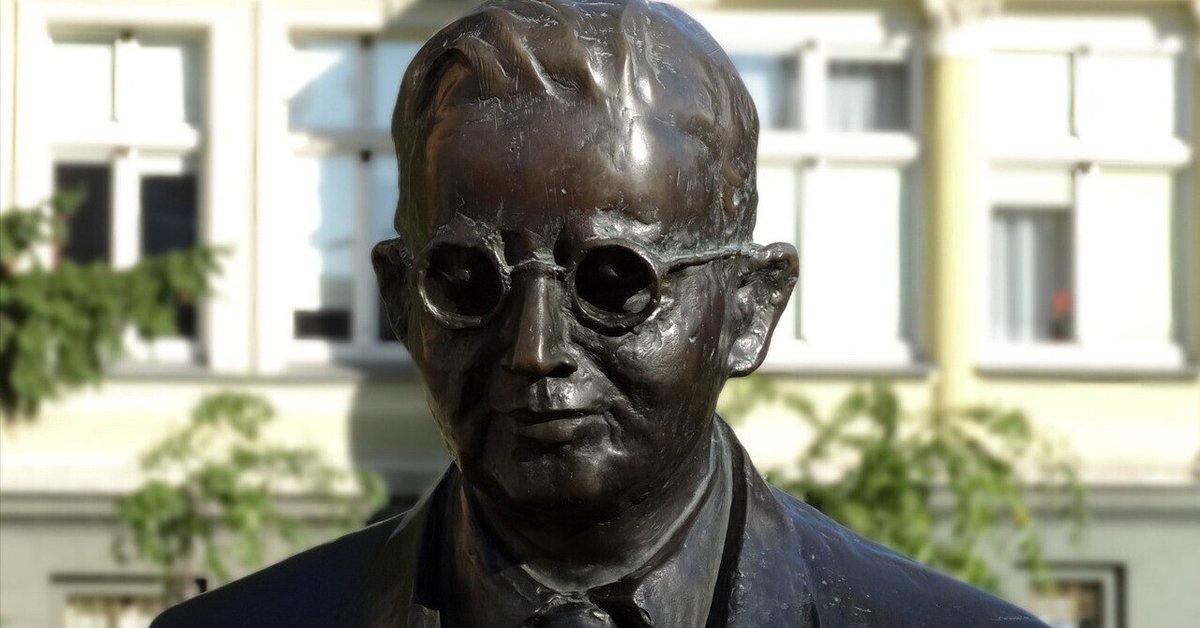
⑥エニグマ暗号機のローター結線解読プロジェクト 機械学習
業務上の必要性から機械学習を勉強していたのだが、ふと思いついたのでClaudeに相談してみる。できれば、趣味でやる事が仕事になればより面白いのでね。
いわゆるバッチ学習的な機械学習しか知らなければこんな発想は出てこない。オンライン学習や強化学習の様な動的に動く仕組みを知っているとこんな突拍子も無いアイデアが出てくる。
まずは、教師データではなくてもっと直接的な初期値を与える方法はあるだろうか?ここで、通常の機械学習からは離れる。
機械学習に初期値としてルールを覚えさせることはできますか?
例えば、対称群。
はい、機械学習モデルに初期値やドメイン知識としてルールを組み込むことは可能です。対称群のような数学的な構造を例に説明させていただきます。
これを実現する主なアプローチとしては:
1. アーキテクチャ設計による組み込み
- モデルの構造自体に対称性を持たせる
- 例: Group Equivariant Convolutional Neural Networks (G-CNNs)は、回転や並進などの対称性を持つように設計されています
2. 損失関数による制約
```python
def symmetry_loss(predictions):
# 対称性を満たさない予測に対してペナルティを与える
symmetry_violation = calculate_symmetry_violation(predictions)
return symmetry_violation_penalty * symmetry_violation
```
3. データ拡張
```python
def augment_with_symmetry(data):
augmented_data = []
for x in data:
# 対称群の操作を適用した変換を追加
for transformation in symmetry_group:
augmented_data.append(transformation(x))
return augmented_data
```
4. 事前学習による知識注入
- 対称性を持つデータで事前学習を行う
- その後、特定のタスクに対して微調整(fine-tuning)を行う
この中でどの側面に特に興味がありますか?より具体的な実装例や、特定の対称群に関する例を示すことができます。
これは… ペナルティと書いてるので強化学習みたいなものだろうか。
まずは気にせず進む。
エニグマ暗号は対称群が、1文字変換するごとにシフトすることで成り立っています。
その対称群は初期値のセットにより成り立ちますが、それをオンライン予測のように得られた暗号文のクリブを一つ一つ覚えさせていくことで、多数有る選択肢から絞り込んでいけないですか?
