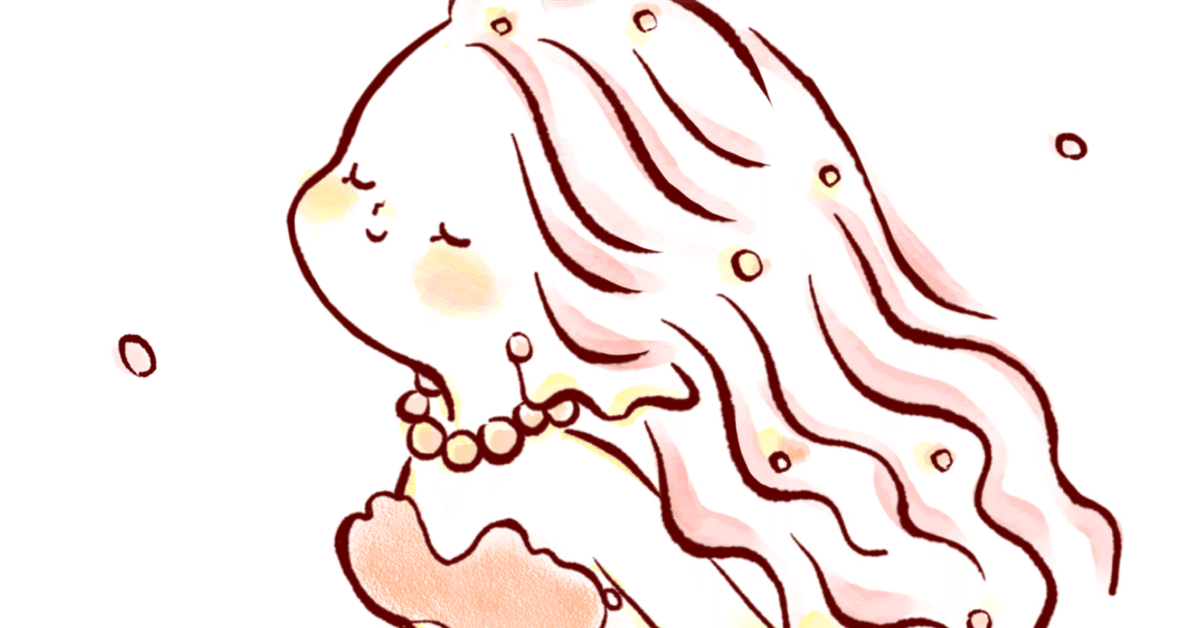
⑤可動ノードとは? 物流ゲーミフィケーション
④で出てきた「可動ノード」とは?これは気になる。
問題の本質はここなんだよな。突っ込んでみる。
要は、「グラフ深層学習」に出てきたダイナミックグラフの事か。
ChatGPT 4oが上限なので、Claudeに話を振る。
ゼロから始めるのか、大変だな…
「可動ノード」という概念を数学的にコード化したいのですが、
実際にコード化する前のモデリングを行います。
対象は、N対N配車を時系列上で展開する実例です。
配車はブレイド群のテンソル積であり、
ブレイド指数を最小化する事が目的です。
イメージは、ビルがあって高層階の床面に地図が描かれています。
高層階が8時の地図、その下の階が9時...
