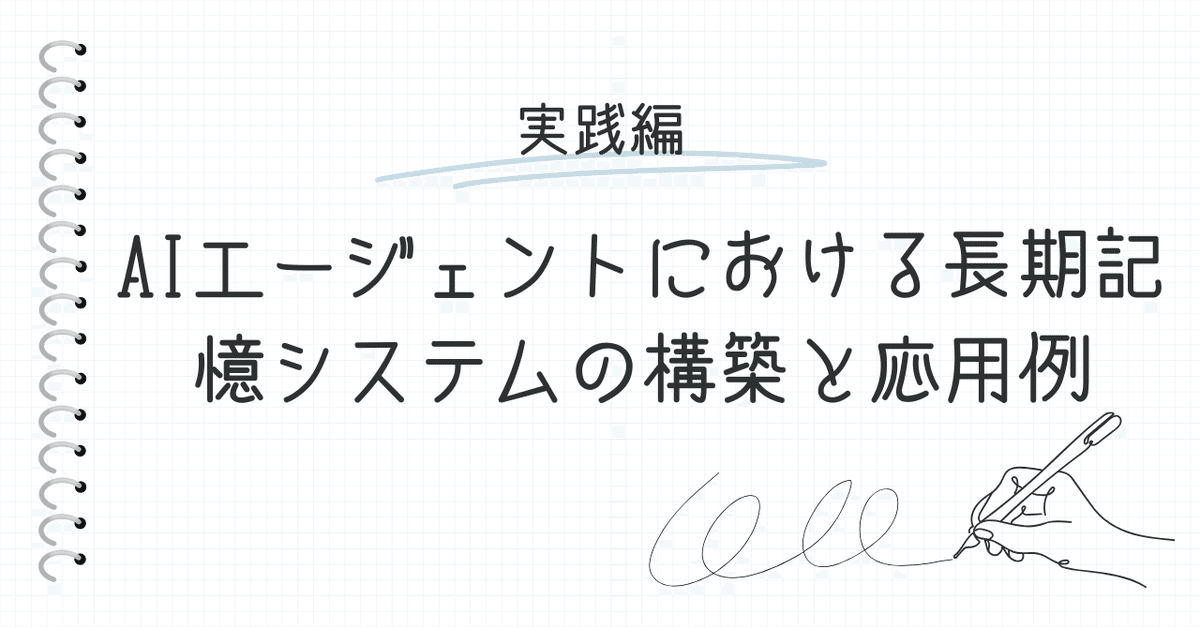
AIエージェントにおける長期記憶システムの構築と応用例」
はじめに
AIアシスタントの進化に伴い、長期的な記憶機能を備えることは、ユーザーエクスペリエンスを大幅に向上させる要因となります。例えば、ユーザーの好みや過去の対話内容を記憶することで、よりパーソナライズされた応答を提供できます。本記事では、Atomic Agentsフレームワークを用いて、シンプルで拡張性のある長期記憶システムの実装方法を解説します。
目標として、以下を達成します:
長期記憶の基本的な仕組みの理解
フレームワークを使用した実践的な実装
今後の応用可能性の提示
これにより、読者は自身のAIシステムに長期記憶を追加するための具体的な手法を学ぶことができます。
長期記憶の基礎
AIにおける長期記憶は、特定の条件下で極めて重要な役割を果たします。以下のようなシナリオでは、長期記憶の機能が特に有用です:
ユーザーの好みや履歴の保持:たとえば、過去の注文履歴や頻繁に使用するフレーズを記憶することで、ユーザーに最適化された体験を提供できます。
複雑なタスクの管理:プロジェクトの進行状況やタスクの依存関係を記憶することで、効率的なタスク管理を実現します。
対話の文脈を維持:長い会話の中で重要なポイントを記憶し、より関連性の高い応答を生成します。
これを実現するためには、以下の基本概念を理解する必要があります:
永続性:記憶されたデータが再起動後も失われないようにする必要があります。
検索性:特定の記憶を容易に検索し、利用できる仕組みが必要です。
柔軟性:記憶の種類やフォーマットが柔軟であり、さまざまな用途に対応できることが求められます。
次のセクションでは、これらの基礎をどのようにAtomic Agentsフレームワークを用いて実現するかを詳しく説明します。
Atomic Agentsは、AIエージェントを迅速かつ効率的に構築するための開発者向けフレームワークです。このフレームワークは、モジュール化された設計により、拡張性とカスタマイズ性を備えています。
主な特徴:
モジュール設計:各コンポーネントが独立しており、必要に応じて追加や変更が可能です。
簡潔な開発プロセス:厳密な入力および出力スキーマを使用して、エージェントやツールを簡単に連携できます。
長期記憶の統合:記憶の保存、検索、利用を容易に行えるため、パーソナライズされた体験を提供します。
このフレームワークを活用することで、以下の利点が得られます:
効率的なプロトタイピング:新しいエージェントの迅速な構築が可能です。
再利用性:既存のコンポーネントを別のプロジェクトに転用できます。
保守性:コードの可読性が高く、エラーの特定や修正が容易です。
Atomic Agentsを利用した具体的なコード例。
from typing import Literal
from pydantic import Field
from datetime import datetime, timezone
from atomic_agents.lib.base.base_io_schema import BaseIOSchema
class BaseMemory(BaseIOSchema):
"""Base class for all memory types"""
content: str = Field(..., description="Content of the memory")
timestamp: str = Field(
default_factory=lambda: datetime.now(timezone.utc).isoformat(),
description="ISO format timestamp of when the memory was created",
)
class CoreBioMemory(BaseMemory):
"""Core biographical information about the user"""
memory_type: Literal["core_bio"] = Field(default="core_bio")
class EventMemory(BaseMemory):
"""Information about significant events or experiences"""
memory_type: Literal["event"] = Field(default="event")
class WorkProjectMemory(BaseMemory):
"""Information about work projects and tasks"""
memory_type: Literal["work_project"] = Field(default="work_project")
次のセクションでは、これらのフレームワークとモデルを使った記憶保存およびクエリプロセスについて詳しく解説します。
記憶モデルの構築
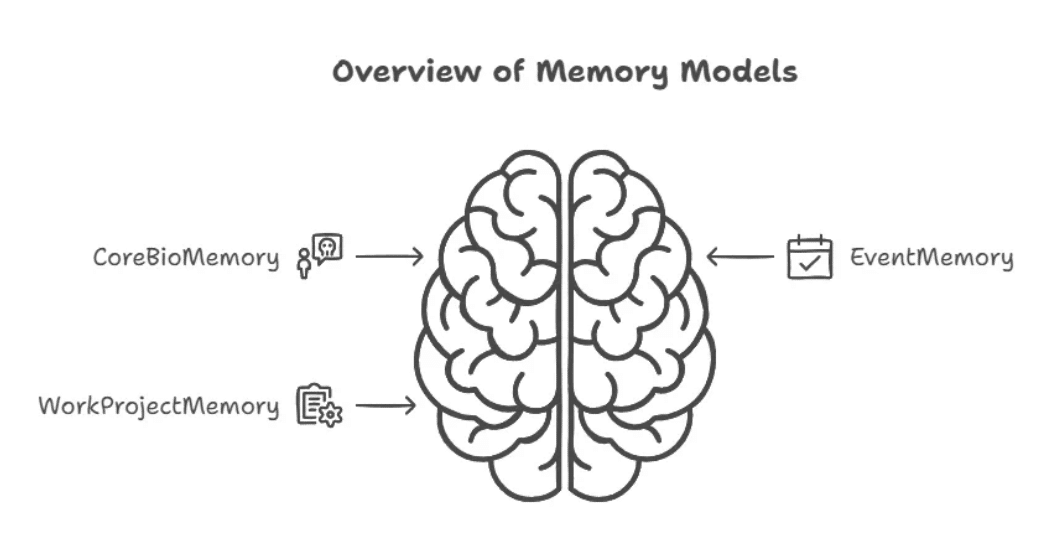
長期記憶システムを実現するためには、適切な記憶モデルを設計することが重要です。Atomic Agentsでは、以下の3つの基本的な記憶モデルを用意しています:
CoreBioMemory:ユーザーの基本的な情報(背景、好み、特性など)を保存します。
EventMemory:重要な出来事や経験を記録します。
WorkProjectMemory:プロジェクトやタスクに関する情報を追跡します。
これらのモデルは、各情報タイプに特化しており、それぞれの記憶が特定の「memory_type」によって識別されます。このアプローチにより、記憶の分類と検索が効率化されます。
以下は、記憶モデルの実装例です:
from typing import Literal
from pydantic import Field
from datetime import datetime, timezone
from atomic_agents.lib.base.base_io_schema import BaseIOSchema
class BaseMemory(BaseIOSchema):
"""Base class for all memory types"""
content: str = Field(..., description="Content of the memory")
timestamp: str = Field(
default_factory=lambda: datetime.now(timezone.utc).isoformat(),
description="ISO format timestamp of when the memory was created",
)
class CoreBioMemory(BaseMemory):
"""Core biographical information about the user"""
memory_type: Literal["core_bio"] = Field(default="core_bio")
class EventMemory(BaseMemory):
"""Information about significant events or experiences"""
memory_type: Literal["event"] = Field(default="event")
class WorkProjectMemory(BaseMemory):
"""Information about work projects and tasks"""
memory_type: Literal["work_project"] = Field(default="work_project")
カスタマイズ可能性
これらの基本モデルを元に、自身のプロジェクトに適した記憶モデルを自由に設計できます。たとえば、新しい「HealthMemory」タイプを追加して、健康状態や運動履歴を記録することも可能です。この柔軟性により、さまざまなユースケースに対応した記憶システムを構築できます。
次のセクションでは、これらの記憶モデルをどのように保存および取得するかを詳しく説明します。
記憶の保存と取得
記憶を保存し、必要に応じて取得することは、長期記憶システムの中核を成します。Atomic Agentsでは、以下の手法を利用してこれを実現します:
ChromaDBを利用した記憶保存
ChromaDBは、記憶データをベクトル形式で保存するためのデータベースです。これにより、高速かつ効率的な検索が可能となります。
各記憶は、メタデータと共に保存され、後で容易に検索できるよう設計されています。
記憶クエリツールの活用方法
記憶の検索には、Atomic Agentsが提供する「記憶クエリツール」を使用します。このツールは、特定のクエリに基づいて関連する記憶を検索し、適切な形式で返します。
クエリ結果は、適切なメモリタイプ(CoreBioMemory, EventMemory, WorkProjectMemoryなど)にマッピングされます。
記憶保存と取得の主なコード例です:
from pydantic import Field
from atomic_agents.lib.base.base_tool import BaseTool, BaseToolConfig
from atomic_agents.lib.base.base_io_schema import BaseIOSchema
from chat_with_memory.services.chroma_db import ChromaDBService
from chat_with_memory.tools.memory_models import (
BaseMemory,
CoreBioMemory,
EventMemory,
WorkProjectMemory,
)
class MemoryStoreInputSchema(BaseIOSchema):
"""Schema for storing memories"""
memory: BaseMemory = Field(..., description="Memory to store")
class MemoryStoreOutputSchema(BaseIOSchema):