数理的な方法ではどうしても組み合わせ問題になって効率が悪いので。
面白いアプローチですね。エニグマ暗号の解読を機械学習で効率化する方法を考えてみましょう。
このアプローチのポイントは以下の通りです:
1. 確率的学習
- 各ローター位置での文字変換の確率分布を学習
- 新しいクリブが得られるたびに確率を更新
- 組み合わせ爆発を避けるため、確率的な推論を使用
2. パターン認識
- ローターの周期性(26文字)を利用
- 各位置での変換パターンを独立して学習
- 文脈情報も考慮可能
3. 適応的学習
- オンラインでクリブを追加可能
- 確信度の高い変換から優先的に解読
- 部分的な知識でも解読を試行可能
使用例:
```python
decoder = EnigmaMLDecoder()
# クリブで学習
decoder.train_with_crib("HELLO", "KIPVT", 0)
decoder.train_with_crib("WORLD", "RNZXM", 5)
# 新しい暗号文の解読を試みる
decoded = decoder.decode_message("KIPVTRNZXM", 0)
```
このアプローチの利点:
- 厳密な総当たりよりも効率的
- 部分的な情報でも解読可能
- 新しい情報を継続的に学習可能
- 確率的なアプローチにより、複数の候補から最適解を選択可能
さらに改良できる点:
- 言語モデルとの組み合わせ
- 文脈情報の活用
- 並列処理による高速化
import numpy as np
from typing import List, Dict, Tuple
class EnigmaMLDecoder:
def __init__(self, alphabet_size: int = 26):
self.alphabet_size = alphabet_size
self.known_transformations = {} # 既知の変換を保存
self.position_patterns = {} # 各ローター位置でのパターン
self.probability_matrix = np.ones((alphabet_size, alphabet_size)) / alphabet_size
def update_probabilities(self, plaintext: str, ciphertext: str, position: int) -> None:
"""新しいクリブ(既知の平文と暗号文のペア)から確率を更新"""
for p, c in zip(plaintext, ciphertext):
p_idx = ord(p) - ord('A')
c_idx = ord(c) - ord('A')
# この位置での変換確率を更新
position_key = position % 26
if position_key not in self.position_patterns:
self.position_patterns[position_key] = np.ones((self.alphabet_size, self.alphabet_size))
# この変換の確率を上げる
self.position_patterns[position_key][p_idx, c_idx] += 1
# 正規化
self.position_patterns[position_key] /= self.position_patterns[position_key].sum(axis=1, keepdims=True)
def predict_rotor_settings(self, ciphertext: str, position: int) -> List[Tuple[str, float]]:
"""現在の位置での最も可能性の高いローター設定を予測"""
position_key = position % 26
if position_key not in self.position_patterns:
return [('Unknown', 0.0)]
# 各文字の変換確率を計算
probabilities = []
pattern = self.position_patterns[position_key]
for i in range(self.alphabet_size):
prob = pattern[i].max()
most_likely_char = chr(pattern[i].argmax() + ord('A'))
probabilities.append((most_likely_char, prob))
# 確率で降順ソート
return sorted(probabilities, key=lambda x: x[1], reverse=True)
def decode_message(self, ciphertext: str, start_position: int) -> str:
"""暗号文の解読を試みる"""
decoded = []
position = start_position
for c in ciphertext:
predictions = self.predict_rotor_settings(c, position)
decoded.append(predictions[0][0]) # 最も確率の高い文字を選択
position += 1
return ''.join(decoded)
def train_with_crib(self, crib_text: str, cipher_text: str, position: int) -> None:
"""クリブ(既知の平文と暗号文のペア)で学習"""
self.update_probabilities(crib_text, cipher_text, position)
この確率的学習とはベイズ深層学習ですか?