拠点間で物流活動がある訳ですが、
それを異なる階への矢印を描画によって。
1時間で移動できる場合はすぐ下の階と矢印が繋がりますが、
遠くになると2時間以上かかるので、ある階をスキップして線を引きます。
この場合、着時刻という概念があるのですが、
むしろ着時刻の設定が無い場合に
ブレイド指数を最小化するのに
着時刻をずらさないといけないのが難しいのです。
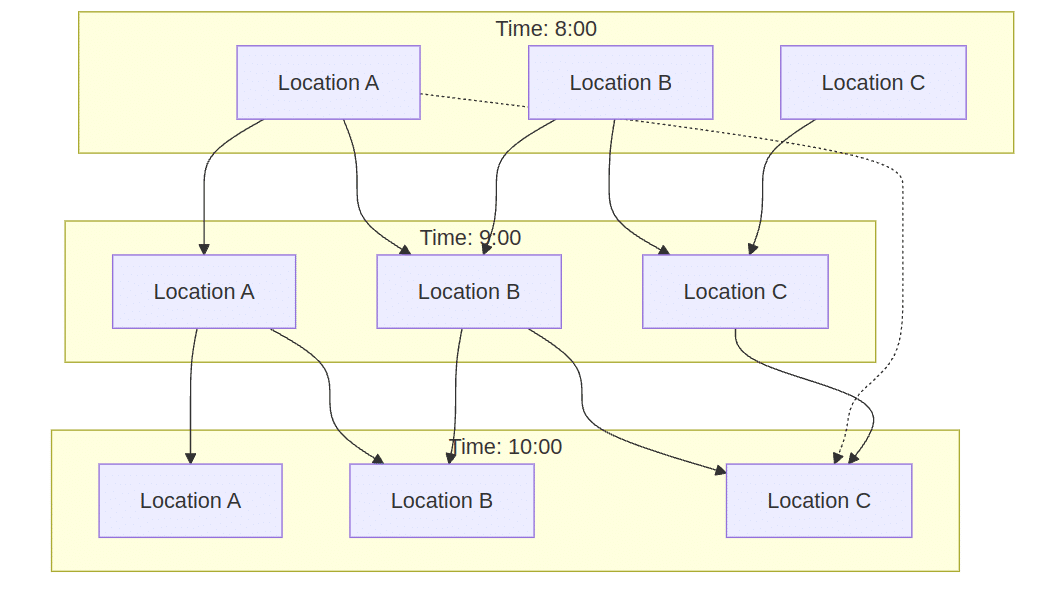
いきなり、こういうのを返してくるから、Claude先生は凄いんだよなぁ…
結論出ちゃうから、むしろ最初からは使わない様にしているくらい。
こういうのを、インタラクティブに作りたいんだよなぁ。
(各階が地図になってるやつ)
今見てると、同じノードが時系列で繋がれているのは良いんだけど、これは実線じゃない方が良いんだよな、細かい話。後で実装する時に直そう。
うん、これはPyGameだな。
マーメイド形式では、こう。
graph TD
subgraph T8[Time: 8:00]
A8[Location A]
B8[Location B]
C8[Location C]
end
subgraph T9[Time: 9:00]
A9[Location A]
B9[Location B]
C9[Location C]
end
subgraph T10[Time: 10:00]
A10[Location A]
B10[Location B]
C10[Location C]
end
%% 1時間の移動
A8 --> B9
B8 --> C9
A9 --> B10
B9 --> C10
%% 2時間の移動
A8 -.-> C10
%% 待機
A8 --> A9
B8 --> B9
C8 --> C9
A9 --> A10
B9 --> B10
C9 --> C10
なるほど…
そして2DではPyGameだし、3DではPanda3Dか…
そして、すぐにClaudeはトークン上限に達してしまう(笑)
最後に、Geminiに訊くか。
すげーな、ごっついコード書いてくるな…(ひいちゃう位)
N対N配車で、こういうモデルを作って(PyGame?)