"""Schema for memory storage output"""
memory: BaseMemory = Field(..., description="Stored memory")
class MemoryStoreConfig(BaseToolConfig):
"""Configuration for the MemoryStoreTool"""
collection_name: str = Field(
default="chat_memories", description="Name of the ChromaDB collection"
)
persist_directory: str = Field(
default="./chroma_db", description="Directory to persist ChromaDB data"
)
class MemoryStoreTool(BaseTool):
"""Tool for storing chat memories using ChromaDB"""
input_schema = MemoryStoreInputSchema
output_schema = MemoryStoreOutputSchema
def __init__(self, config: MemoryStoreConfig = MemoryStoreConfig()):
super().__init__(config)
self.db_service = ChromaDBService(
collection_name=config.collection_name,
persist_directory=config.persist_directory,
)
def run(self, params: MemoryStoreInputSchema) -> MemoryStoreOutputSchema:
"""Store a new memory in ChromaDB"""
memory = params.memory
self.db_service.add_documents(
documents=[memory.content],
metadatas=[{"timestamp": memory.timestamp, "memory_type": memory.memory_type}],
)
return MemoryStoreOutputSchema(memory=memory)
class MemoryQueryInputSchema(BaseIOSchema):
"""Schema for querying memories"""
query: str = Field(..., description="Query string to find relevant memories")
n_results: int = Field(default=5, description="Number of results to return")
class MemoryQueryOutputSchema(BaseIOSchema):
"""Schema for memory query output"""
results: list = Field(..., description="List of retrieved memories")
class MemoryQueryTool(BaseTool):
"""Tool for querying memories from ChromaDB"""
input_schema = MemoryQueryInputSchema
output_schema = MemoryQueryOutputSchema
def __init__(self, config: MemoryStoreConfig = MemoryStoreConfig()):
super().__init__(config)
self.db_service = ChromaDBService(
collection_name=config.collection_name,
persist_directory=config.persist_directory,
)
def run(self, params: MemoryQueryInputSchema) -> MemoryQueryOutputSchema:
"""Query memories from ChromaDB"""
query_results = self.db_service.query(
query_text=params.query,
n_results=params.n_results
)
return MemoryQueryOutputSchema(results=query_results)
これらのコードは、記憶の保存と取得の基本的なワークフローを示しています。次のセクションでは、これらの記憶機能をエージェントに統合する方法を説明します。
エージェントの統合
記憶機能を実際のエージェントに統合することで、ユーザーとのやり取りがより自然で効果的になります。以下の2つの主要なエージェントが統合の鍵を握ります:
メモリ形成エージェント
ユーザーとの対話内容を分析し、長期的に重要な情報を特定します。
必要な情報を選別し、適切な形式で記憶として保存します。
チャットエージェント
ユーザーの現在の問い合わせや状況に基づいて、保存された記憶を活用します。
保存された記憶をもとに、より関連性の高いパーソナライズされた応答を生成します。
メモリ形成エージェントとチャットエージェントの統合例です:
from atomic_agents.agents.base_agent import BaseAgent, BaseAgentConfig, BaseIOSchema
from atomic_agents.lib.components.system_prompt_generator import SystemPromptGenerator
from atomic_agents.lib.components.agent_memory import AgentMemory
from pydantic import Field
class MemoryFormationInputSchema(BaseIOSchema):
"""Input schema for the Memory Formation Agent."""
last_user_msg: str = Field(..., description="The last message from the user in the conversation")
last_assistant_msg: str = Field(..., description="The last message from the assistant in the conversation")
class MemoryFormationOutputSchema(BaseIOSchema):
"""Output schema for the Memory Formation Agent."""
memories_to_store: list = Field(..., description="List of memories to store")
class MemoryFormationAgent(BaseAgent):
"""Agent for forming memories based on conversations."""
input_schema = MemoryFormationInputSchema
output_schema = MemoryFormationOutputSchema
def __init__(self, config: BaseAgentConfig):
super().__init__(config)
self.memory = AgentMemory(max_messages=10)
def run(self, params: MemoryFormationInputSchema) -> MemoryFormationOutputSchema:
"""Analyze conversation and form new memories."""
# Analyze conversation context
new_memory = {
"content": params.last_user_msg,
"timestamp": "2024-12-25T10:00:00Z",
"type": "event"
}
return MemoryFormationOutputSchema(memories_to_store=[new_memory])
class ChatAgentInputSchema(BaseIOSchema):
"""Input schema for the Chat Agent."""
message: str = Field(..., description="User's message")
class ChatAgentOutputSchema(BaseIOSchema):
"""Output schema for the Chat Agent."""
response: str = Field(..., description="Response from the chat agent")
class ChatAgent(BaseAgent):
"""Agent for generating chat responses using memory."""
input_schema = ChatAgentInputSchema
output_schema = ChatAgentOutputSchema
def __init__(self, config: BaseAgentConfig):
super().__init__(config)
self.memory = AgentMemory(max_messages=10)
def run(self, params: ChatAgentInputSchema) -> ChatAgentOutputSchema:
"""Generate a response based on user's message and memory."""