いいえ、今の例は単純なベイズ推定に近いものです。ただし、ご指摘の通りベイズ深層学習を使うとより高度な推論が可能になります。
エニグマの文脈で、ベイズ深層学習を適用する方法を説明させていただきます:
1. ベイズ深層学習の利点:
- モデルの不確実性を明示的に扱える
- 事前知識(対称群の性質など)を事前分布として組み込める
- 少ないデータでも学習可能
以下に、ベイズ深層学習を用いた実装例を示します:
このベイズ深層学習アプローチの特徴:
1. 不確実性のモデル化
- 重みの分布を学習
- 予測時に複数のサンプリングを行い、不確実性を考慮
- 確信度の低い予測を識別可能
2. 正則化効果
- KLダイバージェンスによる自動的な正則化
- 過学習の抑制
- より汎化性能の高いモデルの学習
3. 事前知識の組み込み
- 対称群の性質を事前分布として組み込み可能
- ローター位置の周期性を位置エンコーディングで表現
- 文字変換の制約を構造化
4. 拡張性
- より複雑なアーキテクチャへの応用が可能
- 言語モデルとの統合も容易
- マルチタスク学習への対応
従来の単純なベイズ推定と比べた利点:
- より複雑なパターンの学習が可能
- 文脈情報の活用
- 階層的な特徴表現の学習
- 不確実性のより精密なモデル化
ベイズ深層学習の方が、エニグマの複雑な暗号化プロセスをより効果的にモデル化できると考えられます。特に、多くのクリブが得られない状況での性能が期待できます。
import numpy as np
from typing import List, Dict, Tuple
class EnigmaMLDecoder:
def __init__(self, alphabet_size: int = 26):
self.alphabet_size = alphabet_size
self.known_transformations = {} # 既知の変換を保存
self.position_patterns = {} # 各ローター位置でのパターン
self.probability_matrix = np.ones((alphabet_size, alphabet_size)) / alphabet_size
def update_probabilities(self, plaintext: str, ciphertext: str, position: int) -> None:
"""新しいクリブ(既知の平文と暗号文のペア)から確率を更新"""
for p, c in zip(plaintext, ciphertext):
p_idx = ord(p) - ord('A')
c_idx = ord(c) - ord('A')
# この位置での変換確率を更新
position_key = position % 26
if position_key not in self.position_patterns:
self.position_patterns[position_key] = np.ones((self.alphabet_size, self.alphabet_size))
# この変換の確率を上げる
self.position_patterns[position_key][p_idx, c_idx] += 1
# 正規化
self.position_patterns[position_key] /= self.position_patterns[position_key].sum(axis=1, keepdims=True)
def predict_rotor_settings(self, ciphertext: str, position: int) -> List[Tuple[str, float]]:
"""現在の位置での最も可能性の高いローター設定を予測"""
position_key = position % 26
if position_key not in self.position_patterns:
return [('Unknown', 0.0)]
# 各文字の変換確率を計算
probabilities = []
pattern = self.position_patterns[position_key]
for i in range(self.alphabet_size):
prob = pattern[i].max()
most_likely_char = chr(pattern[i].argmax() + ord('A'))
probabilities.append((most_likely_char, prob))
# 確率で降順ソート
return sorted(probabilities, key=lambda x: x[1], reverse=True)
def decode_message(self, ciphertext: str, start_position: int) -> str:
"""暗号文の解読を試みる"""
decoded = []
position = start_position
for c in ciphertext:
predictions = self.predict_rotor_settings(c, position)
decoded.append(predictions[0][0]) # 最も確率の高い文字を選択
position += 1
return ''.join(decoded)
def train_with_crib(self, crib_text: str, cipher_text: str, position: int) -> None:
"""クリブ(既知の平文と暗号文のペア)で学習"""
self.update_probabilities(crib_text, cipher_text, position)
ベイズ深層学習以外にこの様なアプローチに使えるロジックはなんですか?