インタラクティブに操作するゲームみたいなアプリを作ってみたいんですが
<上のマーメイドをここに書く>
N対N配車を題材としたインタラクティブなゲームアプリ、面白いですね!Pygameを使って実現するアイデア、大いに賛成です。以下に、具体的なステップと実装のポイント、そしてゲームとしての拡張アイデアをまとめました。
1. 基本的なゲームの構造
マップの表現:
与えられたMermaid形式の図を参考に、時間経過とともに移動するノード(Location)をPygameで表現します。
各Locationは円や矩形で表し、時間帯ごとに異なるレイヤーに配置するのが良いでしょう。
ノード間の移動は、線や矢印で表示します。
車両の表現:
プレイヤーが操作する車両(タクシーやトラックなど)を、アイコンや画像で表現します。
車両は、マウスドラッグやキー操作で移動させられるようにします。
乗客の表現:
各Locationに、時間帯ごとに乗車を希望する乗客(アイコンや数字で表現)を配置します。
乗客は、特定の目的地(Location)へ移動したいという要求を持つようにします。
時間経過:
一定時間ごとにゲーム時間を進めるタイマーを実装します。
時間経過に伴い、乗客や車両の位置を更新します。
配車ルール:
N対Nの配車ルールを実装します。
例:
最も近い乗客を優先的に配車する。
目的地が近い乗客を優先的に配車する。
特定の条件(VIP顧客など)を考慮する。
複数の車両で、複数の乗客を目的地に配送する。
スコア:
配車を成功させるたびにスコアを加算します。
効率的な配車や、目的地到着の早さなどを考慮して、スコアを調整するのも良いでしょう。
2. Pygameでの実装
ポイント描画:
pygame.drawモジュールを使って、Locationや車両、乗客を画面に描画します。
時間帯ごとのレイヤー管理には、pygame.Surfaceを使うと便利です。
マウス操作:
pygame.event.get()でマウスイベントを捕捉します。
マウスドラッグによる車両移動は、マウスカーソルの座標と車両の座標を同期させることで実現できます。
キー操作:
pygame.event.get()でキーイベントを捕捉します。
キー操作で車両を選択したり、配車指示を出すようにします。
時間管理:
pygame.time.Clockを使って、ゲームのフレームレートを一定に保ちます。
タイマーイベントを使い、時間経過をシミュレーションします。
オブジェクト指向プログラミング:
Location、車両、乗客をそれぞれクラスとして定義し、オブジェクト指向でゲームを構築すると、コードが整理しやすくなります。
3. ゲームとしての拡張アイデア
配車アルゴリズムの選択:
プレイヤーが複数の配車アルゴリズムを選択できるようにします。
アルゴリズムによって、効率やスコアがどう変化するかを体験できるようにします。
マップの拡張:
Locationの数や種類を増やします。
渋滞や道路工事などの要素を導入して、配車難易度を上げます。
時間帯によって、乗客の数が変化するようにします。
乗客の多様性:
移動距離や時間、緊急度などの異なる要求を持つ乗客を登場させます。
VIP顧客や、特定の車両でしか運べない荷物を登場させるなど。
レベル制:
ゲームクリア条件を達成すると、次のレベルに進めるようにします。
レベルが上がるごとに、難易度を上げるようにします。
リプレイ:
プレイ結果を録画し、後から見直せるようにします。
上手なプレイを参考に、配車スキルを向上させます。
AIによる自動配車:
プレイヤーの代わりに、AIが自動で配車を行うモードを実装します。
AIの配車戦略を参考に、自分の配車スキルを向上させます。
マルチプレイ:
複数人で協力して、配車を効率化するモードを実装します。
競争要素を取り入れ、スコアを競い合うモードも面白いでしょう。
4. 実装例(簡単な概要)
import pygame
import random
# Pygameの初期化
pygame.init()
# 画面サイズ
WIDTH = 800
HEIGHT = 600
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("N対N配車ゲーム")
# 色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# Locationクラス
class Location:
def __init__(self, x, y, name):
self.x = x
self.y = y
self.name = name
self.radius = 20
self.passengers = []
def draw(self):
pygame.draw.circle(screen, WHITE, (self.x, self.y), self.radius)
font = pygame.font.Font(None, 20)
text = font.render(self.name, True, BLACK)
text_rect = text.get_rect(center=(self.x, self.y))
screen.blit(text, text_rect)
# 乗客の表示
for i, passenger in enumerate(self.passengers):
pygame.draw.rect(screen, BLUE, (self.x + self.radius, self.y - self.radius + (i*20),10,10))
def add_passenger(self, destination):
self.passengers.append(Passenger(self, destination))
# Passengerクラス
class Passenger:
def __init__(self, start, destination):
self.start = start
self.destination = destination
# Vehicleクラス
class Vehicle:
def __init__(self, x, y, max_passenger=2):
self.x = x
self.y = y
self.width = 15
self.height = 25
self.speed = 5
self.is_moving = False
self.destination = None
self.passengers = []
self.max_passenger = max_passenger
def draw(self):
pygame.draw.rect(screen, RED, (self.x, self.y, self.width, self.height))
# 乗客表示
for i, passenger in enumerate(self.passengers):
pygame.draw.rect(screen, GREEN, (self.x + self.width, self.y + (i*10), 5,5))
def move(self, dest_x, dest_y):
# 移動先の設定
dx = dest_x - self.x
dy = dest_y - self.y
distance = (dx ** 2 + dy ** 2) ** 0.5
# 移動の処理
if distance > 0:
move_x = dx / distance * self.speed
move_y = dy / distance * self.speed
self.x += move_x
self.y += move_y
if distance < self.speed:
self.x = dest_x
self.y = dest_y
self.is_moving = False
def assign_destination(self, location):