memory_context = "Relevant past memories"
response = f"Using memory context: {memory_context}, I respond to: {params.message}"
return ChatAgentOutputSchema(response=response)
このコードは、エージェントがどのように記憶を活用してユーザーとの会話を強化するかを示しています。次のセクションでは、これらのエージェントを使用した具体的な実装例を紹介します。
実装の例
ここでは、メモリ形成エージェントとチャットエージェントを組み合わせた実際の実装例を紹介します。以下のコードは、ユーザーとの対話の流れを示します。
コード例
from atomic_agents.agents.base_agent import BaseAgentConfig
from memory_formation_agent import MemoryFormationAgent, MemoryFormationInputSchema
from chat_agent import ChatAgent, ChatAgentInputSchema
# エージェントの設定
memory_formation_config = BaseAgentConfig(client=None, model="gpt-4-mini")
chat_agent_config = BaseAgentConfig(client=None, model="gpt-4-mini")
# エージェントのインスタンス作成
memory_formation_agent = MemoryFormationAgent(memory_formation_config)
chat_agent = ChatAgent(chat_agent_config)
# ユーザーとの対話の流れ
user_message = "昨日の会議の要点を覚えていますか?"
assistant_message = "もちろんです。どの部分について詳しく知りたいですか?"
# メモリ形成エージェントで新しい記憶を保存
memory_input = MemoryFormationInputSchema(
last_user_msg=user_message,
last_assistant_msg=assistant_message
)
memory_output = memory_formation_agent.run(memory_input)
print("保存された記憶:", memory_output.memories_to_store)
# チャットエージェントで応答生成
chat_input = ChatAgentInputSchema(message=user_message)
chat_output = chat_agent.run(chat_input)
print("チャットエージェントの応答:", chat_output.response)
主なプロセス
ユーザーからのメッセージを受け取り、過去の会話履歴と照らし合わせます。
メモリ形成エージェントを使用して、重要な情報を記憶に保存します。
チャットエージェントを使用して、記憶に基づいた関連性の高い応答を生成します。
このコードは、Atomic Agentsを利用してどのように記憶システムを構築し、統合できるかを示しています。次のセクションでは、これらの機能の応用可能性と今後の展望について解説します。
応用と今後の展望
長期記憶システムは、さらに多くの応用が期待されます。以下は、そのいくつかの例です:
システムの拡張性
新しいメモリタイプの追加:たとえば、感情状態や健康データを記録するための「EmotionMemory」や「HealthMemory」などの追加が考えられます。
複雑なタスク管理の統合:複数の記憶モデルを組み合わせることで、より包括的なシステムを構築できます。
新しい技術との連携
自然言語処理技術の向上:より高度な言語理解を実現するために、最新のモデルを統合します。
IoTデバイスとの連携:センサーやデバイスからのデータを直接記憶に取り込むことで、リアルタイムの状況把握が可能になります。
応用例
ヘルスケア:患者の病歴や治療計画を記憶し、医療従事者に適切な情報を提供します。
教育:学習者の進捗や理解度を記憶し、パーソナライズされた教育体験を提供します。
ビジネス:顧客の購買履歴やフィードバックを記憶し、カスタマイズされたサービスを提供します。
今後、これらの応用可能性を探求し、より多機能で柔軟なシステムの構築が期待されます。次のセクションでは、この記事のまとめを行い、今後の課題について触れます。
結論
本記事では、Atomic Agentsフレームワークを活用した長期記憶システムの構築方法について解説しました。以下はその要点です:
基本概念の理解:長期記憶がどのようにAIエージェントの能力を向上させるかを学びました。
実装手順の詳細:記憶モデルの構築から保存・取得、エージェントの統合までの具体的な手法を示しました。
応用可能性の提示:教育、ヘルスケア、ビジネスなど、さまざまな分野での活用方法を提案しました。
今後の課題としては、次のようなポイントが挙げられます:
記憶の正確性と関連性の向上:保存された記憶の品質を向上させるためのアルゴリズムの開発。
データセキュリティの強化:特に個人データを扱う場合、プライバシー保護を徹底する仕組みの構築。
ユーザー体験の最適化:対話の自然さや応答のパーソナライズ性をさらに向上させる。
Atomic Agentsフレームワークを活用することで、長期記憶を持つAIエージェントの構築がより身近で簡単になります。この技術を活用し、新たなAIエージェントの可能性を探求してください。
いいなと思ったら応援しよう!
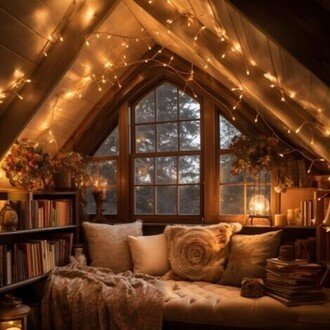