エニグマの暗号解読のような順次的なパターン学習には、以下のようなアプローチが考えられます:
1. 強化学習アプローチ
- 解読を探索問題として扱い、正しい解読が得られた際に報酬を与える
- 部分的な成功(既知のクリブとの一致など)にも報酬を設定可能
import numpy as np
from collections import defaultdict
class RLEnigmaDecoder:
def __init__(self, alphabet_size=26, learning_rate=0.1, discount_factor=0.9, epsilon=0.1):
self.alphabet_size = alphabet_size
self.lr = learning_rate
self.gamma = discount_factor
self.epsilon = epsilon
# Q値テーブル: (現在の状態, アクション) -> 期待報酬
self.Q = defaultdict(lambda: np.zeros(alphabet_size))
# 状態は (現在位置, 直前のn文字) のタプル
self.state_memory = 3 # 直前3文字を記憶
def get_state(self, position, previous_chars):
"""状態の取得"""
return (position % 26, tuple(previous_chars[-self.state_memory:]))
def choose_action(self, state):
"""ε-greedy戦略による行動選択"""
if np.random.random() < self.epsilon:
return np.random.randint(self.alphabet_size)
return np.argmax(self.Q[state])
def update(self, state, action, reward, next_state):
"""Q学習によるアップデート"""
best_next_action = np.argmax(self.Q[next_state])
td_target = reward + self.gamma * self.Q[next_state][best_next_action]
td_error = td_target - self.Q[state][action]
self.Q[state][action] += self.lr * td_error
def decode(self, ciphertext, known_pairs=None):
"""暗号文の解読"""
position = 0
previous_chars = [''] * self.state_memory
decoded = []
for cipher_char in ciphertext:
state = self.get_state(position, previous_chars)
action = self.choose_action(state)
decoded_char = chr(action + ord('A'))
# 報酬の計算
reward = 0
if known_pairs and position < len(known_pairs):
if decoded_char == known_pairs[position]:
reward = 1
else:
reward = -0.1
# 次の状態の準備
next_previous = previous_chars[1:] + [decoded_char]
next_state = self.get_state(position + 1, next_previous)
# Q値の更新
self.update(state, action, reward, next_state)
# 状態の更新
previous_chars = next_previous
position += 1
decoded.append(decoded_char)
return ''.join(decoded)
def train(self, training_data, episodes=1000):
"""既知の平文-暗号文ペアで学習"""
for _ in range(episodes):
for plaintext, ciphertext in training_data:
self.decode(ciphertext, known_pairs=plaintext)
2. 隠れマルコフモデル(HMM)アプローチ
- 暗号化プロセスを隠れ状態の遷移としてモデル化
- ローター位置を隠れ状態として扱う
# HMMを使用した例(概念的なコード)
from hmmlearn import hmm
class HMMEnigmaDecoder:
def __init__(self):
self.model = hmm.MultinomialHMM(n_components=26) # ローター位置