self.destination = location
self.is_moving = True
def add_passenger(self, passenger):
if len(self.passengers) < self.max_passenger:
self.passengers.append(passenger)
return True
else:
return False
def remove_passenger(self, passenger):
self.passengers.remove(passenger)
# Locationの作成
locations = {}
locations["A8"] = Location(100, 100, "A")
locations["B8"] = Location(300, 100, "B")
locations["C8"] = Location(500, 100, "C")
locations["A9"] = Location(100, 300, "A")
locations["B9"] = Location(300, 300, "B")
locations["C9"] = Location(500, 300, "C")
locations["A10"] = Location(100, 500, "A")
locations["B10"] = Location(300, 500, "B")
locations["C10"] = Location(500, 500, "C")
# 移動ルート
routes = {
("A8", "B9"),
("B8", "C9"),
("A9", "B10"),
("B9", "C10"),
("A8","C10")
}
wait_routes = {
("A8","A9"),
("B8", "B9"),
("C8","C9"),
("A9", "A10"),
("B9", "B10"),
("C9", "C10"),
}
# 時間管理
time = 8 #初期時間
time_interval = 60
current_location_names = [f"{c}{time}" for c in ["A","B","C"]]
next_location_names = [f"{c}{time + 1}" for c in ["A","B","C"]]
tick_time = 0
#Vehicleの作成
vehicle = Vehicle(50, 100)
# ゲームループ
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEBUTTONDOWN:
mouse_x, mouse_y = event.pos
# Locationのクリック判定
for key, location in locations.items():
if location.x - location.radius < mouse_x < location.x + location.radius and \
location.y - location.radius < mouse_y < location.y + location.radius:
# 車両が移動中でなければ、移動先を設定
if vehicle.is_moving == False and any(loc == key for loc in current_location_names):
vehicle.assign_destination(location)
# 乗客の乗車処理
if location.passengers:
for passenger in location.passengers:
if vehicle.add_passenger(passenger):
location.passengers.remove(passenger)
break
# 描画
screen.fill(BLACK)
# Locationの描画
for key, location in locations.items():
location.draw()
# ルートの描画
for start_key, end_key in routes:
start_loc = locations.get(start_key)
end_loc = locations.get(end_key)
if start_loc and end_loc:
pygame.draw.line(screen, WHITE, (start_loc.x, start_loc.y), (end_loc.x, end_loc.y))
# 待機ルートの描画
for start_key, end_key in wait_routes:
start_loc = locations.get(start_key)
end_loc = locations.get(end_key)
if start_loc and end_loc:
pygame.draw.line(screen, WHITE, (start_loc.x, start_loc.y), (end_loc.x, end_loc.y), 2)
#車両の描画
vehicle.draw()
# 車両の移動
if vehicle.is_moving and vehicle.destination:
vehicle.move(vehicle.destination.x, vehicle.destination.y)
# 到着時処理
if vehicle.x == vehicle.destination.x and vehicle.y == vehicle.destination.y:
# 乗客の降車
if vehicle.passengers:
for passenger in vehicle.passengers:
if passenger.destination.name == vehicle.destination.name:
vehicle.remove_passenger(passenger)
# 時間経過処理
tick_time += 1
if tick_time % time_interval == 0:
time += 1
tick_time = 0
current_location_names = next_location_names
next_location_names = [f"{c}{time + 1}" for c in ["A","B","C"]]
for location_name in current_location_names:
current_location = locations.get(location_name)
# ランダムな乗客追加
if current_location:
destination_list = [loc for loc in locations.values() if loc != current_location]
if destination_list:
destination = random.choice(destination_list)
current_location.add_passenger(destination)
# 時間表示
font = pygame.font.Font(None, 36)
text = font.render(f"Time: {time}:00", True, WHITE)
screen.blit(text, (10, 10))
pygame.display.flip()
# Pygameの終了
pygame.quit()
5. 次のステップ
上記のコードをベースに、まずは基本的な描画とマウス操作を実装してみてください。
その後、車両の移動や乗客の生成、配車ルールなど、段階的に機能を拡張していくと良いでしょう。
不明な点や実装で困ったことがあれば、お気軽にご質問ください。
このアイデアが、あなたのゲーム開発の一助となれば幸いです!
頑張ってください!
配車において時系列のグラフ構造を扱うのに「可動ノード」という概念を教えてもらいました。
これはダイナミックグラフと同じ概念ですか?
はい、ご認識の通り、「可動ノード」という概念は、ダイナミックグラフ(動的グラフ)と非常に近い概念であると言えます。特に配車のような時系列データが関わる分野では、ほぼ同じ意味で使われることが多いでしょう。
それぞれの概念について、もう少し詳しく見ていきましょう。
ダイナミックグラフ(動的グラフ)
定義: グラフ構造が時間とともに変化するグラフのことです。ノードやエッジの追加、削除、属性の変化などが時間経過に伴って起こります。
特徴:
時間変化をモデル化できるため、現実世界の複雑なシステムや現象を表現するのに適しています。
ソーシャルネットワーク、交通ネットワーク、生物ネットワークなど、様々な分野で活用されています。
時間経過に伴うグラフ構造の変化を分析することで、興味深い洞察が得られます。
例:
ソーシャルネットワークにおける友達関係の変動
道路ネットワークにおける交通状況の変化
配車システムにおける車両の位置や状態の変化
可動ノード
定義: 配車システムなどの特定の文脈で、位置や状態が時間とともに変化するノード(多くの場合、車両やタクシーなど)を指す言葉として使われることが多いです。
特徴:
ダイナミックグラフにおける「ノード」の一種であり、時間変化するグラフ構造を形成します。
配車システムのように、場所や状態が変化する実体(車両)を表現するのに適しています。
可動ノードの位置、状態、移動履歴などを考慮して、効率的な配車計画を立てる必要があります。
例:
タクシー配車システムにおけるタクシーの位置、乗客の有無
配送トラックの配送状況
ドローンによる配送システムにおけるドローンの位置
可動ノードとダイナミックグラフの関係
可動ノードは、ダイナミックグラフを構成する要素の一つです。
配車システムにおいて、車両(可動ノード)の移動や状態の変化は、ダイナミックグラフのエッジやノードの属性変化として捉えることができます。
ダイナミックグラフの分析手法やアルゴリズムは、可動ノードを考慮した配車問題の解決に応用できます。
まとめ
「可動ノード」は、特に配車システムのような特定の文脈で使われる言葉ですが、その本質はダイナミックグラフの概念と深く関連しています。両者とも時間とともに変化するグラフ構造を扱い、現実世界の複雑なシステムをモデル化するのに役立ちます。
したがって、配車における「可動ノード」をダイナミックグラフの概念で捉えることは、より広い視点で問題を理解し、適切な分析や解決策を見つける上で非常に有益です。
もしさらに具体的な質問があれば、遠慮なくお尋ねください。
すると倉庫の在庫問題、製造スケジューリング、N対N配車は全て同じモデルで表現できる事になる。