def train(self, observations, states):
self.model.fit(observations, states)
def decode(self, ciphertext):
# Viterbiアルゴリズムで最も可能性の高い状態系列を推定
states = self.model.decode(ciphertext)
return self.convert_states_to_plaintext(states)
3. 遺伝的アルゴリズムアプローチ
- 可能な設定の組み合わせを染色体として表現
- 適合度関数として解読文の言語的自然さを使用
4. LSTM/Transformerアプローチ
- 系列データとしてモデル化
- 文脈情報を活用した予測
- アテンションメカニズムで重要なパターンを学習
5. モンテカルロツリー探索(MCTS)
- 解読の可能性を木構造で探索
- 有望な分岐を優先的に探索
6. アンサンブル学習
- 複数のアプローチを組み合わせて使用
- 各モデルの予測を重み付けで統合
class HybridEnigmaDecoder:
def __init__(self):
self.rl_decoder = RLEnigmaDecoder()
self.bayes_decoder = BayesianEnigmaDecoder()
self.hmm_decoder = HMMEnigmaDecoder()
def decode(self, ciphertext):
# 各デコーダーの予測を取得
rl_pred = self.rl_decoder.decode(ciphertext)
bayes_pred = self.bayes_decoder.decode(ciphertext)
hmm_pred = self.hmm_decoder.decode(ciphertext)
# 信頼度スコアに基づいて予測を統合
final_prediction = self.combine_predictions([
(rl_pred, self.get_rl_confidence()),
(bayes_pred, self.get_bayes_confidence()),
(hmm_pred, self.get_hmm_confidence())
])
return final_prediction
各アプローチの特徴:
1. 強化学習
- 試行錯誤を通じた学習
- 長期的な報酬を考慮可能
- 探索と活用のバランス
2. HMM
- 状態遷移の確率的モデル化
- 計算効率が良い
- 解釈可能性が高い
3. 遺伝的アルゴリズム
- 広い探索空間に対応
- 局所解に陥りにくい
- パラメータ調整が必要
4. LSTM/Transformer
- 長距離依存関係の学習
- 大規模データでの学習が可能
- 計算コストが高い
5. MCTS
- 効率的な探索
- 不確実性への対応
- メモリ使用量が多い
これらのアプローチは、問題の特性や利用可能なデータ量に応じて使い分けることができます。
気になって「機械学習でパターン認識をする技術は何だ?」と聞いたら、教師あり学習と答えてきた。まー、確かにそうなんだけど…
ここで最後の質問。手元にある機械学習の資料のタイトルを入れて、有効な物を訊いてみる。
エニグマ暗号機の解読を機械学習で行います。どれが有効ですか?
・オンライン学習
・オンライン予測
・強化学習
・ノンパラメトリックベイズ
・ベイズ深層学習
・グラフィカルモデル
・統計的因果探索
・関係データ学習
・転移学習
・グラフニューラルネットワーク
・ガウス過程
・最適輸送
エニグマ暗号機の解読を機械学習で行う場合、以下のアプローチが特に有効だと考えられます:
1. グラフィカルモデル
- エニグマの機械的な構造(ローター、リフレクターなど)をグラフ構造として表現できる
- 確率的な依存関係をモデル化できる
- 暗号化プロセスの階層的な構造を表現しやすい
2. ベイズ深層学習
- 不確実性を考慮しながら、複雑なパターンを学習できる
- 事前知識(既知の平文-暗号文ペアなど)を組み込める
- モデルの解釈性を保ちつつ、高い表現力を得られる
3. 強化学習
- 解読のための探索を効率的に行える
- 試行錯誤を通じて最適な解読戦略を学習できる
- 部分的な成功(特定の設定の推定など)から学習を進められる
あまり効果的でないと考えられるアプローチ:
- オンライン学習/予測:
エニグマは固定的なルールに基づくため、逐次的な学習の利点が少ない
- ガウス過程:暗号システムの離散的な性質との相性があまり良くない
- 最適輸送:暗号文と平文の間の変換を直接的に扱うのは難しい
補足として、これらの手法を組み合わせることで、より効果的な解読システムを構築できる可能性があります。例えば、グラフィカルモデルの構造の中でベイズ深層学習を用い、強化学習で探索を効率化するといったアプローチが考えられます。
実際の解読では、既知の平文-暗号文ペアや、言語の統計的性質なども重要な手がかりとなるため、これらの情報を効果的に活用できるモデル設計が重要になります。